Stop Brute Force Attacks in Spring Security Now!
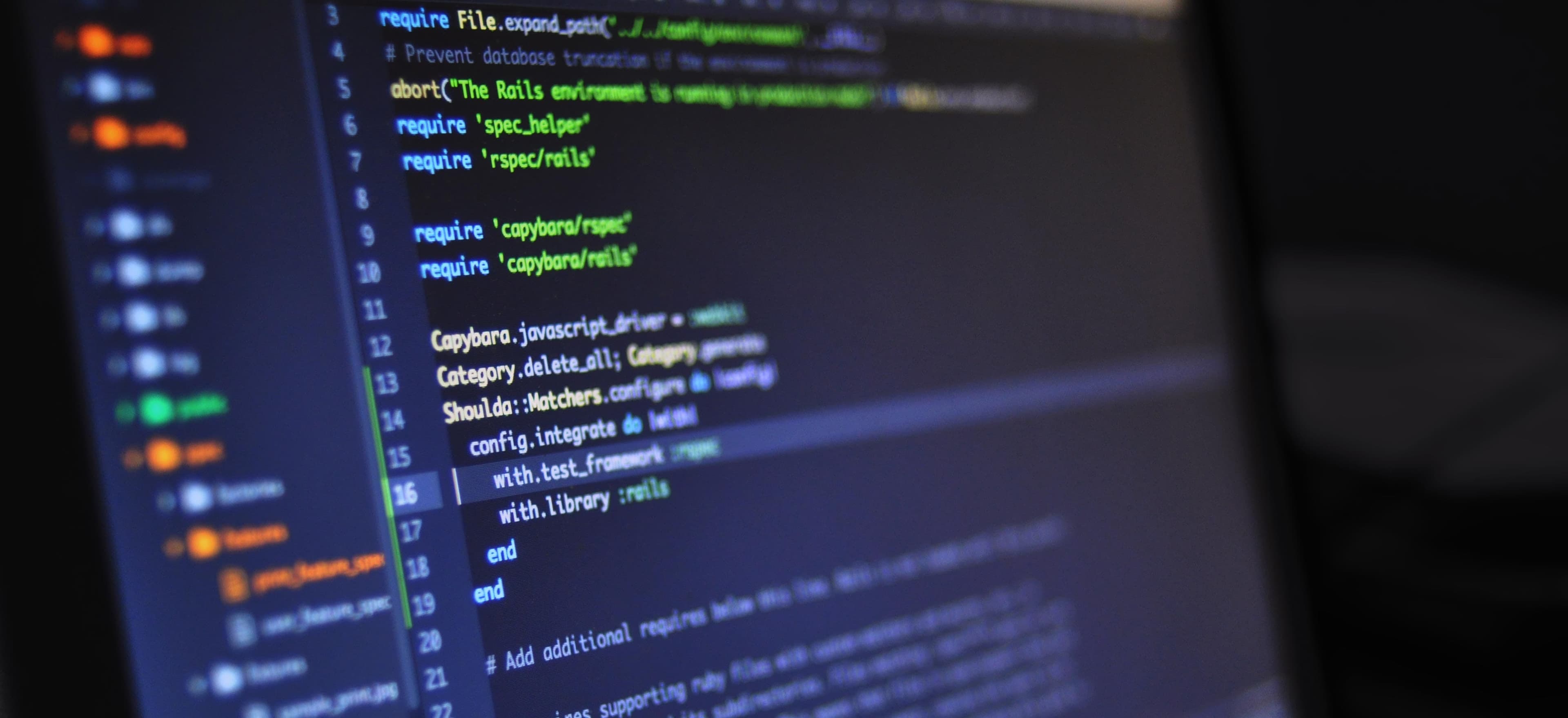
- Published on
Stop Brute Force Attacks in Spring Security Now!
In today’s digital age, cyber threats, including brute force attacks, are an ever-growing concern. As developers, we must ensure the applications we build are secure. In this blog post, we’ll discuss what brute force attacks are, the importance of securing your Spring applications, and how to effectively implement security measures using Spring Security.
What is a Brute Force Attack?
A brute force attack is a method used by cybercriminals to crack passwords or encryption keys by systematically trying all possible combinations until the correct one is found. This technique can be performed manually or by using automated software that can try hundreds or thousands of combinations per second.
Why are Brute Force Attacks Dangerous?
Brute force attacks can compromise user accounts, and data breaches can lead to identity theft and significant financial losses. Moreover, the reputational damage to businesses can take years to recover from.
Why Use Spring Security?
