How Overcomplicated Frameworks Slow Down Your Development
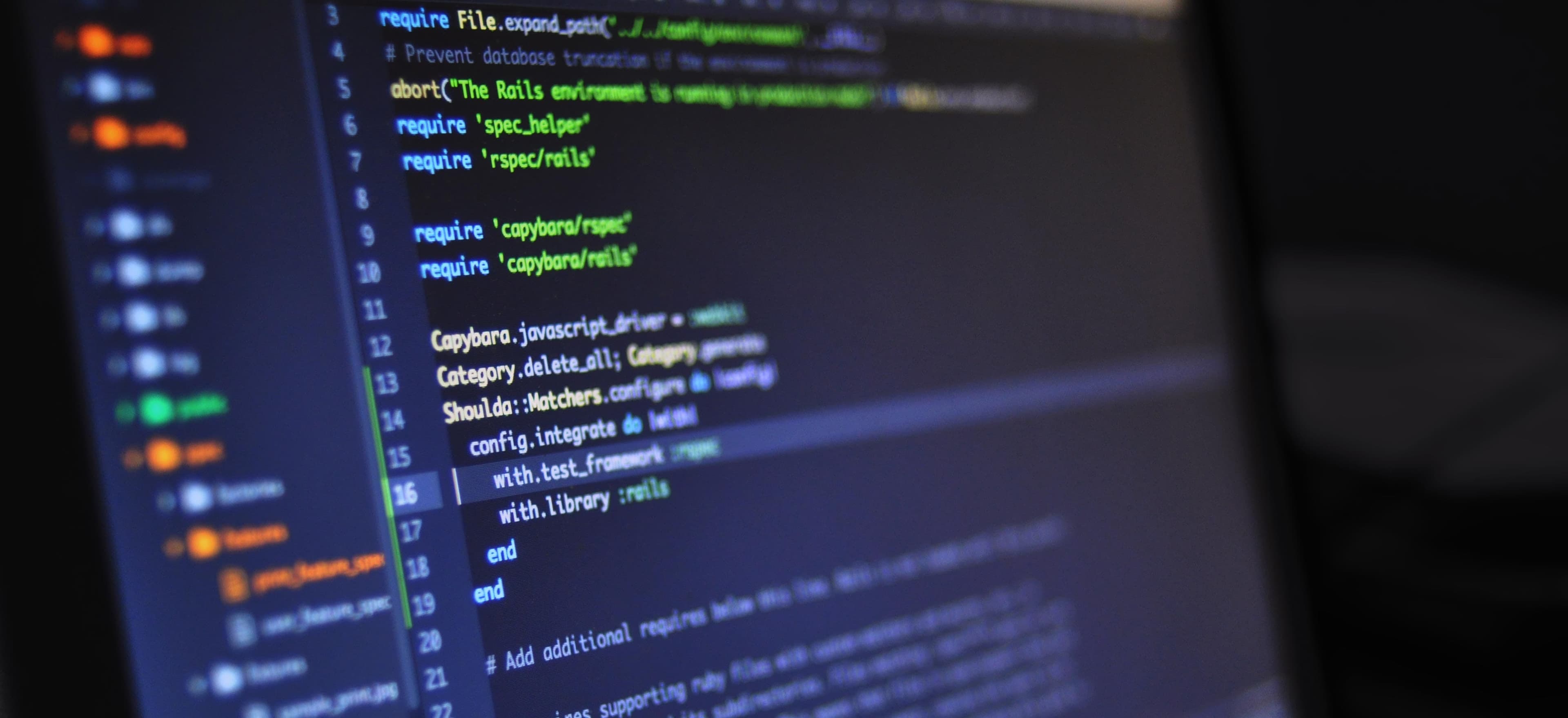
- Published on
How Overcomplicated Frameworks Slow Down Your Development
In the world of software development, choosing the right framework can significantly impact productivity and project outcomes. While frameworks are designed to simplify coding tasks, overcomplicated frameworks can lead to developers experiencing slowdowns, frustration, and ultimately project failures. In this blog post, we will explore how such frameworks inhibit your development process, illustrated with Java code snippets to highlight the concepts discussed.
