Mastering Java Layouts: Common Mistakes to Avoid
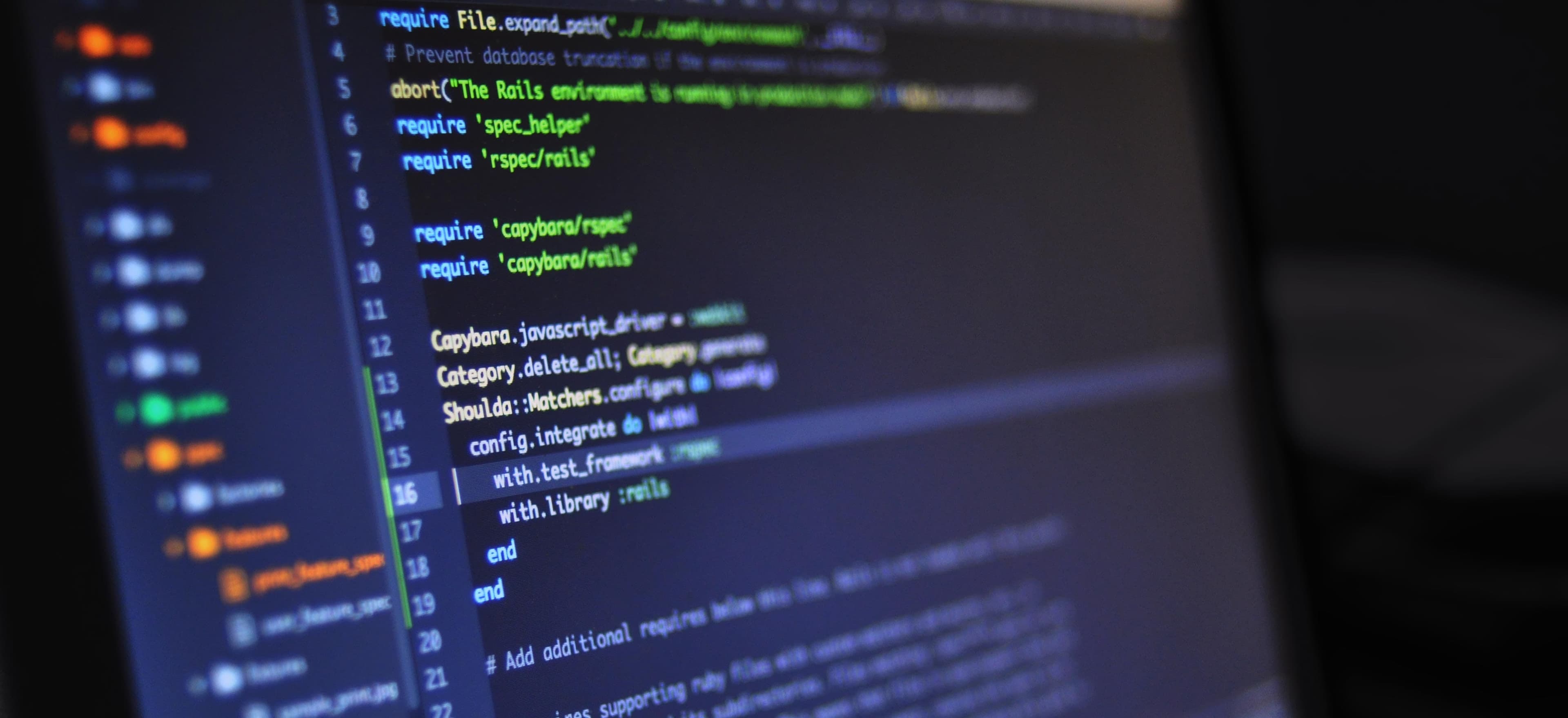
- Published on
Mastering Java Layouts: Common Mistakes to Avoid
Java offers a myriad of powerful tools and frameworks for application development. However, mastering the layouts in a Java graphical user interface (GUI) is paramount for creating effective applications that delight users. Many developers, whether novices or seasoned professionals, find themselves in a common predicament: poor layout decisions that can hinder the user experience. In this post, we'll explore these common mistakes and how to avoid them, while referring to key principles that can enhance your design methodologies.
