Common REST API Pitfalls and How to Avoid Them
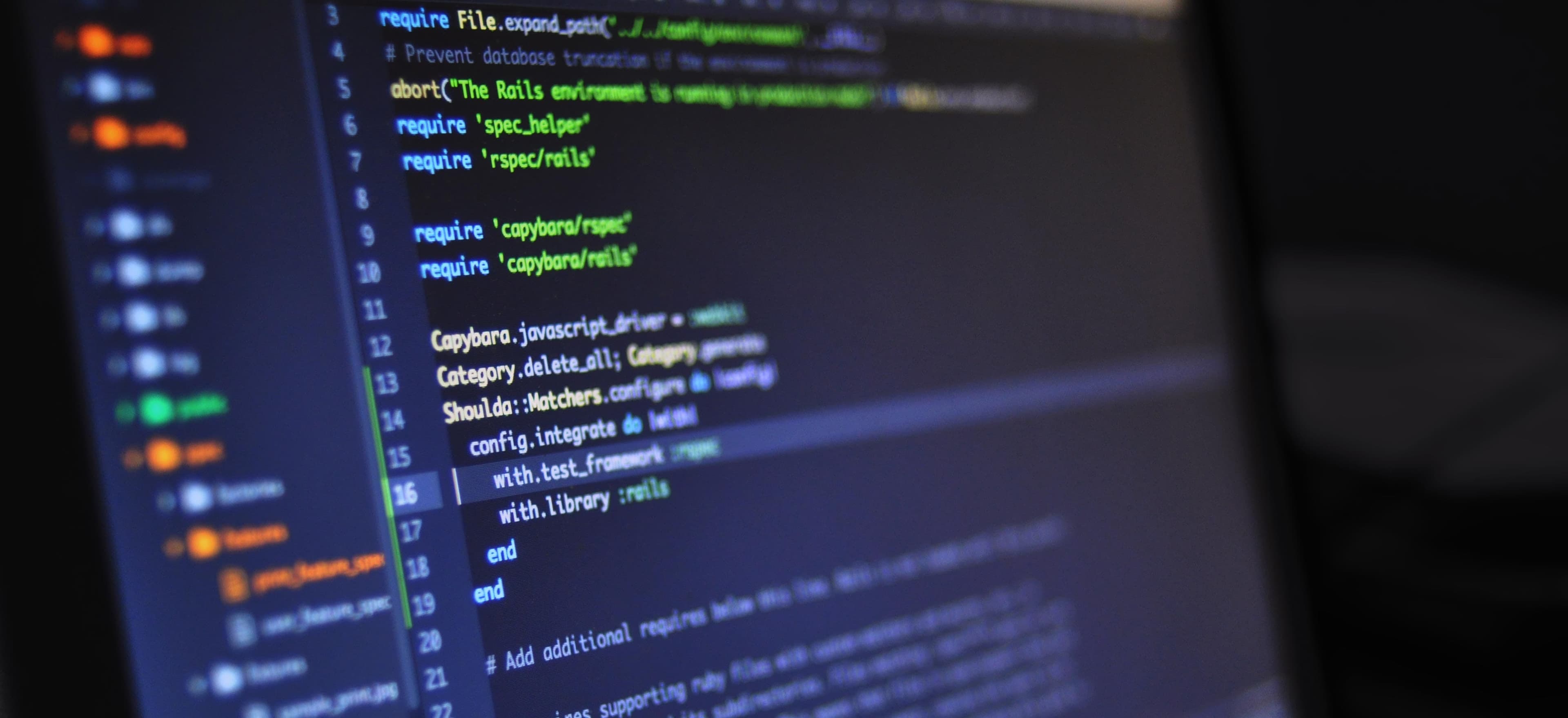
- Published on
Common REST API Pitfalls and How to Avoid Them
In the contemporary world of application development, REST APIs play a pivotal role. They serve as the backbone for seamless communication between client-side and server-side applications. However, despite their advantages, developing a REST API is not without its challenges. This blog post delves into common REST API pitfalls and offers strategies for avoiding them—empowering developers to create more efficient, maintainable APIs.
1. Inconsistent Naming Conventions
The Issue
One of the most frequent pitfalls is inconsistent naming conventions. Poorly named endpoints can lead to confusion among developers and users, hampering the API's usability.
The Solution
Establish a clear naming convention and adhere to it throughout your API. Use plural nouns for collections and singular nouns for individual resources.
