Understanding AssertEquals vs. AssertSame in JUnit Testing
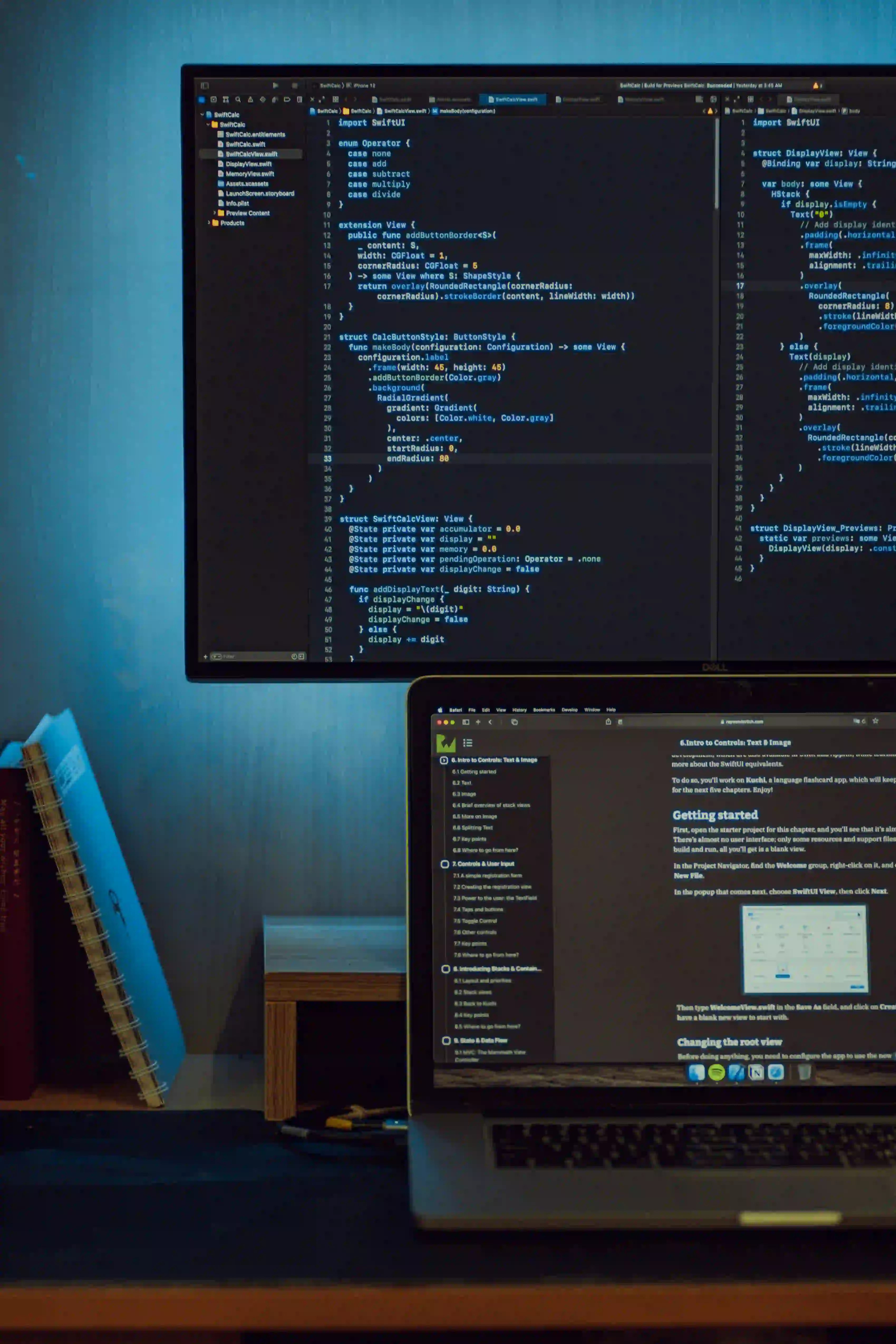
Understanding AssertEquals vs. AssertSame in JUnit Testing
When writing unit tests in Java, understanding how to effectively compare values is crucial for validating your code's correctness. Two essential methods provided by the JUnit framework for this purpose include assertEquals
and assertSame
. Despite what might seem like similar functionality at first glance, these methods serve distinct purposes. This blog post will help clarify these differences, provide code examples, and ultimately guide you in choosing the right method in various scenarios.
Table of Contents
- What is JUnit?
