Extenders: Boosting Software or Harming Code Quality?
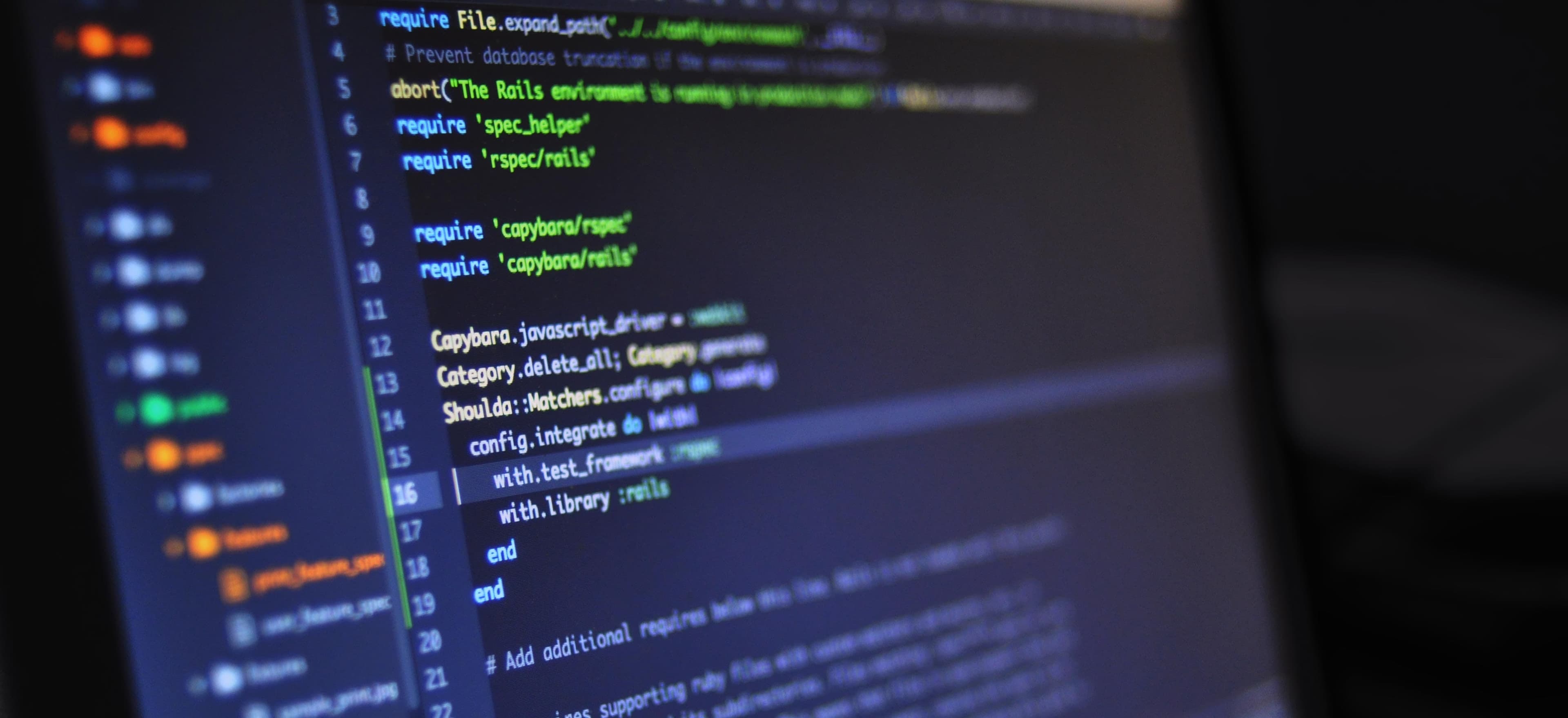
- Published on
Extenders: Boosting Software or Harming Code Quality?
In the realm of software development, the conversation around code quality frequently includes the notion of extensibility. Extensibility allows developers to add new capabilities to a software project. However, the methods employed to achieve extensibility can have a profound influence on the readability, maintainability, and overall quality of code. The concepts of extenders often emerge in this discussion, particularly in languages like Java.
What Are Extenders in Software Development?
At its core, an extender is a design concept that allows a system to be easily extended with new functionality. In Java, this is often achieved through the use of interfaces, abstract classes, or frameworks that permit plugin-style architecture.
Java Example: Using Interfaces to Extend Functionality
Let's consider a basic example demonstrating how to leverage interfaces to create a modular and easily extensible design.