Spring Security provides powerful and customizable authentication and access control capabilities. It is the standard for securing Spring-based applications. With a built-in framework, developers can implement security features without reinventing the wheel.
Steps to Prevent Brute Force Attacks in Spring Security
1. Implement Account Lockout Mechanism
One of the simplest yet effective strategies against brute force attacks is to lock accounts after a certain number of failed login attempts.
Example: Implementing Account Lockout
Here’s how you can implement account lockout in Spring Security:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll()
.and()
.sessionManagement()
.maximumSessions(1)
.expiredUrl("/login?expired=true")
.and()
.sessionManagement()
.sessionFixation().none();
}
// Example: Account Lockout Logic
@Bean
public AuthenticationFailureHandler customFailureHandler() {
return new CustomAuthenticationFailureHandler();
}
}
Commentary
In the code snippet above, we define a custom authentication failure handler. To achieve the account lockout functionality, you should track the number of failed login attempts and lock the account if it exceeds a preset limit.
2. Use CAPTCHA for Login Forms
Integrating CAPTCHA into your login forms can help mitigate brute force attacks by ensuring that a human is attempting to log in.
Example: Integrating Google reCAPTCHA
<form method="post" action="/login">
<input type="text" name="username" placeholder="Username" required>
<input type="password" name="password" placeholder="Password" required>
<div class="g-recaptcha" data-sitekey="your-site-key"></div>
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
<button type="submit">Login</button>
</form>
Commentary
In the HTML snippet above, Google reCAPTCHA is included using its JavaScript API. When users submit the form, the backend should verify the CAPTCHA response before proceeding with login attempts. This effectively reduces the risk posed by automated scripts.
3. Implement Rate Limiting
Rate limiting allows developers to control the number of requests a user can make in a given timeframe. This helps to slow down potential brute force attacks.
Example: Using Spring’s Rate Limiting
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.filter.OncePerRequestFilter;
import javax.servlet.FilterChain;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.concurrent.atomic.AtomicInteger;
@RestController
@RequestMapping("/login")
public class LoginController {
private AtomicInteger requestCount = new AtomicInteger(0);
@GetMapping
public String login() {
int count = requestCount.incrementAndGet();
if (count > 5) { // Limit to 5 attempts
return "Too many attempts. Please try again later.";
}
return "Login Page";
}
public void resetCounter() {
requestCount.set(0);
}
}
Commentary
In this example, we use an AtomicInteger
to keep track of login attempts. Once the count exceeds a certain threshold, we provide feedback to the user, temporarily halting further attempts. You might want to implement a reset mechanism to clear the count after a certain time or on a successful login.
4. Security Headers
Adding security headers to your application can protect against various types of attacks, including brute force attacks.
@Bean
public WebSecurityCustomizer webSecurityCustomizer() {
return (web) -> web.ignoring().antMatchers("/resources/**");
}
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http
.headers()
.defaultsDisabled()
.contentSecurityPolicy("script-src 'self'");
return http.build();
}
Commentary
Here, we configure the SecurityFilterChain
to include security headers, enhancing the application’s defense against common vulnerabilities. A strong Content Security Policy (CSP) can prevent malicious scripts from running, thus indirectly helping to mitigate the risks linked to brute force attempts.
5. Monitor Login Attempts
Continuous monitoring of login attempts can yield useful insights to improve account security. By logging failed attempts, developers can establish patterns and identify potential attackers.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class CustomAuthenticationFailureHandler implements AuthenticationFailureHandler {
private static final Logger logger = LoggerFactory.getLogger(CustomAuthenticationFailureHandler.class);
@Override
public void onAuthenticationFailure(HttpServletRequest request,
HttpServletResponse response,
AuthenticationException exception) throws IOException {
logger.warn("Login attempt failed for user: {}", request.getParameter("username"));
response.sendRedirect("/login?error=true");
}
}
Commentary
In this code, we log each failed attempt, which can feed into further security measures. Awareness of the frequency and source of failed logins can help developers adjust security settings and identify malicious behavior.
Final Considerations
Brute force attacks pose a serious threat to application security. Implementing the strategies discussed in this post will help secure your Spring applications against unauthorized access effectively.
From account lockout mechanisms to CAPTCHA integration and rate limiting, leveraging Spring Security enables developers to build robust security features effortlessly. Monitor login activity and employ security headers to further bolster your defenses.
For those looking for additional resources, consider checking out the Spring Security documentation and OWASP guidelines for comprehensive techniques regarding web security.
Taking preventive measures now will protect your application in the long run. Secure your application and stay one step ahead of attackers!