Common Pitfalls in XML Validation Against XSDs in Java
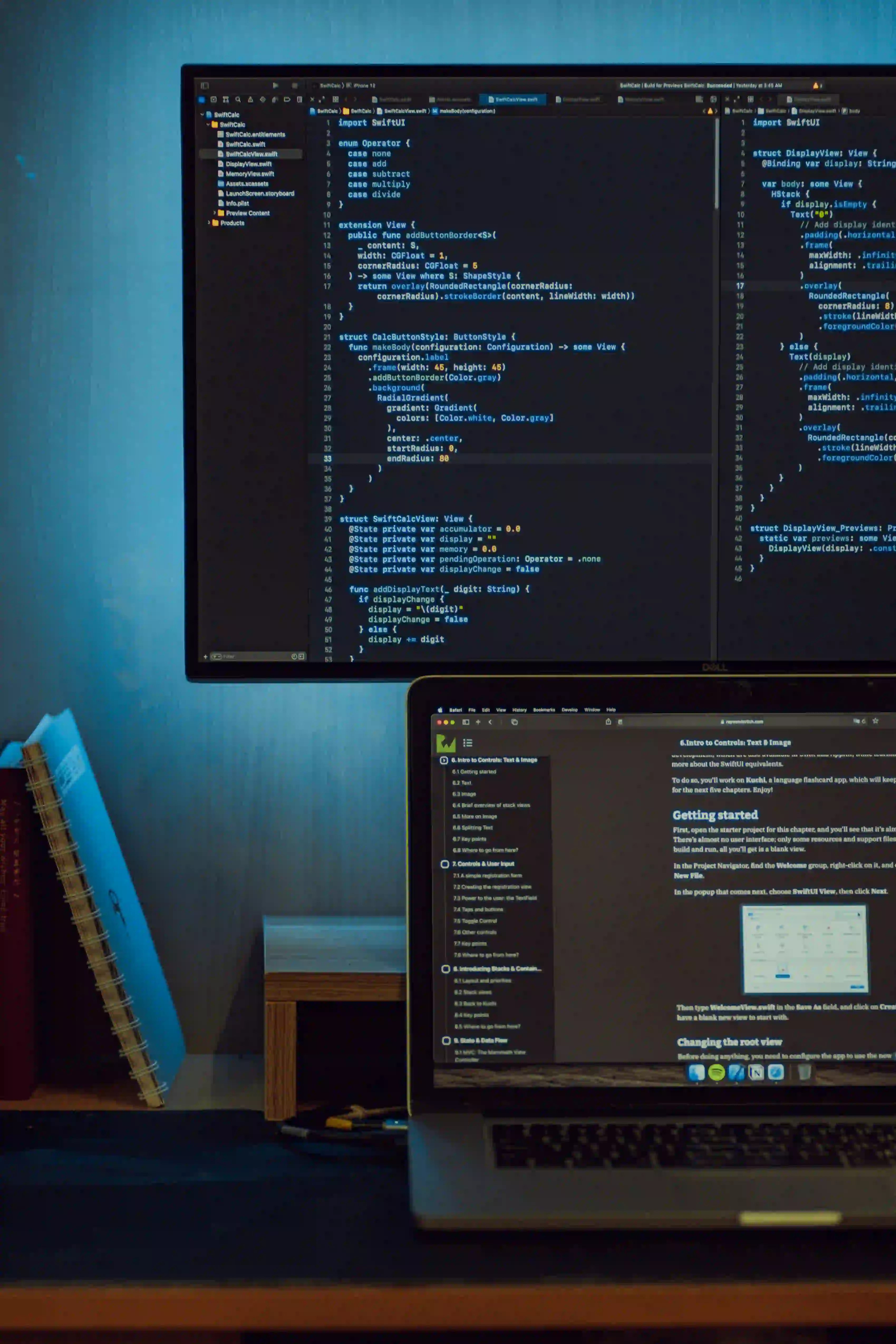
Common Pitfalls in XML Validation Against XSDs in Java
When working with XML in Java, validation against XML Schema Definitions (XSDs) is a critical step to ensure data integrity and compliance with defined formats. However, developers often encounter various pitfalls during this process. This blog post aims to discuss these common pitfalls, provide best practices for overcoming them, and deliver code snippets to illustrate how to effectively handle XML validation.
Understanding XML and XSD
Before diving into the pitfalls, it's essential to clarify the terms.
-
XML (eXtensible Markup Language) is a markup language used to store and transport data. It is designed to be both human-readable and machine-readable.
-
XSD (XML Schema Definition) defines the structure, content, and semantics of XML documents. It acts as a blueprint against which XML files can be validated.
