Mastering RabbitMQ: Overcoming Acknowledgment Confusion
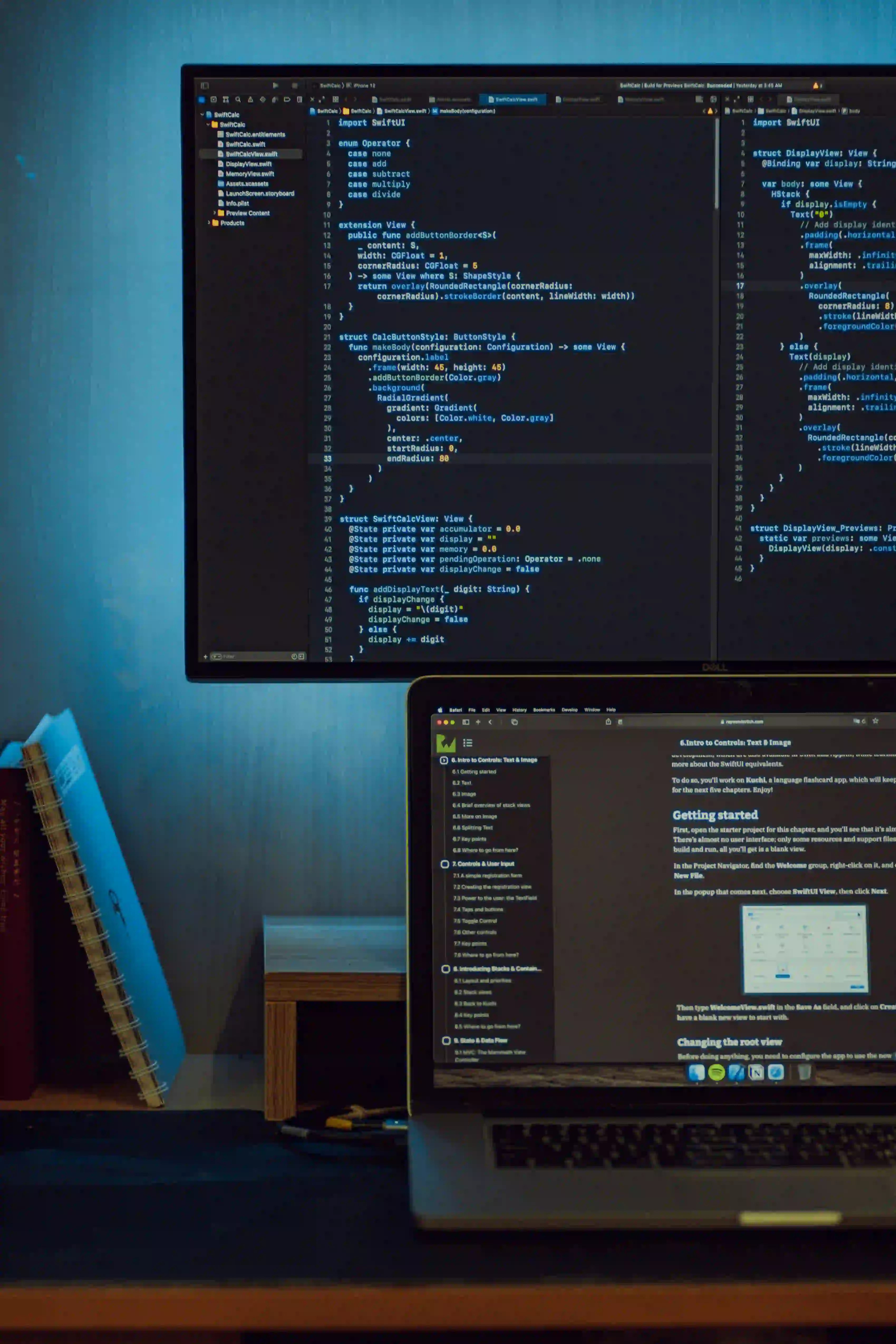
Mastering RabbitMQ: Overcoming Acknowledgment Confusion
RabbitMQ is one of the most popular message brokers used in modern applications. It facilitates communication between different layers of an application and helps to ensure message delivery. However, one of the most confusing aspects of RabbitMQ is its acknowledgment system. In this article, we will dive deep into the acknowledgment mechanism, explore why it is crucial, and provide code snippets illustrating best practices.
Understanding RabbitMQ Acknowledgments
RabbitMQ employs a message acknowledgment system to ensure reliability in message delivery. By default, when a consumer receives messages from a queue, they are not automatically acknowledged. This means that if a consumer fails to process a message for any reason, RabbitMQ will re-queue that message, allowing another consumer to process it.
Why Acknowledgments Matter
Acknowledgments are essential for several reasons:
- Reliability: If a message is not acknowledged, RabbitMQ knows the message needs to be re-delivered.
- Control: Consumers can acknowledge messages in a way that best fits their processing logic.
