Mastering Common Design Patterns: Key Interview Questions
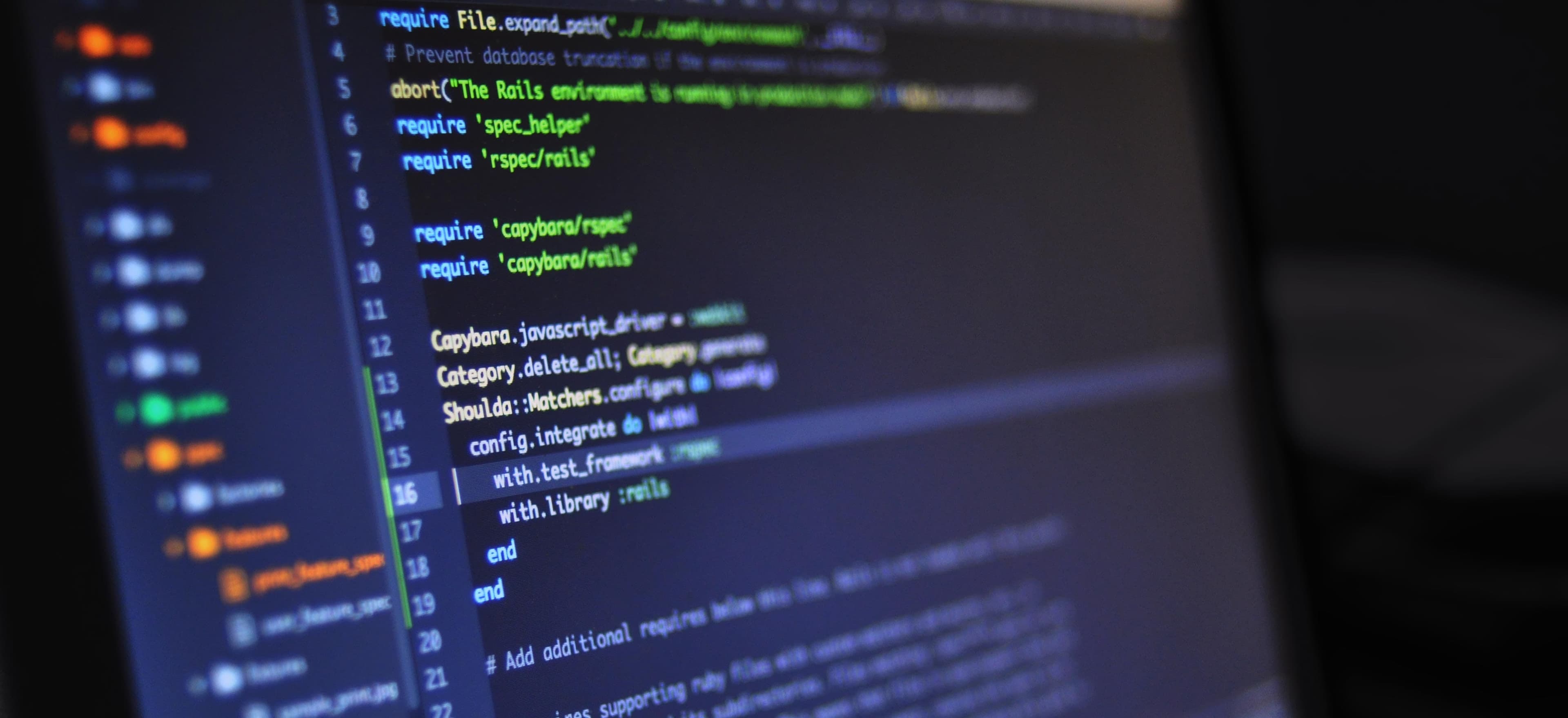
- Published on
Mastering Common Design Patterns: Key Interview Questions
In the world of software development, understanding design patterns is not just advantageous; it is often critical for success. Design patterns provide proven solutions to common problems and can make your code more efficient, maintainable, and scalable. Furthermore, interviewers frequently assess candidates on their knowledge of design patterns during technical interviews.
This blog post aims to cover the most common design patterns, the key interview questions you might encounter, and provide insights into why these patterns matter.
Table of Contents
- What are Design Patterns?
- Why are Design Patterns Important?
- Types of Design Patterns
- Creational Patterns
- Structural Patterns
- Behavioral Patterns
- Common Design Patterns and Key Interview Questions
- Singleton
- Factory Method
- Observer
- Strategy
- Conclusion
What are Design Patterns?
Design patterns are general reusable solutions to recurring problems in software design. They represent best practices that experienced developers have learnt over time. While they are not finished designs that can be directly transformed into code, they serve as templates or guidelines for creating object-oriented code in various scenarios.
Why are Design Patterns Important?
Design patterns can significantly enhance your software development process for several reasons:
- Reusability: Patterns can often be reused across different projects, saving development time.
- Maintainability: Code that adheres to well-known patterns is generally easier to read and understand.
- Scalability: Designs that follow these patterns can adapt more easily to changes.
