Navigating Pitfalls of Default Methods in Java Interfaces
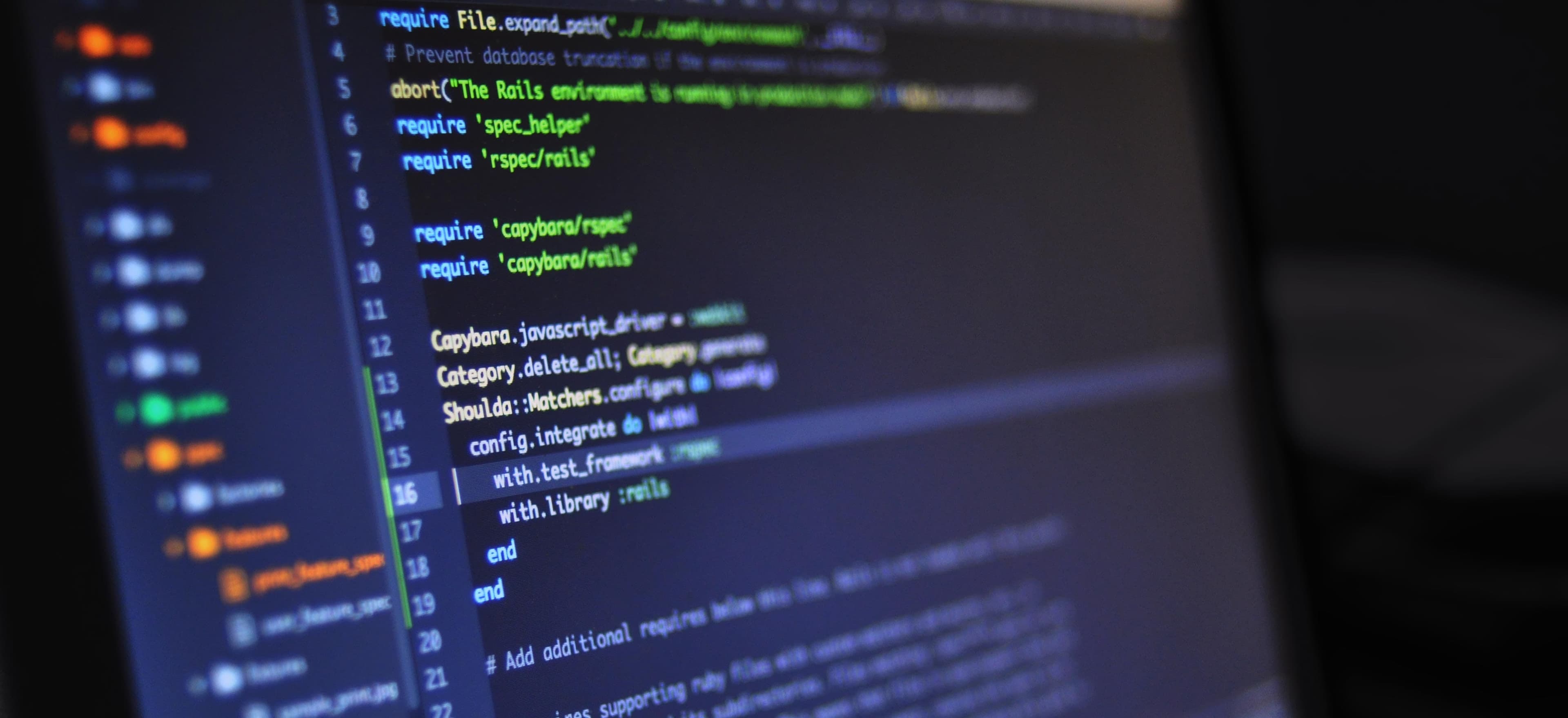
- Published on
Navigating Pitfalls of Default Methods in Java Interfaces
Java 8 ushered in a significant evolution in the language with the introduction of default methods in interfaces. While this feature offers developers enhanced flexibility in designing APIs, it can also lead to a series of challenges if not properly understood and utilized. This blog post will walk you through the intricacies of default methods - their benefits, potential pitfalls, and best practices to keep in mind.
