Java Solutions for Common Dialogflow Chatbot Issues
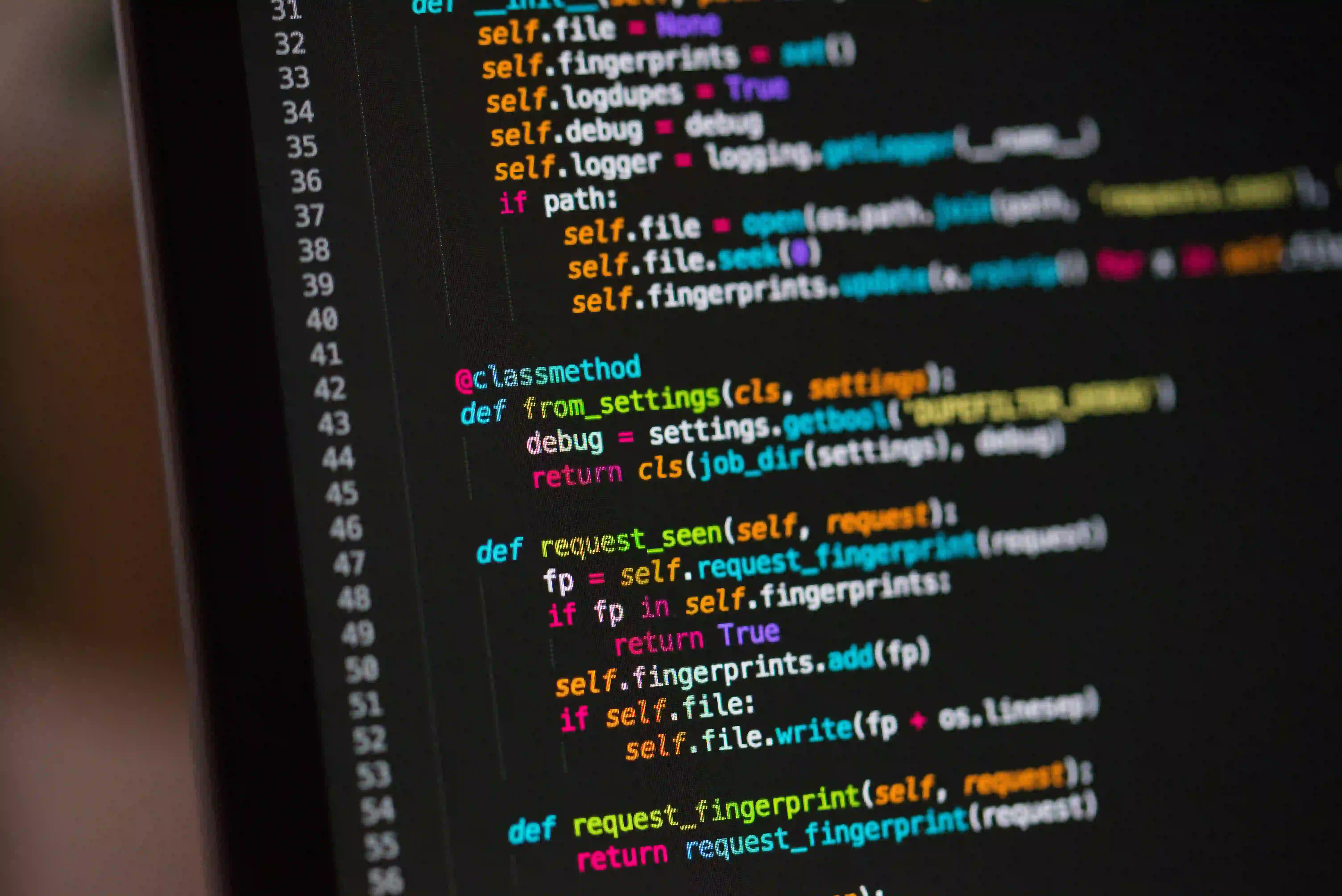
Java Solutions for Common Dialogflow Chatbot Issues
Chatbots have become a pivotal part of customer interaction in the digital age. As organizations increasingly implement chatbots, Dialogflow emerges as a powerful platform for building conversational interfaces. However, working with Dialogflow presents several challenges, especially for developers using Java. In this blog post, we will explore common issues faced during Dialogflow chatbot development and provide practical Java solutions to overcome them. For further insights, you can check out the article titled Overcoming Common Challenges in Dialogflow Chatbot Development.
Understanding Dialogflow
Before diving into solutions, it's crucial to understand what Dialogflow is. Dialogflow is a Google-owned platform that utilizes natural language processing (NLP) to facilitate interactions between users and chatbots. It allows developers to create conversational agents that can understand and respond to user intents effectively.
However, challenges may arise during the development process. Here are some common issues developers encounter, along with Java-based solutions.
Common Challenges and Java Solutions
1. Authentication with Google Cloud
One of the first hurdles while working with Dialogflow is successfully authenticating with Google Cloud. Authentication is crucial for ensuring secure access to the Dialogflow API.
