Integrating JUnit 5 in Legacy Pre-Java 8 Projects
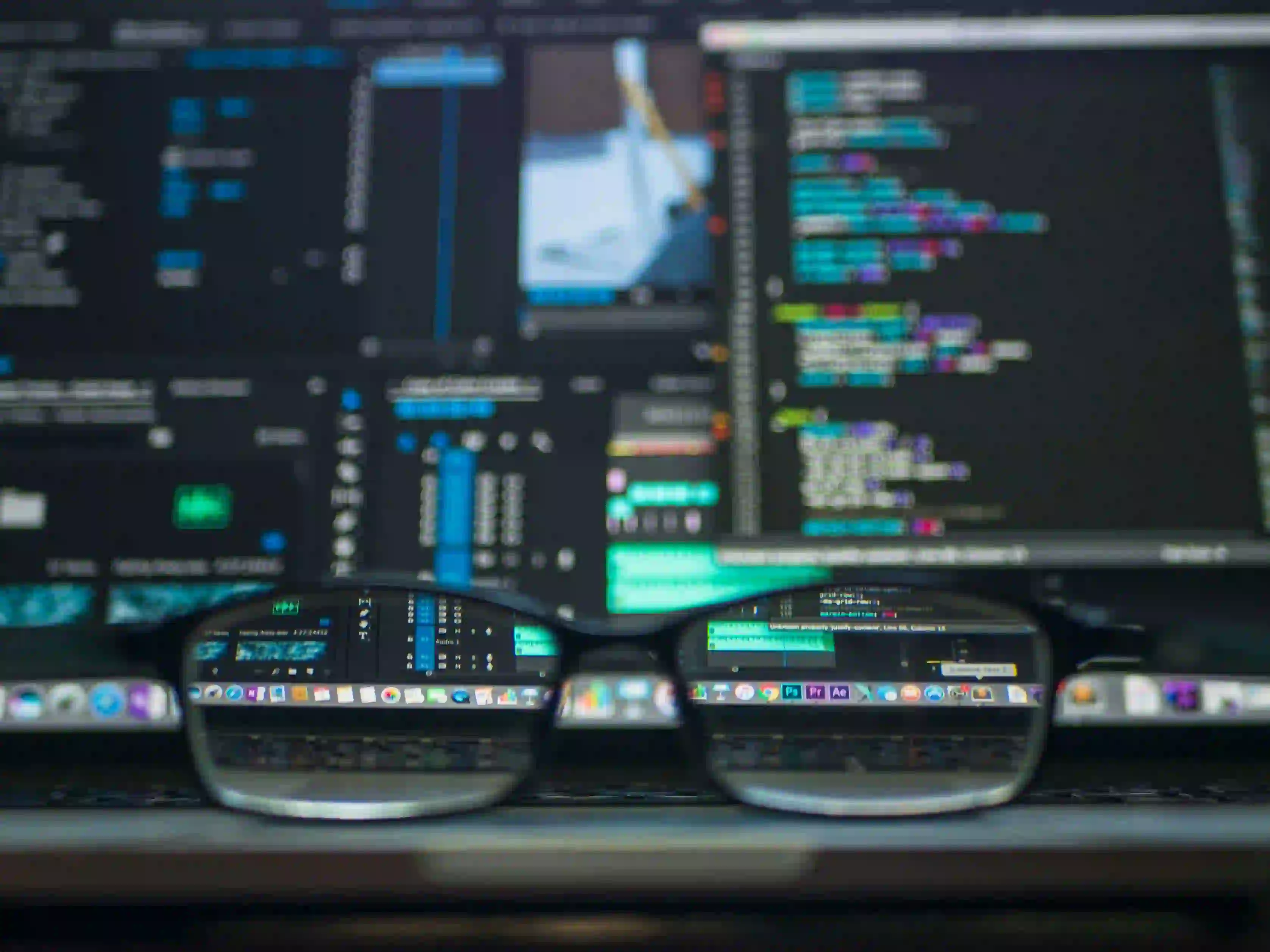
Integrating JUnit 5 in Legacy Pre-Java 8 Projects
As software developers, we often encounter the challenge of improving legacy codebases. Many older Java projects still rely on pre-Java 8 features and structures. One common enhancement is the integration of modern testing frameworks such as JUnit 5. This blog post illustrates how to effectively introduce JUnit 5 into legacy Java projects, bringing modern testing capabilities to an outdated environment.
Understanding JUnit 5
JUnit 5 is the latest version of the popular testing framework for Java, consisting of three main components:
- JUnit Platform: The foundation to launch testing frameworks.
- JUnit Jupiter: A new programming model and extension model for writing tests.