What are Default Methods?
Before diving into the pitfalls, let's clarify what default methods are. A default method in a Java interface provides a way to add new methods to interfaces without breaking the existing implementations. By using the default
keyword, developers can define a method body within the interface itself. This is particularly useful for maintaining backward compatibility.
public interface Vehicle {
default void start() {
System.out.println("Vehicle is starting.");
}
void stop();
}
class Car implements Vehicle {
@Override
public void stop() {
System.out.println("Car is stopping.");
}
}
Why Use Default Methods?
- Backwards Compatibility: Default methods allow the addition of new functionality to interfaces without disrupting existing implementations.
- Code Reusability: Common functionality can be provided in the interface, preventing code duplication.
- Ease of Adoption: New features can be introduced gradually; old clients can continue to operate without modification.
Common Pitfalls
With every feature, certain pitfalls should be navigated carefully. Here are some commonly encountered issues related to default methods:
1. Diamond Problem
One of the most notorious issues with default methods is the diamond problem, which occurs when a class inherits multiple interfaces that have the same default method. This creates ambiguity for the Java compiler.
interface A {
default void show() {
System.out.println("A");
}
}
interface B extends A {
default void show() {
System.out.println("B");
}
}
interface C extends A {
default void show() {
System.out.println("C");
}
}
// Ambiguous class
class D implements B, C {
@Override
public void show() {
B.super.show(); // Explicitly choose B's implementation
}
}
Commentary: In the example above, class D
must explicitly override the show()
method to resolve the ambiguity when implementing interfaces B
and C
. Failing to do so will result in a compilation error.
2. Undesired Method Overriding
Another pitfall is the unintended overriding of default methods. When a class implements an interface with a default method, it may unintentionally override it, resulting in loss of original functionality.
class E implements A {
// This method overrides the default implementation
@Override
public void show() {
System.out.println("Custom E");
}
}
Commentary: By overriding the show()
method, E
forgoes the default behavior of A
. This might not only cause confusion but can also lead to functionality being lost unintentionally.
3. Complex Inheritance Trees
Utilizing default methods can lead to more complex inheritance trees, which can complicate maintenance and understanding of the code.
interface X {
default void display() {
System.out.println("X");
}
}
interface Y extends X {
default void display() {
System.out.println("Y");
}
}
interface Z extends X {
// No default display method
}
class MyClass implements Y, Z {
@Override
public void display() {
Y.super.display(); // Must resolve ambiguity.
}
}
Commentary: In this case, MyClass implements both Y and Z, but it must clarify which display method to use. Such situations can lead to increased cognitive load on developers and make the application harder to maintain.
4. Performance Considerations
While default methods can reduce boilerplate code and improve readability, they may introduce some overhead due to virtual method calls. Each call to a default method involves additional indirection compared to calling a static method.
A misuse of default methods, especially in performance-sensitive sections of code, may lead to performance degradation.
Best Practices
To navigate these pitfalls effectively, consider the following best practices:
- Use Default Methods Sparingly: Only when absolutely necessary for backward compatibility or code reuse.
- Document Behavior Clearly: Ensure that it’s clear what the default method does and how it should be overridden.
- Favor Composition Over Inheritance: Sometimes, using composition can solve problems that arise from defaults in inheritance hierarchies.
- Refactor Default Methods: If a default method is overly complex, consider refactoring it into a utility class or helper methods.
The Closing Argument
Default methods in Java interfaces can greatly enhance an API's flexibility and usability. However, they come with their unique set of challenges. By being aware of these pitfalls and following best practices, developers can leverage default methods effectively while maintaining clean and manageable code.
For further reading on interfaces in Java and their evolution, check out the Java Documentation and learn about the Java Interface Definition.
Engaging with Java's features requires a thoughtful approach to ensure that the flexibility offered by the language does not compromise the clarity, maintainability, and performance of your applications.
Checkout our other articles