Preventing Memory Leaks in Java Web Applications
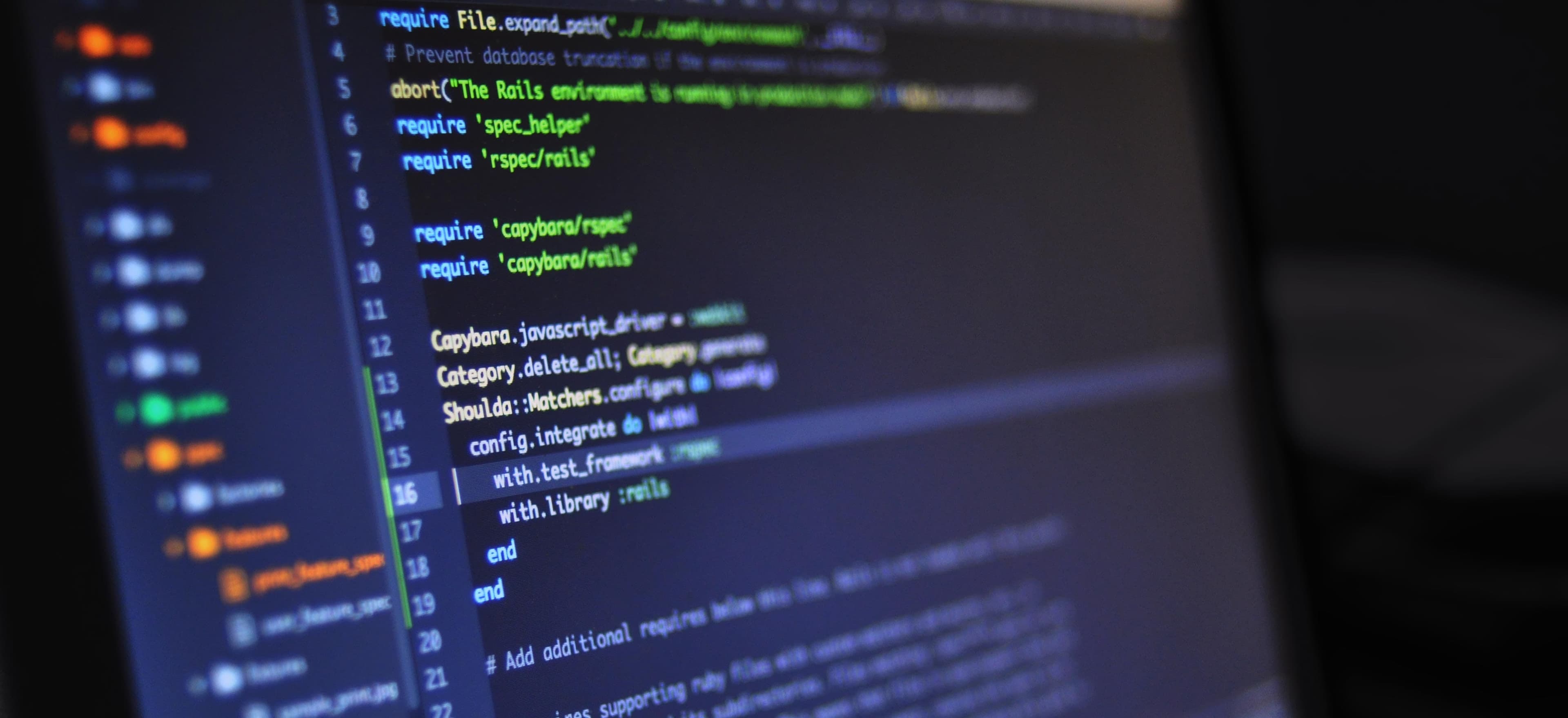
- Published on
Preventing Memory Leaks in Java Web Applications
In the era of responsive web development, ensuring that Java web applications run smoothly is paramount. One of the most pressing issues developers face is memory leaks. These leaks slow down application performance, lead to unresponsive interfaces, and increase server costs when scaling up. In this blog post, we'll embark on a journey to explore the causes of memory leaks in Java web applications and effective techniques to prevent them.
