Java 21: Tackling Compatibility Issues in Legacy Code
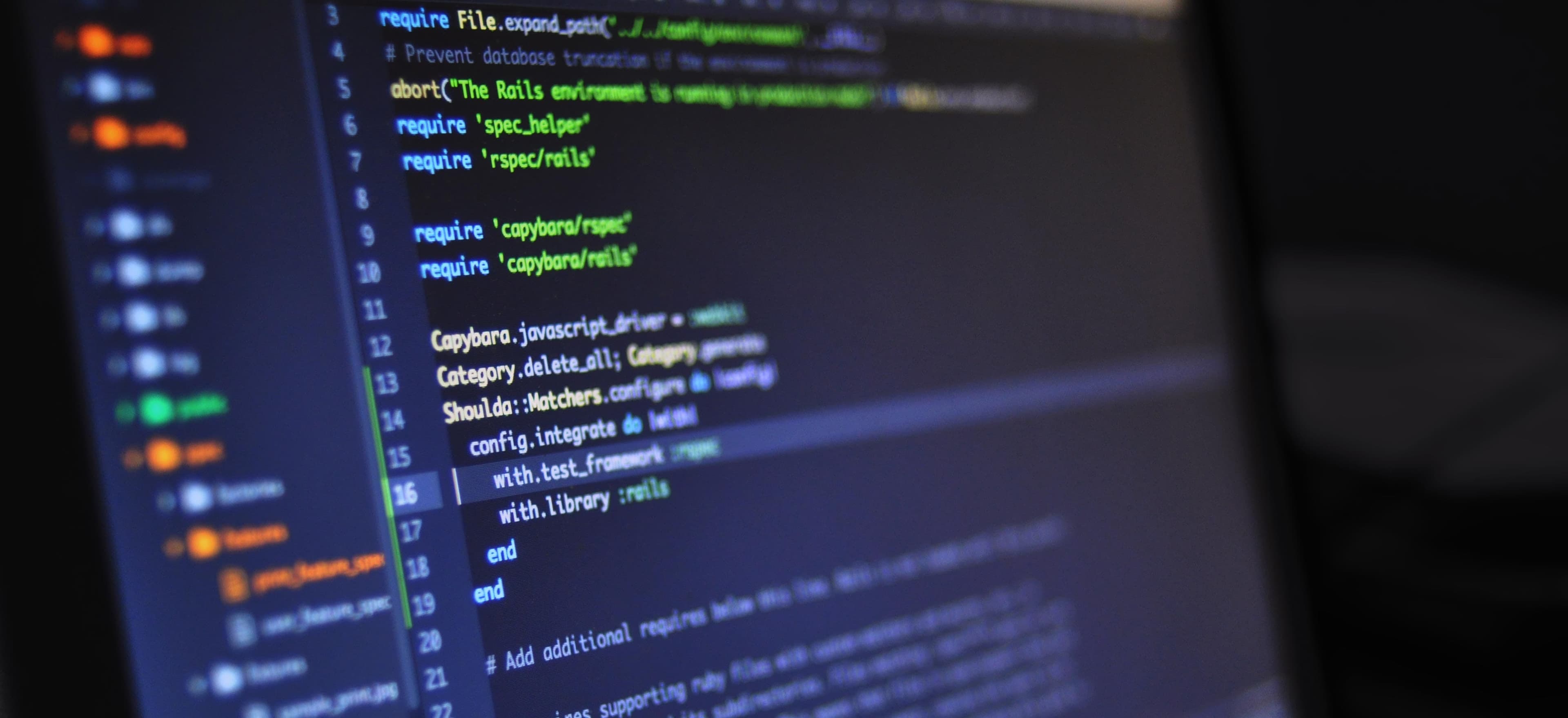
- Published on
Java 21: Tackling Compatibility Issues in Legacy Code
As the world of software development continues to evolve, Java remains one of the most widely used programming languages. With its recent update—Java 21—developers are presented with new features to enhance productivity and application performance. However, these improvements often come with compatibility challenges, particularly for legacy codebases. In this blog post, we will explore how Java 21 impacts legacy code and how developers can navigate potential compatibility issues.
