Choosing Between Object-Oriented and Functional Programming
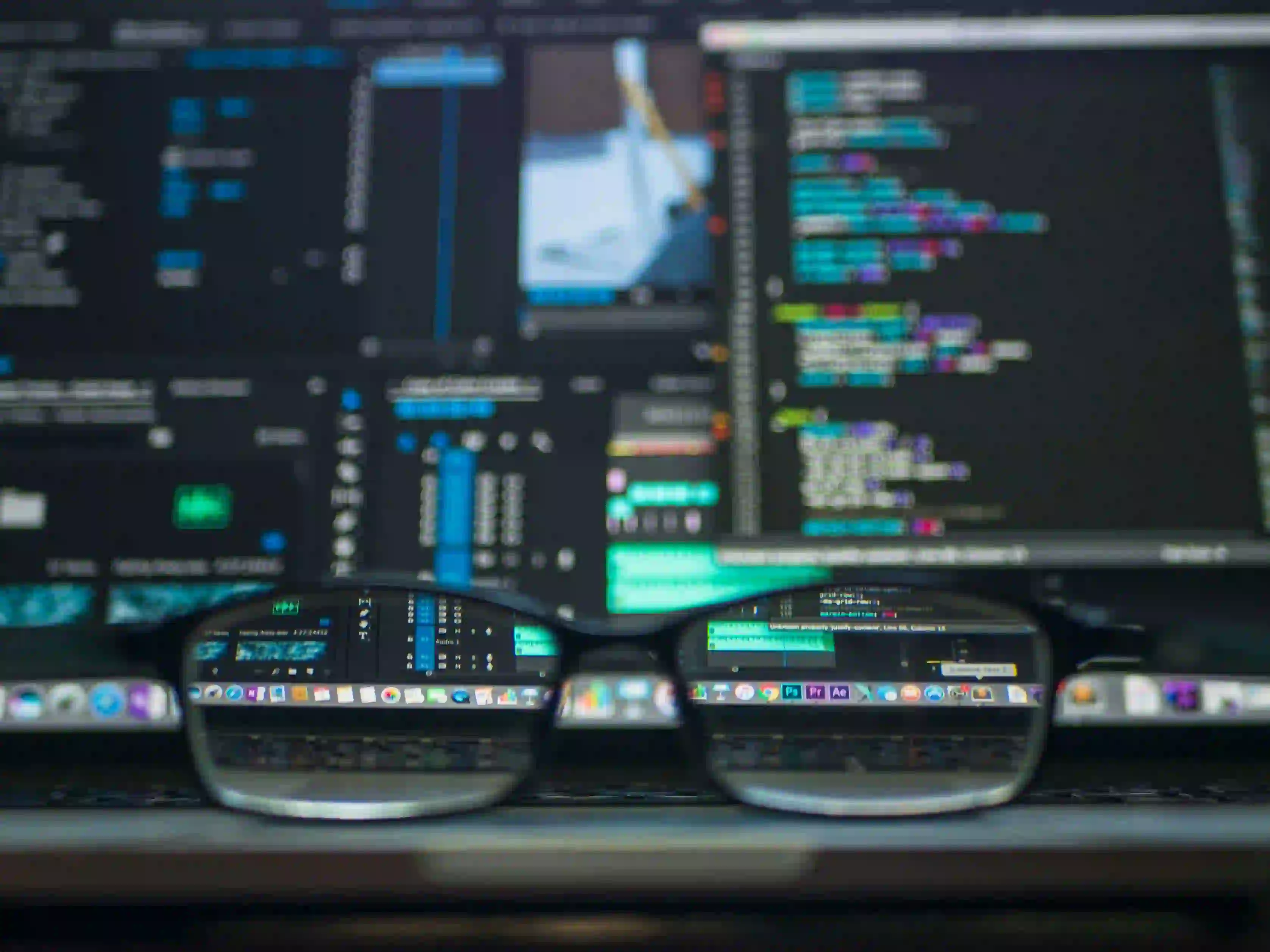
Choosing Between Object-Oriented and Functional Programming
In the realm of programming languages, developers often find themselves at a crossroads when deciding how to structure their code. Among the most widely debated paradigms are Object-Oriented Programming (OOP) and Functional Programming (FP). Both have their merits and drawbacks, influencing the way applications are designed and maintained. In this blog post, we will explore these two programming paradigms, examine their key characteristics, and help you make an informed choice for your next project.