Why XML Validation is Important
Validating XML against XSD helps catch errors early in the development process. It ensures that the XML document adheres to the required structure, data types, and constraints defined in the XSD. This validation can prevent runtime exceptions and data inconsistencies in an application.
Setting Up XML Validation in Java
Java provides built-in support for XML parsing and validation. The javax.xml.validation
package is typically used for this purpose. Here's a basic outline of how you can validate XML against XSD in Java.
import javax.xml.XMLConstants;
import javax.xml.transform.stream.StreamSource;
import javax.xml.validation.Schema;
import javax.xml.validation.SchemaFactory;
import javax.xml.validation.Validator;
import java.io.File;
public class XmlValidator {
public void validate(String xmlFilePath, String xsdFilePath) throws Exception {
SchemaFactory factory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
Schema schema = factory.newSchema(new File(xsdFilePath));
Validator validator = schema.newValidator();
validator.validate(new StreamSource(new File(xmlFilePath)));
}
}
Why This Code Works
- SchemaFactory: This class provides access to a schema factory for the specified namespace.
- Schema: Represents an in-memory representation of an XML schema.
- Validator: An instance created from the Schema object that is responsible for validating XML documents.
Common Pitfalls in XML Validation
Despite how straightforward the above code appears, several common pitfalls can lead to frustration and failed validations. Below, we discuss these pitfalls in detail.
1. Improperly Defined XSD
One of the most common issues is having an XSD that doesn't define an acceptable structure for the corresponding XML.
Solution: Always ensure that the XSD schema accurately represents your XML structure. Use XML editing tools like Oxygen XML Editor to help validate your XSD structure.
Example of an XSD:
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema">
<xs:element name="book">
<xs:complexType>
<xs:sequence>
<xs:element type="xs:string" name="title"/>
<xs:element type="xs:string" name="author"/>
<xs:element type="xs:int" name="year"/>
</xs:sequence>
</xs:complexType>
</xs:element>
</xs:schema>
2. Namespace Mismatches
XML elements can be defined within a specific namespace. Failing to declare namespaces correctly in your XSD could lead to validation errors.
Solution: Always include the correct namespace declarations in both your XML and XSD files.
XML Example with Namespace:
<book xmlns="http://example.com/books">
<title>Java Programming</title>
<author>John Doe</author>
<year>2023</year>
</book>
3. Type Mismatch
Type mismatches between the XML values and their defined types in the XSD can also cause validation failures.
Solution: Ensure that data types in XML follow the types specified in the XSD. For example, if an element is of type xs:int
, the corresponding XML value must be an integer.
4. Schema Compilation and Caching Issues
Sometimes, improper schema compilation or caching can lead to unexpected validation results, especially when you modify your XSD.
Solution: Always clear any schema caches during development in your application if you modify an existing XSD.
5. Ignoring Validation Exceptions
People sometimes catch exceptions but fail to log the details. Ignoring validation exceptions can lead to silent failures.
Solution: Always log or handle exceptions properly to make debugging easier. Modify the previous example to include exception logging.
public void validate(String xmlFilePath, String xsdFilePath) {
try {
// validation code...
} catch (SAXException e) {
System.out.println("Validation failed: " + e.getMessage());
} catch (IOException e) {
System.out.println("IO error: " + e.getMessage());
}
}
6. Not Using a Validator for Each XSD
If your XML file requires multiple XSD validations, sometimes developers would only validate against one. This can lead to incomplete validation.
Solution: Ensure that you validate against each XSD requirement, especially if your XML includes multiple namespaces or types.
Best Practices for XML Validation in Java
-
Use the Latest Libraries: Always utilize the latest versions of Java XML libraries to take advantage of performance improvements and bug fixes.
-
Unit Tests: Implement unit tests for your XML validation to verify that edge cases are handled properly.
-
Use Predefined Schema: Whenever possible, use a predefined schema from trusted sources to reduce the risk of errors.
-
Documentation: Maintain good documentation for the XML structure and its corresponding XSD for easier maintenance and onboarding of new developers.
-
Tooling: Leverage XML validation tools and libraries to assist in the validation process. For instance, consider using libraries like Apache Xerces or javax.xml.
Lessons Learned
XML Validation against XSDs in Java is essential for maintaining data integrity and reliability in applications. Avoiding common pitfalls can significantly enhance the development process. By understanding the nuances of the validation libraries and adhering to best practices, developers can facilitate smoother XML processing.
By being aware of the common pitfalls and employing effective strategies, you can ensure that your XML documents consistently meet the defined specifications, ultimately enhancing the robustness of your Java applications.
For more information on XML handling in Java, you can refer to the official Oracle documentation on Java XML Validation.
By systematically understanding the pitfalls and employing the best practices outlined in this blog, you can prevent typical issues encountered during XML validation in Java while ensuring a seamless experience. Happy coding!