Understanding Spring Dependency Injection: Common Pitfalls
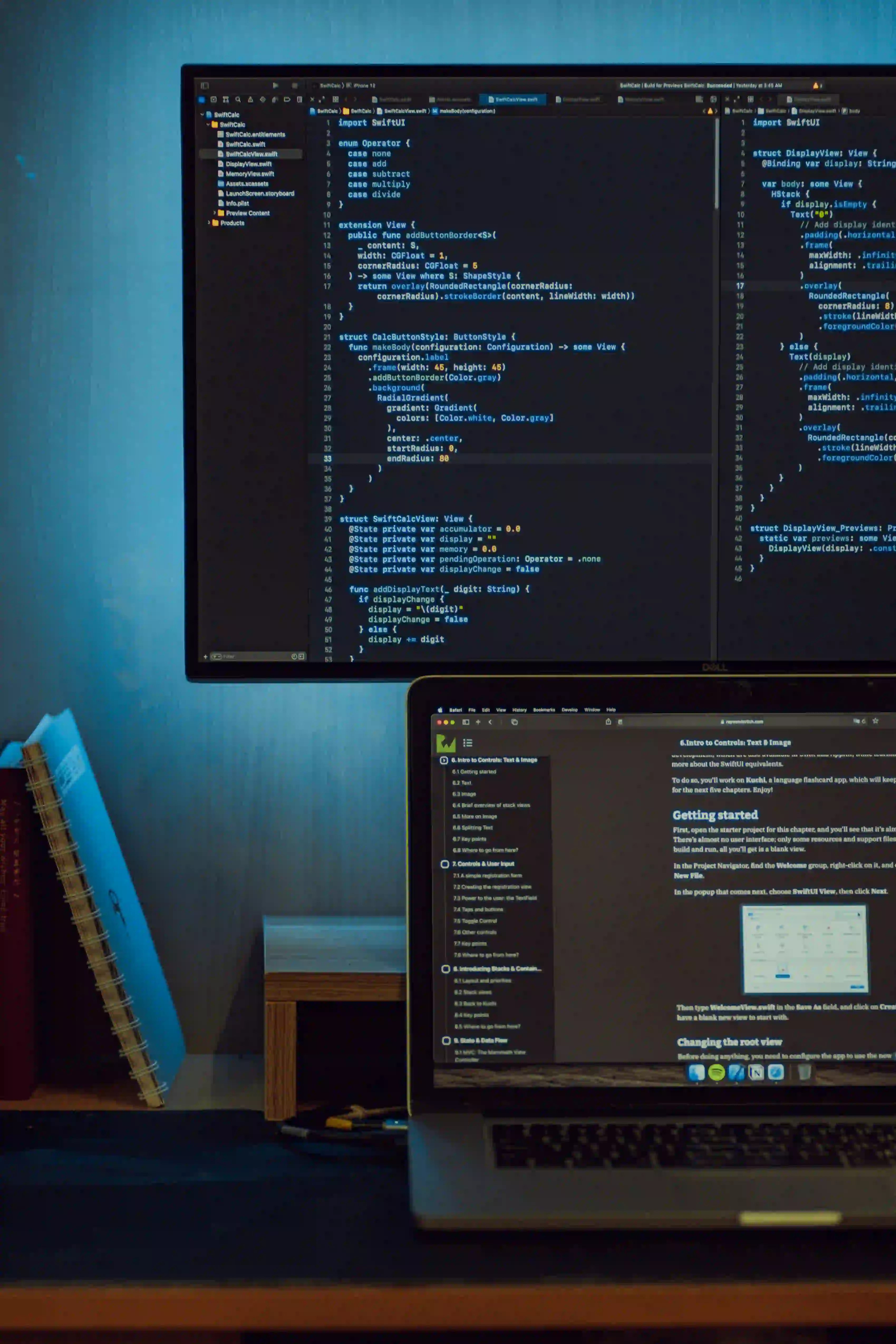
Understanding Spring Dependency Injection: Common Pitfalls
Spring Framework has revolutionized the way Java applications are constructed, particularly through its powerful Dependency Injection (DI) capabilities. DI simplifies complex relationships, promotes loose coupling, and enhances testability. Nevertheless, like any powerful tool, it comes with its own set of challenges. In this blog post, we’ll explore some common pitfalls developers encounter when working with Spring’s Dependency Injection and provide strategies to overcome them.
What is Dependency Injection?