What is Legacy Code?
Legacy code refers to outdated code that is still in use, typically because it supports critical systems or functions. Often, this code is no longer maintained, may lack documentation, and might rely on outdated languages or practices. As new versions of Java are released, the question arises—how does this new generation of Java interact with the old?
Key Features of Java 21
Java 21 introduces several enhancements that can aid in refactoring legacy systems. Some of the most notable advancements include:
- Pattern Matching: This feature simplifies the process of type checking and casting.
- Virtual Threads: Aimed at simplifying concurrent programming and managing scalability.
- Sealed Classes: Provides better control over inheritance and enhances code security and maintainability.
These features can significantly improve your code's readability and performance. However, they may also present challenges if legacy code relies on older paradigms.
Compatibility Issues in Legacy Code
When upgrading to Java 21, there are common compatibility issues developers may face:
- Dependencies on Deprecated APIs: Many older APIs may have been deprecated or removed altogether, leading to compilation errors.
- Changes in Language Constructs: Syntax changes can lead to unexpected behavior in older applications.
- Concurrency Models: Code built around previous concurrency models may not mesh well with the new virtual threads system.
Assessing Your Legacy Codebase
Before upgrading, it is essential to assess your legacy codebase thoroughly. Here are steps to take:
- Code Review: Regularly review your code to identify dependencies on deprecated or outdated APIs.
- Automated Tests: Ensure that you have a robust suite of automated tests to catch regressions.
- Static Code Analysis: Utilize tools like SonarQube or PMD to identify potential issues in your legacy code.
Gradual Migration Strategy
Instead of upgrading your entire application at once, consider a gradual migration strategy. Here’s how:
- Identify Critical Components: Focus on updating code that directly impacts performance or security.
- Modular Upgrades: Break down updates into smaller, manageable modules.
- Use Feature Flags: Implement new features behind flags to allow for easy rollbacks if necessary.
Code Snippets: Upgrade Strategies
Here are some code snippets demonstrating how to refactor legacy code in the context of Java 21 features.
Example 1: Pattern Matching
Instead of traditional instanceof
checks and casting, you can use pattern matching:
// Legacy code using instanceof
if (obj instanceof String) {
String str = (String) obj;
// Process string
}
// Modern Java 21 pattern matching
if (obj instanceof String str) {
// Process string
}
Why? This eliminates the need for casting and fosters cleaner, more understandable code.
Example 2: Virtual Threads
For managing concurrent processes, you can utilize virtual threads introduced in Java 21:
Runnable task = () -> {
// Simulating a blocking task
System.out.println("Executing Task");
};
Thread.ofVirtual().start(task);
Why? This enhances scalability and simplifies the model you use to manage concurrency, alleviating some of the headaches traditionally associated with threads.
Example 3: Sealed Classes
Sealed classes can help manage the structure of your objects more effectively:
public sealed interface Shape permits Circle, Square {}
public final class Circle implements Shape {
// Circle implementation
}
public final class Square implements Shape {
// Square implementation
}
Why? This approach allows you to restrict the types of subclasses, leading to more robust type-checking and reducing the potential for misuse.
Addressing Common Challenges
You may run into a few roadblocks when migrating to Java 21:
- Integration with Third-Party Libraries: If libraries you depend on are not yet compatible with Java 21, consider checking for updates or alternatives.
- Team Training: Ensure that your team is well-equipped with the knowledge of new Java 21 features and best practices. Regular workshops and coding sessions can help.
Resources for Further Learning
To further support your transition to Java 21, explore the following resources:
In Conclusion, Here is What Matters
As you move to Java 21, taking a thoughtful approach to compatibility issues in legacy code is paramount. Use Java's new features to refine and enhance your previous work, but proceed with caution. Assess your codebase thoroughly, implement a strategic migration, and invest in team education to ease the transition. With Java’s evolution, the opportunity to modernize your legacy systems is not just a necessity but an exciting challenge.
Upgrading is not merely about moving to the next version; it's about improving your code, your team's productivity, and your application's long-term viability. Embrace the changes, and Java will continue to be a powerful ally in your development journey.
Feel free to share your thoughts or questions below. Happy coding!