Overcoming Common Pitfalls in Architecture Spike Katas
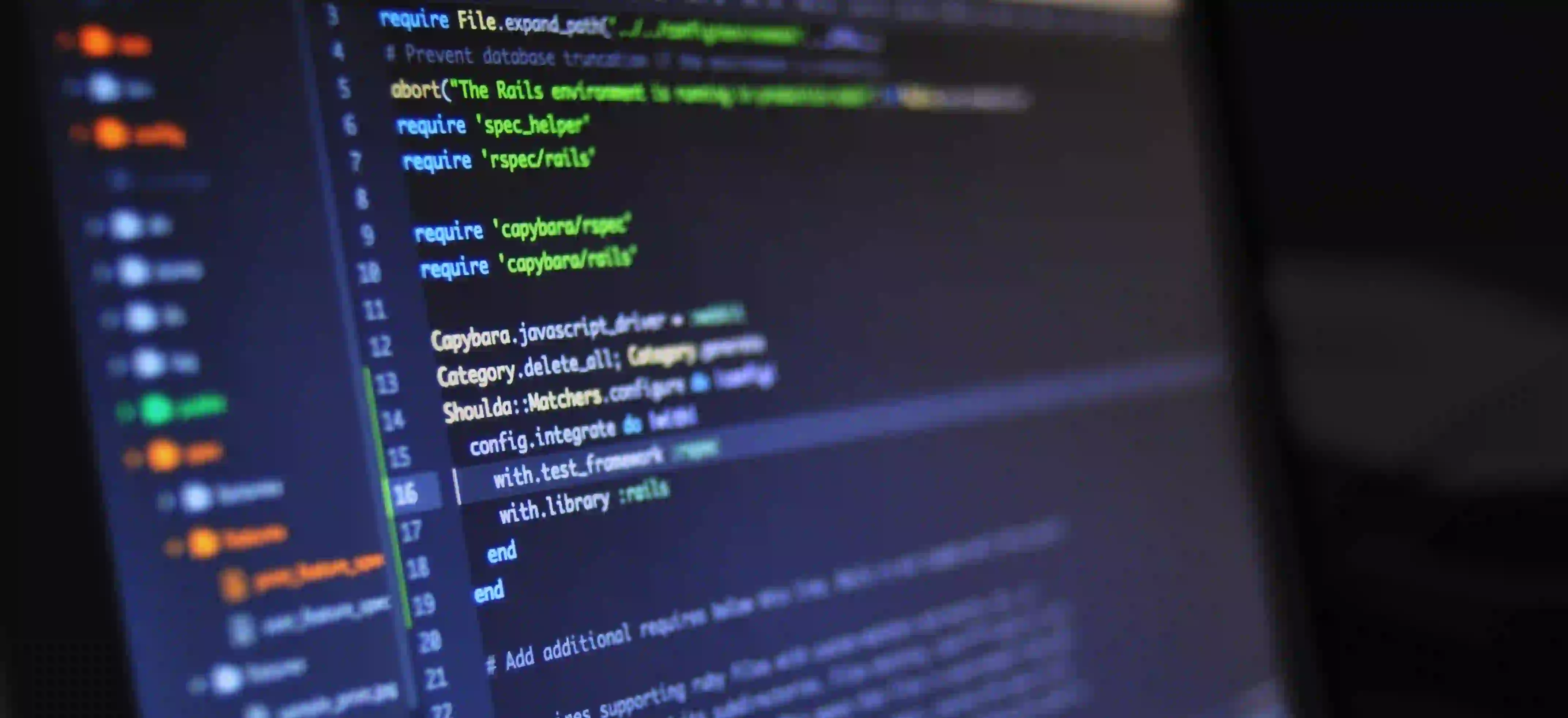
Overcoming Common Pitfalls in Architecture Spike Katas
Architecting software solutions is a critical phase in the software development lifecycle. One effective method for exploring and evaluating different architectural options is through the use of architecture spike katas. However, like any method, they come with their own challenges. In this blog post, we'll discuss common pitfalls in architecture spike katas and guide you on how to overcome them effectively.
What are Architecture Spike Katas?
Before diving into the pitfalls, it's essential to understand what architecture spike katas are. Spike katas are short, focused coding exercises designed to explore a particular technical challenge or architectural decision. They allow teams to experiment with various design patterns, technologies, and approaches without the pressure of real production code.
