Troubleshooting Compact Number Formatting in Applications
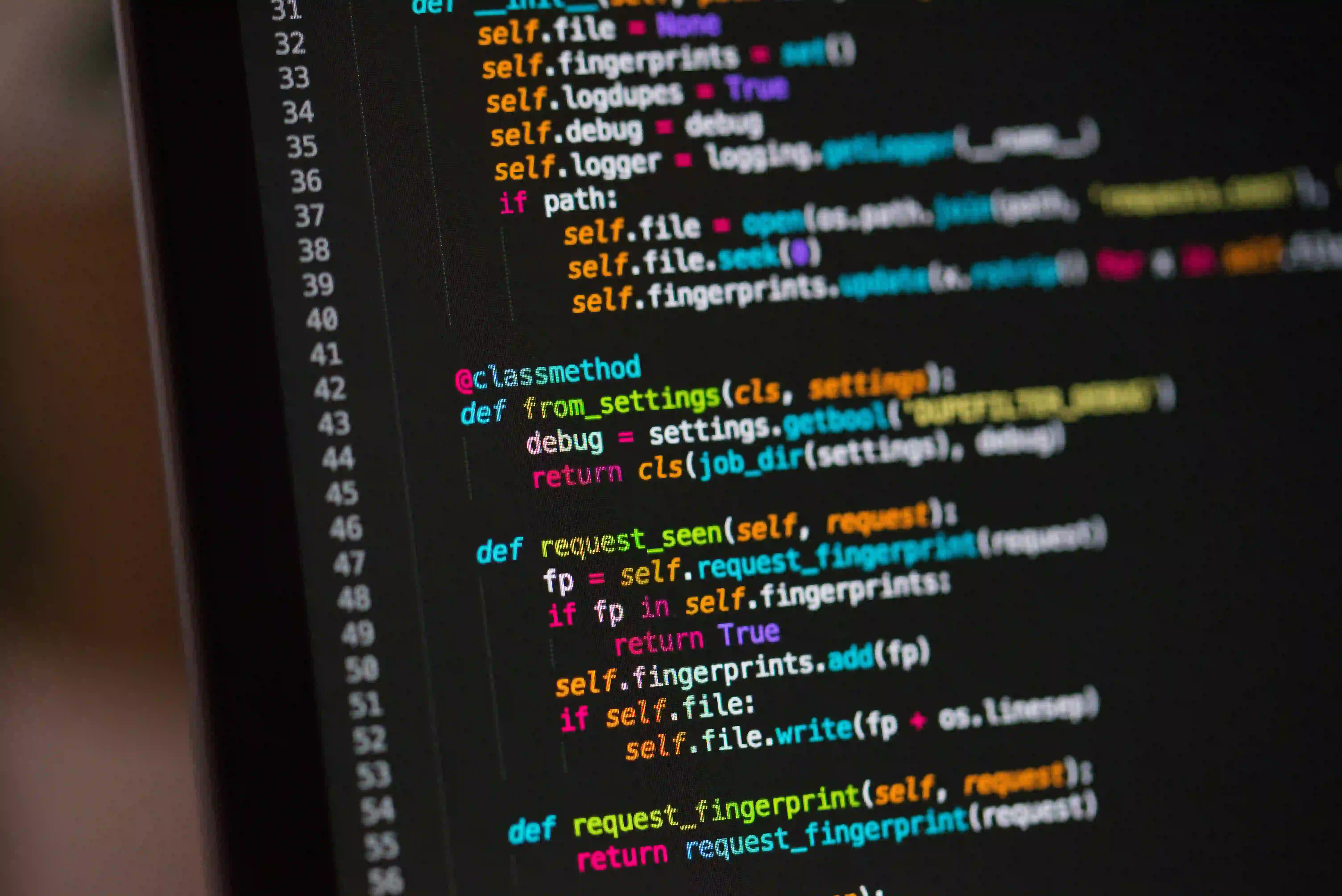
Troubleshooting Compact Number Formatting in Applications
In today's data-driven world, presenting numerical information effectively can significantly impact the end-user experience. One powerful way to enhance the readability of numerical figures is through compact number formatting. While it simplifies the representation of large numbers, implementing it can come with its share of challenges. This post will guide you through troubleshooting compact number formatting issues in your applications using Java.
What is Compact Number Formatting?
Compact number formatting allows developers to display numbers in a more legible way, using abbreviated forms like "K" for thousands, "M" for millions, and "B" for billions. This approach not only saves space but also makes it easier for readers to grasp large figures quickly.
