Mastering Memory Management: Avoiding Leaks in Java Web Apps
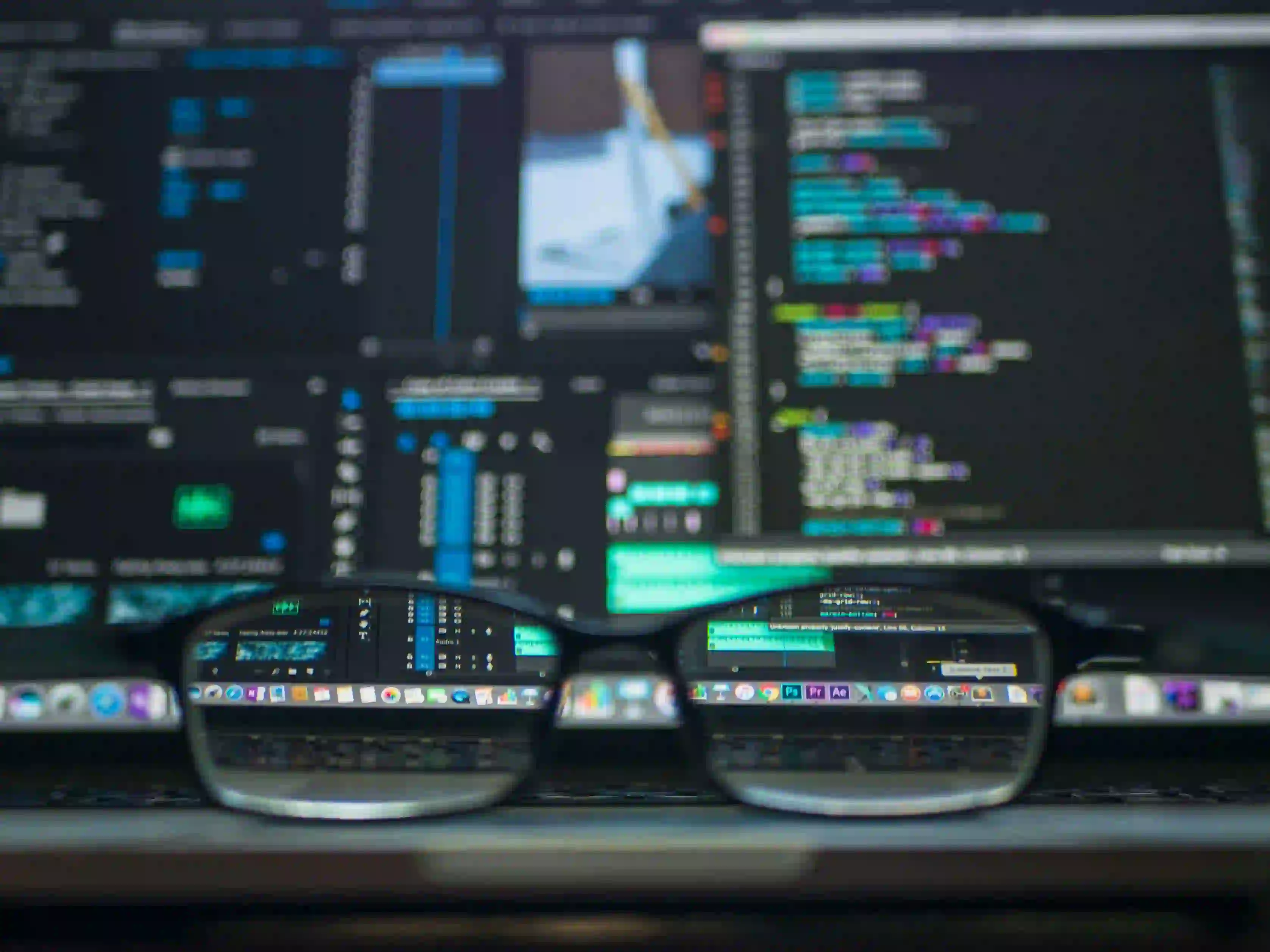
Mastering Memory Management: Avoiding Leaks in Java Web Apps
In the world of Java web applications, efficient memory management is paramount. Memory leaks can lead to performance degradation, application crashes, and user dissatisfaction. In this post, we’ll explore strategies for avoiding memory leaks in Java web applications, particularly Single Page Applications (SPAs).
