Common Pitfalls in Architecting Data-Intensive Apps
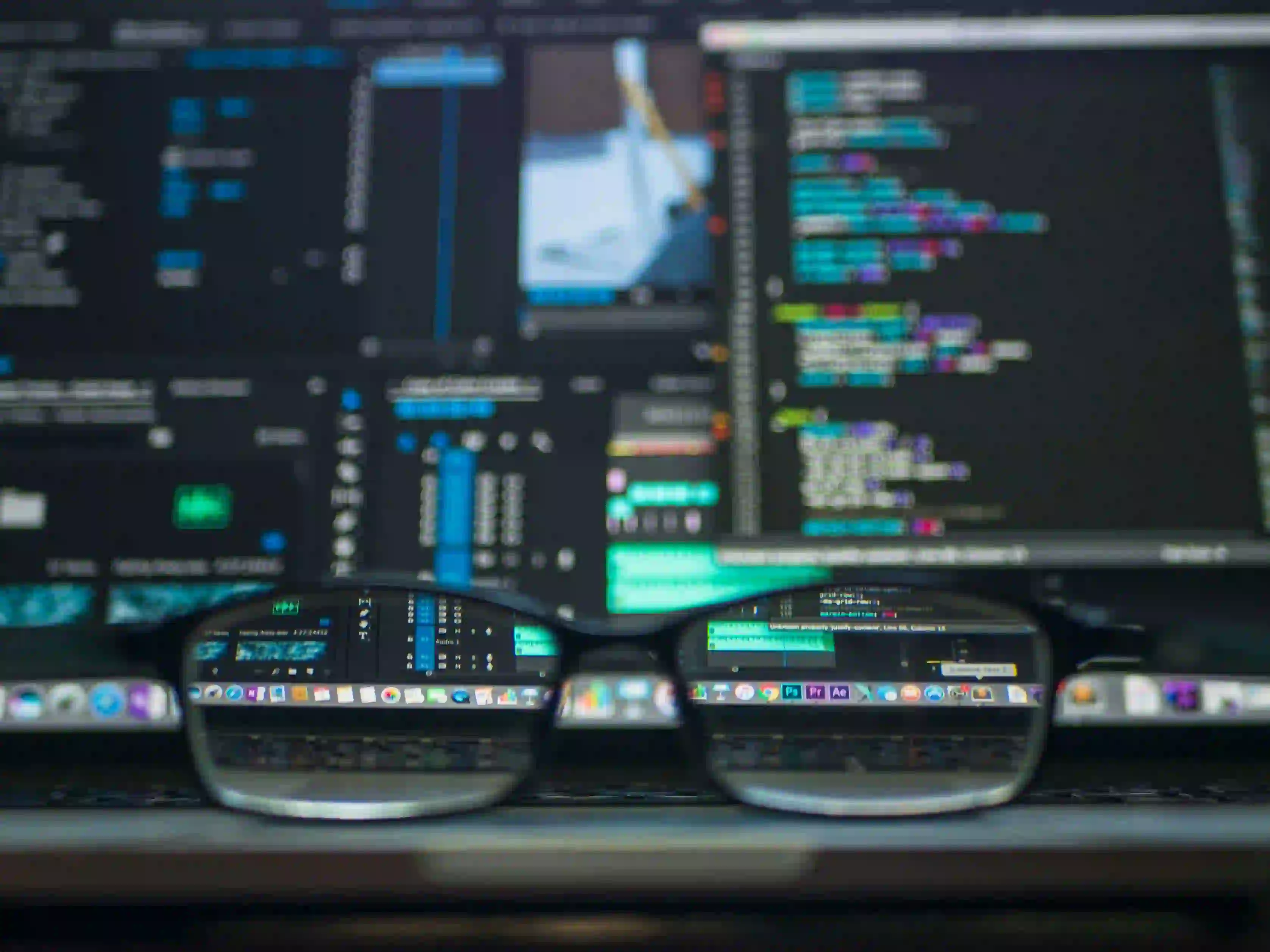
Common Pitfalls in Architecting Data-Intensive Apps
In today's world, data is king. Organizations are generating and processing vast amounts of data, necessitating effective solutions to build robust data-intensive applications. However, architects and developers often stumble into several common pitfalls. Understanding these pitfalls is crucial to developing applications that are not only functional but also scalable, maintainable, and efficient.
1. Underestimating Data Complexity
Why It Matters
Data isn’t always straightforward. It can be semi-structured, unstructured, or even hierarchical. Underestimating the complexity of data types can lead to significant architectural challenges down the line.
Key Takeaway
Be diligent in analyzing the data types your application will handle. Use tools like entity-relationship diagrams (ERDs) to map out data relationships in the early stages of development.