Understanding Object-Oriented Programming
Object-Oriented Programming revolves around the concept of "objects," which are instances of classes. These objects encapsulate data (attributes) and methods (functions) that operate on the data. The key features of OOP include:
- Encapsulation: Bundling the data and methods that operate on the data, hiding the internal state of the object from the outside world.
- Inheritance: Allowing a new class to inherit the properties and behaviors of an existing class.
- Polymorphism: Enabling different classes to be treated as instances of the same class through a common interface.
Here is a simple example demonstrating encapsulation and inheritance in Java:
// Base class
class Animal {
protected String name;
public Animal(String name) {
this.name = name;
}
public void makeSound() {
System.out.println("Animal makes sound");
}
}
// Subclass
class Dog extends Animal {
public Dog(String name) {
super(name);
}
@Override
public void makeSound() {
System.out.println(name + " barks");
}
}
// Demo
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog("Buddy");
myDog.makeSound(); // Output: Buddy barks
}
}
In this example, we create an Animal
base class and a Dog
subclass. The Dog
class inherits properties from Animal
and overrides the makeSound
method specific to dogs.
Advantages of Object-Oriented Programming
- Modularity: OOP facilitates code organization by keeping related properties and behaviors together in classes.
- Reusability: Classes can be reused across different parts of an application, leading to reduced redundancy.
- Maintainability: Changes in one part of the application can be made with minimal impact on other parts.
Disadvantages of Object-Oriented Programming
- Complexity: OOP can introduce unnecessary complexity, especially in larger systems.
- Performance: The abstraction layers present in OOP can result in performance overhead compared to procedural programming.
Understanding Functional Programming
Functional Programming, on the other hand, emphasizes the use of pure functions and immutable data. The focus in FP is on what to solve rather than how to solve it. Key features include:
- First-Class Functions: Functions are treated as first-class citizens, allowing them to be passed as arguments or returned from other functions.
- Immutability: Once created, data cannot be changed, which helps avoid side effects.
- Higher-Order Functions: Functions that take other functions as arguments or return them as results, enabling powerful abstractions.
Here is a simple example of functional programming concepts using Java (Java introduced functional programming aspects in Java 8):
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using a higher-order function to get the square of each number
List<Integer> squares = numbers.stream()
.map(num -> num * num) // Lambda expression for square
.collect(Collectors.toList());
System.out.println(squares); // Output: [1, 4, 9, 16, 25]
}
}
In this example, we use Java's Stream API to apply a function (squaring each number) to a list of integers. By using a lambda expression, we have effectively stated what we want without worrying about how the transformation occurs step-by-step.
Advantages of Functional Programming
- Simplicity: By avoiding mutable state and side effects, FP leads to simpler code and easier reasoning about program logic.
- Concurrency: Immutability makes it easier to run the program in parallel and enhances safety.
Disadvantages of Functional Programming
- Learning Curve: The paradigm shift from imperative to functional programming requires a different mindset.
- Performance: Due to its emphasis on immutability, FP can lead to overhead in memory consumption.
When to Choose Object-Oriented Programming
Choosing OOP is often appropriate in scenarios where:
- The project has a lot of interconnected components that benefit from organized classes and easily manageable state.
- The application is naturally represented in hierarchical structures, such as GUI applications, simulations, or game development.
When to Choose Functional Programming
Conversely, opt for FP in cases where:
- The application processes a lot of data where the transformation is the primary operation.
- You are working in an environment that supports concurrency, such as data science or real-time applications.
Bridging the Two Paradigms
Interestingly, many modern programming languages encompass features from both paradigms. Java, for instance, includes functional programming capabilities while remaining fundamentally object-oriented. This flexibility allows developers to use the strengths of both approaches cohesively.
For a more extensive study on the concepts of functional programming versus object-oriented programming, consider checking out Functional Programming in Java by Venkat Subramaniam, which dives deeper into how these paradigms can be utilized effectively together.
My Closing Thoughts on the Matter
Choosing between Object-Oriented and Functional Programming is essential for any developer seeking to create efficient, maintainable, and scalable applications. Understanding the strengths and weaknesses of both paradigms can guide you toward the most appropriate approach based on project requirements.
Whether you find yourself leaning towards the organized structure of OOP or the elegant simplicity of FP, embracing the nuances of each programming paradigm will ultimately enhance your skills and broaden your toolkit.
Continuous learning and exploring these paradigms through practice will lead to not only healthier codebases but also a deeper understanding of the principles that govern software development. Always remember: the best tool is the one that fits the job. Happy coding!