- Failure Handling: If a consumer crashes while processing, unacknowledged messages can be re-delivered to ensure no loss of data.
Acknowledgment Modes
RabbitMQ supports two acknowledgment modes:
-
Auto Acknowledgment: The message is automatically marked as acknowledged when it is sent to the consumer. This is suitable for lightweight processing where message loss is acceptable.
-
Manual Acknowledgment: The consumer explicitly acknowledges a message after successfully processing it. This method is preferred when message integrity is paramount.
Code Snippet: Basic RabbitMQ Setup
To illustrate our discussion, let’s set up a basic RabbitMQ producer and consumer in Java.
Maven Dependency
Ensure you have the RabbitMQ Java client in your Maven pom.xml
:
<dependency>
<groupId>com.rabbitmq</groupId>
<artifactId>amqp-client</artifactId>
<version>5.14.0</version>
</dependency>
Producer Code
Here is a simple code snippet for a RabbitMQ producer:
import com.rabbitmq.client.Channel;
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.ConnectionFactory;
public class Producer {
private final static String QUEUE_NAME = "hello";
public static void main(String[] argv) throws Exception {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
try (Connection connection = factory.newConnection();
Channel channel = connection.createChannel()) {
channel.queueDeclare(QUEUE_NAME, false, false, false, null);
String message = "Hello World!";
channel.basicPublish("", QUEUE_NAME, null, message.getBytes());
System.out.println(" [x] Sent '" + message + "'");
}
}
}
Explanation
- ConnectionFactory: Manages the connection parameters to RabbitMQ. Setting the host is essential for connecting to the broker.
- Channel: A channel is where most of the API for accessing RabbitMQ resides.
- queueDeclare: Ensures that the queue exists before we send a message.
- basicPublish: Here, we send our message to the queue.
This example illustrates the basics of producing messages. Now, let’s look at the consumer side, where acknowledgment comes into play.
Code Snippet: Consumer Code with Manual Acknowledgment
Here’s a simple consumer implementation that acknowledges messages:
import com.rabbitmq.client.*;
public class Consumer {
private final static String QUEUE_NAME = "hello";
public static void main(String[] argv) throws Exception {
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
try (Connection connection = factory.newConnection();
Channel channel = connection.createChannel()) {
channel.queueDeclare(QUEUE_NAME, false, false, false, null);
System.out.println(" [*] Waiting for messages. To exit press CTRL+C");
DeliverCallback deliverCallback = (consumerTag, delivery) -> {
String message = new String(delivery.getBody(), "UTF-8");
System.out.println(" [x] Received '" + message + "'");
// Manually acknowledge the message
channel.basicAck(delivery.getEnvelope().getDeliveryTag(), false);
};
// Start consuming messages with manual acknowledgment
channel.basicConsume(QUEUE_NAME, false, deliverCallback, consumerTag -> { });
}
}
}
Important Highlights
- DeliverCallback: Handles delivery notifications. Here, you can process your message and decide when to acknowledge it.
- basicAck: This explicitly acknowledges the message, indicating to RabbitMQ that it has been handled successfully.
- autoAck Parameter: Set to
false
inchannel.basicConsume()
, preventing messages from being automatically acknowledged.
Handling Message Processing Failures
In many applications, message processing might fail for various reasons, such as a database outage or an exception in the application logic. Here is how you can handle such cases:
Example Handling Logic
DeliverCallback deliverCallback = (consumerTag, delivery) -> {
String message = new String(delivery.getBody(), "UTF-8");
try {
// Simulate processing the message
processMessage(message);
// Acknowledge if processing is successful
channel.basicAck(delivery.getEnvelope().getDeliveryTag(), false);
} catch (Exception e) {
// Log the error and optionally requeue the message
System.err.println("Message processing failed: " + e.getMessage());
channel.basicNack(delivery.getEnvelope().getDeliveryTag(), false, true);
}
};
Explanation
- Try-Catch Block: Wrap your processing logic in a try-catch block to better handle errors.
- basicNack: If processing fails, use this method to indicate that RabbitMQ should requeue the message for another consumer to process.
This approach helps maintain reliability in a dynamic working environment. For essential additional reading on RabbitMQ error handling, check out the official RabbitMQ documentation.
In Conclusion, Here is What Matters
The acknowledgment system in RabbitMQ plays a critical role in ensuring that messages are processed correctly. Whether using automatic or manual acknowledgments, understanding the implications of each choice plays a crucial role in system reliability.
From the producer that sends messages to the consumer that processes them, acknowledgment flows through the system, ensuring that messages are neither lost nor left unprocessed.
By mastering RabbitMQ acknowledgments, you can build more robust applications that manage communication effectively—ensuring your application remains reliable, resilient, and scalable.
If you're interested in learning more about RabbitMQ, its features, and how to get started, don't hesitate to explore more on the RabbitMQ website.
Happy coding!