Solution:
To authenticate, you can use service account keys. Here's how to implement authentication in your Java application:
import com.google.api.client.googleapis.auth.oauth2.GoogleCredential;
import com.google.api.services.dialogflow.v2.Dialogflow;
import com.google.api.services.dialogflow.v2.DialogflowScopes;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Collections;
public class DialogflowAuth {
private static final String PROJECT_ID = "your_project_id";
private static final String CREDENTIALS_FILE_PATH = "path_to_your_credentials.json";
public static Dialogflow authorize() throws IOException {
GoogleCredential credential = GoogleCredential.fromStream(new FileInputStream(CREDENTIALS_FILE_PATH))
.createScoped(Collections.singleton(DialogflowScopes.DIALOGFLOW));
return new Dialogflow.Builder(credential.getTransport(), credential.getJsonFactory(), credential)
.setApplicationName(PROJECT_ID)
.build();
}
}
Why this code?
This code uses the Google API libraries to authenticate with Dialogflow. The GoogleCredential
class simplifies the process of managing access credentials and scopes. This ensures that your Java application can interact securely with your Dialogflow agent.
2. Error Handling
When building a chatbot, handling errors gracefully is paramount. Common errors could range from network issues to unrecognized intents.
Solution:
Implement basic error handling using try-catch blocks to catch exceptions and provide user-friendly messages.
try {
Dialogflow dialogflow = DialogflowAuth.authorize();
// Call Dialogflow API methods here
} catch (IOException e) {
System.out.println("Error authenticating with Dialogflow: " + e.getMessage());
} catch (Exception e) {
System.out.println("An unexpected error has occurred: " + e.getMessage());
}
Why this code?
The use of try-catch blocks allows for smoother operation and better user experience. By catching exceptions, developers can avoid application crashes and provide meaningful feedback to the users.
3. Language Support for Different Locales
Developers often need to handle user inputs in various languages. Dialogflow supports multiple languages, but your Java application must be correctly configured to accommodate this.
Solution:
Set up your Dialogflow agent with language information in your requests. A sample implementation would look like this:
import com.google.api.services.dialogflow.v2.model.DetectIntentRequest;
import com.google.api.services.dialogflow.v2.model.QueryInput;
public void detectIntent(String sessionId, String query, String languageCode) {
DetectIntentRequest request = new DetectIntentRequest()
.setQueryInput(new QueryInput().setText(new TextInput()
.setText(query)
.setLanguageCode(languageCode)));
try {
detectIntentResponse = dialogflow.projects().agent().sessions().detectIntent(sessionId, request).execute();
System.out.println("Response: " + detectIntentResponse.getQueryResult().getFulfillmentText());
} catch (IOException e) {
e.printStackTrace();
}
}
Why this code?
This code demonstrates how to send a user query to the Dialogflow agent while specifying the desired language. By customizing the languageCode
, the chatbot can respond accurately based on the locale of the user input.
4. Session Management
Managing sessions can be tricky without a proper strategy, especially when dealing with multiple users simultaneously.
Solution:
Utilize a session identifier to ensure that interactions are correctly mapped to the user.
import java.util.HashMap;
import java.util.Map;
public class SessionManager {
private Map<String, Session> sessions = new HashMap<>();
public void startSession(String userId) {
sessions.put(userId, new Session(userId));
}
public Session getSession(String userId) {
return sessions.get(userId);
}
}
Why this code?
This implementation of a simple session manager allows you to keep track of different user sessions effectively. Using a HashMap
ensures quick access and management of user-specific interactions.
5. Integration with Other Services
Often, chatbots need to integrate with external services, whether for querying a database or accessing APIs.
Solution:
Make HTTP requests using libraries such as OkHttp
or Apache HttpClient
.
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class ExternalServiceIntegration {
private final OkHttpClient client = new OkHttpClient();
public String fetchExternalData(String url) throws IOException {
Request request = new Request.Builder()
.url(url)
.build();
try (Response response = client.newCall(request).execute()) {
return response.body().string();
}
}
}
Why this code?
The code uses OkHttp
for its efficiency and simplicity. It establishes an HTTP GET request to an external API and returns the fetched data, enabling the chatbot to enrich interactions with additional information.
Closing Remarks
While building a chatbot with Dialogflow in Java, you will likely face various challenges. However, with the right strategies and code snippets, you can streamline the process and enhance your chatbot's capabilities.
Having covered the common issues you may face when developing chatbots using Dialogflow, you are now better equipped to tackle these challenges head-on. Always remember that the development process is iterative, so learning from each deployment helps refine your solutions further.
For more insights on dialog management and overcoming challenges while developing Dialogflow chatbots, be sure to read Overcoming Common Challenges in Dialogflow Chatbot Development.
By embracing these Java solutions, you can create a smooth, user-friendly chatbot experience that meets the needs of your organization and its customers. Happy coding!