Mastering Spring Boot Controllers with Custom Parameters
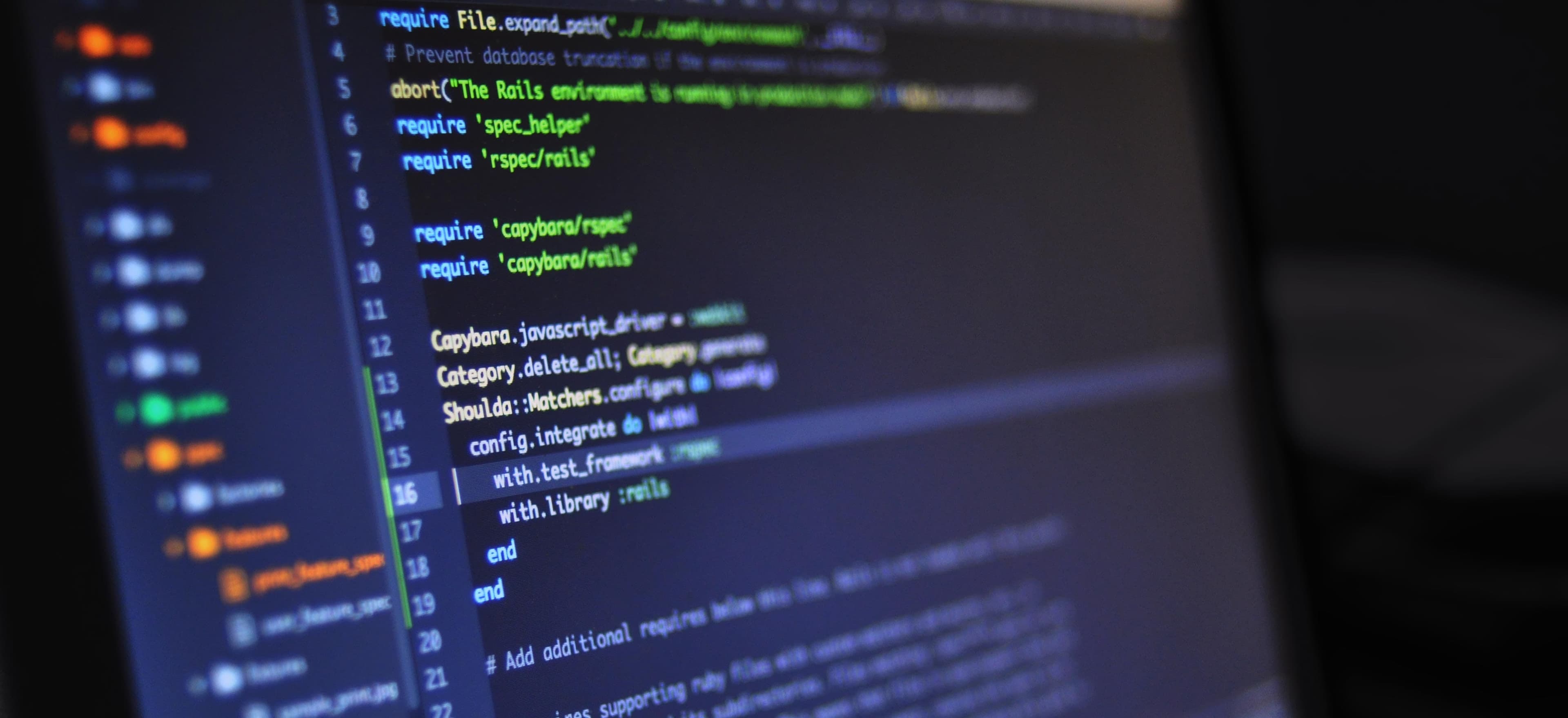
- Published on
Mastering Spring Boot Controllers with Custom Parameters
Spring Boot has revolutionized how we develop Java applications by simplifying the setup and configuration aspects. One of its essential features lies in the use of controllers to handle web requests. The ability to create custom parameters within these controllers can significantly enhance the functionality of your application, making it more interactive and dynamic. In this post, we'll explore how to create and utilize custom parameters in Spring Boot controllers, with examples along the way.