// Define an interface for a Shape
public interface Shape {
double area();
}
// Implement the interface in different classes
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
}
public class Square implements Shape {
private double side;
public Square(double side) {
this.side = side;
}
@Override
public double area() {
return side * side;
}
}
// Using the shapes
public class Main {
public static void main(String[] args) {
Shape circle = new Circle(5);
Shape square = new Square(4);
System.out.println("Circle Area: " + circle.area());
System.out.println("Square Area: " + square.area());
}
}
Commentary on the Code
-
Interface Creation: The
Shape
interface establishes a contract for implementing classes. It enforces a consistent structure for various shapes. -
Extensibility: By adhering to the
Shape
interface, we can easily introduce new shapes (likeTriangle
,Rectangle
, etc.) without modifying existing code. -
Readability: This code remains organized and readable, making it straightforward for other developers to extend functionality in the future.
Pros and Cons of Using Extenders
It's vital to consider both the advantages and disadvantages of employing extenders in your software:
Pros:
- Improved Modularity: With a modular architecture, developers can add or modify features in isolation.
- Better Code Maintainability: Code changes can be confined to the relevant modules, leading to easier debugging and updates.
- Increased Flexibility: As requirements evolve, extensible software can adapt without significant overhauls.
Cons:
- Complexity: Introducing too many extensions can lead to convoluted architectures that are difficult to understand.
- Potential Performance Overhead: In some cases, a system designed for extensibility may incur performance penalties due to the added layers.
- Risk of Over-engineering: Sometimes, developers may create extensibility where it is not required, making the system unnecessarily complex.
The Role of Design Patterns
Design patterns play a significant role in enabling extensibility in a software project. Patterns such as the Factory Method, Strategy, and Observer are commonly employed to foster low coupling and high cohesion in extensible systems.
Factory Method Pattern Example
Consider the following implementation that uses the Factory Method pattern for creating shapes:
// ShapeFactory Interface
public interface ShapeFactory {
Shape createShape();
}
// CircleFactory Class
public class CircleFactory implements ShapeFactory {
private double radius;
public CircleFactory(double radius) {
this.radius = radius;
}
@Override
public Shape createShape() {
return new Circle(radius);
}
}
// SquareFactory Class
public class SquareFactory implements ShapeFactory {
private double side;
public SquareFactory(double side) {
this.side = side;
}
@Override
public Shape createShape() {
return new Square(side);
}
}
// Main Method to Utilize Factories
public class Main {
public static void main(String[] args) {
ShapeFactory circleFactory = new CircleFactory(5);
ShapeFactory squareFactory = new SquareFactory(4);
Shape circle = circleFactory.createShape();
Shape square = squareFactory.createShape();
System.out.println("Circle Area: " + circle.area());
System.out.println("Square Area: " + square.area());
}
}
Commentary on Factory Method
-
Decoupling Object Creation: The factories encapsulate the logic for creating shapes, ensuring that the main code remains free of instantiation logic.
-
Easier Extensions: New shapes can be introduced simply by adding new factory classes.
-
Single Responsibility Principle: Each factory has a clear responsibility, adhering to good software design practices.
Balancing Extenders with Code Quality
Given the pros and cons, developers must strike a balance between implementing extensibility and maintaining code quality.
Clear Documentation
Keep documentation up to date to ensure that other developers understand how to extend the application efficiently and correctly.
Code Reviews
Regularly conducting code reviews can help identify areas that may become complex with excessive extensibility. Use these sessions to refactor or clarify the existing implementation.
Techniques for Limiting Complexity
- Limit the Number of Extensions: Set guidelines on how many layers of abstraction are acceptable.
- Favor Composition Over Inheritance: This promotes flexibility without the pitfalls of a complex inheritance hierarchy.
To Wrap Things Up
The role of extenders in software development can be a double-edged sword. They have the potential to enhance functionality and contribute positively to code quality if implemented thoughtfully. However, mismanagement can lead to complexity that affects maintainability and readability.
As you navigate the crossroads of extensibility and quality, consider leveraging design patterns and clear structures while keeping an eye on the principles of good software design. Knowledge of Java's features and capabilities is also essential in this journey. For more in-depth reading, check this guide on Java design patterns or explore the principles of clean code.
By harnessing the power of extensibility while vigilantly maintaining code quality, you can create software solutions that stand the test of time. Happy coding!