Java's Built-in Support for Compact Number Formatting
Java provides a built-in way to format numbers compactly through the java.text.NumberFormat
class, introduced in Java 8. The new getCompactNumberInstance()
method allows developers to create instances that can automatically abbreviate large numbers.
import java.text.NumberFormat;
import java.util.Locale;
public class CompactNumberExample {
public static void main(String[] args) {
long amount = 12345678; // Example large number
NumberFormat compactFormat = NumberFormat.getCompactNumberInstance(Locale.US, NumberFormat.Style.SHORT);
String compactNumber = compactFormat.format(amount); // Returns "12M"
System.out.println("Compact number: " + compactNumber);
}
}
In this example, we utilize the getCompactNumberInstance()
method to convert a large number into a more digestible format. The Locale
parameter ensures that formatting is consistent with regional standards.
Common Troubleshooting Scenarios
-
Unexpected Output
Sometimes, you may encounter unexpected results when using compact number formatting. This can typically happen due to an incorrect locale or style. Ensure that you are using the appropriate combination of these parameters.
Solution: Verify locale and style settings. The style can be set to either
SHORT
orLONG
, dictating how abbreviations are presented.☕snippet.javaNumberFormat compactFormatLong = NumberFormat.getCompactNumberInstance(Locale.US, NumberFormat.Style.LONG); // For example, 12345678 will be displayed as "12 million"
-
Locale Issues
Compact number formatting is heavily dependent on the locale you set. If you're seeing unexpected abbreviations or symbols, it's time to review your locale specifications.
Solution: Make sure you are using the correct
Locale
. For example, usingLocale.GERMANY
can yield different results compared toLocale.US
.☕snippet.javaNumberFormat germanCompactFormat = NumberFormat.getCompactNumberInstance(Locale.GERMANY, NumberFormat.Style.SHORT); String germanFormatted = germanCompactFormat.format(123456); // Expected output would be "123,4 Tsd" instead of "123K"
-
Inferring Incorrect Values
Careful consideration must be given when formatting values for display versus the actual values used in your calculations. If your application pulls data from an API or a database, ensure that you're not inadvertently truncating or rounding the values before formatting.
Solution: Always validate the data retrieved from external sources before formatting. Utilize debugging tools to log the numbers prior to formatting.
☕snippet.java// Suppose we retrieve this value from a database long retrievedValue = getValueFromDatabase(); // function that fetches value System.out.println("Retrieved Value: " + retrievedValue); // Log value before formatting
-
Integration with User Interface (UI)
Ensuring that compact numbers display correctly in the UI can often lead to formatting and alignment issues, especially in JavaFX or Swing applications.
Solution: Pay attention to UI components and their properties. Ensure proper padding is applied to maintain alignment.
☕snippet.javaJLabel compactNumberLabel = new JLabel(compactFormat.format(amount)); compactNumberLabel.setHorizontalAlignment(SwingConstants.RIGHT); // Align to the right
-
Performance Implications
Applying compact number formatting repeatedly in a high-traffic application can lead to performance lag.
Solution: Cache formatted values if they are static or do not change often. This reduces the overhead of repeated formatting.
☕snippet.javaMap<Long, String> cache = new HashMap<>(); long amountToFormat = 987654321; cache.putIfAbsent(amountToFormat, compactFormat.format(amountToFormat)); // Only calculates if not previously cached
Best Practices for Implementing Compact Number Formatting
-
Choose the Right Locale: Always set the locale appropriately based on your user demographic. This ensures they see numbers they are familiar with.
-
Validate Input Values: Before formatting, validate all inputs to ensure you get reliable outputs.
-
Consider UI Alignment: As mentioned above, maintain spaces or padding in UI so that compact numbers don’t disrupt visual flow.
-
Profile Performance: When developing high-load applications, profile the performance of number formatting. Avoid redundant calculations by caching results when possible.
-
Testing: Unit test your formatting logic, covering different locales and corner cases.
Additional References
To gain a deeper understanding of number formatting in Java, consider exploring the following resources:
- Java - Official Documentation on NumberFormat
- Localization and Internationalization in Java
- Java. Templating and Formatting Guide
The Bottom Line
Troubleshooting compact number formatting in Java applications can seem daunting, but understanding the common issues and adopting best practices makes the process much more manageable. By ensuring correct locale usage, validating input values, optimizing for UI alignment and performance, you can create applications that not only present data clearly but also enhance the user experience.
With practice and careful attention to detail, you can master compact number formatting and elevate your applications' usability. Happy coding!