Troubleshooting Runtime Access to Maven Artifacts and SCM Versions
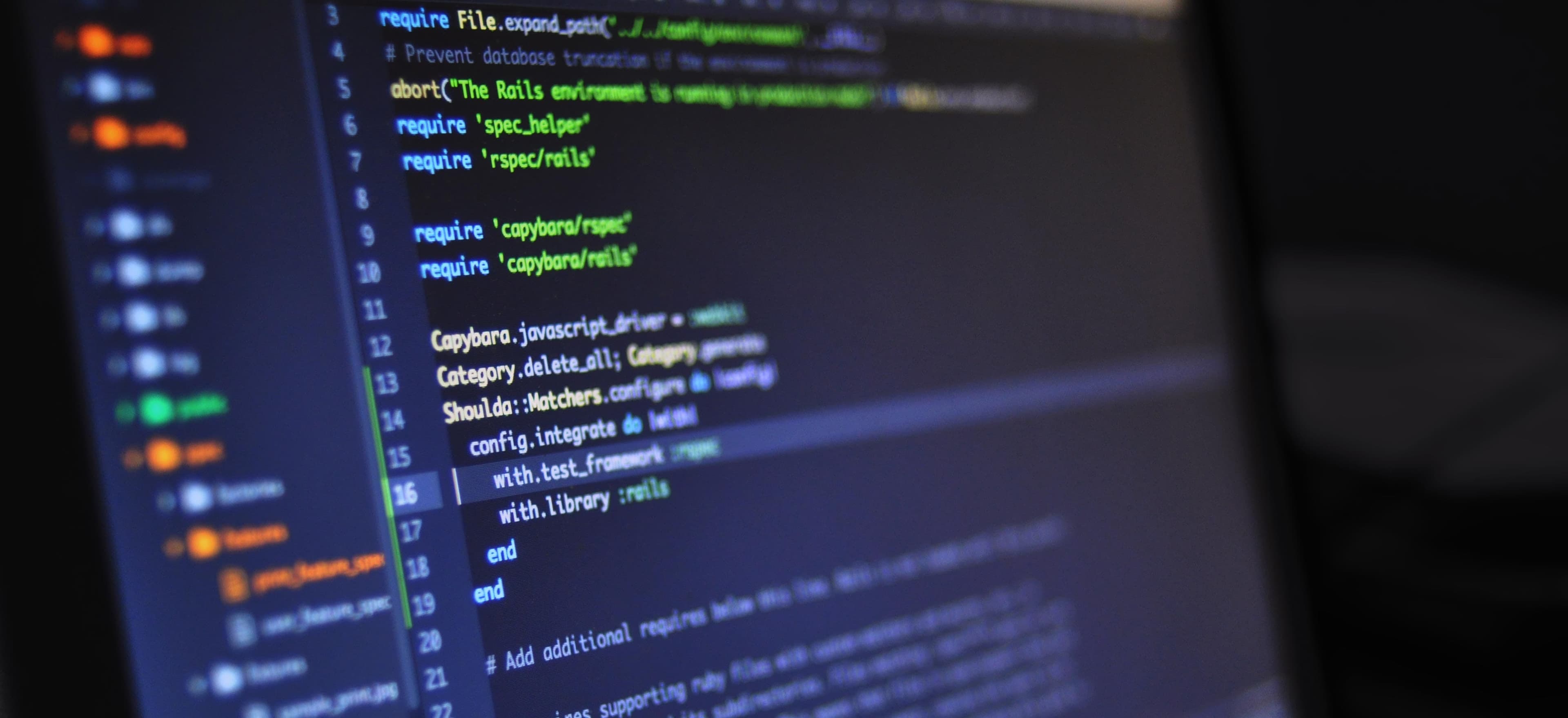
- Published on
Troubleshooting Runtime Access to Maven Artifacts and SCM Versions
When working with Maven, managing your dependencies and ensuring that you're using the correct artifact versions is crucial. Sometimes, developers face issues that lead to runtime errors related to Maven artifacts and Source Control Management (SCM) versions. In this post, we will talk about how to troubleshoot these issues effectively.
