Common Mistakes to Avoid When Starting Android Development
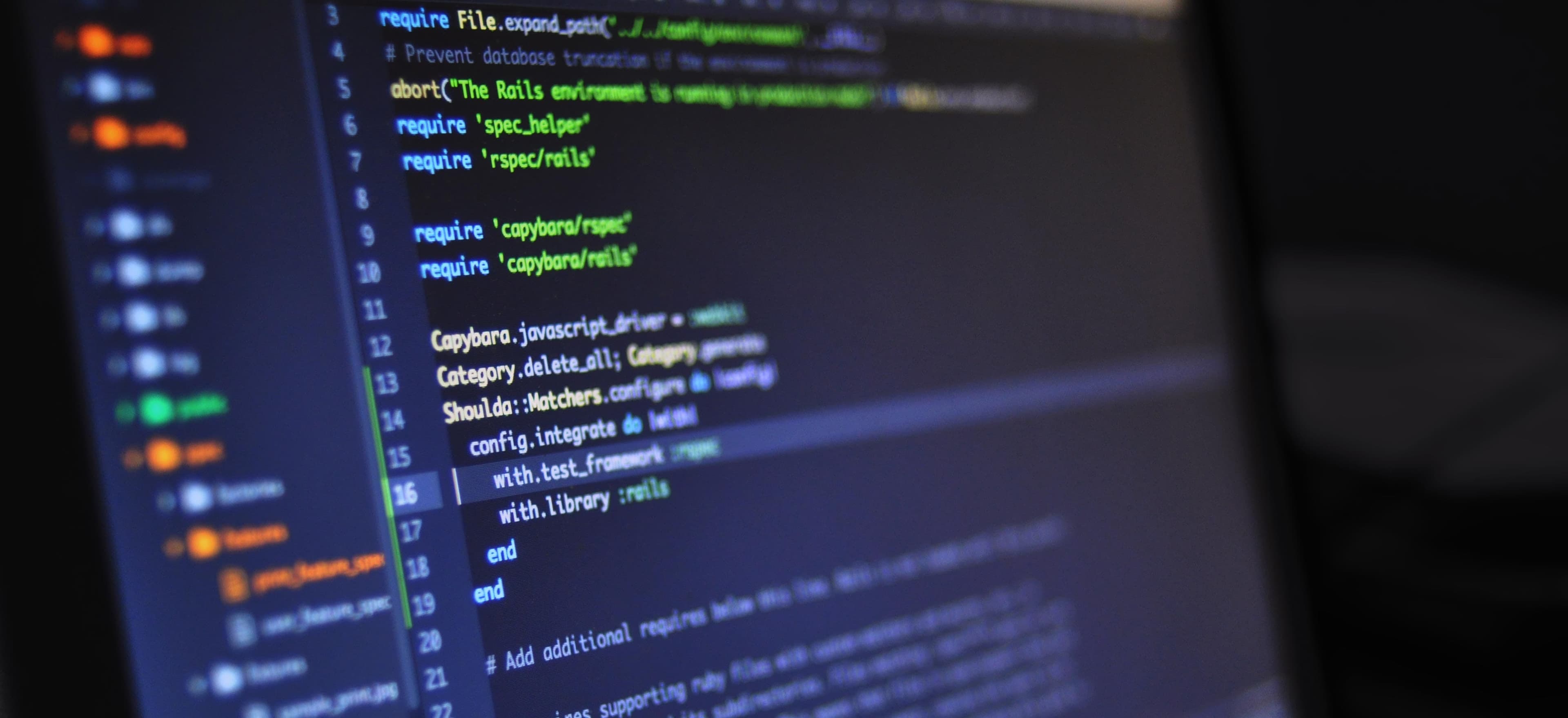
- Published on
Common Mistakes to Avoid When Starting Android Development
Android development can be an exciting and rewarding journey, but it is also fraught with potential pitfalls. As a beginner, you might encounter numerous challenges that can hinder your progress or lead to frustration. This blog post aims to highlight common mistakes made by novice developers in the realm of Android development and provide actionable insights to help you avoid them.
1. Ignoring Official Documentation
One of the most critical errors that beginners make is ignoring the official documentation. The Android developer documentation is rich with information about the Android SDK, APIs, best practices, and more.
Why This is Important:
Understanding the official guidelines can save you time and effort in the long run. They help you avoid deprecated methods and provide insights into new features.
