Boost Your Groovy Scripts with Picocli: Common Pitfalls
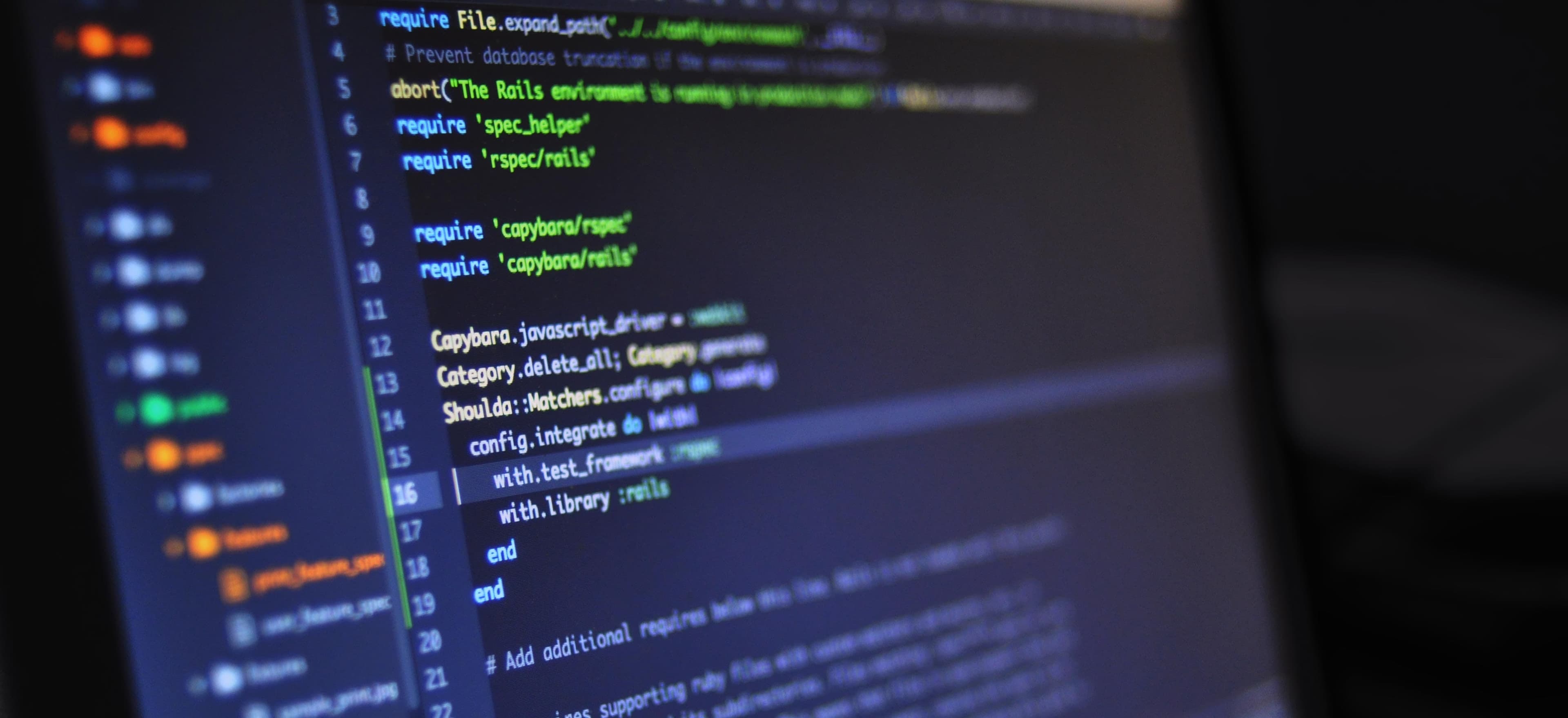
- Published on
Boost Your Groovy Scripts with Picocli: Common Pitfalls
When developing command-line applications in Groovy, enhancing the ease of argument parsing and command-line interaction is essential. Many developers turn to libraries like Picocli for this purpose. Picocli is a powerful Java library that helps you create console applications with a wealth of features. This blog post will delve into common pitfalls you may encounter while using Picocli with Groovy scripts and provide insights on how to overcome them.
Table of Contents
- What is Picocli?
- Setting Up Picocli in Your Groovy Project
- Common Pitfalls
- Not Using Annotations Correctly
- Ignoring Default Values
- Overlooking Exception Handling
- Best Practices When Using Picocli
- Conclusion
What is Picocli?
