Common JAX-RS Annotation Mistakes to Avoid
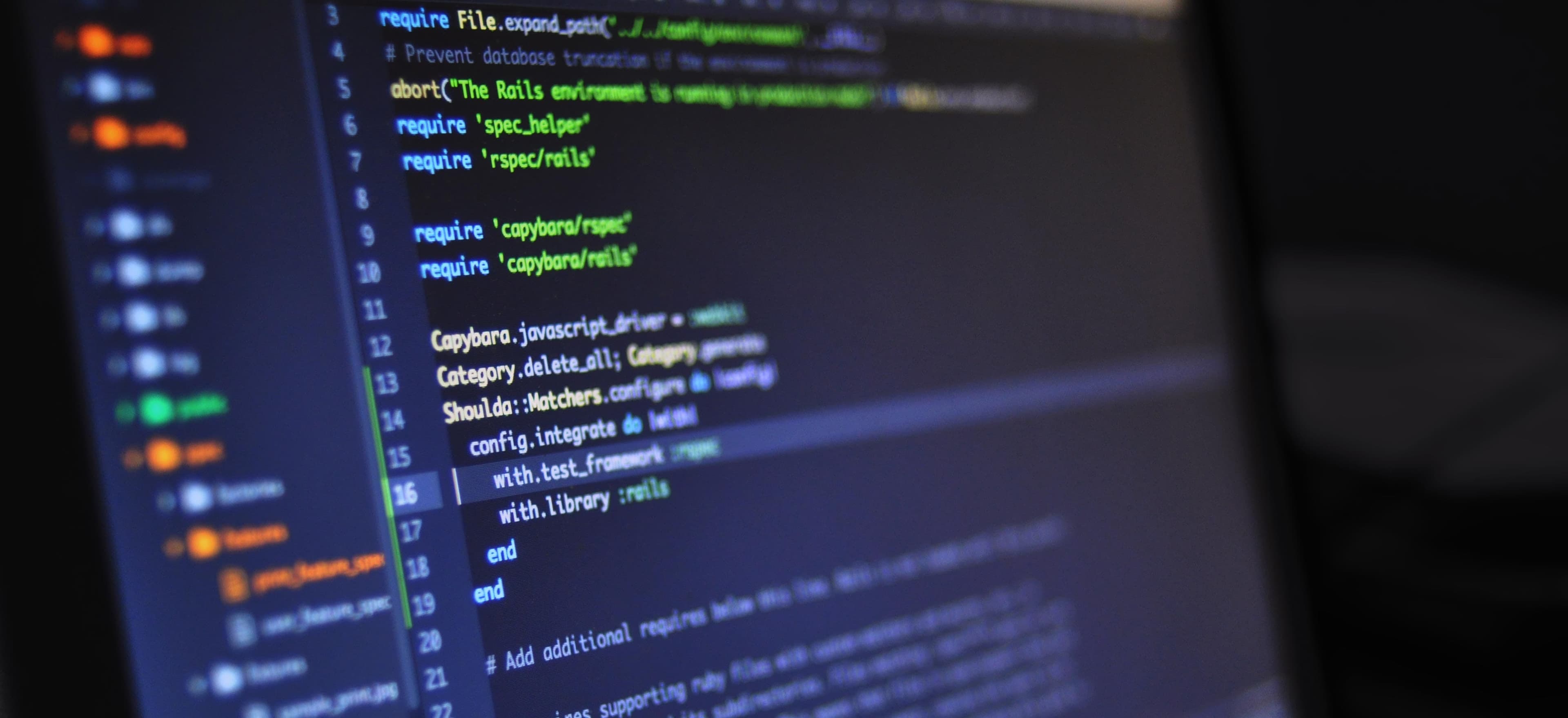
- Published on
Common JAX-RS Annotation Mistakes to Avoid
Java has become one of the leading programming languages in the world, and JAX-RS (Java API for RESTful Web Services) plays a crucial role in simplifying RESTful web service development. However, even seasoned developers can trip up when using JAX-RS annotations. This blog post will discuss common mistakes you should avoid when working with JAX-RS annotations, providing clarity and best practices to enhance your development experience.
Understanding JAX-RS Annotations
JAX-RS annotations are used to define resources and configure how HTTP requests are handled. The core annotations include:
@Path
- Specifies the URI path for a resource.@GET
,@POST
,@PUT
,@DELETE
- Indicate the HTTP methods the resource can handle.@Consumes
and@Produces
- Define the media types that the resource can consume and produce.
Before diving into mistakes, let’s consider a basic JAX-RS example to set the context.