Understanding the Importance of Layouts
When designing Java GUIs, the layout is the first impression users will have of your application. A good layout:
- Enhances usability
- Supports accessibility
- Creates an aesthetically pleasing environment
To understand the impact a layout can have, think about an article you recently read, titled Master Column Positioning: Avoid Common Layout Blunders!. This article emphasizes the concept of effective positioning in layouts, something we will delve deeper into.
Common Mistakes in Java Layout Design
1. Not Using Layout Managers
One of the most frequent pitfalls for Java developers is neglecting to utilize layout managers. Java provides several built-in layout managers such as FlowLayout
, BorderLayout
, and GridLayout
. Each has its unique behavior.
Example of a FlowLayout:
import javax.swing.*;
import java.awt.*;
public class FlowLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("FlowLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new FlowLayout());
frame.add(new JButton("Button 1"));
frame.add(new JButton("Button 2"));
frame.add(new JButton("Button 3"));
frame.setSize(300, 200);
frame.setVisible(true);
}
}
Here, FlowLayout
arranges components in a sequence, allowing them to wrap to the next line if there's insufficient space. A critical mistake is hardcoding component placements or relying solely on absolute positioning, which restricts flexibility and scalability.
2. Overcomplicating Layouts
While it might be tempting to create complex nested layouts to achieve a desired appearance, this often leads to confusion and increased maintenance costs. Simplicity is a crucial principle in Java layout design.
Tip: Simpler layouts load faster, are easier to understand, and less prone to errors. Always consider whether you can achieve the same result with a simpler approach.
3. Ignoring Resizable Components
A common oversight is not considering how components will adjust to different screen sizes or window resizing. Many GUIs today must accommodate various devices and screen resolutions.
Example of a GridLayout:
import javax.swing.*;
import java.awt.*;
public class GridLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("GridLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new GridLayout(2, 2)); // 2 rows and 2 columns
frame.add(new JButton("Button 1"));
frame.add(new JButton("Button 2"));
frame.add(new JButton("Button 3"));
frame.add(new JButton("Button 4"));
frame.setSize(300, 200);
frame.setVisible(true);
}
}
In this example, GridLayout
organizes components into a grid format, which allows them to resize automatically when the window resizes. Not properly utilizing such layouts can lead to components overlapping or leaving unnecessary spaces.
4. Neglecting the Use of Insets and Gaps
Margins and spacing are critical to creating a clean and professional GUI. Java layout managers often allow you to set insets and gaps, and failing to do so can result in cluttered interfaces.
Example of setting gaps with GridBagLayout:
import javax.swing.*;
import java.awt.*;
public class GridBagLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("GridBagLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.insets = new Insets(10, 10, 10, 10); // Set margins around components
gbc.gridx = 0; // X position
gbc.gridy = 0; // Y position
frame.add(new JButton("Button A"), gbc);
gbc.gridx = 1;
gbc.gridy = 0;
frame.add(new JButton("Button B"), gbc);
frame.setSize(300, 200);
frame.setVisible(true);
}
}
Using GridBagLayout
is beneficial because it allows for precise placements, and with the insets, you can ensure that components are spaced nicely, improving both aesthetics and usability.
5. Lack of Testing Across Platforms
Another significant error is failing to test your GUI layouts across various platforms. Java's Swing framework is cross-platform, which means what looks good on one operating system may not work on another.
Tips for Ensuring Cross-platform Compatibility:
- Test on multiple operating systems: Windows, MacOS, and Linux.
- Utilize tools like JUnit to automate layout testing.
Key Takeaways: Mastering Layouts in Java
Mastering Java layouts is a journey. To avoid common mistakes, always engage with the best practices: leverage layout managers, maintain simplicity, ensure component responsiveness, use proper margins, and rigorously test your interfaces.
For further insights into layout positioning, I highly recommend checking out Master Column Positioning: Avoid Common Layout Blunders!.
By applying these principles and strategies, you can create a far more effective and visually appealing user interface. Remember, a well-structured layout is not just about aesthetics; it’s about enhancing the user experience and making your application a joy to use. Happy coding!
Checkout our other articles