How to Fix Common Issues with Remember Me in Spring Security
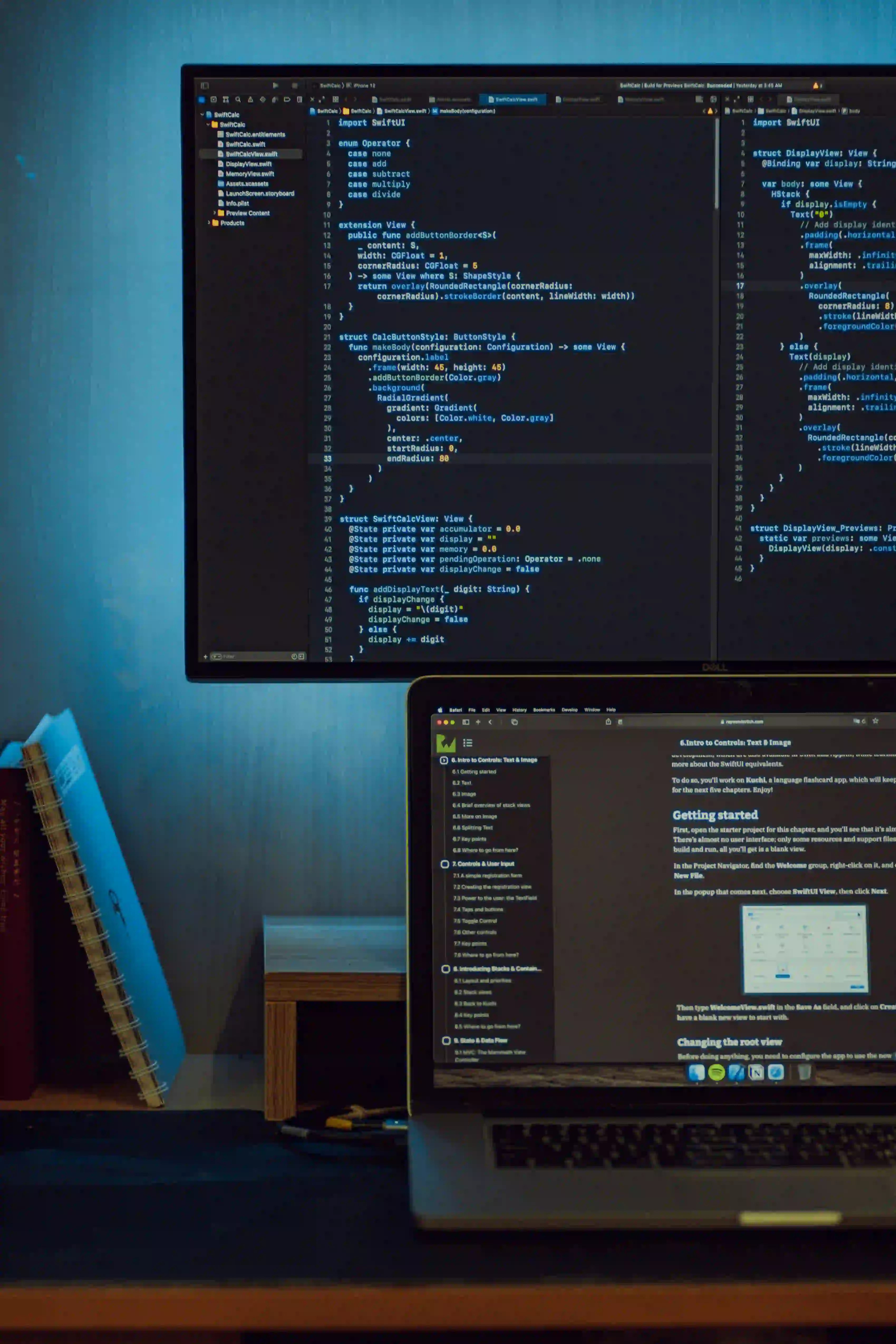
How to Fix Common Issues with Remember Me in Spring Security
Spring Security is a powerful framework that provides comprehensive security services for Java applications. One of its common features is the "Remember Me" functionality, which enhances user experience by allowing users to stay logged in even after closing the browser window. However, developers often run into issues while implementing this feature. In this blog post, we will delve into the common issues related to the "Remember Me" functionality in Spring Security, discuss their causes, and provide solutions.
