Common Pitfalls When Starting with JavaFX 20
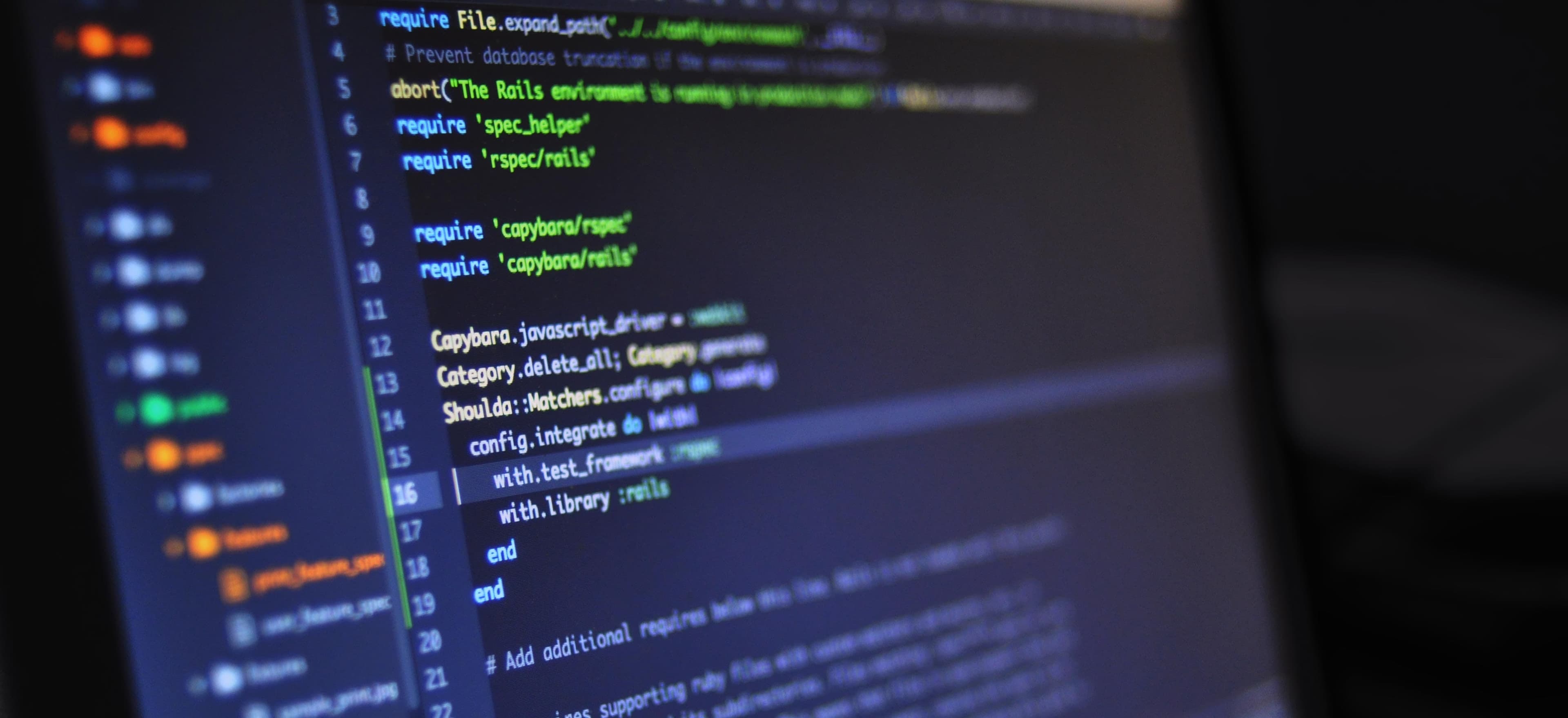
- Published on
Common Pitfalls When Starting with JavaFX 20
JavaFX 20 brings a powerful set of features to Java developers, enabling them to create rich and engaging GUI applications. However, transitioning to a new framework often comes with its own set of challenges. In this blog post, we will explore some common pitfalls that beginners encounter when working with JavaFX 20 and ways to avoid them.
1. Underestimating the Learning Curve
One of the primary pitfalls beginners face is underestimating the learning curve associated with JavaFX. Transitioning from a console application to a GUI-based framework is not trivial. JavaFX employs concepts like scenes, stages, canvases, and layouts which may be entirely new to many developers.
