Common Mistakes When Implementing Android Compass Code
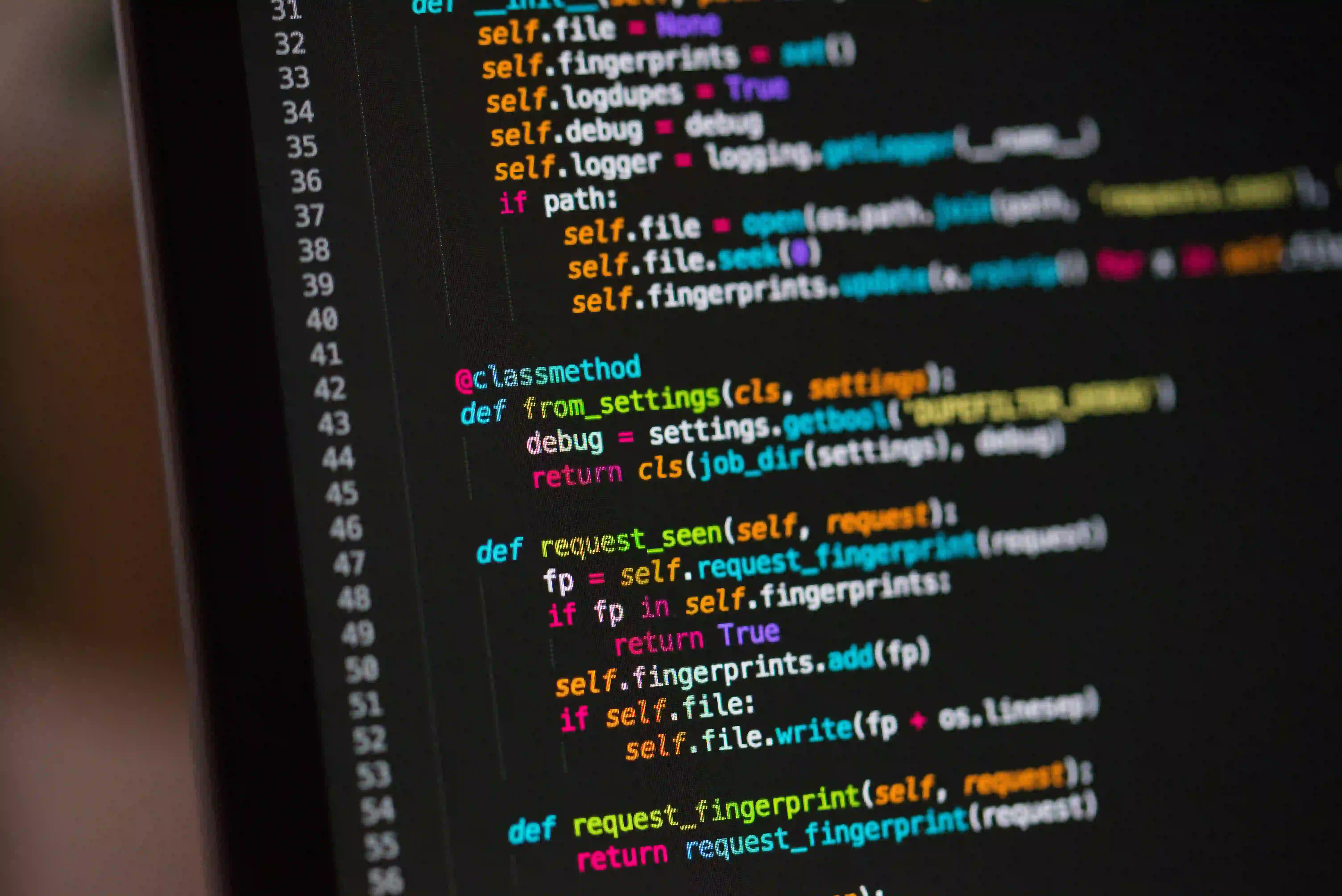
Common Mistakes When Implementing Android Compass Code
Creating a compass app on Android can be a fun and informative project for developers at all levels. However, navigating through the code to ensure that you have an accurate and responsive compass system can lead to several common pitfalls. In this blog post, we will explore these mistakes in detail and provide exemplary code snippets to guide you toward successful implementation.
Table of Contents
- Understanding the Fundamentals
- Mistake 1: Overlooking Permissions
- Mistake 2: Ignoring Sensor Accuracy
- Mistake 3: Failing to Handle Sensor Events Properly
- Mistake 4: Not Calibrating the Magnetometer
- Mistake 5: Neglecting User Experience
- Conclusion
Understanding the Fundamentals
Before we jump into the common mistakes, let's review what you need to build a compass in Android.
A compass relies primarily on the magnetometer and the accelerometer to determine your orientation relative to the Earth's magnetic field. To implement this, you typically set up listeners for these sensors and use the data they provide to calculate your heading.