For our discussion, we will draw some insight from existing resources such as the article 5 Tips to Prevent Memory Leaks in SPAs.
Understanding Memory Leaks in Java
Before we delve into prevention techniques, let’s briefly discuss what memory leaks are. In Java, memory is managed by the garbage collector, which automatically reclaims memory that is no longer in use. However, there are situations where memory is still referenced but has become unnecessary — these situations lead to memory leaks.
A classic example of a memory leak is when a developer creates static collections or listeners without appropriate cleanup. Over time, unnecessary objects linger, consuming valuable resources and potentially slowing down or crashing an application.
Strategies to Prevent Memory Leaks
Here are several strategies to prevent memory leaks in Java web applications:
1. Use Weak References
Weak references are a crucial part of Java memory management, especially for caches and listener implementations.
import java.lang.ref.WeakReference;
import java.util.HashMap;
public class Cache {
private HashMap<String, WeakReference<Object>> cache = new HashMap<>();
public void put(String key, Object value) {
cache.put(key, new WeakReference<>(value));
}
public Object get(String key) {
WeakReference<Object> ref = cache.get(key);
return ref != null ? ref.get() : null;
}
}
Why this works: By using WeakReference
, the garbage collector is allowed to reclaim memory used by key objects when they are no longer in strong reference. This ensures that you can manage memory more effectively, preventing full memory use.
2. Removing Listeners Appropriately
In Java, it is common to attach listeners to events. However, if these listeners are not removed when they are no longer needed, they can prevent garbage collection of the objects they reference.
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
public class ButtonExample {
private JButton button;
public ButtonExample() {
button = new JButton("Click me");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.out.println("Button clicked");
}
});
}
public void removeListener() {
for (ActionListener listener : button.getActionListeners()) {
button.removeActionListener(listener);
}
}
}
Why this works: The removeListener
method ensures that when you're done using the button, all attached listeners are removed, breaking any unwanted references.
3. Scope Limitation Inside Anonymous Classes
Anonymous classes used inside methods can hold the enclosing class's instance as a reference. If not correctly managed, this can lead to memory leaks.
public class EnclosingClass {
public void method() {
Button button = new Button();
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
// Accessing method from enclosing class
someMethod();
}
});
}
}
Why this is problematic: The anonymous class holds a reference to EnclosingClass
, meaning that it can’t be garbage collected until the listener is removed. Instead, consider using a static inner class or external class to avoid maintaining that reference.
4. Avoiding Static Collections
Static fields can unintentionally hold references to objects. It is wise to avoid uncontrolled use of static collections.
import java.util.ArrayList;
import java.util.List;
public class EventBus {
private static List<Listener> listeners = new ArrayList<>();
public static void register(Listener listener) {
listeners.add(listener);
}
public static void deregister(Listener listener) {
listeners.remove(listener);
}
}
Why this is risky: If you don’t deregister listeners when they are no longer needed, you introduce a scenario where the objects remain in memory indefinitely. Instead, consider instance-based collections.
5. Utilizing Profiling Tools
Java provides powerful profiling tools that help identify memory leaks before they become problematic. Tools like VisualVM, YourKit, or Eclipse Memory Analyzer can give insights into memory usage patterns.
- VisualVM: Monitor resource consumption of our Java applications.
- Eclipse Memory Analyzer: Provides a comprehensive view of memory snapshots and can identify memory leaks.
To Wrap Things Up
To sum it up, effectively managing memory in Java web applications is crucial. Implementing the strategies highlighted above will contribute significantly to reducing the risks of memory leaks, thereby enhancing application performance.
For those interested in building robust web applications, understanding and controlling memory usage are as important as creating efficient algorithms. Make recycling memory a part of your development repertoire.
For more strategies focused on SPAs specifically, be sure to check the article 5 Tips to Prevent Memory Leaks in SPAs. By applying these concepts, you can ensure your Java applications remain efficient, stable, and ready to serve your users effectively.