Speeding Up Swagger Documentation for Java Spring CRUD APIs
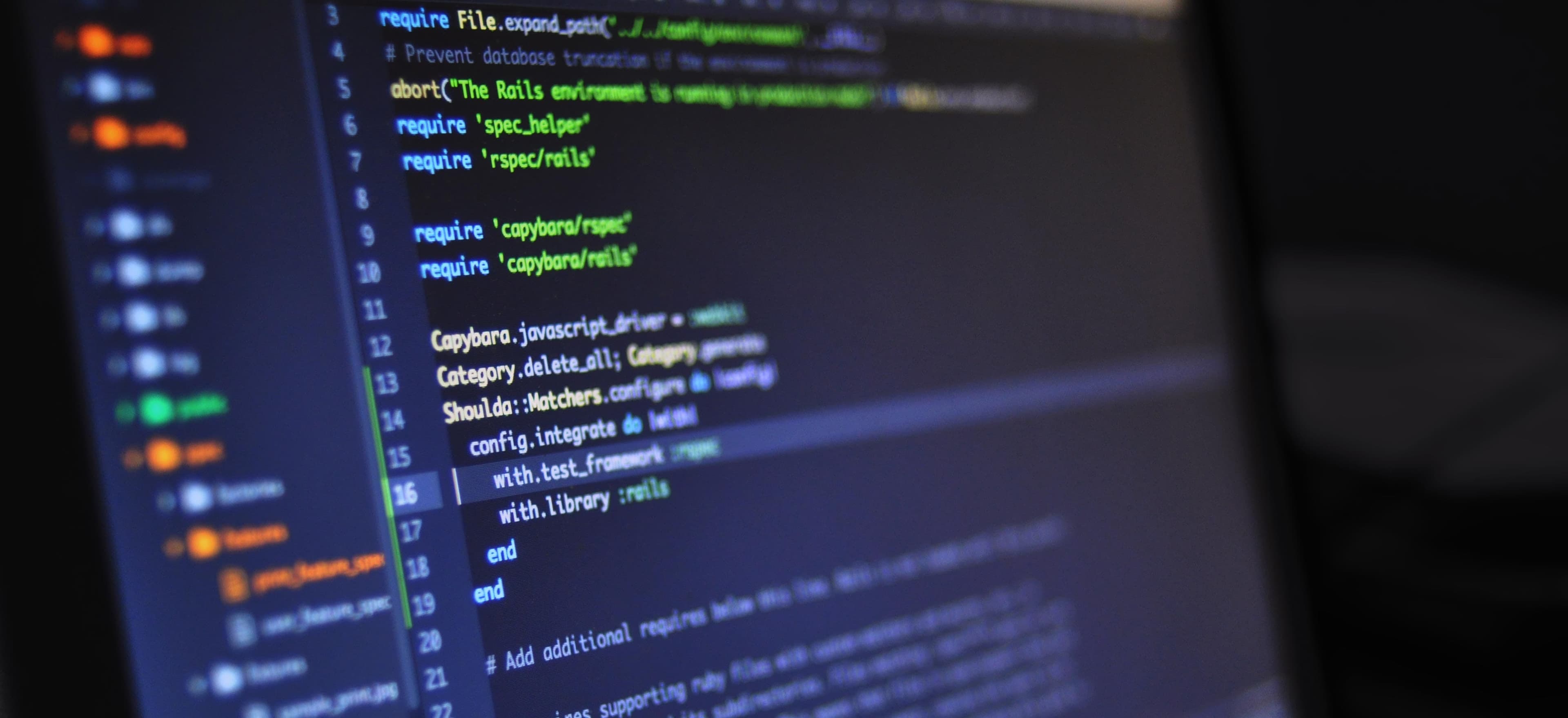
- Published on
Speeding Up Swagger Documentation for Java Spring CRUD APIs
Swagger, now known as OpenAPI, provides a powerful interface for designing and documenting APIs. It enhances collaboration and understanding among development teams and stakeholders. This blog post will guide you on speeding up the Swagger documentation process specifically for Java Spring CRUD APIs, making your API documentation a seamless experience.
Why Swagger Documentation?
API documentation is crucial for any software development lifecycle. Swagger offers several benefits:
- Interactive Documentation: Users can interact with your API directly from the documentation.
- Easy Testing: You can test API endpoints without additional tools.
- Standardization: Ensures a consistent format across all API endpoints.
- Code Generation: Automatically generate client libraries or server stubs.
In Spring applications, integrating Swagger is straightforward, but optimization can save time and improve efficiency.