These exercises usually involve:
- Hands-on coding to prototype solutions
- Exploration of architectural trade-offs
- Collaborative learning among team members
Understanding these aspects sets the stage for effectively managing and executing spike katas.
Common Pitfalls in Architecture Spike Katas
1. Lack of Clear Objectives
One of the most significant pitfalls in architecture spike katas is entering the exercise without clear objectives. Without a defined goal, the team may end up exploring irrelevant options or duplicating efforts.
Solution:
- Define Clear Objectives: Before starting, outline what you want to achieve. For example, if you're considering a new microservices architecture, clarify whether you're trying to validate scalability, fault tolerance, or performance.
// Example of setting up objectives through comments
// Objective: Validate the scalability of the user service
public class UserService {
// Code to manage users
}
By setting clear objectives, your work becomes targeted and efficient.
2. Overthinking the Design
Another common issue is over-engineering the solution. Teams may get caught up in the technical intricacies, spending more time on perfecting code than on learning what they intended to explore.
Solution:
- Aim for Simplicity: Keep your initial prototypes simple. Aim for a minimum viable product (MVP) that can effectively demonstrate the architectural decision.
// Simple implementation to demonstrate architecture instead of over-engineering
public class SimpleUserService {
public String getUser(int id) {
// Simple retrieval without complex error handling or caching
return "User" + id; // Replace with actual user retrieval logic
}
}
By focusing on fundamental solutions, you minimize distractions from the main goal.
3. Neglecting to Document Findings
In the hustle of coding, documentation often takes a back seat. Neglecting to document your findings can lead to wasted effort in the future, especially when team members change.
Solution:
- Implement Documentation: Document each step, including assumptions, tests performed, and outcomes.
# Findings from UserService Spike Kata
## Objective:
Validate the response time of user retrievals via REST.
## Testing:
- Tested with 1000 concurrent users.
- Average response time: 50ms.
## The Bottom Line:
The simple retrieval method is scalable under current load.
Documentation codifies your learnings, making your efforts repeatable and transferable.
4. Failing to Involve the Whole Team
Architecture spike katas are most beneficial when all relevant stakeholders participate. Often, teams may disregard input from sections like QA, operations, or business analysis.
Solution:
- Cross-Disciplinary Participation: Invite diverse roles into the kata sessions to garner different insights. Their unique perspectives can lead to improved decision-making and more holistic solutions.
// Example of collaborative input
public class UserService {
private final NotificationService notificationService;
// Constructor dependency injection to include feedback from different roles
public UserService(NotificationService notificationService) {
this.notificationService = notificationService;
}
}
Emphasizing collaboration strengthens the product.
5. Ignoring Refactoring
A common outcome of spike katas is a code base that evolves quickly but may not adhere to best practices. Teams may neglect refactoring, resulting in technical debt.
Solution:
- Refactor as You Go: Allocate time for refactoring back into your kata sessions. Ensure a clean code structure is maintained throughout.
// Refactored example to improve readability and modularity
public class UserService {
private Map<Integer, User> userStore = new HashMap<>();
public User getUser(int id) {
return userStore.get(id);
}
public void addUser(User user) {
userStore.put(user.getId(), user);
}
}
Maintaining clean code mitigates future challenges.
6. Not Running Tests
A pitfall many teams encounter is skipping tests. While the primary goal is exploration, not testing can lead to wrong assumptions about performance and functionality.
Solution:
- Implement Tests Gradually: Use TDD (Test-Driven Development) principles to gradually introduce testing into your katas. Validate your findings with unit tests.
// Example of a simple unit test for UserService
import static org.junit.jupiter.api.Assertions.assertEquals;
public class UserServiceTest {
@Test
public void testGetUser() {
UserService userService = new UserService();
userService.addUser(new User(1, "Alice"));
assertEquals("Alice", userService.getUser(1).getName());
}
}
Validating assumptions through tests cultivates reliability.
The Bottom Line
Architecture spike katas can serve as powerful tools for exploring architectural options, but they require discipline and strategy to avoid common pitfalls. By defining clear objectives, prioritizing simplicity, involving the whole team, and maintaining a focus on documentation, collaboration, and testing, you can maximize the benefits of your katas.
For further reading on effective software architecture practices, you may find Martin Fowler's article on microservices insightful, as well as The Twelve-Factor App, a methodology for building software-as-a-service applications.
Start your next architecture spike kata today, and turn your explorations into robust, production-worthy solutions!