Optimizing Java Search Algorithms: Max/Min Techniques
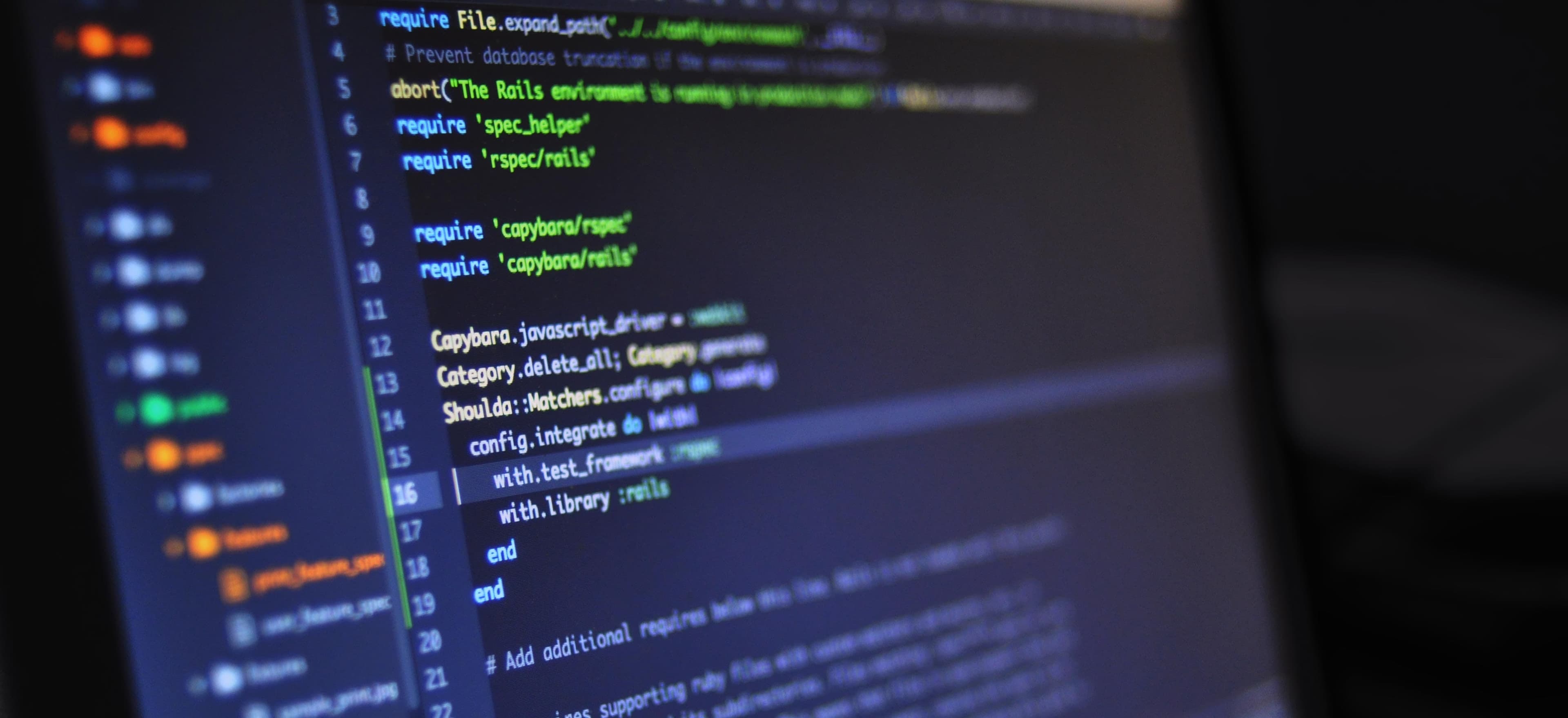
- Published on
Optimizing Java Search Algorithms: Max/Min Techniques
In the world of programming, choosing the right algorithm can be a game changer. Whether you are building complex applications or engaging in simple coding exercises, understanding how to efficiently conduct search operations is key. This blog post will explore maximizing and minimizing search algorithms in Java, shedding light on strategies to optimize their performance.
A well-known technique to find maximum and minimum in a dataset is through search algorithms. In this exploration, we'll delve deep into Java implementations while drawing inspiration from a related article titled Mastering Binary Search: Finding Max/Min in JavaScript.
