Java Layout Management: Preventing Common Column Mistakes
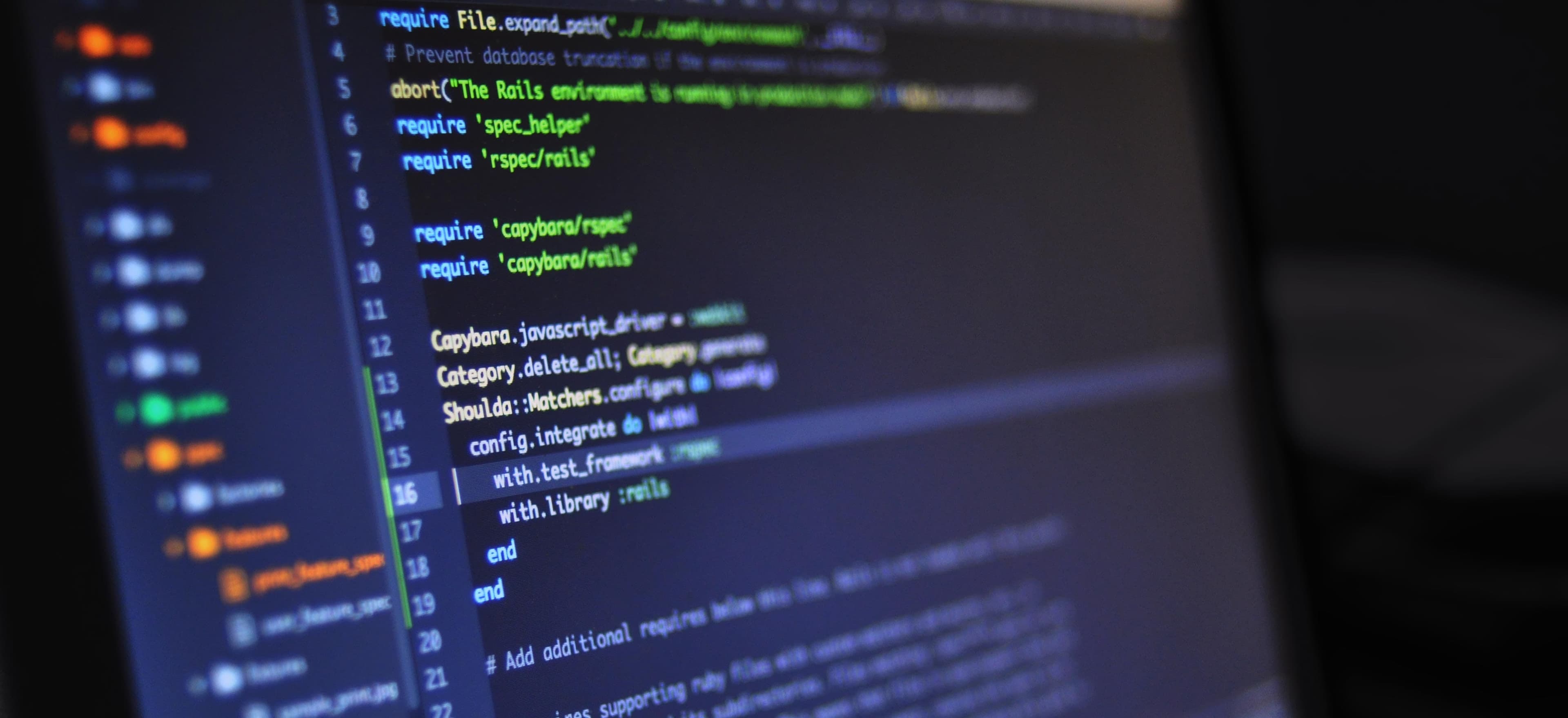
- Published on
Java Layout Management: Preventing Common Column Mistakes
When developing user interfaces in Java, layout management becomes crucial. Efficiently arranging components in your graphical user interface (GUI) ensures a seamless user experience. However, many developers encounter common mistakes related to column positioning, leading to disorganized layouts. In this blog post, we'll explore Java layout management, focusing on column positioning to prevent those blunders.
Understanding Java's Layout Managers
Java provides various layout managers, each serving a unique purpose:
- FlowLayout: Organizes components in a left-to-right flow, wrapping them as needed.
- BorderLayout: Divides the container into five sections: North, South, East, West, and Center.
- GridLayout: Arranges components in a grid with equal-sized cells.
- BoxLayout: Aligns components either vertically or horizontally, adhering to their preferred sizes.
These layout managers help prevent clutter and ensure responsive designs. As noted in the existing article, Master Column Positioning: Avoid Common Layout Blunders!, improper usage can lead to confusion and inefficiency in your application.