- Overview of assertEquals
- Overview of assertSame
- Key Differences Between assertEquals and assertSame
- When to Use Each
- Code Examples
- Conclusion
What is JUnit?
JUnit is a widely used testing framework in the Java ecosystem. It allows developers to write test cases to validate the behavior of their code. JUnit provides a rich set of assertions which you can use to verify whether your code is behaving as expected. It supports both unit and integration testing, making it an essential tool for any Java developer.
Overview of assertEquals
assertEquals
is used to check whether two values are equal. The method compares the actual results produced by your code with the expected results. The signature for assertEquals
is as follows:
void assertEquals(Object expected, Object actual)
- Parameters:
expected
: This is the value that you expect from the method you're testing.actual
: This is the value returned by the method under test.
Why Use assertEquals?
- Value Comparison: You want to check if two values are equivalent in a logical sense.
- Flexibility: It can handle primitive types and objects, calling the
equals()
method to check for equality of object instances.
Overview of assertSame
assertSame
, on the other hand, is used to check whether two references point to the same object. This means that it verifies both reference equality and identity. Here's how to use assertSame
:
void assertSame(Object expected, Object actual)
- Parameters:
expected
: This is the expected reference.actual
: This is the actual reference pulled from the method you're testing.
Why Use assertSame?
- Reference Check: You want to determine if two object references are identical.
- Memory Management: Useful when checking singleton classes or shared instances.
Key Differences Between assertEquals and assertSame
| Feature | assertEquals | assertSame |
|----------------|----------------------------------|-----------------------------------|
| Comparison Type| Value-based (logical equality) | Reference-based (identity) |
| Method Used | Uses equals()
method | Uses ==
operator |
| Applicability | Broad (primitives, objects) | Narrow (object references only) |
| Failure Message | Detailed, relevant to values | Directly identifies difference in references |
When to Use Each
-
Use
assertEquals
when:- You want to verify values, such as numbers, strings, or even custom object properties, where two separate instances can logically be considered equal.
Example:
☕snippet.javaassertEquals(5, calculateSum(2, 3)); // Asserts that the sum is 5
-
Use
assertSame
when:- You need to ensure that two references point to the exact same instance in memory, especially in singleton patterns.
Example:
☕snippet.javaMySingleton instance1 = MySingleton.getInstance(); MySingleton instance2 = MySingleton.getInstance(); assertSame(instance1, instance2); // Asserts that both references point to the same object.
Code Examples
Let’s dive deeper with some practical examples.
Example Using assertEquals
Imagine you have a Calculator
class that has a multiply
method:
public class Calculator {
public int multiply(int a, int b) {
return a * b;
}
}
Here’s a test for the multiply
method using assertEquals
:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testMultiply() {
Calculator calculator = new Calculator();
int expected = 12;
int actual = calculator.multiply(3, 4);
assertEquals(expected, actual); // Checks for value equality
}
}
In this example, even if multiply
returns a new Integer
object each time, the value check is what matters.
Example Using assertSame
Now consider a scenario with a singleton pattern:
public class DatabaseConnection {
private static DatabaseConnection instance = null;
private DatabaseConnection() {
// Private constructor
}
public static DatabaseConnection getInstance() {
if (instance == null) {
instance = new DatabaseConnection();
}
return instance;
}
}
Here’s a test using assertSame
:
import static org.junit.Assert.assertSame;
import org.junit.Test;
public class DatabaseConnectionTest {
@Test
public void testSingleton() {
DatabaseConnection conn1 = DatabaseConnection.getInstance();
DatabaseConnection conn2 = DatabaseConnection.getInstance();
assertSame(conn1, conn2); // Checks that both references point to the same instance
}
}
In this case, assertSame
is vital to ensure that our singleton design properly returns the same instance across various requests.
The Closing Argument
Understanding when to use assertEquals
and assertSame
is essential for effective testing in Java. While assertEquals
is great for value comparisons, assertSame
focuses on reference comparisons. Selecting the appropriate assertion method ensures your tests are precise and reliable.
Whether you are performing unit tests or integration tests, employing these assertions wisely will enhance your testing suite's robustness, helping you deliver higher-quality software.
For additional reading on JUnit and its features, check JUnit's official documentation for more tips and techniques.
By following these guidelines and examples, you should now have a clearer understanding of how to use assertEquals
and assertSame
effectively in JUnit testing. Happy coding!