Optimizing Java Algorithms for Max/Min Value Searches
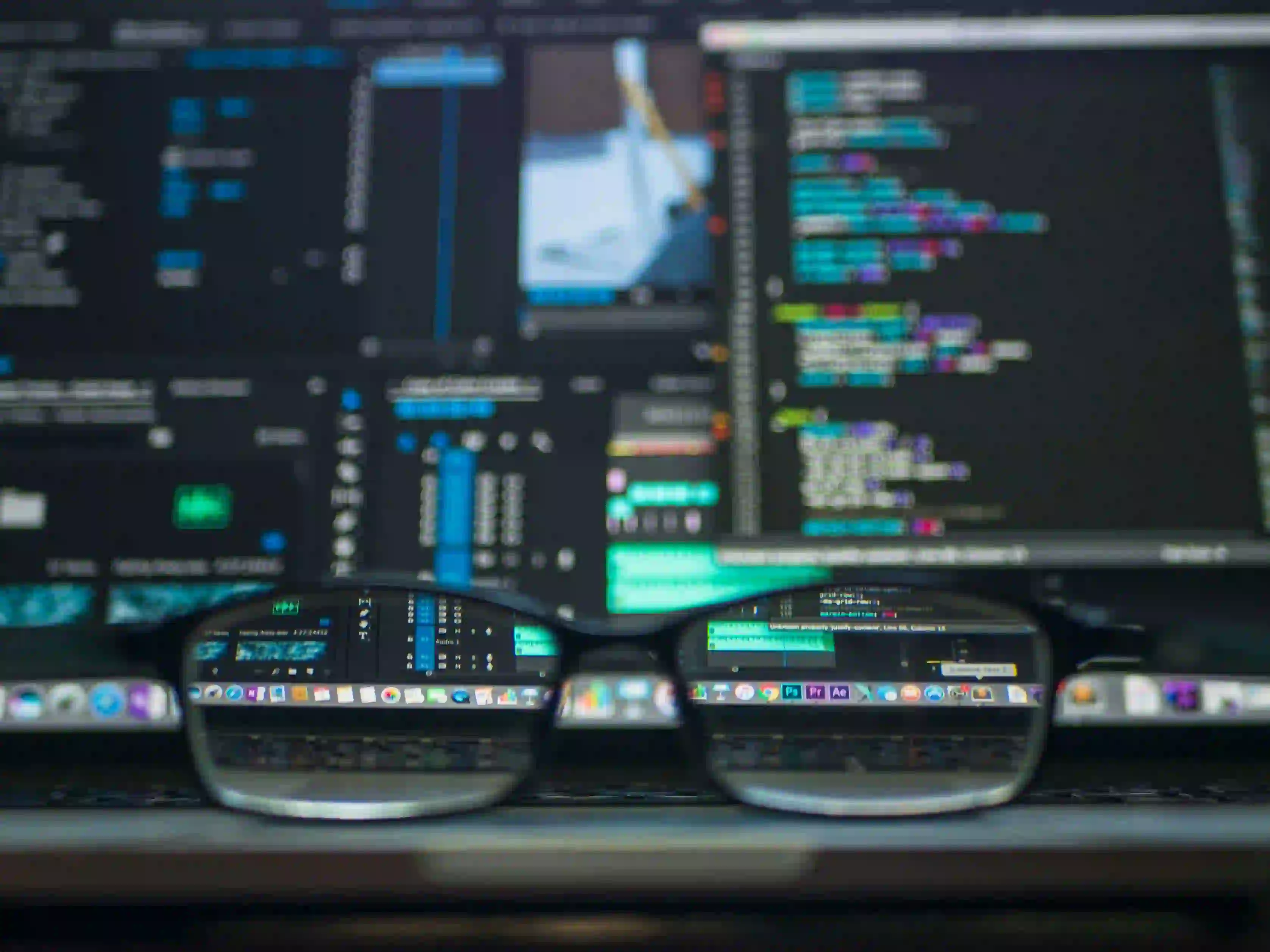
Optimizing Java Algorithms for Max/Min Value Searches
In the world of programming, efficient algorithms are crucial, especially when it comes to searching for maximum or minimum values. These searches can significantly affect the performance of applications, especially when handling large data sets. While Java has a rich set of libraries to simplify these tasks, knowing how to implement your own optimized searches can be a game changer. In this article, we will explore effective strategies for searching max/min values in Java, drawing parallels with concepts discussed in the existing article Mastering Binary Search: Finding Max/Min in JavaScript.
Understanding the Problem
Before diving into the code, let's clarify what we mean by maximizing or minimizing values. In programming tasks, you're often faced with a scenario where you need to extract the highest or lowest value from an array or list. This may seem straightforward, but the way you perform this operation can greatly influence performance.
Time Complexity Matters
It's essential to understand time complexity when working with algorithms. A simple linear search has a time complexity of O(n), meaning it scans through each element. For larger data sets, this can lead to increased latency. However, with sorting (O(n log n)) or leveraging binary search for sorted arrays, you can significantly reduce the search time.
