Avoiding Common Java Pitfalls When Connecting to PostgreSQL
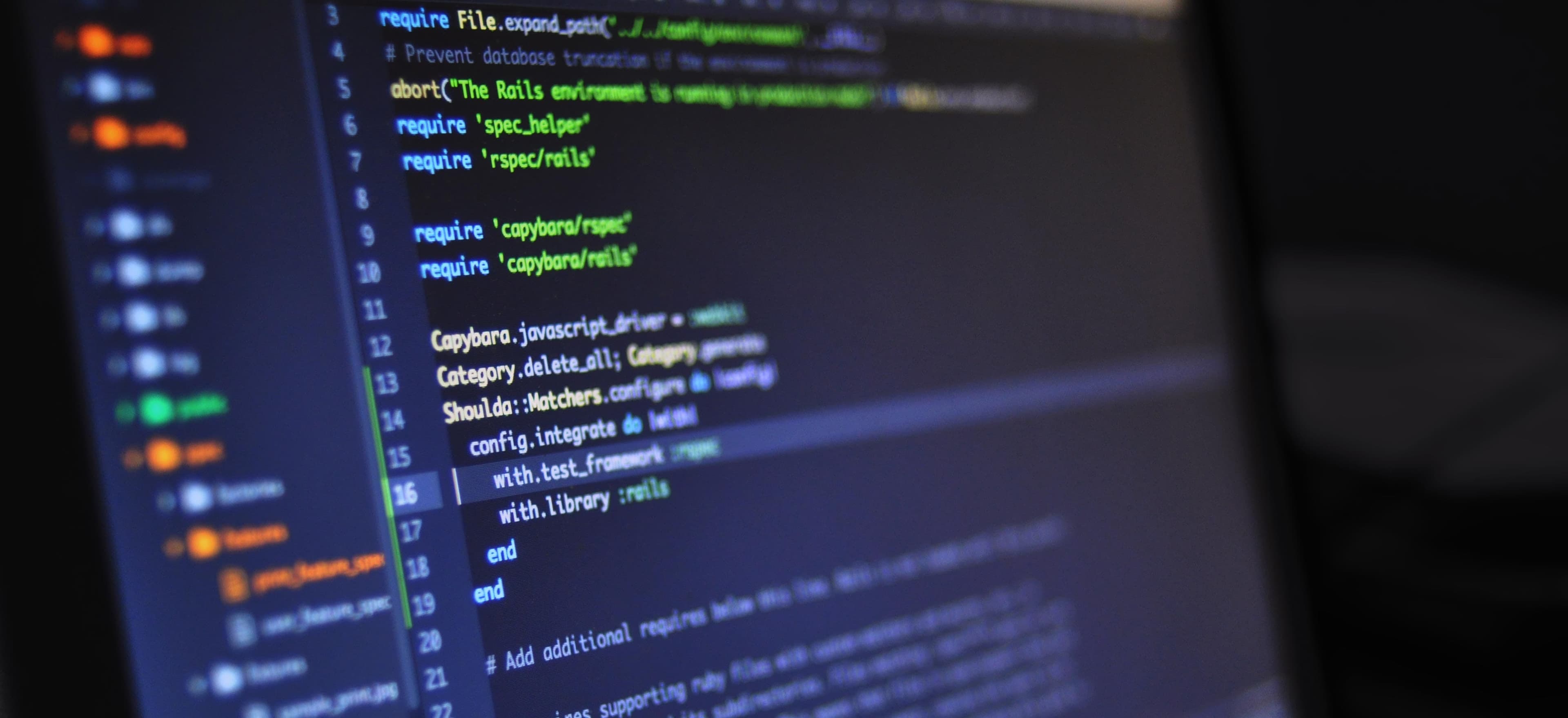
- Published on
Avoiding Common Java Pitfalls When Connecting to PostgreSQL
Connecting a Java application to a PostgreSQL database can seem daunting at first. However, with the right knowledge and best practices, you can establish a seamless connection without running into common pitfalls. This article aims to guide you through the essentials of connecting Java to PostgreSQL, and it will highlight some common pitfalls you should watch out for.
Table of Contents
- Prerequisites
- Setting Up PostgreSQL
- Maven Dependencies
- Basic Connection Example
- Common Pitfalls
- Conclusion
Prerequisites
Before you dive into the code, ensure you have the following prerequisites in place:
- Java Development Kit (JDK) installed (version 8 or higher).
- PostgreSQL installed and configured on your machine.
