Optimizing Java's Binary Search for Max/Min Values
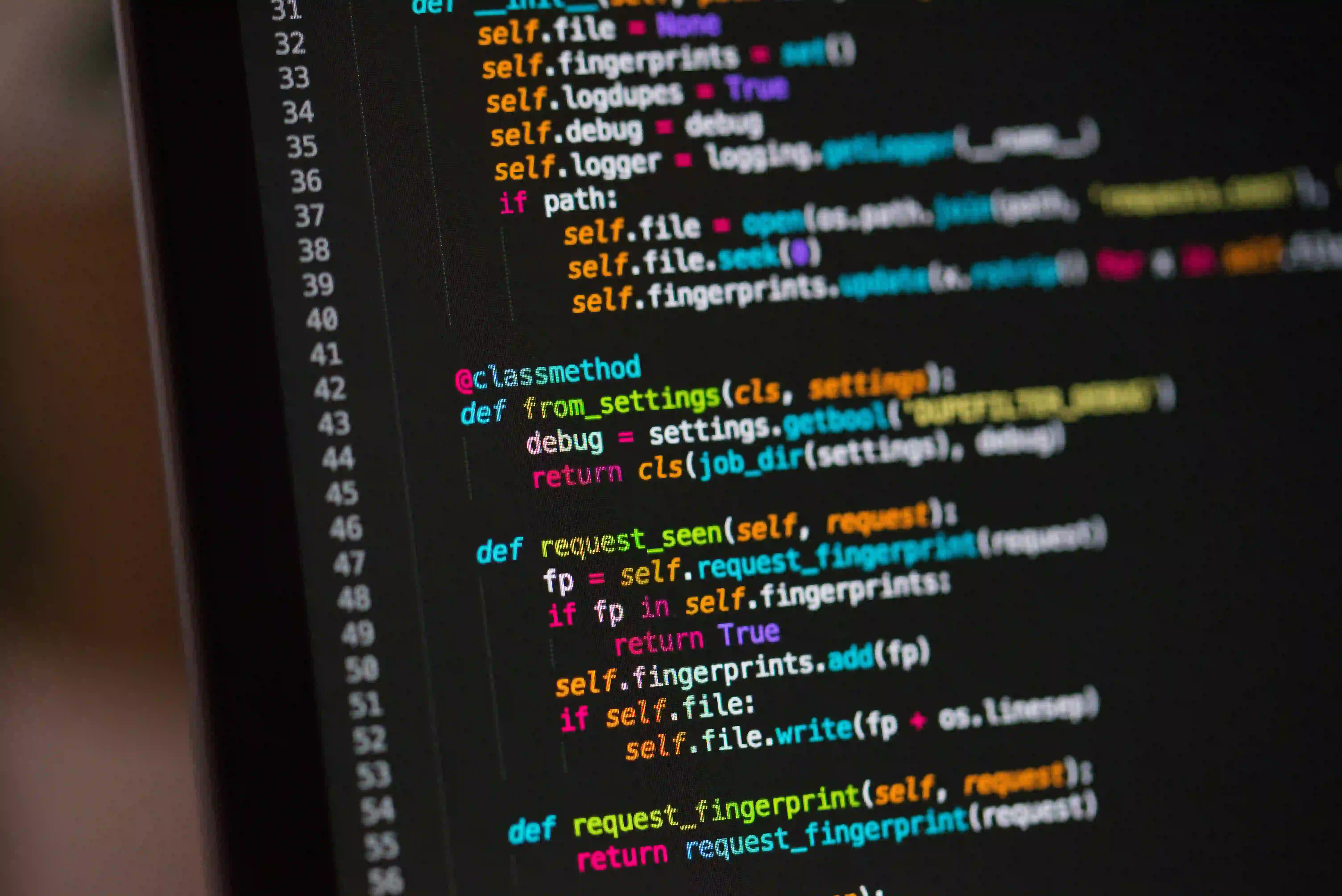
Optimizing Java's Binary Search for Max/Min Values
Binary search is a well-known algorithm that efficiently finds the position of a target value within a sorted array. But what happens when you're looking for the maximum or minimum value of a numerical sequence? In this post, we will explore ways to optimize binary search specifically for locating these values, with a particular focus on its implementation in Java.