Understanding the Remember Me Functionality
The "Remember Me" feature in Spring Security essentially uses a cookie stored in the user's browser to automatically authenticate them after their session expires. It's critical for improving user experience, especially for applications where frequent logins can be cumbersome.
Example Configuration
Here’s a basic snippet that shows how to enable the "Remember Me" functionality in Spring Security:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.permitAll()
.and()
.rememberMe()
.key("uniqueAndSecret") // A unique key for remember-me functionality
.tokenValiditySeconds(86400); // Cookie validity time
}
}
Why This Code?
In this snippet, the rememberMe()
method is configured with a unique key and the cookie validity period is set to 86400 seconds (24 hours). The tokenValiditySeconds
property is crucial as it defines how long the user should remain logged in.
Common Issues with Remember Me
1. Remember Me Not Working
Problem: Sometimes, despite implementing the remember function, users are prompted to log in again after closing and reopening their browser.
Cause: This often occurs due to insufficient or incorrect configurations, or cookie policies that prevent cookies from being stored.
Solution: Make sure you have correctly configured your cookie properties and ensured that cookies are not being blocked. Additionally, check the key; using a hardcoded string for "remember-me" is not secure. Store your key securely and ensure it is consistent across different environments.
2. Invalid Cookie Handling
Problem: Users receive an error indicating that their remember me cookie is invalid.
Cause: This can happen if the token associated with the remember me cookie is expired, tampered with, or the key used to generate it has changed.
Solution: If you're changing the key (e.g. during environment changes such as moving from development to production), old tokens which were generated with the previous key will become invalid. To mitigate this, you can set up a plan to rotate keys safely without breaking existing sessions or implement a mechanism to log users out of their sessions when keys change.
3. Cookie Not Being Set
Problem: When users log in, the cookie is not being set in their browsers.
Cause: This can typically be attributed to browser cookie settings – especially if the application is not using HTTPS, or if the cookie properties are not correct.
Solution: First, ensure that you have HTTPS enabled for your application. Configure your cookie settings in a secure manner:
rememberMe()
.useSecureCookie(true)
This ensures that the cookie is only sent over secure connections.
4. Multiple Domains and Subdomains
Problem: The "Remember Me" feature does not work across different domains or subdomains.
Cause: By default, cookies are domain-specific and typically not shared across different domains.
Solution: You can set the cookie domain explicitly:
rememberMe()
.rememberMeCookieDomain("yourdomain.com")
Be cautious with security implications tied to sharing cookies across subdomains.
Best Practices for Remember Me Configuration
-
Use Secure Cookies: Always use
useSecureCookie(true)
to prevent cookies from being sent over unsecured connections. -
Use a Strong Key: Use a strong secret key for signing the remember-me token, ideally stored in a secure way (environment variables or key management services).
-
Regular Monitoring: Monitor and log authentication attempts and token events to understand usage and potential security risks.
-
Token Expiration Strategy: Think carefully about how long you want the user to remain logged in and balance user experience with security workers.
The Closing Argument
Implementing the "Remember Me" functionality in Spring Security provides users with a seamless login experience. However, it brings its own set of challenges. By understanding potential issues, experimenting with best practices, and applying robust solutions, you can enhance both security and user experience in your applications.
For further reading on Spring Security's documentation regarding remember me services, you can refer to the Spring Security Reference.
Staying informed about these common pitfalls while implementing remember-me functionality will not only help you avoid them but also make your applications more user-friendly. Be proactive about security while enhancing usability, and your users will thank you for it!