Why Layout Managers Matter
Layout managers:
- Adapt to Different Screen Sizes: They respond to dynamic resizing, making apps compatible across devices.
- Reduce Manual Positioning: Saves time and minimizes the risk of element overlaps or missed placements.
- Make Code More Readable: Clear component arrangement improves maintainability over time.
Key Considerations for Column Management
When dealing with column-based layouts, here are some considerations to keep in mind:
- Choosing the Right Layout Manager: Understand the purpose of each layout. Selecting a GridLayout for a column-based design is often suitable as it directly relates to how rows and columns are handled.
- Setting Up Constraints: For layouts like GridBagLayout, constraints are vital. They control how components interact with the grid, ensuring fluid positioning.
- Testing for Resizing: Ensure that your layout adapts well to different window sizes. Identify how components shift as the window is resized.
Implementing Column Positioning with GridLayout
Let’s explore a practical example using GridLayout
. This layout simplifies the alignment of components into rows and columns.
Example Code
import javax.swing.*;
import java.awt.*;
public class ColumnLayoutDemo {
public static void main(String[] args) {
JFrame frame = new JFrame("Column Layout Demo");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Setting GridLayout with 3 rows and 2 columns
frame.setLayout(new GridLayout(3, 2));
// Adding components to the frame
frame.add(new JButton("Button 1"));
frame.add(new JButton("Button 2"));
frame.add(new JButton("Button 3"));
frame.add(new JButton("Button 4"));
frame.add(new JButton("Button 5"));
frame.add(new JButton("Button 6"));
// Finalize the frame setup
frame.setSize(400, 300);
frame.setVisible(true);
}
}
Why GridLayout
?
- The
GridLayout
allows for an organized way to display buttons in a grid manner, making it easy for users to interact with them. - It automatically adjusts the button sizes to fill the assigned space, which prevents gaps and misalignment.
Key Points in Layout Management
-
Component Order: In
GridLayout
, the order in which components are added determines their position in columns and rows. This ordering is intuitive and prevents oversight. -
Dynamic Resizing: The layout manager allows for expansions and contractions; thus, you don't need to worry about fixed sizes.
-
Less Manual Adjustment: By using layout managers like
GridLayout
, developers can avoid complicated calculations for component placements.
Advanced Column Management with GridBagLayout
While GridLayout
is excellent for simple arrangements, sometimes the functionality and customization offered by GridBagLayout
is necessary. This layout manager allows for much more control regarding component size and position.
Example Code
import javax.swing.*;
import java.awt.*;
public class AdvancedColumnLayoutDemo {
public static void main(String[] args) {
JFrame frame = new JFrame("Advanced Column Layout Demo");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
// Adding components with constraints
gbc.fill = GridBagConstraints.HORIZONTAL;
gbc.gridx = 0;
gbc.gridy = 0;
gbc.weightx = 0.5;
frame.add(new JButton("Button 1"), gbc);
gbc.gridx = 1;
gbc.gridy = 0;
frame.add(new JButton("Button 2"), gbc);
gbc.gridx = 0;
gbc.gridy = 1;
gbc.gridwidth = 2;
frame.add(new JButton("Button 3 (Spans 2 Columns)"), gbc);
// Finalize the frame setup
frame.setSize(600, 200);
frame.setVisible(true);
}
}
Why GridBagLayout
?
-
Fine-Grain Control: You can specify exactly how much space a component should take up (e.g., using
weightx
to control horizontal filling). -
Complex Layouts: It's suitable for creating more sophisticated layouts where components need varying amounts of space.
Common Pitfalls in Column Management
Even with a solid understanding of layout managers, mistakes can still arise. Here are some common pitfalls to avoid:
Overlooking Default Sizes
If you do not set minimum or preferred sizes for your components, they can end up too small or too large when resized.
Improperly Configured Constraints
In layouts like GridBagLayout
, misconfigured constraints can lead to visual chaos. Always ensure you understand the implications of each setting.
Inconsistent Component Sizes
Mixing different component sizes can result in a visually unappealing layout. Aim for uniformity when necessary.
In Conclusion, Here is What Matters
Effective column management in Java lays the foundation for solid, responsive user interfaces. Utilizing layout managers such as GridLayout
and GridBagLayout
can help prevent common mistakes and create clear, organized designs.
By adhering to the principles of layout management and carefully considering the context in which you're applying columns, you can enhance the user experience significantly. For a deeper dive into column positioning, check out the insights in Master Column Positioning: Avoid Common Layout Blunders!.
With this understanding, you are now better equipped to tackle the complexities of Java layout management and create visually appealing applications. Happy coding!
Checkout our other articles