Maximizing Visibility: Monitor Your ExecutorService Effectively
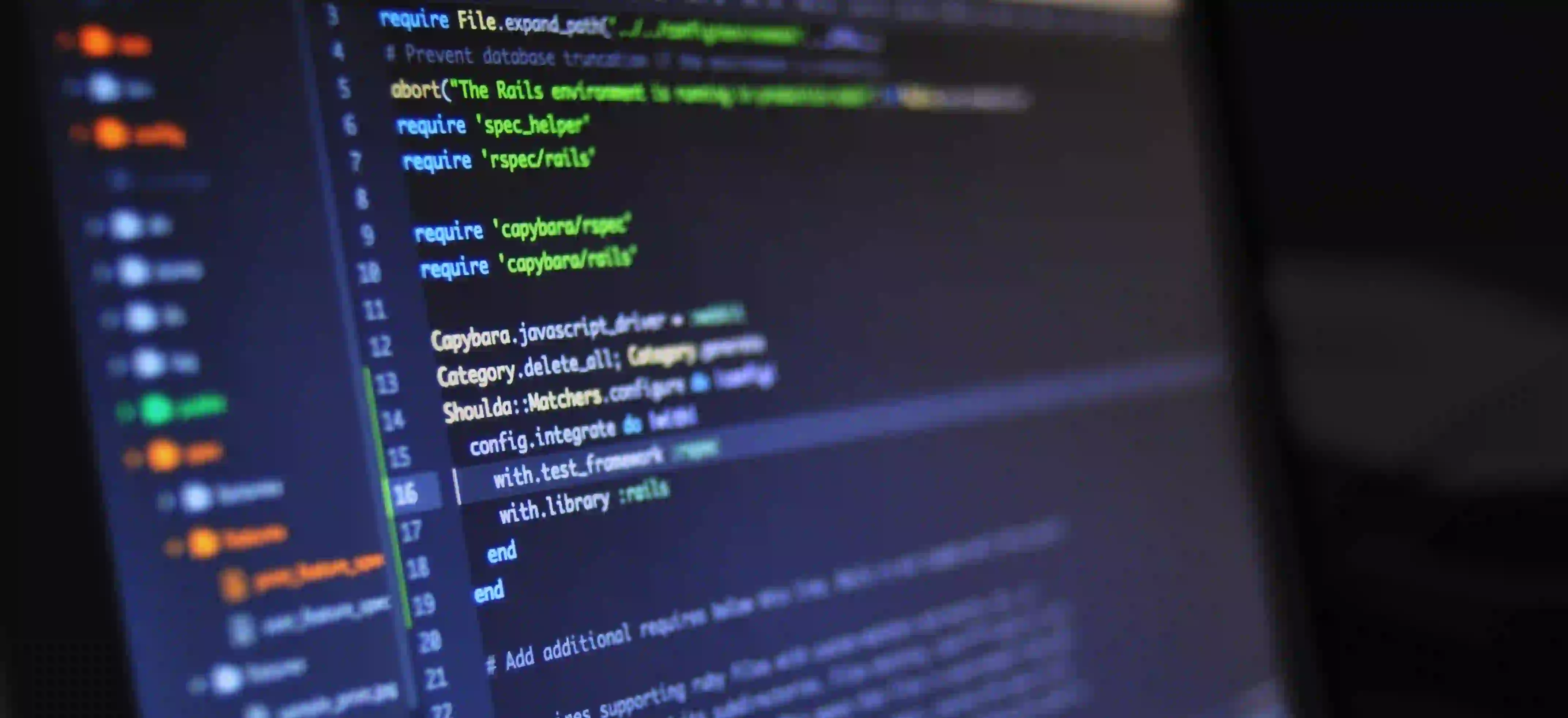
Maximizing Visibility: Monitor Your ExecutorService Effectively
In the world of Java programming, concurrency is a vital aspect of building efficient applications. The ExecutorService
framework plays a crucial role in managing and controlling thread execution. However, to ensure that your applications run smoothly and efficiently, it's essential to monitor the ExecutorService
effectively. In this blog post, we will delve into the techniques and tools available for monitoring your ExecutorService
, thereby maximizing visibility and optimizing performance.
Understanding ExecutorService
Before diving into monitoring strategies, let's clarify what ExecutorService
is. Introduced in Java 5, ExecutorService
is part of the java.util.concurrent
package. It abstracts the complexities of creating and managing threads, allowing developers to focus on designing concurrent programs.