Before diving into the pitfalls, let’s briefly explain what Dependency Injection is. At its core, DI is a design pattern used to implement IoC (Inversion of Control), allowing the creation of objects to be delegated to an external entity—typically a container like the Spring Framework.
Essentially, a class will not instantiate its dependencies directly but will receive them from a constructor, setter method, or a field, thus decoupling the class from the actual instantiation logic.
Example of Dependency Injection
Here’s a simple example to illustrate the concept:
public class UserService {
private UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserById(Long id) {
return userRepository.findById(id);
}
}
In this case, UserService
depends on UserRepository
. Instead of creating an instance of UserRepository
directly inside UserService
, it receives it through its constructor—this is Dependency Injection.
Common Pitfalls of Spring Dependency Injection
1. Misconfiguring Bean Scopes
Spring provides several scopes for your beans: singleton, prototype, request, session, and application. Developers often forget to specify the appropriate scope, which can lead to unintended consequences.
Example of Scope Misconfiguration
@Component
@Scope("prototype") // Correct usage for prototype scope
public class UserService {
// ...
}
Why This Matters
Using singleton
when prototype
is needed can create shared state between requests, leading to potentially dangerous side effects in a web application. Always double-check which scope best fits your bean's lifecycle.
2. Circular Dependencies
Circular dependencies occur when two beans depend on each other. For example, if ClassA
requires ClassB
and vice versa, you have a circular dependency that can confuse the Spring container.
Example of Circular Dependency
@Component
public class ClassA {
private ClassB classB;
@Autowired
public ClassA(ClassB classB) {
this.classB = classB;
}
}
@Component
public class ClassB {
private ClassA classA;
@Autowired
public ClassB(ClassA classA) {
this.classA = classA;
}
}
Why This Matters
Spring cannot resolve the dependencies here by default and will throw an exception during initialization. One way to address this is through setter injection:
@Component
public class ClassA {
private ClassB classB;
@Autowired
public void setClassB(ClassB classB) {
this.classB = classB;
}
}
@Component
public class ClassB {
private ClassA classA;
@Autowired
public void setClassA(ClassA classA) {
this.classA = classA;
}
}
3. Overusing @Autowired
While @Autowired
is a powerful annotation for automatically injecting dependencies, using it excessively can clutter your code and obscure the true dependencies of a class.
Why Dependency Injection Matters
Example of Overusing @Autowired
@Component
public class ExampleService {
@Autowired private UserService userService;
@Autowired private OrderService orderService;
@Autowired private NotificationService notificationService;
// and many more...
}
The Cost
Having too many @Autowired
fields makes it harder to comprehend a class's actual dependencies. Instead, aim for constructor injection, as seen before, which leads to cleaner APIs and encourages immutability.
4. Ignoring Qualifiers
When multiple beans of the same type exist, Spring may struggle to determine which to inject. Utilizing @Qualifier
ensures the right bean is injected.
Example of Ignoring Qualifiers
@Component
public class UserService {
@Autowired
private UserRepository userRepository; // Ambiguous if multiple implementations exist
}
The Benefit of Using Qualifiers
Here’s how you can clarify:
@Autowired
@Qualifier("mongoUserRepository")
private UserRepository userRepository; // Now clearly specifies which implementation to use
5. Not Leveraging Profiles
When developing applications with multiple environments (development, testing, production), using Spring profiles can easily configure beans differently based on the active profile.
Example Without Profiles
@Component
public class DataSourceConfig {
// Configuration for H2 database for dev
}
How to Utilize Profiles
@Profile("dev")
@Bean
public DataSource devDataSource() {
// Dev-specific DataSource
}
@Profile("prod")
@Bean
public DataSource prodDataSource() {
// Prod-specific DataSource
}
6. Poorly Defined Component Scanning
Spring automatically detects and registers beans through component scanning. However, if your configuration doesn't include the necessary packages, you might end up with unregistered beans.
A Common Misstep
@Configuration
@ComponentScan(basePackages = "com.example") // Missing child package: com.example.services
public class AppConfig {
}
Correcting the Course
Ensure all relevant packages are clearly listed in the basePackages
attribute or use basePackageClasses
to reference specific classes.
The Last Word
Understanding the intricacies of Spring Dependency Injection can significantly enhance your Java applications. By being aware of common pitfalls—such as misconfigured scopes, circular dependencies, overusing @Autowired
, incorrectly applying qualifiers, not utilizing profiles, and defining poor component scanning—you can avoid numerous headaches.
Resources for Further Reading
Invest time in mastering these principles to optimize your skills and elevate your application development process. Happy coding!