Basic Compass Code Structure
Here's a simple outline of how setting up sensors may look:
public class CompassActivity extends AppCompatActivity implements SensorEventListener {
private SensorManager sensorManager;
private Sensor accelerometer;
private Sensor magnetometer;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_compass);
sensorManager = (SensorManager) getSystemService(SENSOR_SERVICE);
accelerometer = sensorManager.getDefaultSensor(Sensor.TYPE_ACCELEROMETER);
magnetometer = sensorManager.getDefaultSensor(Sensor.TYPE_MAGNETIC_FIELD);
}
@Override
protected void onResume() {
super.onResume();
sensorManager.registerListener(this, accelerometer, SensorManager.SENSOR_DELAY_UI);
sensorManager.registerListener(this, magnetometer, SensorManager.SENSOR_DELAY_UI);
}
@Override
protected void onPause() {
super.onPause();
sensorManager.unregisterListener(this);
}
// Implement SensorEventListener methods...
}
This code initializes the Sensor Manager and registers listeners for the accelerometer and magnetometer.
Mistake 1: Overlooking Permissions
One of the most common mistakes in Android development is neglecting to declare necessary permissions in your AndroidManifest.xml
. While the compass itself does not require special permissions beyond the normal operation of sensors, ensuring accurate functioning may call for access to location services.
If location access is needed, add the following to your manifest:
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
While this isn’t mandatory for basic compass functionality, it is useful if you later expand your app to display orientation alongside location features.
Mistake 2: Ignoring Sensor Accuracy
Developers often overlook the accuracy of the sensors. The SensorManager
can provide information about the accuracy of the sensor readings. Ignoring this can lead to misinterpretations:
@Override
public void onSensorChanged(SensorEvent event) {
if (event.sensor.getType() == Sensor.TYPE_MAGNETIC_FIELD) {
// Handle magnetic field data
} else if (event.sensor.getType() == Sensor.TYPE_ACCELEROMETER) {
// Handle accelerometer data
}
}
@Override
public void onAccuracyChanged(Sensor sensor, int accuracy) {
if (accuracy == SensorManager.SENSOR_STATUS_UNRELIABLE) {
// Handle unreliability
Log.w("Compass", "Sensor accuracy is unreliable. Calibration needed!");
}
}
Adding accuracy handling can help manage situations where sensor data fluctuates significantly, ensuring more stable outputs.
Mistake 3: Failing to Handle Sensor Events Properly
Implementing the SensorEventListener
interface involves handling sensor data correctly. A common mistake is processing both sensors’ data inefficiently:
private float[] gravity;
private float[] geomagnetic;
@Override
public void onSensorChanged(SensorEvent event) {
if (event.sensor.getType() == Sensor.TYPE_ACCELEROMETER) {
gravity = event.values.clone();
} else if (event.sensor.getType() == Sensor.TYPE_MAGNETIC_FIELD) {
geomagnetic = event.values.clone();
}
if (gravity != null && geomagnetic != null) {
float[] R = new float[9];
float[] I = new float[9];
if (SensorManager.getRotationMatrix(R, I, gravity, geomagnetic)) {
float orientation[] = new float[3];
SensorManager.getOrientation(R, orientation);
float azimuth = Math.toDegrees(orientation[0]);
// Process azimuth value...
}
}
}
Here, the key point is to ensure that you only calculate the heading when both sensor data are available to avoid erroneous outputs.
Mistake 4: Not Calibrating the Magnetometer
Magnetometers can be affected by nearby metallic objects and magnitudes, leading to inaccuracies. Many apps implement compass calibration, but it often gets overlooked during development.
If you're using the magnetometer readings directly without taking the user's environment into account, you may mislead the user. Make sure to add instructions to calibrate the compass by moving the device in a figure-eight motion for best results.
Mistake 5: Neglecting User Experience
While technical implementation is crucial, the user experience (UX) is equally important. An efficient UX includes a clear design, smooth animations, and simple instructions for users.
Here are some UX considerations:
- Visual Feedback: Show the current direction using an intuitive arrow or graphical representation.
- Calibration instructions: Make sure users know how to calibrate the compass when prompted.
<ImageView
android:id="@+id/compass_image"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
In your code, you can update this ImageView
to rotate based on the calculated azimuth:
compassImage.setRotation(-azimuth); // Negate to align with true north
Lessons Learned
Developing a compass app incorporates several nuances that, when ignored, can lead to frustrating experiences for users. From managing sensor permissions to ensuring accuracy and enhancing user experience, being aware of these common mistakes can set your app on the path to success.
As you develop your compass application, keep iterating, testing, and refining your strategy. Happy coding!
For more information on how to implement sensors effectively in your app, visit the Android Developers Guide.
Feel free to share your experiences with compass app development in the comments below! If you found this post helpful, don’t forget to check out our other Android programming articles.