Establishing the Context to Spring Boot Controllers
Before delving into custom parameters, it’s crucial to establish what a Spring Boot controller is. A controller is a central component in any MVC (Model-View-Controller) architecture, typically annotated with @RestController
or @Controller
. It serves as the endpoint that processes incoming HTTP requests, interacts with the service layer, and sends responses back to the client.
Basic Structure of a Spring Boot Controller
Here is a simple example of a Spring Boot controller:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api")
public class MyController {
@GetMapping("/hello")
public String hello() {
return "Hello, World!";
}
}
In this code:
- We define a REST controller using the
@RestController
annotation. - The class is mapped to
/api
, making it easily extendable for various endpoints. - A simple GET request to
/hello
returns a greeting message.
Custom Parameters in Spring Boot Controllers
Custom parameters allow for greater flexibility in APIs, enabling you to handle various types of input from clients. We can collect parameters from the request body, path variables, and query strings. Let's explore how to work with these parameters effectively.
Handling Path Variables
Path variables are part of the URL and allow you to capture dynamic values within a request path. Here’s how to utilize them in a Spring Boot controller.
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/users/{id}")
public String getUserById(@PathVariable String id) {
return "User with ID: " + id;
}
}
Explanation of Code:
- The
@GetMapping("/users/{id}")
annotation sets up a route that includes a path variable. - The method
getUserById
takesid
as a path variable, which is automatically extracted from the URL. - This structure provides a clear and intuitive way to retrieve users based on their unique identifiers.
Using Query Parameters
Query parameters allow you to pass optional metadata for your requests. They appear after a question mark in the URL, and multiple parameters can be passed as key-value pairs.
Here’s how to implement query parameters in your controller:
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api")
public class SearchController {
@GetMapping("/search")
public String search(@RequestParam String query,
@RequestParam(defaultValue = "10") int limit) {
return "Searching for: " + query + " with limit: " + limit;
}
}
Code Breakdown:
- The
@RequestParam
annotation enables you to extract query parameters. In this case,query
is a mandatory parameter, whilelimit
has a default value if not provided. - This allows users to search for items easily, with a predetermined limit on the number of results.
Handling Request Bodies
Sometimes, you need to receive a more complex data structure. This is where the request body comes into play, typically in POST or PUT requests.
Here’s an example where we use a request body:
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api")
public class ProductController {
@PostMapping("/products")
public String addProduct(@RequestBody Product product) {
return "Added Product: " + product.getName();
}
}
class Product {
private String name;
private double price;
// Getters and Setters
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
Explanation:
- The
@PostMapping("/products")
annotation creates a single endpoint to manage product creation. - The
Product
class is a simple POJO that maps to the incoming JSON object. - The use of
@RequestBody
enables automatic deserialization from JSON to your Java object, streamlining data handling.
Validating Custom Parameters
Validating custom parameters ensures your application behaves as expected and enhances security. To implement validation, you can use annotations from the javax.validation
package:
import org.springframework.validation.annotation.Validated;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Min;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api")
@Validated
public class OrderController {
@PostMapping("/orders")
public String placeOrder(@RequestBody @Valid Order order) {
return "Order for " + order.getItem() + " placed successfully.";
}
}
class Order {
@NotNull
private String item;
@Min(1)
private int quantity;
// Getters and Setters
public String getItem() {
return item;
}
public void setItem(String item) {
this.item = item;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
}
Code Insights:
- Here we use
@Validated
at the class level to enable validation. - The
@NotNull
and@Min
annotations ensure the incoming data meets the specified criteria. - This practice prevents erroneous data from being processed further in your application.
The Last Word
Creating custom parameters in Spring Boot controllers elevates the capability of your RESTful services. By leveraging path variables, query parameters, and request bodies, you can create dynamic and robust APIs capable of handling diverse scenarios. Additionally, integrating validation practices enhances data integrity and security.
For deeper insights into Spring Boot and REST design practices, refer to the Spring Boot Documentation, or explore Designing APIs with Spring Boot.
By mastering these concepts, you elevate your Java programming skills, turning simple applications into meaningful, user-centric experiences. Happy coding!
Checkout our other articles