Example
Suppose you are building an e-commerce application. You might think of product data as simple key-value pairs:
class Product {
private String id;
private String name;
private double price;
// Getters and Setters
}
But when you consider multiple data types, such as product reviews, tags, or even images, you will find that you need a more sophisticated design.
class Product {
private String id;
private String name;
private double price;
private List<Review> reviews; // Each product can have multiple reviews
private List<String> tags; // Tags for search optimization
private String imageUrl; // Storing image reference
// Getters and Setters
}
class Review {
private String userId;
private String content;
private int rating;
// Getters and Setters
}
2. Ignoring Scalability
Why It Matters
Scalability is a cornerstone of application architecture, especially for data-intensive apps that may see rapid growth. If you design your application to handle a limited dataset, you will encounter bottlenecks as your user base grows.
Key Takeaway
Consider adopting a microservices architecture to improve scalability. This way, different services can be scaled independently based on their load.
Example
If you're using a monolithic architecture, a failure in one module could compromise the whole application. In contrast, a microservices setup allows for modular growth:
@RestController
@RequestMapping("/products")
public class ProductService {
@GetMapping("/{id}")
public ResponseEntity<Product> getProduct(@PathVariable String id) {
// Logic to retrieve product details
}
}
You can scale the product service independently if it begins to take on more traffic without affecting other services.
3. Neglecting Data Governance
Why It Matters
As data volumes grow, so does the need for governance. Neglecting data governance can lead to data breaches, compliance issues, and poor data quality.
Key Takeaway
Establish a data governance framework early in your development cycle. Assign roles, responsibilities, and automated processes for data management.
Example
Adopting a Role-Based Access Control (RBAC) system ensures that only authorized users can access sensitive data:
enum Role {
ADMIN, USER, GUEST
}
class User {
private String username;
private Role role;
public boolean hasAccess(Resource resource) {
// Logic to check user role against the resource permissions
}
// Getters and Setters
}
4. Overcomplicating Technology Choices
Why It Matters
Choosing technologies for the sake of novelty rather than necessity can lead to an unnecessarily complicated system. Complex architectures can confuse teams and make maintenance a Nightmare.
Key Takeaway
Stick with established patterns and frameworks that your team is already familiar with. Opt for simpler solutions that meet your requirements without adding layers of complexity.
Example
Instead of opting for a sophisticated distributed database for a project that doesn’t require that level of complexity...
CREATE TABLE users (
id SERIAL PRIMARY KEY,
username VARCHAR(100),
email VARCHAR(255) UNIQUE NOT NULL
);
...consider starting with a traditional RDBMS like PostgreSQL or MySQL, which can handle a variety of workloads without the additional overhead.
5. Ignoring Performance Optimization
Why It Matters
Performance gaps can lead to poor user experiences and increased operational costs. What might seem fast with minimal data may become sluggish as load increases.
Key Takeaway
Build performance tests into your development pipeline. Evaluate objectives for queries and optimize where necessary.
Example
Instead of loading all user data at once, consider using pagination to improve response times:
public List<User> getUsers(int pageNumber, int pageSize) {
Pageable pageable = PageRequest.of(pageNumber, pageSize);
return userRepository.findAll(pageable).getContent();
}
By only retrieving the data you need at any moment, you can minimize load times and system strain.
6. Not Planning for Failure
Why It Matters
No application is immune to failure. Failing to plan for outages, unavailability, or data loss can lead to catastrophic consequences.
Key Takeaway
Implement strategies for fault tolerance, redundancy, and disaster recovery.
Example
Using backup systems or replicas allows your application to recover quickly in the event of a failure. For instance, consider using data replication in your database:
CREATE SERVER my_replica FOREIGN DATA WRAPPER postgres_fdw
OPTIONS (host 'my_database_host', dbname 'my_database', port '5432');
This way, even if your primary database goes down, you have another reliable source.
My Closing Thoughts on the Matter
Architecting data-intensive applications presents a range of challenges. By avoiding these common pitfalls—underestimating complexity, ignoring scalability, neglecting data governance, overcomplicating technology choices, overlooking performance, and failing to plan for failure—you position your development process for success.
Each step in addressing these challenges can lead to more resilient, scalable, and maintainable applications that can adapt to changing data landscapes. Keeping abreast of best practices will help you design data architecture that not only functions efficiently but also scales as your organization grows.
For more information on architecting effective data solutions, consider exploring resources like Martin Fowler's Blog on Data Gardening or Building Microservices by Sam Newman.
With foresight and planning, you can mitigate risks and create a solid foundation for your data-intensive applications.