Example:
// Bad naming
GET /user/123
POST /adduser
// Good naming
GET /users/123
POST /users
Why This Matters: Consistent naming enhances readability and predictability, making it easier for consumers to understand and navigate your API.
2. Poor Versioning Strategy
The Issue
APIs evolve over time. A lack of versioning can lead to breaking changes that disrupt existing clients.
The Solution
Adopt a versioning strategy early on. A common method is to include version numbers directly in your URL.
Example:
// Versioning
GET /v1/users
GET /v2/users
Why This Matters: By implementing versioning, clients can continue to use older versions while you make improvements, maintaining backward compatibility.
3. Ignoring the HTTP Methods
The Issue
Another common mistake is misusing HTTP methods. For instance, using a GET request to update resources can lead to unexpected behaviors.
The Solution
Follow the RESTful principles of HTTP methods:
- GET: Retrieve information
- POST: Create a new resource
- PUT: Update an existing resource
- DELETE: Remove a resource
Example:
// Correct usage
// @GET: Retrieve
@GetMapping("/users/{id}")
public User getUser(@PathVariable Long id) {
return userService.findUserById(id);
}
// @POST: Create
@PostMapping("/users")
public User createUser(@RequestBody User user) {
return userService.saveUser(user);
}
Why This Matters: Correctly implementing HTTP methods leads to a RESTful API that adheres to standard practices, enhancing predictability.
4. Lack of Proper Error Handling
The Issue
A/API endpoints often respond with generic error messages when something goes wrong. This makes debugging difficult for API consumers.
The Solution
Implement specific error codes and messages in your API responses. Use standard HTTP status codes and provide additional context within the body of the response.
Example:
// Error handling
@ExceptionHandler(UserNotFoundException.class)
@ResponseStatus(HttpStatus.NOT_FOUND)
public ErrorResponse handleUserNotFound(UserNotFoundException ex) {
return new ErrorResponse("User not found", ex.getMessage());
}
Why This Matters: A well-defined error handling mechanism allows clients to diagnose issues quickly, enhancing their overall experience.
5. Forgetting About Security
The Issue
Many developers underestimate the importance of security in API design. Exposing endpoints without proper authentication can lead to data breaches.
The Solution
Implement authentication and authorization protocols. Common approaches include OAuth and API tokens.
Example:
@RestController
@RequestMapping("/api")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users")
@PreAuthorize("hasRole('USER') or hasRole('ADMIN')")
public List<User> getAllUsers() {
return userService.findAllUsers();
}
}
Why This Matters: Securing your API ensures that only authorized users can access sensitive endpoints, protecting both your data and your clients.
6. Overloading Endpoints
The Issue
One of the common pitfalls in API design is overloading endpoints with too much functionality. This leads to complexity and reduces maintainability.
The Solution
Keep endpoints focused; each should serve a single purpose. If an endpoint is doing too much, consider breaking it into smaller, specialized endpoints.
Example:
// Overloaded
POST /users/123/activateAndNotify
// Better approach
POST /users/123/activate
POST /notifications/send
Why This Matters: Focused endpoints are easier to understand and maintain, offering a clearer abstraction to API consumers.
7. Neglecting Rate Limiting
The Issue
APIs that do not implement rate limiting are vulnerable to abuse, which can lead to server overload.
The Solution
Implement rate limiting to control the number of requests a user can make in a given timeframe.
Example:
@Scheduled(fixedRate = 1000)
public void enforceRateLimiting() {
// Logic to track and limit incoming requests
}
Why This Matters: Rate limiting protects your API from overuse and ensures fair resource allocation among users.
8. Limited Documentation
The Issue
Documentation is often neglected in the development process, creating hurdles for users trying to implement the API.
The Solution
Invest time in providing clear and comprehensive documentation. Tools like Swagger can help generate interactive API documentation automatically.
Why This Matters: Well-documented APIs enhance user experience, making integration smoother and reducing support queries.
In Conclusion, Here is What Matters
Building a successful REST API requires attention to detail, an understanding of best practices, and proactive planning. By avoiding these common pitfalls, developers can deliver robust APIs that provide a great user experience and are easier to maintain.
For a deeper delve into REST API best practices, consider reading The RESTful API Design Rulebook and exploring API security best practices.
Incorporating these strategies will empower you to create secure, efficient, and user-friendly REST APIs. With careful planning and adherence to industry standards, you'll enhance the quality and maintainability of your APIs, leading to greater success in your development projects.
Checkout our other articles