Max/Min Search in Unsorted Arrays
Let's start with the basic scenario: finding the maximum or minimum value in an unsorted array.
Code Example: Finding Max/Min
Here’s how you can find the maximum and minimum values in an unsorted array:
public class MinMaxFinder {
public static int[] findMinMax(int[] numbers) {
int max = Integer.MIN_VALUE; // Start with the smallest possible value
int min = Integer.MAX_VALUE; // Start with the largest possible value
for (int number : numbers) {
if (number > max) {
max = number; // Update max if current number is greater
}
if (number < min) {
min = number; // Update min if current number is smaller
}
}
return new int[]{min, max};
}
}
Commentary
In this code, we initialize max
and min
to extreme values. This guarantees that any number in the array will adjust these initial values on the first comparison. The loop then iterates through the array, checking each number and updating max
and min
accordingly. This approach runs in O(n) time complexity, which is optimal for unsorted arrays.
Optimizing for Sorted Arrays with Binary Search
Once you have a sorted array, you can take advantage of the properties of binary search. For finding max or min values in a sorted array, you can directly access the first or last elements.
Code Example: Finding Max/Min in a Sorted Array
public class SortedMinMaxFinder {
public static int[] findMinMax(int[] sortedNumbers) {
if (sortedNumbers == null || sortedNumbers.length == 0) {
throw new IllegalArgumentException("Array cannot be null or empty");
}
return new int[]{sortedNumbers[0], sortedNumbers[sortedNumbers.length - 1]};
}
}
Commentary
This method utilizes the knowledge that in a sorted array, the minimum is always the first element, and the maximum is always the last element. This makes our solution O(1), which is the most efficient complexity for maximum and minimum searches in sorted data.
Handling Special Cases
Real-world data can contain special cases worth considering. For instance, if your array is empty or contains null values, your algorithm should gracefully handle these conditions.
Code Example: Updated Min/Max Finder with Error Handling
public class RobustMinMaxFinder {
public static int[] findMinMax(int[] numbers) {
if (numbers == null || numbers.length == 0) {
throw new IllegalArgumentException("Array cannot be null or empty");
}
int max = Integer.MIN_VALUE;
int min = Integer.MAX_VALUE;
for (int number : numbers) {
if (number > max) {
max = number;
}
if (number < min) {
min = number;
}
}
return new int[]{min, max};
}
}
Commentary
This code improves robustness by adding checks to ensure that the input is valid. Adding null
or empty checks prevents potential runtime errors. User-friendliness in error messaging helps developers understand and troubleshoot issues in their implementations quickly.
Multithreaded Approaches
For large-scale data or databases, employing multithreading can also optimize the max/min search. The idea here is to divide the array into smaller chunks and process them in parallel threads, subsequently combining the results.
Code Example: Simple Multithreaded Max/Min Finder
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class ParallelMinMaxFinder {
private static class MinMaxTask implements Callable<int[]> {
private final int[] numbers;
public MinMaxTask(int[] numbers) {
this.numbers = numbers;
}
@Override
public int[] call() {
int max = Integer.MIN_VALUE;
int min = Integer.MAX_VALUE;
for (int number : numbers) {
if (number > max) {
max = number;
}
if (number < min) {
min = number;
}
}
return new int[]{min, max};
}
}
public static int[] findMinMax(int[] numbers, int numThreads) throws InterruptedException, ExecutionException {
int length = numbers.length;
int chunkSize = length / numThreads;
ExecutorService executor = Executors.newFixedThreadPool(numThreads);
Future<int[]>[] futures = new Future[numThreads];
for (int i = 0; i < numThreads; i++) {
int start = i * chunkSize;
int end = (i == numThreads - 1) ? length : (i + 1) * chunkSize;
futures[i] = executor.submit(new MinMaxTask(Arrays.copyOfRange(numbers, start, end)));
}
int globalMin = Integer.MAX_VALUE;
int globalMax = Integer.MIN_VALUE;
for (Future<int[]> future : futures) {
int[] result = future.get();
globalMin = Math.min(globalMin, result[0]);
globalMax = Math.max(globalMax, result[1]);
}
executor.shutdown();
return new int[]{globalMin, globalMax};
}
}
Commentary
This approach introduces a callable task to find min and max in chunks of the main array. It utilizes Java's ExecutorService
to handle the threads efficiently. After processing, it combines results to provide the final min and max values. This method can significantly enhance performance when dealing with extensive datasets.
Closing the Chapter
Optimizing algorithms for finding maximum and minimum values on data sets is essential for improving application performance. Java provides robust methodologies, but understanding the fundamentals behind these techniques can lead to more efficient programming.
From simple searches in unsorted arrays to optimized binary searches in sorted arrays, each approach has its use cases. Furthermore, considering multithreading for large-scale data can take your solutions to the next level.
For further reading on efficient searching techniques, you may want to explore the article Mastering Binary Search: Finding Max/Min in JavaScript, which delves into similar concepts in JavaScript while providing deeper insights into these algorithmic methods. Stay tuned for more discussions and coding examples to enhance your Java skills!