Why This Matters
Failing to allocate enough time to learn JavaFX concepts can lead to frustration. Beginners often try to apply their existing knowledge from standard Java programming to JavaFX, which may not yield the expected results.
Tip: Start Slow
Take your time to understand the structure of JavaFX applications. Familiarize yourself with its core components:
- Stage: Represents the main window of your application.
- Scene: Contains all the graphical content.
- Node: Represents any visible component (buttons, text fields, etc.)
2. Ignoring the Scene Graph System
JavaFX utilizes a scene graph architecture, where every visual component is a node in a tree structure. It is essential to understand how this hierarchy works.
Example Code Snippet
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class SceneGraphExample extends Application {
@Override
public void start(Stage primaryStage) {
Button btn = new Button("Click Me");
btn.setOnAction(e -> System.out.println("Button clicked!"));
StackPane root = new StackPane();
root.getChildren().add(btn); // Adding button to the scene graph
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("Scene Graph Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why This Matters
In the example above, we create a basic JavaFX application where a button is added to the scene graph. Not understanding the significance of the scene graph can lead to misconfigurations and layout issues.
Tip: Visualize the Scene Graph
Use tools like Scene Builder, which provides a visual interface for designing your UI elements. This can help you visualize the scene graph and avoid errors in node management.
3. Misusing Threading
JavaFX is single-threaded, which means that any updates to the user interface (UI) must happen on the JavaFX Application Thread. Operating outside of this thread can cause unexpected behavior or crashes.
Example Code Snippet
import javafx.application.Application;
import javafx.application.Platform;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class ThreadingExample extends Application {
@Override
public void start(Stage primaryStage) {
Button btn = new Button("Click to Change Text");
btn.setOnAction(e -> {
// Running the UI update on the JavaFX Application Thread
Platform.runLater(() -> btn.setText("Updated!"));
});
StackPane root = new StackPane();
root.getChildren().add(btn);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("Threading Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why This Matters
If you attempt to manipulate UI components from a background thread, you might encounter runtime exceptions. The use of Platform.runLater()
ensures thread safety, allowing you to enact changes on the UI thread.
Tip: Always Know Your Thread Context
Make it a habit to understand which thread you're in while coding. This awareness can save you from threading issues that can emerge during the runtime of your application.
4. Overcomplicating Layouts
JavaFX offers multiple layout panes (like VBox, HBox, GridPane, etc.) that help arrange UI elements in a structured manner. However, many beginners tend to overcomplicate their layouts, leading to unnecessary complexity.
Why This Matters
Overusing nested layout panes can obscure your code and complicate maintenance. It is often more effective to use a single layout pane aligned with appropriate CSS for styling.
Tip: Use CSS for Styling
JavaFX supports CSS for styling components, which promotes cleaner and more maintainable code. Here’s a simple example:
.button {
-fx-background-color: #3498db;
-fx-text-fill: white;
-fx-font-size: 14px;
-fx-padding: 10px;
}
.button:hover {
-fx-background-color: #2980b9;
}
How to Apply CSS
You can apply this stylesheet to your JavaFX application as follows:
scene.getStylesheets().add("style.css");
This straightforward separation of concerns—where functionality and styling are managed independently—makes your application easier to manage.
5. Neglecting Documentation and Community Resources
JavaFX has extensive documentation, and the Java community is abundant with resources. Despite this, many beginners neglect these materials and try to work through problems independently.
Why This Matters
By overlooking the documentation, you may miss out on critical best practices or features that could simplify your coding process.
Tip: Make Use of Resources
- Official JavaFX Documentation: JavaFX Documentation
- Community Forums: Engaging with forums such as Stack Overflow or Reddit can provide insights and solutions to common problems.
My Closing Thoughts on the Matter
Starting with JavaFX 20 can open up a world of possibilities for Java developers. By being aware of these common pitfalls and addressing them early, you can accelerate your learning curve and create more robust applications.
Remember, persistence is key. Allow yourself the time and space to explore, make mistakes, and learn from them. Whether you are creating a simple UI or a complex application, understanding these aspects will greatly enhance your JavaFX journey.
So, what are you waiting for? Dive into JavaFX 20, keep these tips in mind, and start building engaging applications!