In interviews, showcasing your understanding of design patterns can demonstrate your ability to write clean and efficient code, which is a key desirable trait for software developers.
Types of Design Patterns
Design patterns can be categorized into three main types:
- Creational Patterns: Deal primarily with object creation mechanisms.
- Structural Patterns: Focus on how objects are composed to form larger structures.
- Behavioral Patterns: Concerned with communication between objects.
Creational Patterns
Creational patterns deal with object creation, allowing for flexibility and reuse of code. Some common creational patterns include:
- Singleton
- Factory Method
Structural Patterns
Structural patterns mainly focus on the composition of classes and objects. Notable structural patterns include:
- Adapter
- Decorator
Behavioral Patterns
Behavioral patterns deal with object collaboration and responsibility. Important behavioral patterns comprise:
- Observer
- Strategy
Common Design Patterns and Key Interview Questions
Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to it. This is particularly useful when managing resources, such as database connections.
Example Code
public class Singleton {
private static Singleton instance;
private Singleton() { }
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Commentary
In the above example, the private constructor prevents other classes from instantiating the Singleton class directly. Instead, a public static method, getInstance
, is provided to manage the instantiation. This is crucial for resource management scenarios.
Interview Question
- What are the pros and cons of using the Singleton pattern?
- Pros: Global access, controlled instantiation.
- Cons: Difficulties with testing and potential for global state.
Factory Method Pattern
The Factory Method pattern defines an interface for creating objects but allows subclasses to alter the type of objects that will be created. This promotes loose coupling by minimizing dependencies between classes.
Example Code
public abstract class Product {
public abstract void use();
}
public class ConcreteProductA extends Product {
public void use() {
System.out.println("Using Product A");
}
}
public class ConcreteProductB extends Product {
public void use() {
System.out.println("Using Product B");
}
}
public abstract class Creator {
public abstract Product factoryMethod();
}
public class ConcreteCreatorA extends Creator {
public Product factoryMethod() {
return new ConcreteProductA();
}
}
public class ConcreteCreatorB extends Creator {
public Product factoryMethod() {
return new ConcreteProductB();
}
}
Commentary
In this example, we have a Creator
class and its subclasses ConcreteCreatorA
and ConcreteCreatorB
. This separation allows for different Product
creations without altering the client code. It exemplifies the open/closed principle of object-oriented design.
Interview Question
- How does the Factory Method pattern promote loose coupling?
- By treating the creation of objects through an interface, which reduces dependency on concrete classes.
Observer Pattern
The Observer pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. It is commonly used in event handling systems.
Example Code
import java.util.ArrayList;
import java.util.List;
interface Observer {
void update(String message);
}
class ConcreteObserver implements Observer {
private String name;
public ConcreteObserver(String name) {
this.name = name;
}
public void update(String message) {
System.out.println(name + " received: " + message);
}
}
class Subject {
private List<Observer> observers = new ArrayList<>();
public void addObserver(Observer observer) {
observers.add(observer);
}
public void notifyObservers(String message) {
for (Observer observer : observers) {
observer.update(message);
}
}
public void changeState(String message) {
System.out.println("State changed: " + message);
notifyObservers(message);
}
}
Commentary
In this Observer pattern implementation, the Subject
maintains a list of observers and notifies them when its state changes. This decoupling allows adding or removing observers without affecting the subject itself.
Interview Question
- Can you explain a real-world scenario where the Observer pattern would be a suitable choice?
- Situations like a stock market application where users need real-time updates about stock price changes.
Strategy Pattern
The Strategy pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. It lets the algorithm vary independently from clients that use it.
Example Code
interface Strategy {
int execute(int a, int b);
}
class AdditionStrategy implements Strategy {
public int execute(int a, int b) {
return a + b;
}
}
class SubtractionStrategy implements Strategy {
public int execute(int a, int b) {
return a - b;
}
}
class Context {
private Strategy strategy;
public Context(Strategy strategy) {
this.strategy = strategy;
}
public int executeStrategy(int a, int b) {
return strategy.execute(a, b);
}
}
Commentary
In this example, different computation strategies (Addition or Subtraction) are implemented and can be used interchangeably without modifying the client code. This illustrates the flexibility and ease of extending functionality.
Interview Question
- How does the Strategy pattern help to minimize conditional statements?
- By encapsulating the algorithms in separate strategy classes, it eliminates the need for conditional logic to choose which algorithm to apply.
Key Takeaways
Understanding design patterns is crucial not just for acing interviews but for writing clean, maintainable, and future-proof code. Familiarity with patterns like Singleton, Factory Method, Observer, and Strategy empowers you to tackle various design challenges with confidence.
As software systems grow in complexity, the insights derived from applying these patterns become invaluable. They not only improve your coding skills but also elevate your overall approach to software design.
To learn more about design patterns, check out Design Patterns Explained by Eric Gamma et al., which serves as a classic reference.
Now, go ahead and deepen your knowledge by practicing these patterns in your projects, and you will find yourself preparing well for any software development interviews. Happy coding!
Checkout our other articles