- Basic understanding of Java and SQL.
Setting Up PostgreSQL
If you have not set up PostgreSQL yet, here is how you can do it:
-
Download and Install PostgreSQL: Visit the official PostgreSQL website and follow the installation instructions for your operating system.
-
Create a Database: Open your command line (Terminal or Command Prompt) and enter the PostgreSQL shell using the following command:
psql -U postgres
To create a new database, execute:
CREATE DATABASE mydatabase;
-
Create a User: You will also need a user to connect to your database. Run the following command in the PostgreSQL shell:
CREATE USER myuser WITH PASSWORD 'mypassword';
-
Grant Access: Grant the user access to the previously created database:
GRANT ALL PRIVILEGES ON DATABASE mydatabase TO myuser;
Maven Dependencies
To connect your Java application to PostgreSQL, you'll need the PostgreSQL JDBC driver. If you are using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.3.1</version> <!-- Check for the latest version -->
</dependency>
This dependency ensures that your project has access to the required JDBC classes to establish the connection.
Basic Connection Example
Once you have set up PostgreSQL and Maven, you can write your Java code to connect to the database. Here’s a basic example:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class PostgresConnection {
private static final String URL = "jdbc:postgresql://localhost:5432/mydatabase";
private static final String USER = "myuser";
private static final String PASSWORD = "mypassword";
public static Connection getConnection() {
Connection conn = null;
try {
conn = DriverManager.getConnection(URL, USER, PASSWORD);
System.out.println("Connection established successfully.");
} catch (SQLException e) {
e.printStackTrace();
System.out.println("Connection failed.");
}
return conn;
}
public static void main(String[] args) {
getConnection();
}
}
Commentary on the Code
-
DriverManager: The
DriverManager.getConnection()
method establishes the connection using your database URL, username, and password. It's essential to catchSQLException
to handle any errors that might occur during the connection phase. -
Error Handling: Proper error handling is crucial. It’s a best practice to log or print stack traces for debugging purposes; ignore this, and you may find troubleshooting quite challenging.
-
Closing the Connection: It's vital to close the connection once you are done. You can do this by implementing a try-with-resources statement or closing the connection in a finally block.
Common Pitfalls
While establishing a connection is relatively straightforward, several common pitfalls can trip you up.
1. Incorrect JDBC URL
The JDBC URL format for PostgreSQL is:
jdbc:postgresql://host:port/database
Make sure to replace host
, port
, and database
with your parameters. Using an incorrect URL will throw an exception. For a detailed look at connection issues, take a glance at Common Pitfalls When Connecting Postgres to Express.
2. Driver Class Not Found Exception
Java requires the PostgreSQL JDBC driver to be on your classpath. If Maven dependencies are not set up correctly, or you forget to include the JAR in the build path, you will encounter a ClassNotFoundException
.
3. Firewall and Network Issues
If your PostgreSQL server is hosted remotely, ensure that your server firewall allows access on the PostgreSQL port (default is 5432). If not opened, your application won't be able to connect.
4. Connection Pooling
Establishing a new database connection for each request can lead to performance bottlenecks. It’s advisable to use a connection pool (e.g., HikariCP) to manage connections efficiently. Here’s a basic usage example:
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>5.0.1</version> <!-- Check for the latest version -->
</dependency>
Example of using HikariCP:
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
import java.sql.Connection;
import java.sql.SQLException;
public class HikariCPExample {
private static HikariDataSource dataSource;
static {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:postgresql://localhost:5432/mydatabase");
config.setUsername("myuser");
config.setPassword("mypassword");
dataSource = new HikariDataSource(config);
}
public static Connection getConnection() throws SQLException {
return dataSource.getConnection();
}
public static void main(String[] args) {
try (Connection connection = getConnection()) {
System.out.println("Connection from HikariCP established successfully.");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
5. Handling Exceptions
Failing to handle exceptions properly can leave your application in an unpredictable state. Always ensure you are catching and logging exceptions. This will help you pinpoint issues quickly.
Closing Remarks
Connecting your Java application to a PostgreSQL database does not have to be a cumbersome task. By following the outlined steps and avoiding the common pitfalls mentioned above, you can build robust and efficient database connections. Ensure you adhere to best practices like connection pooling and proper error handling for the best results.
For further reading on issues that can arise in database connections, refer back to Common Pitfalls When Connecting Postgres to Express.
By keeping these best practices in mind, you can focus on developing your application instead of dealing with troublesome database connections. Happy coding!