Streamlining Docker Deployments with Jib and Payara Errors
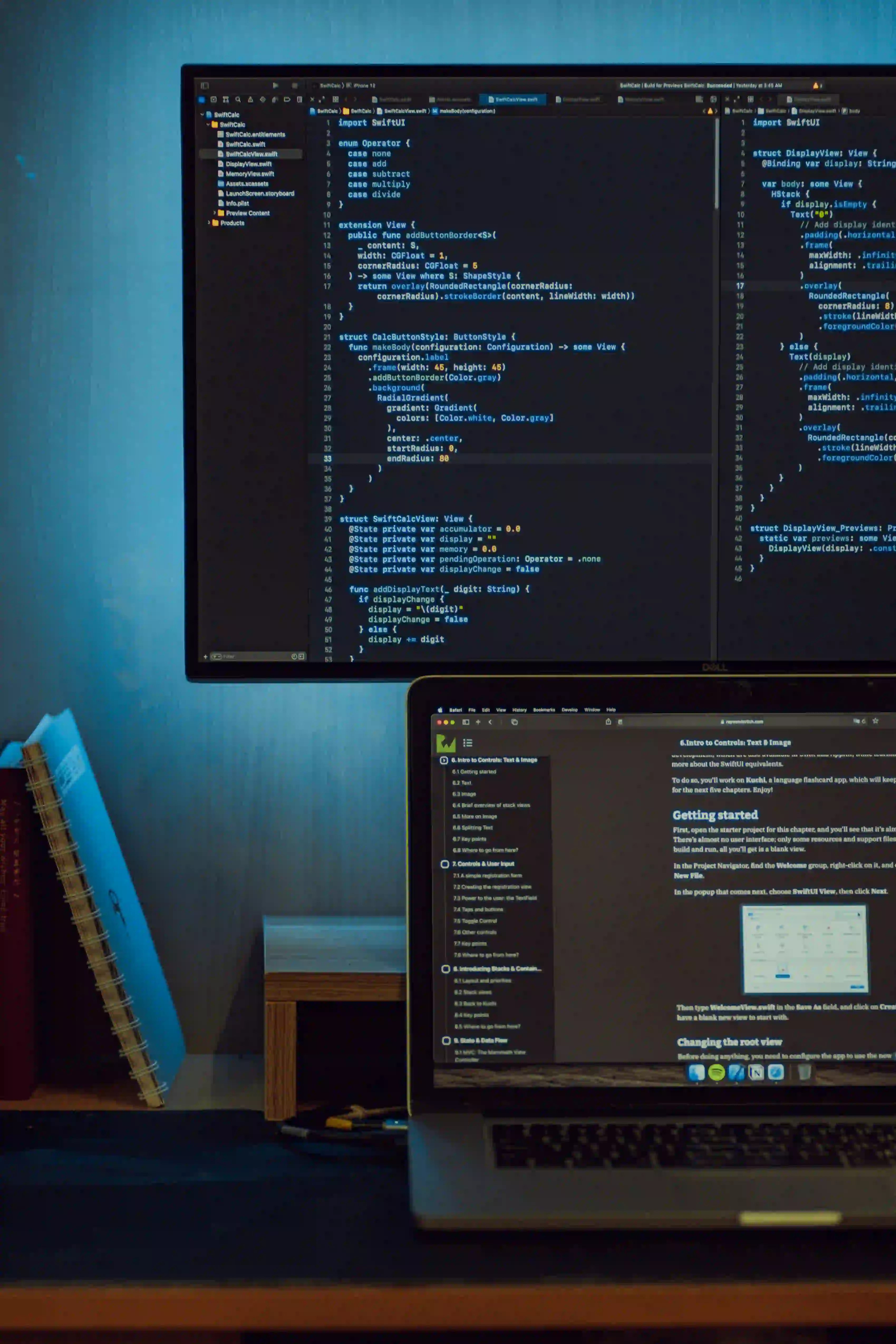
Streamlining Docker Deployments with Jib and Handling Payara Errors
In the modern software development landscape, effective containerization is essential for maintaining smooth application deployments. Docker has rapidly emerged as the go-to solution for developers, allowing applications to run consistently in different environments. When it comes to Java applications, using Jib for building Docker images directly from Maven or Gradle enhances the deployment process significantly. In conjunction with a robust server like Payara, you can achieve an efficient, reliable deployment pipeline.