Basic JAX-RS Example
import javax.ws.rs.*;
import javax.ws.rs.core.MediaType;
@Path("/users")
public class UserResource {
@GET
@Produces(MediaType.APPLICATION_JSON)
public List<User> getUsers() {
// Returns a list of users in JSON format
return userService.getAllUsers();
}
}
In this example, we define a resource to handle HTTP GET requests on the /users
path, producing a JSON representation of a list of users.
Common Mistakes to Avoid
1. Overlooking the Use of @Path
Annotation
One common mistake is neglecting the @Path
annotation on resource classes and methods. Every JAX-RS resource needs a path; otherwise, it becomes inaccessible.
Example of Mistake
public class UserResource {
@GET
public List<User> getUsers() {
// Error: Missing @Path annotation
}
}
Correct Approach
@Path("/users")
public class UserResource {
@GET
public List<User> getUsers() {
return userService.getAllUsers();
}
}
Make sure every method and class that should handle REST calls has the @Path
annotation.
2. Ignoring HTTP Method Specificity
When using multiple HTTP method annotations (e.g., @GET
, @POST
, etc.), it's crucial to ensure they match the intended functionality.
Example of Mistake
@Path("/users")
public class UserResource {
@POST
public void getUsers() { // Mistakenly using @POST for a GET action
// Should handle creating a user, not retrieving
}
}
Correct Approach
@Path("/users")
public class UserResource {
@GET
public List<User> getUsers() {
return userService.getAllUsers();
}
@POST
public Response createUser(User user) {
return Response.status(Response.Status.CREATED).entity(userService.createUser(user)).build();
}
}
Clear method intentions help maintain code clarity and adherence to REST principles.
3. Misusing @Consumes
and @Produces
Another frequent error lies in misappropriating these annotations. They dictate what data your service can accept or return, and failure to implement them appropriately can lead to communication breakdowns between the client and server.
Example of Mistake
@POST
@Consumes(MediaType.TEXT_PLAIN) // Limitations on data format can cause issues
public Response createUser(User user) {
// This should handle more complex JSON data.
}
Correct Approach
@POST
@Consumes(MediaType.APPLICATION_JSON) // Accepts JSON data
@Produces(MediaType.APPLICATION_JSON) // Returns JSON response
public Response createUser(User user) {
userService.createUser(user);
return Response.status(Response.Status.CREATED).entity(user).build();
}
Choosing the correct media types ensures that your service interacts effectively with clients.
4. Neglecting Error Handling and Response Codes
Developers sometimes forget to include appropriate HTTP response codes and error handling in their JAX-RS methods. This impacts the usability of your API significantly.
Example of Mistake
@GET
@Path("{id}")
public User getUser(@PathParam("id") String id) {
return userService.findUserById(id); // No error handling
}
Correct Approach
@GET
@Path("{id}")
@Produces(MediaType.APPLICATION_JSON)
public Response getUser(@PathParam("id") String id) {
User user = userService.findUserById(id);
if (user == null) {
return Response.status(Response.Status.NOT_FOUND).build();
}
return Response.ok(user).build();
}
5. Failing to Utilize Dependency Injection Properly
Using JAX-RS alongside a framework like CDI (Contexts and Dependency Injection) can simplify development by managing object lifecycles. Failing to leverage this can lead to manual object creation and hinder code maintainability.
Example of Mistake
@Path("/users")
public class UserResource {
private UserService userService = new UserService(); // Manual instantiation
@GET
public List<User> getUsers() {
return userService.getAllUsers();
}
}
Correct Approach
import javax.inject.Inject;
@Path("/users")
public class UserResource {
@Inject // Automatic dependency injection
private UserService userService;
@GET
public List<User> getUsers() {
return userService.getAllUsers();
}
}
Using dependency injection not only cleans up your resource classes but also adds testability and flexibility to your code.
6. Not Grouping Similar Resources
To improve the organization and readability of your API, it’s a good practice to group similar resources under a common parent path. Failing to do so can lead to cluttered URI patterns.
Example of Mistake
@Path("/users")
public class UserResource {
// Class handling user-related requests
}
@Path("/orders")
public class OrderResource {
// Class handling order-related requests
}
Correct Approach
@Path("/api") // Introducing a common base path
public class UserResource {
@Path("/users")
// User-related resource methods
}
@Path("/api") // Same base path
public class OrderResource {
@Path("/orders")
// Order-related resource methods
}
This structure promotes better API navigation and organization.
The Bottom Line
Mastering JAX-RS annotations can significantly enhance your RESTful web service development. By avoiding these common mistakes, you streamline your code, improve performance, and reduce maintenance efforts. Always remember to utilize proper annotations, maintain clear structures, and handle errors gracefully.
For deeper insights into JAX-RS, you may find these resources valuable:
- JAX-RS Official Documentation
- Understanding RESTful Services through JAX-RS
Embrace these practices to elevate your Java web service development and create APIs that stand the test of time. Happy coding!
Checkout our other articles