Setting Up Swagger in a Spring Boot Application
Before we dive into speed optimization, let us ensure that your Spring Boot application is correctly set up with Swagger.
Here’s how to set up Swagger in a Spring Boot application:
Step 1: Add Dependencies
Add the following dependencies in your pom.xml
if you’re using Maven:
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-boot-starter</artifactId>
<version>3.0.0</version>
</dependency>
For Gradle users, add this in the build.gradle
file:
implementation 'io.springfox:springfox-boot-starter:3.0.0'
Step 2: Configure Swagger
Create a Swagger configuration class to customize the documentation output.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.any())
.build();
}
}
In this configuration, RequestHandlerSelectors.any()
scans all your controllers, while PathSelectors.any()
includes all the available endpoints. Adjust these selections based on your needs for enhanced specifications.
Step 3: Access Swagger UI
Run your Spring Boot application and navigate to http://localhost:8080/swagger-ui/
. You should see your API documentation displayed clearly.
Speeding Up the Documentation Process
Now that you have Swagger integrated, let's explore ways to speed up your Swagger documentation for CRUD APIs.
1. Leveraging Annotations
To enhance the speed of your documentation, leverage annotations provided by Swagger. Use annotations directly in your controller methods and models.
Here are the key annotations:
@Api
: Used at the class level to document the overall API.@ApiOperation
: Describes an individual API operation (method).@ApiParam
: Used for adding metadata to method parameters.@ApiResponses
: Documents expected responses for your API methods.
Example of Using Annotations
Here's a simple CRUD controller example:
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiParam;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@Api(value="User Management System")
@RestController
@RequestMapping("/api/users")
public class UserController {
@ApiOperation(value="Get all users")
@GetMapping
public List<User> getAllUsers() {
// method implementation
}
@ApiOperation(value="Create a new user")
@PostMapping
public User createUser(@ApiParam(value = "User object to be created") @RequestBody User user) {
// method implementation
}
@ApiOperation(value="Update an existing user")
@PutMapping("/{id}")
public User updateUser(@PathVariable Long id, @RequestBody User user) {
// method implementation
}
@ApiOperation(value="Delete a user")
@DeleteMapping("/{id}")
public void deleteUser(@PathVariable Long id) {
// method implementation
}
}
Using these annotations allows you to describe your API endpoints clearly, speeding up the documentation process. The metadata will also help other users understand how to interact with your API without diving deep into the code.
2. Customizing Responses
Documenting potential responses and error codes can significantly enhance the API documentation.
@ApiResponses(value = {
@ApiResponse(code = 200, message = "Successfully retrieved users"),
@ApiResponse(code = 404, message = "Users not found"),
@ApiResponse(code = 500, message = "Internal server error")
})
Adding these can help users effectively interpret the API's behavior under different conditions.
3. Organizing Tags
Grouping routes by tags allows users to navigate your documentation more efficiently. Use the @Api
annotation with the tags
parameter to categorize your operations:
@Api(tags = {"User Management"})
@RestController
@RequestMapping("/api/users")
public class UserController { ... }
4. Automating Model Documentation
Use @ApiModel
and @ApiModelProperty
annotations on your DTO classes for automatic documentation of request and response objects.
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
@ApiModel(description = "Details about the user")
public class User {
@ApiModelProperty(notes = "The unique ID of the user")
private Long id;
@ApiModelProperty(notes = "The name of the user")
private String name;
// Getters and setters
}
This ensures all aspects of your API are well documented without extensive manual effort.
5. Streamlining the Build Process
Consider using profiles in your application.yml to switch Swagger on and off and enable verbose API generation only during development. This streamlines deployment environments while ensuring clear documentation in development.
spring:
profiles:
active: dev
---
spring:
profiles: dev
swagger:
enabled: true
---
spring:
profiles: prod
swagger:
enabled: false
By managing Swagger visibility based on profiles, you can keep documentation relevant and not distract from production builds.
Final Thoughts
Optimizing Swagger documentation for your Java Spring CRUD APIs doesn't only make it faster; it improves usability and comprehension for your development and user communities alike.
- Annotations streamline documenting API functionalities.
- Organized responses and tags increase navigation efficiency.
- Model documentation accurately describes the structures being manipulated.
Improving documentation allows better collaboration amongst developers, testers, and users, leading to fewer misunderstandings and a more sustainable development environment.
Further Reading:
By adopting these strategies, you can enhance your Swagger documentation speed significantly, ensuring a robust and user-friendly API experience.