Continuous Delivery vs. Continuous Deployment: Key Misunderstandings
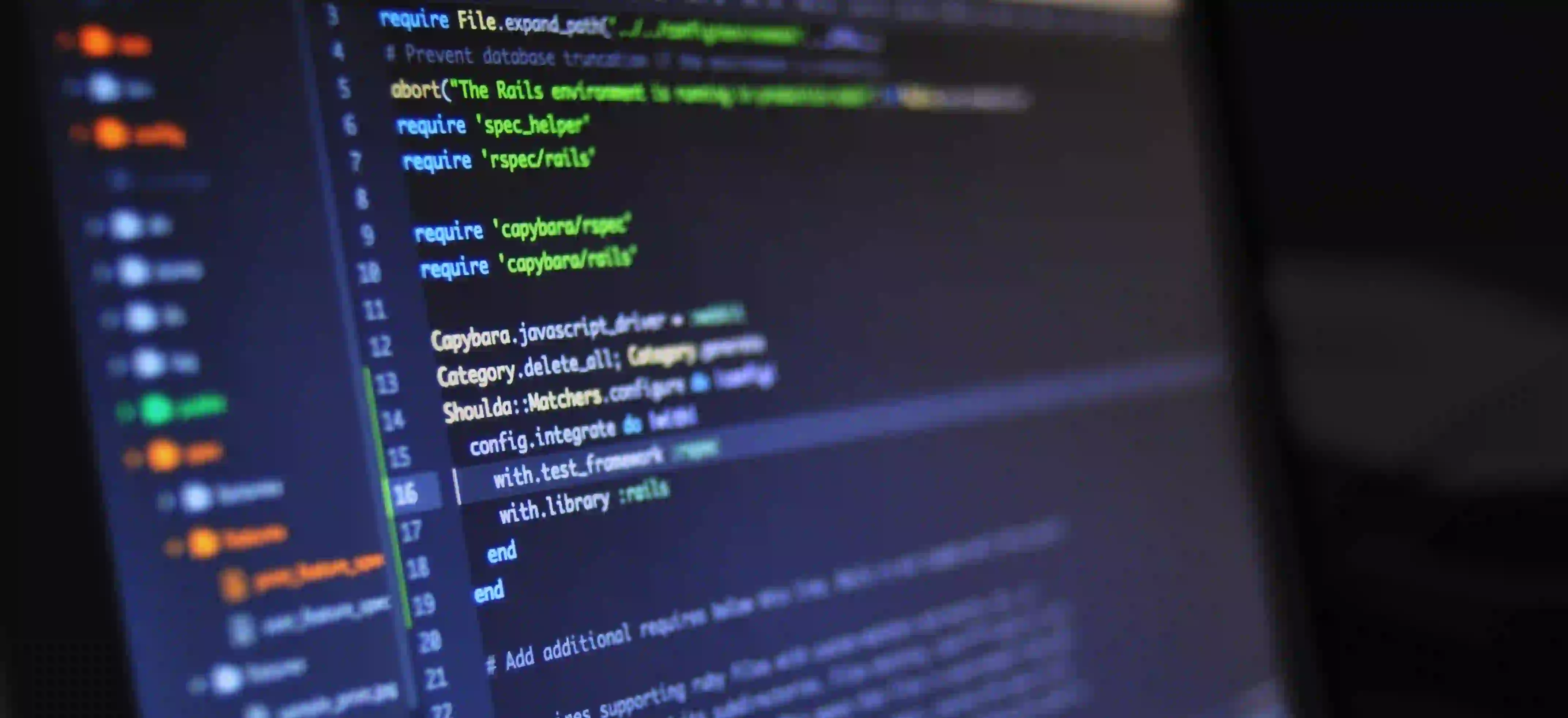
Continuous Delivery vs. Continuous Deployment: Key Misunderstandings
In an era where rapid software development is non-negotiable, both Continuous Delivery (CD) and Continuous Deployment have garnered significant attention. However, many professionals in the industry often confuse these two methodologies. In this blog post, we will explore the distinctions between Continuous Delivery and Continuous Deployment, clarify common misunderstandings, and emphasize their importance in successful software development.
Understanding Continuous Delivery and Continuous Deployment
Before diving into misunderstandings, let's establish what Continuous Delivery and Continuous Deployment mean.
Continuous Delivery (CD)
Continuous Delivery is a software development practice where code changes are automatically prepared for production release. It ensures that software can be reliably released at any time. Key characteristics include:
- Automated Testing: Each change undergoes rigorous automated testing to ensure stability.
- Release Readiness: The code is always in a state that can be deployed to production, often through an automated deployment pipeline.
- Deployment on Demand: Releases are triggered manually by developers or operations teams.
Example of Continuous Delivery:
public class DeploymentPipeline {
public void deploy() {
// Run automated tests
boolean testsPass = runTests();
if (testsPass) {
// Deploy to staging
deployToStaging();
// Await approval for production deployment
notifyTeamForApproval();
} else {
System.out.println("Tests failed. Fix issues before deploying.");
}
}
private boolean runTests() {
// Simulate running tests
return true; // Placeholder for actual test results
}
private void deployToStaging() {
System.out.println("Code deployed to staging environment.");
}
private void notifyTeamForApproval() {
System.out.println("Team notified for production deployment approval.");
}
}
In this example, the code pipelines through automatic testing and waiting for manual intervention before going live. Continuous Delivery creates a safety net, ensuring only tested code reaches users when approved.