Example:
- Reading the Android Developer Guides will give you a foundational understanding of various components, such as Activities, Fragments, and Services.
Actionable Tip:
Always refer to the documentation when faced with challenges or when exploring new features.
2. Not Following the Android Architecture Guidelines
Creating an Android app without understanding the architecture components can lead to a tightly-coupled codebase. It's crucial to employ a clear architecture for your app.
Why This is Important:
Using a proper architecture (like MVVM or MVP) improves code readability and maintainability, making it easier to test and develop in the long run.
Example:
Using MVVM architecture, you might structure your code like this:
// ViewModel Class
public class MyViewModel extends ViewModel {
private MutableLiveData<List<String>> myData;
public LiveData<List<String>> getData() {
if (myData == null) {
myData = new MutableLiveData<>();
loadData();
}
return myData;
}
private void loadData() {
// Fetch data asynchronously
}
}
Actionable Tip:
Familiarize yourself with Architecture Components like LiveData and ViewModel. They can be beneficial in maintaining separation of concerns.
3. Skipping Unit Testing
Many beginners neglect the importance of unit testing their code. While it may seem tedious at the outset, skipping this step can lead to a world of hurt when bugs arise.
Why This is Important:
Testing your code ensures reliability and functionality. It also prevents future regressions when new features are added.
Example: Here’s how you might write a simple unit test using JUnit:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class ExampleUnitTest {
@Test
public void addition_isCorrect() {
assertEquals(4, 2 + 2);
}
}
Actionable Tip:
Integrate unit testing into your workflow early. Use frameworks like JUnit and Mockito for effective testing.
4. Using Inefficient Layouts
Layout inefficiencies can lead to sluggish applications. Beginners often use nested layouts without realizing their impact on performance.
Why This is Important:
Complex layouts not only affect application speed but also create potential issues during UI rendering.
Example:
Instead of using multiple nested LinearLayout
, consider using a ConstraintLayout
:
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/myTextView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
Actionable Tip:
Always aim to use ConstraintLayout
as a primary layout. It leads to better performance and easier design.
5. Hardcoding Strings
Beginners often hardcode strings directly in code. While it may seem convenient, this can lead to maintainability issues.
Why This is Important:
Hardcoded strings make localization or updates cumbersome. A single change necessitates looking through multiple code files.
How to Do It Right:
Instead, store strings in the strings.xml
file:
<resources>
<string name="app_name">My App</string>
<string name="welcome_message">Welcome to My App!</string>
</resources>
Then reference them in your code:
String welcomeMessage = getString(R.string.welcome_message);
Actionable Tip:
Always use strings.xml
for any textual content. This aids in localization and keeps your code cleaner.
6. Neglecting Performance Optimization
Another common mistake is neglecting performance optimization in Android apps. Beginners might focus solely on functionality, overlooking how efficiently an app runs.
Why This is Important:
Poor performance can lead to a bad user experience, resulting in uninstalls and negative reviews.
Example Optimization Techniques Include:
- Caching data to minimize network requests.
- Using background threads for CPU-intensive tasks.
- Avoiding overdraw by eliminating unnecessary views.
Actionable Tip:
Familiarize yourself with Android profiling tools to identify bottlenecks and improve performance.
7. Underestimating the Importance of Permissions
Understanding Android permissions is crucial. Beginners often neglect handling app permissions properly.
Why This is Important:
Without correct permission handling, your app may crash or behave unpredictably. Also, proper permission handling enhances user trust.
Example:
You can request permissions dynamically like this:
if (ContextCompat.checkSelfPermission(this,
Manifest.permission.READ_CONTACTS)
!= PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this,
new String[]{Manifest.permission.READ_CONTACTS},
REQUEST_CONTACT);
}
Actionable Tip:
Always declare permissions in your AndroidManifest.xml
and handle them gracefully at runtime.
Wrapping Up
Starting on the right foot in Android development requires understanding common pitfalls. By being aware of these mistakes—such as ignoring documentation, not following architectural guidelines, or neglecting testing—you can streamline your learning process, create efficient applications, and ultimately elevate your development skills.
In conclusion, the road ahead may be challenging, but by avoiding these common mistakes and taking strategic steps, you can enhance your key competencies in Android development. Engage continuously with resources, stay updated with best practices, and keep coding. You can follow reputable sources like the Android Developers Blog for the latest trends and techniques.
With perseverance and the right mindset, your Android development journey will be successful and rewarding. Happy coding!