To illustrate its basic usage, consider the following code snippet:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ExecutorServiceExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(5);
for (int i = 0; i < 10; i++) {
final int taskId = i;
executor.submit(() -> {
System.out.println("Executing task " + taskId + " by " + Thread.currentThread().getName());
});
}
executor.shutdown();
}
}
Commentary on the Code
In this simple example, we create a fixed thread pool with a maximum of five threads, then submit ten tasks. Each task prints its identifier and the name of the thread executing it. This setup demonstrates the ease of creating concurrent tasks using ExecutorService
.
Now, monitoring these tasks' execution is key to understanding their performance, identifying bottlenecks, and ensuring that your application behaves predictably.
Why Monitor ExecutorService?
Monitoring ExecutorService
is essential for several reasons:
- Performance Insights: Gain insights into how quickly tasks are executing and resource utilization.
- Debugging: Detect potential deadlocks and thread starvation, which can lead to performance degradation.
- Resource Management: Ensure that resources are not being wasted by over-provisioning threads.
- Scaling Applications: Inform decisions when scaling applications to handle varying loads.
Monitoring Techniques
1. Using JMX (Java Management Extensions)
Java Management Extensions (JMX) provides a way to manage and monitor applications. You can expose your ExecutorService
via JMX, allowing you to gather statistics on thread activity, task execution times, and more.
Here's how to implement JMX monitoring:
import java.lang.management.ManagementFactory;
import java.lang.management.ThreadMXBean;
public class JMXMonitoring {
public static void main(String[] args) {
// Get the thread management bean
ThreadMXBean threadBean = ManagementFactory.getThreadMXBean();
// Get the number of live threads
System.out.println("Live Thread Count: " + threadBean.getThreadCount());
// Monitor thread states
for (long threadId : threadBean.getAllThreadIds()) {
System.out.println("Thread ID: " + threadId + " is in state: " + threadBean.getThreadInfo(threadId).getThreadState());
}
}
}
Commentary on JMX Code
In the code above, we retrieve the current thread count and their respective states using ThreadMXBean
. This information is crucial when troubleshooting issues like bottlenecks or deadlocks.
2. Custom Callbacks
Another powerful technique is implementing custom callbacks. By wrapping task execution, you can log execution times, success, and failure rates. Here’s how you can achieve that:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class CustomCallbackExecutor {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(3);
for (int i = 0; i < 5; i++) {
final int taskId = i;
executor.submit(() -> {
long startTime = System.currentTimeMillis();
try {
System.out.println("Task " + taskId + " is running");
// Simulate some task processing
Thread.sleep((taskId + 1) * 1000);
System.out.println("Task " + taskId + " completed");
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
System.out.println("Task " + taskId + " was interrupted");
} finally {
long endTime = System.currentTimeMillis();
System.out.println("Task " + taskId + " took " + (endTime - startTime) + " ms");
}
});
}
executor.shutdown();
}
}
Commentary on Custom Callback Code
This implementation tracks execution time for each task. This way, you can gain insights into performance and potential issues, such as unexpected long execution times that could warrant investigation.
3. Third-Party Monitoring Tools
In addition to the built-in Java tools, there are several third-party libraries and applications that can provide advanced monitoring capabilities:
- Prometheus & Grafana: These tools can be integrated into your Java applications for real-time metrics monitoring and visualization. You can expose custom metrics, capture thread pool statistics, and analyze performance trends over time.
- Elastic Stack: The Elastic Stack (ELK) can also be used to monitor logs generated by your
ExecutorService
. You can set up alerts based on error rates or execution times to keep your applications running smoothly.
Best Practices for ExecutorService Monitoring
- Limit Thread Pool Sizes: Use realistic limits on thread pools to prevent oversubscription of resources.
- Graceful Shutdown: Always ensure that your
ExecutorService
shuts down gracefully to avoid resource leaks. - Error Handling: Implement retry mechanisms where appropriate, along with error logging, to handle failures gracefully.
- Dynamic Tuning: Consider using
ScheduledThreadPoolExecutor
or similar for dynamic adjustment of thread pool sizes based on application load.
Key Takeaways
Monitoring your ExecutorService
is not just a good practice; it is essential for ensuring your Java applications run efficiently and predictably. By leveraging JMX, custom callbacks, and third-party tools, you can gain valuable insights into your application's performance and responsiveness.
As you enhance your monitoring capabilities, keep in mind the best practices mentioned to optimize your concurrency model further. Investing time in monitoring today will yield significant dividends in performance and reliability down the road.
For deeper insights into concurrency in Java, you might also want to check out Java Concurrency in Practice by Brian Goetz, which is an excellent resource for best practices and design patterns in concurrent programming.
Happy coding and monitoring!