- JUnit Vintage: A means to run JUnit 3 and JUnit 4 based tests on the JUnit 5 platform.
If you're still using JUnit 4 or earlier, upgrading brings benefits like better annotations, a more powerful extension model, and improved support for parameterized tests.
Why Migrate to JUnit 5?
- Improved Features: With new annotations (@BeforeAll, @AfterEach, etc.), JUnit 5 simplifies the writing of tests and promotes better organization.
- Flexibility: It allows integration with various other frameworks and tools, offering greater flexibility for all testing scenarios.
- Better JVM Support: JUnit 5 is designed for Java 8 and later, but with the Vintage component, it can also handle older tests.
Initial Setup: Configuring Your Build Tool
Maven
If your legacy project relies on Maven, integrate JUnit 5 by adding the following dependency:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.9.3</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
<version>5.9.3</version>
<scope>test</scope>
</dependency>
This configuration allows you to run both JUnit 5 tests and legacy tests in the same environment, greatly enhancing your project while maintaining backward compatibility.
Gradle
For Gradle-based projects, include the following snippets in your build.gradle
:
testImplementation 'org.junit.jupiter:junit-jupiter:5.9.3'
testImplementation 'org.junit.vintage:junit-vintage-engine:5.9.3'
After properly configuring your build tool, the next step involves setting up your test classes.
Refactoring Test Classes
Migrating to JUnit 5 requires adjusting your test classes to utilize the new annotations and structure. In JUnit 4, a typical test class might look like this:
import org.junit.Before;
import org.junit.Test;
import static org.junit.Assert.*;
public class MyLegacyTest {
private MyClass myClass;
@Before
public void setUp() {
myClass = new MyClass();
}
@Test
public void testMethod() {
assertEquals("Expected Value", myClass.methodUnderTest());
}
}
Adjusting to JUnit 5
Refactor the test class to follow JUnit 5 conventions. The equivalent class would be:
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MyLegacyTest {
private MyClass myClass;
@BeforeEach
public void setUp() {
myClass = new MyClass();
}
@Test
public void testMethod() {
assertEquals("Expected Value", myClass.methodUnderTest());
}
}
Key Changes Explained
-
Annotations: The
@Before
annotation is replaced with@BeforeEach
, which clarifies its role in each test invocation. The@Test
annotation remains the same but is now scoped underorg.junit.jupiter.api.Test
. -
Assertions: When transitioning from
org.junit.Assert
, you'll be usingorg.junit.jupiter.api.Assertions
. The assert methods are analogous, making the transition relatively seamless.
You can find more on JUnit 5 Documentation to explore additional annotations and functionalities.
Running Tests
IDE Configuration
Most modern integrated development environments (IDEs), like IntelliJ IDEA or Eclipse, support JUnit 5 out of the box. Just update the IDE to the latest version, and you can run your tests seamlessly.
Command Line
Run your tests from the command line using Maven with:
mvn test
Or using Gradle:
gradle test
Writing New Tests with JUnit 5
As you begin to write new tests, JUnit 5 offers powerful features to take advantage of. Here are a few notable examples:
Parameterized Tests
Parameterized tests allow for running the same test with different inputs, which drastically cuts down redundancy.
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.ValueSource;
import static org.junit.jupiter.api.Assertions.assertTrue;
public class MyNewTest {
@ParameterizedTest
@ValueSource(strings = { "hello", "world" })
void testWithMultipleValues(String word) {
assertTrue(word.length() > 3);
}
}
Custom Assertions
Creating custom assertions can also simplify your tests. For example:
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CustomAssertions {
public static void assertTwoNumbersEqual(int expected, int actual) {
assertEquals(expected, actual, "The numbers are not equal");
}
}
Now, you can invoke CustomAssertions.assertTwoNumbersEqual(expectedValue, actualValue)
in various test cases for clarity and consistency.
Integration with CI/CD Pipelines
Modern software development relies heavily on Continuous Integration/Continuous Deployment (CI/CD). Ensure that your updated tests are included in pipelines by configuring the respective environments to invoke Maven or Gradle commands as required.
The Closing Argument
Integrating JUnit 5 into legacy pre-Java 8 projects revitalizes your testing practices. Although the transition requires some adjustments to your code, the benefits—such as enhanced readability, maintainability, and modern features—are worth the effort.
By following the guidelines outlined in this article, you can smoothly integrate JUnit 5, allowing you to write robust and efficient tests that cater to the demands of modern application development.
For additional reading on best practices in testing and JUnit, check out articles on Test-Driven Development (TDD) or the JUnit 5 User Guide.
Happy testing!