Understanding Memory Leaks
Before we dive into solutions, let's define what a memory leak is. A memory leak occurs when your application allocates memory but fails to release it after use. This causes the application to slowly but surely consume more memory until the system resources are depleted.
In Java, the Garbage Collector (GC) usually handles memory management by cleaning up unused objects. However, poorly managed references can result in memory leaks, especially in complex web applications.
Identifying Causes of Memory Leaks
Understanding the most common culprits will help you prevent memory leaks effectively:
- Long-lived References: Static collections or class loaders holding onto large objects.
- Event Listeners: Failing to deregister listeners that keep object references alive.
- Thread Pools: Unmanaged threads that capture too much data over their lifecycle.
- Poorly Scoped Resources: Resources that remain in memory longer than they should.
By identifying these issues, developers can take proactive steps toward resolution.
Best Practices to Prevent Memory Leaks
1. Use Weak References
Java provides a way to avoid memory leaks via weak references. When you use a WeakReference
, the Java Garbage Collector can reclaim the referenced object when memory is low, even if there are still references to it.
Here’s how to implement it:
import java.lang.ref.WeakReference;
import java.util.HashMap;
import java.util.Map;
class Cache {
private Map<String, WeakReference<Object>> cache = new HashMap<>();
public void put(String key, Object value) {
cache.put(key, new WeakReference<>(value));
}
public Object get(String key) {
WeakReference<Object> ref = cache.get(key);
return ref != null ? ref.get() : null;
}
}
Why Use Weak References?
Using WeakReference
allows the garbage collector the flexibility to remove objects that are not in use, thus freeing up memory while maintaining cache functionality.
2. Deregister Event Listeners
Event listeners can commonly lead to memory leaks if they are not deregistered properly. For example, if a UI component listens to a data model but fails to remove itself when the component is destroyed, the component will not be garbage collected.
Here's an example:
class ViewModel {
private List<DataUpdateListener> listeners = new ArrayList<>();
public void addListener(DataUpdateListener listener) {
listeners.add(listener);
}
public void removeListener(DataUpdateListener listener) {
listeners.remove(listener);
}
public void notifyListeners() {
for (DataUpdateListener listener : listeners) {
listener.update();
}
}
}
Why Deregister Listeners?
Ensuring that listeners are deregistered when no longer needed prevents dangling references that can keep large objects alive, which, in turn, helps free up memory.
3. Manage Thread Pools Carefully
Thread pools can lead to memory leaks if they're not managed properly. If threads in a pool hold onto large data sets or objects longer than necessary, it can consume excessive memory.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
private ExecutorService executor = Executors.newFixedThreadPool(10);
public void executeTask(Runnable task) {
executor.execute(task);
}
public void shutdown() {
executor.shutdown();
}
}
Why Keep Threads in Check?
Managing thread pools allows you to control resource allocation effectively, reducing the risk of memory leaks caused by long-running references.
4. Limit Scope of Variables
Objects declared outside of a method's scope could remain in memory longer than necessary. Scoping your variables properly ensures that they are eligible for garbage collection once they go out of scope.
public void process() {
String data = fetchData(); // Limited to this method's scope
System.out.println(data);
}
Why Scoping Matters?
Keeping the variable's lifecycle limited aids the garbage collector, allowing it to faithfully reclaim memory when it is no longer used.
5. Utilize Profiling Tools
Tools such as Java VisualVM or Eclipse Memory Analyzer can help you analyze object allocation and identify memory leaks in real-time. Regularly using these tools helps you stay proactive.
Why Profile?
Profiling provides insights into your application’s memory usage, helping you catch leaks before they become problematic.
Additional Resources
For further reading on memory management techniques applicable to single-page applications (SPAs), be sure to check out the article on 5 Tips to Prevent Memory Leaks in SPAs. It provides additional strategies that can complement what we discussed here.
My Closing Thoughts on the Matter
Memory leaks can be insidious, but with careful design and a proactive approach to managing references and resources, developers can mitigate these issues in Java web applications. By adopting practices such as using weak references, deregistering event listeners, managing thread lifecycles, and limiting variable scope, developers not only enhance application performance but also improve reliability.
Remember, the key to preventing memory leaks is vigilance; regularly review and utilize profiling tools to safeguard against potential pitfalls. Happy coding!
Checkout our other articles