Tackling Java Integration Issues in Dialogflow Chatbots
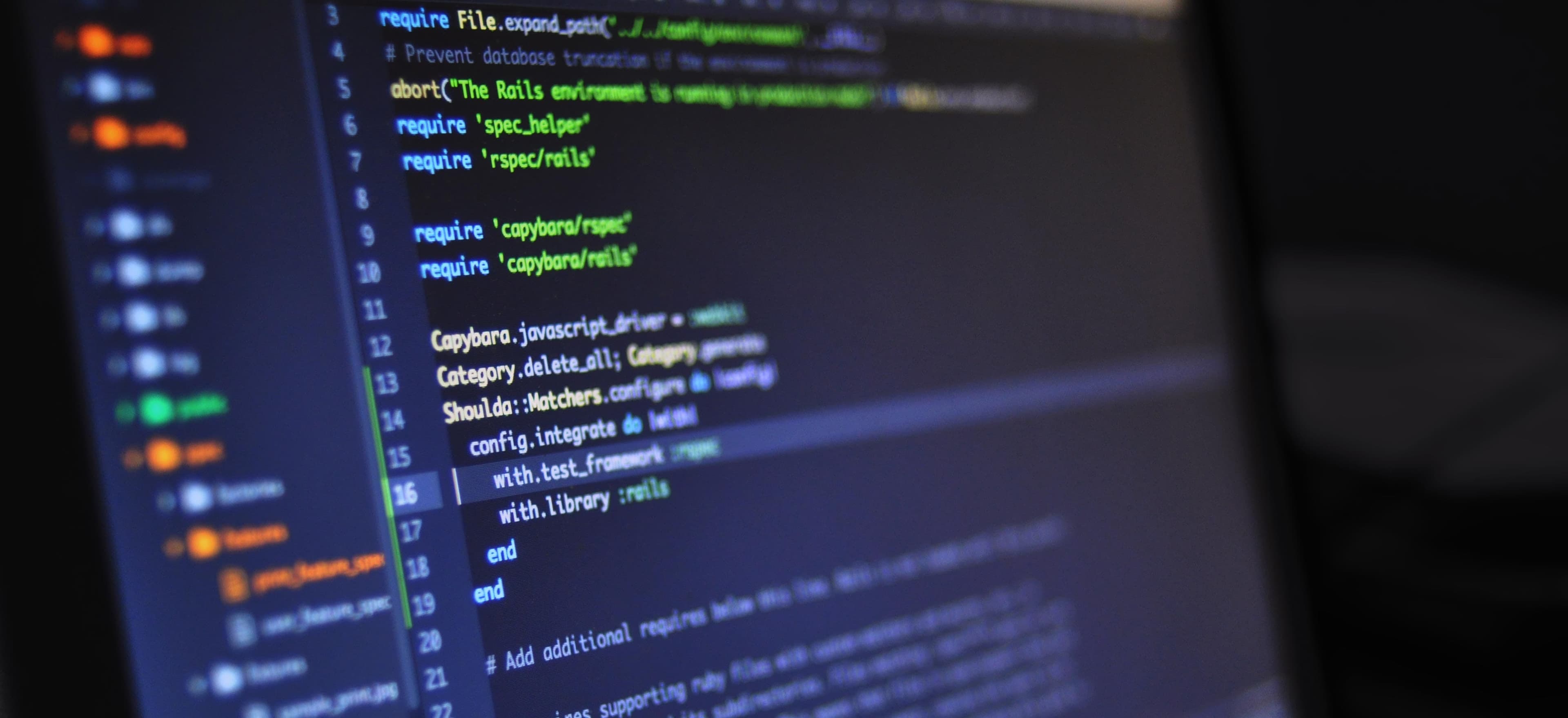
- Published on
Tackling Java Integration Issues in Dialogflow Chatbots
The landscape of modern applications is continuously evolving, and chatbots have taken center stage in enhancing user interaction and providing immediate responses. Google’s Dialogflow simplifies the creation of sophisticated chatbots, leveraging natural language processing to enable seamless conversations. However, when integrating Java as the back-end for your Dialogflow chatbot, developers often encounter several challenges. This blog post aims to equip you with the knowledge to tackle Java integration issues when building chatbots, ensuring a smoother development process and more effective applications.
Understanding Dialogflow and Java Integration
Before we delve into the challenges, let's clarify why Java is commonly utilized in backend development and how it interacts with Dialogflow.
Dialogflow is a natural language understanding platform that allows developers to design conversational user interfaces. By integrating Dialogflow with Java, you can handle business logic, communicate with databases, and provide personalized responses. Java, known for its scalability and performance, is a fitting choice for such backend operations.
Core Challenges in Java-Dialogflow Integration
Transitioning between Dialogflow and Java applications can present obstacles. Here are some common issues developers face:
-
API Calls and Webhook Setup: Dialogflow expects a webhook to handle fulfillment requests, which often involves setting up API calls correctly.