Continuous Deployment
Continuous Deployment takes Continuous Delivery to the next step. Every change that passes automated tests is automatically deployed to production. Characteristics include:
- Fully Automated Process: No manual intervention is needed post testing; everything after coding is automated.
- Frequent Releases: Changes are pushed to production frequently, sometimes multiple times a day.
- Immediate Feedback: Bugs are addressed more swiftly as the feedback loop between users and developers is shortened.
Example of Continuous Deployment:
public class AutoDeployment {
public void autoDeploy() {
// Run automated tests
boolean testsPass = runTests();
if (testsPass) {
// Deploy to production
deployToProduction();
} else {
logErrors();
}
}
private boolean runTests() {
// Simulate running tests
return true; // Placeholder for actual test results
}
private void deployToProduction() {
System.out.println("Code automatically deployed to production environment.");
}
private void logErrors() {
System.err.println("Tests failed. Logging errors for further investigation.");
}
}
In this snippet, automatic deployment follows a successful test run without manual checkpoints. Continuous Deployment accelerates the delivery process, enhancing the pace of innovation.
Key Misunderstandings
1. Continuous Delivery Equals Continuous Deployment
One of the most significant misunderstandings is equating Continuous Delivery with Continuous Deployment. Though they share some principles, they are fundamentally different.
- Continuous Delivery involves a manual step before the production release. The team must verify and approve the deployment.
- Continuous Deployment automates the entire deployment process, requiring no additional approvals.
2. Testing is Optional in Continuous Deployment
Another misconception is that testing isn't essential in Continuous Deployment. In fact, robust automation tests are the bedrock of both methodologies.
Importance of Testing:
- Quality Assurance: Automated tests safeguard against bugs and reemphasize code quality.
- Security Risks: Unverified code can expose applications to security vulnerabilities.
3. Continuous Deployment is Suitable for All Projects
While Continuous Deployment can benefit many projects, it isn't universally applicable. Specific scenarios warrant careful consideration of when to deploy:
- Regulatory Compliance: Industries such as finance or healthcare may require stringent compliance checks.
- Potential User Impact: Projects with significant user bases must evaluate the risks of deploying faulty software.
4. Implementing Continuous Deployment is Easy
Many teams believe that adopting Continuous Deployment is a straightforward switch from their current process. However, transitioning involves cultural change and investment in automation.
- Team Buy-in: Continuous deployment requires a shift in mindset and collaboration among all team members.
- Tooling: Investing in continuous integration and deployment (CI/CD) tools (e.g., Jenkins, GitLab CI/CD) plays a crucial role.
5. Continuous Deployment Guarantees Flawless Releases
While Continuous Deployment accelerates the pace of delivery, it doesn't ensure perfection. Here’s why:
- Rollback Mechanisms: Even with automated tests, some bugs may slip through or emerge post-deployment. Robust rollback mechanisms are crucial to revert to stable builds.
- Monitoring and Feedback: Continuous monitoring after deployment offers the ability to understand user behavior and issue emergence.
Best Practices for Implementation
To thrive in Continuous Delivery or Continuous Deployment, consider adopting the following best practices:
1. Create a Robust Testing Suite
All automated tests—unit tests, integration tests, and end-to-end tests—should be implemented. This ensures that every part of your application undergoes rigorous testing. For Java developers, using JUnit or TestNG can help create and manage these tests efficiently.
2. Break Down Monoliths
Strive for microservices if applicable. Smaller and independently deployable services enhance agility and isolate potential issues, leading to more manageable deployments.
3. Embrace a Culture of Collaboration
Foster open communication among developers, operations, and QA teams. A culture of shared responsibility promotes accountability and teamwork, facilitating more effective deployments.
4. Invest in CI/CD Tools
Utilize modern CI/CD tools to create robust pipelines. These tools automate integration and deployment processes, ensuring consistency. Some popular choices include:
- Jenkins: A powerful open-source automation server.
- GitLab CI: Built into GitLab, it streamlines processes from code commit to deployment.
- CircleCI: Offers flexibility in cloud-based CI/CD environments.
5. Monitor and Adapt
Once you've deployed, continuously monitor application performance. Use tools like Prometheus or Grafana for real-time insights and identify areas for improvement.
Wrapping Up
Understanding the distinctions between Continuous Delivery and Continuous Deployment is crucial for implementing these practices effectively in your software development process. While Continuous Delivery emphasizes readiness and control, Continuous Deployment pursues speed and automation.
As methodologies evolve, keeping abreast of these principles will empower teams to release high-quality software efficiently. By addressing key misunderstandings, emphasizing best practices, and proactively engaging with continuous integration tools, developers can navigate the intricacies of modern software development with confidence and precision.
For further reading, consider exploring Continuous Delivery: Reliable Software Releases through Build, Test, and Deployment Automation by Jez Humble and David Farley, which provides deeper insights into these methodologies.
By understanding and correctly implementing these critical concepts, you can enhance your team's performance and ultimately deliver higher quality software to your end users. Happy coding!