The Complexity Conundrum
Frameworks are collections of pre-written code that serve as a foundation for your applications, allowing developers to focus on solving the problem rather than reinventing the wheel. However, some frameworks come with a steep learning curve and unnecessarily complex features.
Why Complexity Matters
-
Steep Learning Curves: When developers must invest significant time to understand a complex framework, they can become bogged down before even beginning their actual work.
-
Increased Development Time: Overcomplicated tools often require more lines of code and additional boilerplate code, slowing down the implementation of new features.
-
Hidden Bugs: Complex interactions within an intricate framework can lead to multiple layers of potential bugs, making maintenance more difficult.
Consider the following Java example:
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class Application {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
MyService myService = context.getBean(MyService.class);
myService.performService();
}
}
In this code snippet, we are using Spring Framework - a powerful yet intricate framework. For new developers, understanding the dependency injection in context along with bean management can be daunting.
A Simple Alternative
Now, let’s consider using a simpler approach, perhaps without an extensive framework to understand how frameworks can complicate simple tasks:
public class MyService {
public void performService() {
System.out.println("Service is performed without complex framework!");
}
public static void main(String[] args) {
MyService myService = new MyService();
myService.performService();
}
}
This example demonstrates a straightforward service invocation without the layer of complexity introduced by the framework. Here, there are no dependencies to manage, and new developers can quickly grasp the code's intent.
Performance Implications
Framework complexity can also impact performance. Large frameworks often come with additional features, libraries, and dependencies which increase load times and reduce application responsiveness. The more extensive the framework, the greater the potential lag in performance.
Real-world Scenarios
In a larger project, using a heavy framework for a simple CRUD application can lead to unnecessary complications. For instance, implementing a lightweight servlet for such use-cases would be more efficient than engaging a full-stack framework that requires extensive configuration.
import javax.servlet.http.*;
import javax.servlet.*;
import java.io.*;
public class SimpleServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<h1>Hello, World!</h1>");
}
}
This simple servlet can be deployed with minimal configuration compared to a complex framework that requires setup and environment configuration. Your development team can build and deploy swiftly without abundant abstractions.
Maintainability and Scalability
Overcomplicated frameworks can create a significant barrier during the maintenance phase of development. If your team is not well-versed in the framework, it complicates updating, debugging, or adding new features.
Fragmented Knowledge
In teams where multiple developers interact with a complex framework, knowledge fragmentation can occur. Each developer might understand different parts, leading to inconsistences and excess lead time to share knowledge.
Simplifying Maintenance
One way to overcome these hurdles is by implementing coding standards and documentation to help developers who are less familiar with a given framework. Alternatively, you can opt for simpler frameworks or libraries designed with clarity in mind.
Consider using simpler libraries that focus solely on specific tasks. For instance, libraries like Hibernate can simplify data access without the necessity for a full-stack framework.
import org.hibernate.Session;
import org.hibernate.SessionFactory;
public class HibernateExample {
public void saveUser(User user) {
SessionFactory sessionFactory = HibernateUtil.getSessionFactory();
Session session = sessionFactory.openSession();
session.beginTransaction();
session.save(user);
session.getTransaction().commit();
session.close();
}
}
Here, Hibernate allows you to focus just on the data persistence aspect, without getting overwhelmed by unrelated concerns.
In Conclusion, Here is What Matters
Overcomplicated frameworks can slow down development by increasing learning curves, reducing performance, complicating maintainability, and creating communication barriers. While frameworks are built to facilitate the development process, it’s vital to find the balance between functionality and simplicity.
When embarking on a new project, consider the scope of your application, the experience level of your team, and your long-term maintenance strategy. Sometimes, the best framework is the most straightforward one — where you can get your project up and running without excessive complexity.
If you want to explore more about selecting the right frameworks, check out Choosing the Right Framework for Your Project for guidance. An informed decision can help keep your development process efficient and effective.
For a deeper understanding of how simpler methodologies can save time, explore the Agile Software Development Practices that emphasize not only continuous learning and adaptability but also simplicity and efficiency in the codebase.
By prioritizing simplicity in your development process, you can ensure your team remains productive, your code remains clean, and your projects are successful.