We'll also draw parallels to an existing discussion in the article Mastering Binary Search: Finding Max/Min in JavaScript, which delves into similar concepts in JavaScript.
Understanding Binary Search
The Basics
At its core, binary search operates on a sorted array by splitting the array into halves and progressively narrowing down the possible locations of the target value. The basic idea is to determine whether the middle element is less than, greater than, or equal to the target value.
Complexity
This search method has a time complexity of O(log n) due to the halving process, making it extremely efficient compared to linear search (O(n)), which scans each element one by one.
public static int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2; // Prevent potential overflow
if (arr[mid] == target) {
return mid; // Target found
} else if (arr[mid] < target) {
left = mid + 1; // Move right
} else {
right = mid - 1; // Move left
}
}
return -1; // Target not found
}
Why Binary Search?
Binary search is not only faster; it can be adapted for various use cases, such as finding the maximum or minimum values in specific constraints, like unimodal arrays or specific value properties.
Finding Max/Min Values Using Binary Search
Problem Definition
Instead of looking for a specific target value, you may want to find the maximum or minimum value under certain conditions, such as:
- The maximum value in a bitonic array (an array that first increases and then decreases).
- The transition point in a bitonic array.
In the next sections, we'll dissect these scenarios and provide solutions.
Example: Finding the Maximum in a Bitonic Array
A bitonic array is an array that first strictly increases, then strictly decreases. Our aim is to locate the maximum value efficiently using binary search.
Implementation
Here's a simplified implementation in Java:
public static int findMaxInBitonic(int[] arr) {
int left = 0;
int right = arr.length - 1;
while (left < right) {
int mid = left + (right - left) / 2;
// Check if the mid element is greater than the next element
if (mid > 0 && arr[mid] > arr[mid + 1]) {
right = mid; // Maximum lies to the left (including mid)
} else {
left = mid + 1; // Maximum lies to the right
}
}
return arr[left]; // left and right converge to the maximum
}
Code Explanation
- Initialization: We set left to 0 and right to the last index of the array.
- Mid Calculation: Find the midpoint to divide the search space.
- Comparison: If the middle value is greater than its next value, then the maximum is on the left side; otherwise, it lies on the right side.
- Termination: When left equals right, we have found our maximum.
Finding the Minimum in a Bitonic Array
Finding the minimum value in the same type of array involves a slightly different approach, focusing on the decreasing part.
Implementation
public static int findMinInBitonic(int[] arr) {
int left = 0;
int right = arr.length - 1;
while (left < right) {
int mid = left + (right - left) / 2;
// Compare with previous element to ensure the left part is decreasing
if (mid > 0 && arr[mid] < arr[mid - 1]) {
return arr[mid]; // mid is the minimum
} else if (arr[mid] < arr[right]) {
right = mid; // Minimum lies towards the left
} else {
left = mid + 1; // Minimum lies towards the right
}
}
return arr[left]; // Final left equals right
}
Code Explanation
- Identify Midpoint: Calculate the mid index as before.
- Find the Minimum: If the middle element is less than its previous element, it is the minimum.
- Adjust Bounds: Similar to the maximum case, adjust the search bounds based on comparisons.
Additional Considerations
Edge Cases
- Empty Array: Always check for empty input to avoid errors.
- Single Element Array: Should trivially return that element.
Testing Your Implementation
It's crucial to write test cases to verify that your implementation is correct. You can use JUnit, a widely-used testing framework in Java.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class BitonicTest {
@Test
public void testFindMaxInBitonic() {
int[] arr = {1, 3, 8, 12, 4, 2};
assertEquals(12, findMaxInBitonic(arr));
}
@Test
public void testFindMinInBitonic() {
int[] arr = {3, 2, 1, 0, -1, -5};
assertEquals(-5, findMinInBitonic(arr));
}
}
A Final Look
Mastering binary search enables you to tackle not just direct searches but also multidimensional queries such as finding the maximum or minimum values in diverse conditions like bitonic arrays. This advanced skill will undoubtedly enhance your programming repertoire.
For those interested in a broader discussion on binary search applications, check out the insightful article on Mastering Binary Search: Finding Max/Min in JavaScript. As always, practice is crucial. Explore and implement these examples, enrich your understanding, and keep coding!
Ultimately, mastering these concepts will not only improve the efficiency of your algorithms but also enhance the performance of your applications significantly. Happy coding!