-
JSON Parsing: The communication between Dialogflow and your Java backend typically involves exchanging JSON data. Parsing this data can introduce complexity.
-
Asynchronous Handling: The nature of chatbots means that responses must be quick and seamless, necessitating effective asynchronous processing.
-
Error Handling: Properly managing errors between Dialogflow and your Java application is critical for maintaining an effective user experience.
By identifying these challenges, we can focus on solutions.
Solution 1: Correctly Setting Up API Calls and Webhook
To enable Dialogflow to send requests to your Java application, you must create a RESTful webhook endpoint.
Example Code Snippet: Setting Up a Webhook in Java
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class DialogflowWebhook extends HttpServlet {
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String requestBody = request.getReader().lines()
.collect(Collectors.joining(System.lineSeparator()));
// Process the Dialogflow request here
// Send a response back to Dialogflow
response.setContentType("application/json");
response.getWriter().write("{\"fulfillmentText\": \"Hello from Java!\"}");
}
}
Commentary on the Code
This Java servlet listens for POST requests from Dialogflow. Here’s the breakdown:
- HttpServlet: We extend this class to create a servlet that handles POST requests.
- doPost: This method is invoked when Dialogflow makes a request, allowing us to capture the incoming data.
- response: The JSON response sent back to Dialogflow contains text that the chatbot will read aloud to the user. This structure needs to be maintained for a seamless chat experience.
For setting up your web server and deploying this servlet, you can rely on platforms like Google Cloud Run.
Solution 2: Parsing JSON Data
The interaction with Dialogflow results in complex JSON data structures that need to be parsed correctly. Using libraries such as Jackson or Gson can simplify this process.
Example Code Snippet: Using Gson for JSON Parsing
import com.google.gson.Gson;
public class DialogflowRequest {
public String queryResult;
// Constructor and other necessary methods
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws IOException {
String requestBody = request.getReader().lines()
.collect(Collectors.joining(System.lineSeparator()));
Gson gson = new Gson();
DialogflowRequest dialogflowRequest = gson.fromJson(requestBody, DialogflowRequest.class);
// Use dialogflowRequest.queryResult for further processing
}
Commentary on the Code
- Gson: This library helps convert JSON strings into Java objects and vice versa, streamlining the process.
- DialogflowRequest Class: It's crucial to create a class structure that matches the incoming JSON format for effective mapping.
This approach can significantly reduce errors in data handling and improve the clarity of your code.
Solution 3: Managing Asynchronous Processing
When it comes to chatbots, response times are essential. You might want to call external services that take time to respond or run computations that delay feedback. To mitigate this, consider using asynchronous processing techniques available in Java, such as CompletableFuture.
Example Code Snippet: CompletableFuture for Asynchronous Behavior
import java.util.concurrent.CompletableFuture;
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws IOException {
CompletableFuture.supplyAsync(() -> {
// Perform time-consuming tasks, e.g., fetching data from external APIs
// Return a string or object as a response
}).thenAccept(result -> {
try {
response.setContentType("application/json");
response.getWriter().write(result);
} catch (IOException e) {
e.printStackTrace();
}
});
}
Commentary on the Code
- CompletableFuture.supplyAsync: This method runs the task in a separate thread, keeping the main thread free to handle other requests.
- thenAccept: Once the asynchronous task is complete, this method processes the result, ensuring that responses are sent back to Dialogflow promptly.
This strategy leads to increased efficiency and better overall user experience.
Solution 4: Effective Error Handling
Errors can occur at any point when integrating Java with Dialogflow. Proper error handling helps maintain the robustness of your application. Ensure that you handle exceptions gracefully and provide meaningful feedback.
Example Code Snippet: Basic Error Handling
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws IOException {
try {
// Processing logic...
} catch (Exception e) {
response.setStatus(HttpServletResponse.SC_INTERNAL_SERVER_ERROR);
response.getWriter().write("{\"error\": \"An error occurred while processing your request.\"}");
e.printStackTrace(); // For debugging purposes
}
}
Commentary on the Code
- try-catch Block: Surrounding processing code with a try-catch block captures exceptions, allowing you to manage them effectively.
- HTTP Status Codes: Return relevant status codes to indicate success or failure, which can be critical in debugging.
Bringing It All Together
Integrating Java with Dialogflow can enhance your chatbot's capabilities but comes with its challenges. By understanding and resolving common issues related to API calls, JSON parsing, asynchronous processing, and error handling, you can build a robust and efficient chatbot.
For further reading, consider exploring the article titled Overcoming Common Challenges in Dialogflow Chatbot Development, which provides valuable insights applicable to this integration process.
Java provides a powerful backbone to your Dialogflow chatbot, and by employing best practices outlined above, you can ensure a streamlined, effective integration that improves user experience. Happy coding!