This blog post will detail how to streamline your Docker deployments using Jib while addressing some common Payara errors you may encounter. We will provide clear code snippets and explanations to ensure you understand the 'why' behind each step.
Understanding Jib and Payara
What is Jib?
Jib is a tool designed for seamlessly building Docker images for Java applications. Unlike traditional Dockerfile usage, Jib allows developers to create optimized images directly from their build tools (Maven or Gradle), without needing a Docker daemon running or managing Dockerfiles.
Why Use Payara?
Payara Server is an enhanced version of GlassFish, which offers various features, including support for Java EE and microservices. It also comes with built-in support for container deployments, making it an ideal choice for hosting your Java applications in Docker containers.
Setting Up Your Environment
To get started, ensure you have the following:
- Java Development Kit (JDK) 8 or later.
- Maven or Gradle installed on your machine.
- Payara Server setup for your Java EE applications.
Build Your Project with Jib
Maven Configuration
First, we’ll configure a simple Java web application using Maven that you can deploy to Payara. Here’s a snippet of the pom.xml
file with Jib configuration:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>myapp</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<build>
<plugins>
<plugin>
<groupId>com.google.cloud.tools</groupId>
<artifactId>jib-maven-plugin</artifactId>
<version>3.3.1</version>
<configuration>
<to>
<image>myregistry/myapp</image>
</to>
</configuration>
</plugin>
</plugins>
</build>
</project>
Why Jib Plugin?
In this configuration:
- The
<packaging>
type is specified aswar
, denoting a web application. - The Jib Maven plugin is integrated to streamline the Docker image build process.
- The
<to>
section indicates the target image name.
Running Jib
To build and push the Docker image, navigate to your project directory in the command line and execute:
mvn compile jib:build
Gradle Configuration
If you're using Gradle, here’s how to configure the build.gradle
:
plugins {
id 'java'
id 'com.google.cloud.tools.jib' version '3.3.1'
}
group 'com.example'
version '1.0-SNAPSHOT'
jib {
to {
image = 'myregistry/myapp'
}
}
Benefits of Jib
Jib’s iterative build process ensures that layers are cached, so only modified files and dependencies are rebuilt. This results in faster deployment times without the overhead of managing Dockerfiles.
Deploying to Payara
To deploy your application on Payara, you typically would:
- Start Payara Server.
- Use the Payara admin console to deploy the application.
- Or deploy using the command line, which is ideal for automated CI/CD pipelines.
For example, to deploy via the command line to Payara, use:
asadmin deploy [path-to-your-war-file]
Common Payara Errors and Solutions
Even as you enjoy the advantages of Jib and Payara, you might face errors that can disrupt your deployment process. Here’s how to troubleshoot a few common mistakes.
Error: "class not found"
This error typically arises if the application cannot locate a required class at runtime.
Solution
Ensure that all dependencies are included in your pom.xml
or build.gradle
. In Jib, you can configure dependencies via:
<dependencies>
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>8.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
Error: "HTTP 404: Not Found"
This error likely indicates that the requested resource is not correctly deployed or the context path is not set correctly.
Solution
Verify that your application is properly deployed in Payara. Ensure your web.xml
file defines the correct context path, and check the Payara admin console for deployment messages.
Error: "Out of Memory Error"
If your application runs out of memory during deployment, you might have insufficient heap size allocated to the Payara Server.
Solution
Increase the JVM heap size by modifying the asenv.conf
file:
# For UNIX
AS_JAVA_OPTS=-Xmx2048m
# For Windows
set AS_JAVA_OPTS=-Xmx2048m
The Bottom Line
Streamlining your Docker deployments with Jib in tandem with Payara can revolutionize your Java application deployment workflow. Jib's direct integration with Maven and Gradle removes unnecessary steps in the Docker image creation process, while Payara allows effortless hosting of your applications. By understanding how to handle common Payara errors, you will be better equipped to maintain smooth operations and quickly address caching or configuration issues.
Don’t hesitate to explore further into Jib and Payara, as both tools offer extensive features that can enhance your deployment pipeline.
Relevant Links
- Explore the official Jib documentation for an in-depth understanding.
- Dive into the Payara user guide for tips on effective server management.
- Check out Containerizing Java Applications to learn more about best practices in containerization.
By continuously learning and refining these practices, you will elevate your deployment processes, ensure stability in your applications, and provide a superior experience for your end-users.