Picocli is a command-line parsing library for Java that makes it easy to create rich command-line applications. It supports a wide variety of argument types, complex command hierarchies, and automatic help generation. For Groovy developers, it integrates seamlessly, bringing robust capabilities to enhance user interaction.
For more information about Picocli, check out the official Picocli documentation.
Setting Up Picocli in Your Groovy Project
To get started with Picocli in your Groovy project, you first need to include the dependency using either Gradle or Maven. Here’s how you can do it using Gradle:
dependencies {
implementation 'info.picocli:picocli:4.6.1'
}
Once you have Picocli included in your project, you can begin defining your command-line interface (CLI) classes.
Sample Code Snippet
Here's a small example of a command-line application using Picocli:
import picocli.CommandLine
import picocli.CommandLine.Command
import picocli.CommandLine.Option
@Command(name = 'calculator', description = 'Performs basic arithmetic operations')
class Calculator implements Runnable {
@Option(names = '-a', description = 'First operand')
double operandA
@Option(names = '-b', description = 'Second operand')
double operandB
@Option(names = '-o', description = 'Operation (add, subtract, multiply, divide)')
String operation
void run() {
switch (operation) {
case 'add':
println "Result: ${operandA + operandB}"
break
case 'subtract':
println "Result: ${operandA - operandB}"
break
case 'multiply':
println "Result: ${operandA * operandB}"
break
case 'divide':
if (operandB != 0) {
println "Result: ${operandA / operandB}"
} else {
println "Error: Division by zero"
}
break
default:
println "Invalid operation: $operation"
}
}
}
// Entry point
CommandLine.run(new Calculator(), args)
Why This Code Matters
In this example:
- The
Command
annotation specifies the command's name and description for help generation. - The
Option
annotation defines the command-line options. Each option corresponds to a field in your class. - The
run()
method handles the application logic based on the options provided by the user.
This structure not only enhances readability but also utilizes Picocli's powerful built-in features.
Common Pitfalls
While working with Picocli, you might encounter several pitfalls. Let's explore a few of the more common ones.
Not Using Annotations Correctly
One of the most frequent mistakes is misusing or neglecting annotations altogether. Picocli relies heavily on annotations to determine how to parse command-line inputs.
Example:
If you forget to annotate a method or property with @Option
, Picocli won't recognize it, leaving you with unhandled command-line arguments.
Ignoring Default Values
Default values are essential for command-line applications, enabling smoother user experiences by allowing options to be omitted. Failing to set default values can lead to exceptions or unwanted behavior.
Example:
In the earlier code snippet, you can add a default value for the operation:
@Option(names = '-o', description = 'Operation (add, subtract, multiply, divide)', defaultValue = "add")
String operation
Overlooking Exception Handling
Exception handling is crucial for providing user-friendly messages when something goes wrong. If you do not catch and handle errors effectively, it can lead to poor user experiences.
Example:
Taking the division scenario from earlier, ensure to handle cases where operandB
is zero:
case 'divide':
if (operandB == 0) {
println "Error: Division by zero not allowed!"
} else {
println "Result: ${operandA / operandB}"
}
break
Best Practices When Using Picocli
-
Use Annotations Wisely: Make full use of the various annotations Picocli provides, such as
@Command
,@Option
, and@Parameters
. -
Set Defaults: Always provide default values where applicable to streamline the user experience.
-
Implement Validation: Simple validation checks can mitigate potential issues. For example, if you're expecting a numeric value, make sure the user input is validated.
-
Utilize Help Command: Picocli automatically generates help commands. Always ensure your application provides this feature to guide users.
-
Read the Documentation: As with any library, make sure to read the Picocli documentation for best practices and updates.
In Conclusion, Here is What Matters
Picocli offers a powerful solution for building command-line applications in Groovy. While it simplifies many aspects of argument parsing and command execution, being aware of common pitfalls is essential to avoid frustration and enhance user experience.
By correctly utilizing annotations, setting default values, appropriately handling exceptions, and adhering to best practices, you can significantly improve your Groovy script's effectiveness. With these insights, you are now better equipped to leverage Picocli for your command-line applications.
Embrace the power of Picocli and change the way you build CLI applications in Groovy today!
Checkout our other articles