Understanding Maven Artifacts
Maven artifacts are the packaged libraries or modules that your Java application depends upon. Artifacts can be JAR files, WAR files, or even a simple POM file. The Maven repository holds these dependencies, and it ensures that your application has everything it needs to run smoothly.
Common Issues with Maven Artifacts
-
Incorrect Version References: This occurs when a POM file references an artifact version that does not exist in the repository.
-
Corrupted Local Repository: Sometimes, the local
.m2/repository
can get corrupted, leading to exceptions. -
Proxy Configuration Issues: If you are behind a corporate firewall, your Maven setup might not be able to connect to the remote repositories.
Resolving Version References
Often you might encounter an issue similar to the following when running your application:
Failed to execute goal on project my-app: Could not resolve dependencies for project (...)
To fix version reference issues:
1. Check the POM File:
Make sure you have the correct dependencies declared in your pom.xml
. An example of a dependency declaration is shown below:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>30.1-jre</version>
</dependency>
Ensuring that the groupId
, artifactId
, and version
are all correct is essential.
2. Use the mvn dependency:tree
Command:
This command gives you a detailed view of all your dependencies and their versions:
mvn dependency:tree
Searching for what dependency is causing issues can greatly help isolate and debug the problem.
Managing SCM Versions
Maven can also be set up to retrieve artifacts from SCM versions. SCM versions refer to managing the versions of your project’s source code. It could lead to discrepancies if the version you are working with in your source control system does not match with what Maven fetches.
Common Issues with SCM Management
-
SCM URL Configuration Errors: If your POM file's SCM tag is misconfigured, it can lead to problems.
-
Version Mismatch: Your local SCM might have different branches or commits that don’t match the expected Maven configuration.
Tips for SCM URL Configuration
Ensure that your SCM configuration in the pom.xml
is correctly formatted:
<scm>
<connection>scm:git:git://github.com/username/repo.git</connection>
<developerConnection>scm:git:ssh://github.com/username/repo.git</developerConnection>
<url>https://github.com/username/repo</url>
</scm>
Key Aspects to Note:
- URL: Make sure that the URL is accessible.
- Connection Types: Ensure you are using the right type for your environment (HTTP, HTTPS, SSH).
Verifying and Resolving SCM Version Issues
To quarantine version mismatches, consider the following:
1. Check Local Branch:
Make sure you are on the correct branch that corresponds to your version tag defined in the pom.xml
.
git checkout branch-name
2. Use Git Tags:
Tags can help maintain versions of released pieces. Ensure your project has appropriate tags:
git tag -l
This command lists all tags. Make sure that the version included in your POM is indeed tagged in your repository.
General Troubleshooting Steps
1. Clear Your Local Repository
When facing persistent issues, try clearing your local repository cache:
rm -rf ~/.m2/repository/com/google/guava
This removes the cached Guava dependency, forcing Maven to fetch it again from the remote repository.
2. Run with Debugging Information
Sometimes the default error messages can be vague. Running Maven with the -X
option can provide more detailed output:
mvn clean install -X
3. Update Your Settings
If using proxies, ensure that your settings.xml
in .m2
is correctly configured. An example would look like this:
<proxies>
<proxy>
<id>example-proxy</id>
<active>true</active>
<protocol>http</protocol>
<host>your.proxy.hostname</host>
<port>8080</port>
<username>proxyuser</username>
<password>somepass</password>
<nonProxyHosts>www.google.com|*.example.com</nonProxyHosts>
</proxy>
</proxies>
Proper proxy configuration ensures Maven can retrieve artifacts from remote repositories efficiently.
A Final Look
Troubleshooting runtime access to Maven artifacts and SCM versions may seem daunting, but understanding the underlying concepts and following structured troubleshooting steps can help alleviate most issues. Keeping your dependencies organized, maintaining clear SCM configurations, and adapting best practices for managing versions will lead to smoother project maintenance.
For further reading on Maven, you can explore the official Apache Maven documentation. Additionally, reviewing Maven best practices can significantly improve your workflow.
By keeping these troubleshooting tips and best practices in mind, you can minimize runtime issues and enhance your development experience. Happy coding!