Understanding the Basics
Before jumping into the specifics, let's discuss what we're addressing with max/min search algorithms. At its core, searching for the maximum or minimum in a collection of values is about efficiency. The two most commonly used data structures for searching purposes are arrays and lists.
Why Max/Min Algorithms?
- Efficiency: Searching for maximum or minimum values, especially in large datasets, can be computationally intensive. Optimizing these algorithms can drastically enhance performance.
- Applications: From statistical analyses to game development, max/min operations are frequently encountered.
- Algorithm Familiarity: Understanding these algorithms lays a foundation for grasping more advanced concepts in computer science.
The Linear Search Approach
The simplest method to find the min or max in an array is through linear search. This involves traversing each element of the array to check for the maximum or minimum value.
Implementation
public class LinearSearch {
public static int findMax(int[] arr) {
int max = arr[0];
for (int i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i]; // Update max if current element is greater
}
}
return max;
}
public static int findMin(int[] arr) {
int min = arr[0];
for (int i = 1; i < arr.length; i++) {
if (arr[i] < min) {
min = arr[i]; // Update min if current element is lesser
}
}
return min;
}
public static void main(String[] args) {
int[] numbers = {3, 5, 1, 8, 4};
System.out.println("Max: " + findMax(numbers)); // Outputs: Max: 8
System.out.println("Min: " + findMin(numbers)); // Outputs: Min: 1
}
}
Commentary
Although the linear search is straightforward, its time complexity is O(n). This means that in the worst-case scenario, you will need to check each element of the array. This is inefficient for large datasets.
The Divide and Conquer Strategy
To enhance performance, employ a divide-and-conquer approach. This technique can drastically decrease the number of comparisons necessary to find the max or min values.
Implementation
public class DivideAndConquer {
public static int findMax(int[] arr, int left, int right) {
if (left == right) {
return arr[left]; // Base case: only one element
} else {
int mid = (left + right) / 2;
int leftMax = findMax(arr, left, mid);
int rightMax = findMax(arr, mid + 1, right);
return Math.max(leftMax, rightMax); // Return the max of the two halves
}
}
public static int findMin(int[] arr, int left, int right) {
if (left == right) {
return arr[left]; // Base case: only one element
} else {
int mid = (left + right) / 2;
int leftMin = findMin(arr, left, mid);
int rightMin = findMin(arr, mid + 1, right);
return Math.min(leftMin, rightMin); // Return the min of the two halves
}
}
public static void main(String[] args) {
int[] numbers = {3, 5, 1, 8, 4};
System.out.println("Max: " + findMax(numbers, 0, numbers.length - 1)); // Outputs: Max: 8
System.out.println("Min: " + findMin(numbers, 0, numbers.length - 1)); // Outputs: Min: 1
}
}
Commentary
With this approach, the time complexity is O(log n) compared to O(n) in linear search. It effectively reduces the problem size and combines the results from subproblems. However, this method is slightly more complex to implement and can be less efficient than linear search for very small arrays.
When to Use Each Approach?
- Linear Search: Ideal for small datasets where simplicity matters more than performance.
- Divide and Conquer: Best for larger datasets where the performance gain is significant.
Using Java Collections
Java Collections Framework provides robust machinery for searching through data structures. For instance, using Collections.max()
and Collections.min()
allows you to avoid manual implementations.
Implementation
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
public class CollectionsMaxMin {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(3, 5, 1, 8, 4);
int max = Collections.max(numbers);
int min = Collections.min(numbers);
System.out.println("Max: " + max); // Outputs: Max: 8
System.out.println("Min: " + min); // Outputs: Min: 1
}
}
Commentary
Using Java’s built-in capabilities can often be the best option when working with collections, both for ease and performance. However, understanding the underlying operations can be beneficial for optimization and debugging purposes.
Key Takeaways
Optimizing search algorithms, particularly for finding maximum and minimum values, is a fundamental skill in Java programming. Whether you choose linear search, divide and conquer, or leverage the Java Collections Framework, understanding the principles behind these techniques will enable you to write more efficient code.
Refer to the article Mastering Binary Search: Finding Max/Min in JavaScript for insights into how similar concepts are applied in JavaScript, reinforcing your grasp of these crucial algorithms.
By continuously practicing these techniques and understanding their nuances, you will be well on your way to mastering search algorithms in Java. Don’t forget to optimize based on the context of your application, as performance often depends on the specific use case!