Mastering Java Frameworks: Overcoming IE Media Query Issues
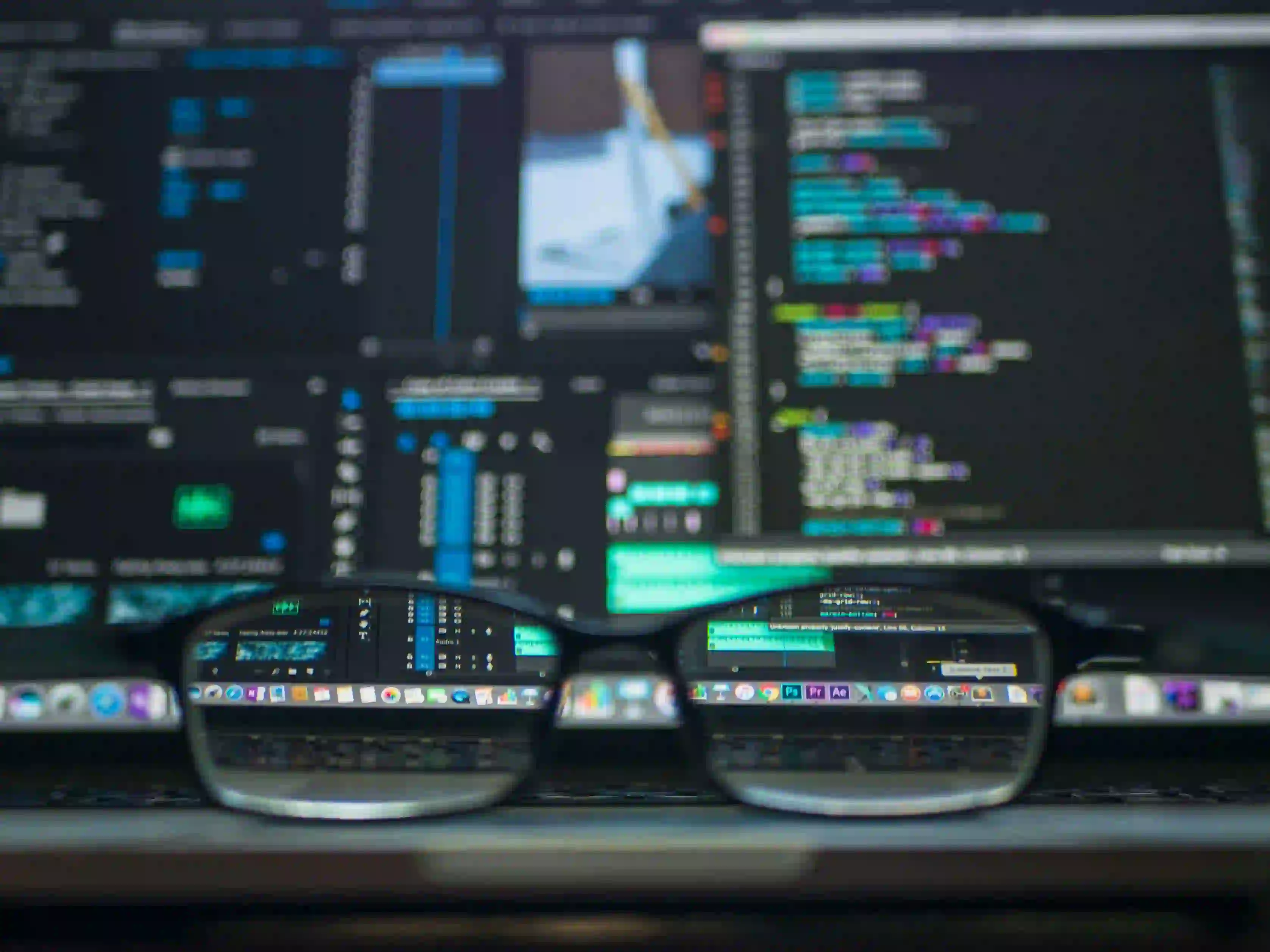
Mastering Java Frameworks: Overcoming IE Media Query Issues
When developing modern web applications, compatibility across different browsers—including Internet Explorer (IE)—can be quite challenging. This is especially true when dealing with CSS for responsive design, particularly media queries. While Java developers primarily focus on backend logic, front-end issues, like CSS compatibility, can still affect the overall user experience. In this article, we'll delve into how Java frameworks can assist in addressing these issues, especially in the context of problematic media queries in Internet Explorer.
Understanding Media Queries
Media queries are a key feature of CSS that enable responsive web designs. They allow developers to apply different styles based on device characteristics, such as screen size, orientation, or resolution. For instance, you may want a single-column layout on small screens but switch to a multi-column layout on larger displays.
A simple media query example looks like this:
@media (max-width: 600px) {
body {
background-color: lightblue;
}
}
In this case, the styling will only apply when the viewport width is 600 pixels or less.
Issues with Internet Explorer
While media queries are widely supported in most modern browsers, older versions of Internet Explorer (especially IE 8 and below) struggle with them. This can lead to inconsistencies in rendering, making your applications look formatted poorly across platforms.
For a deeper dive into resolving CSS issues related to media queries in IE, please refer to the article "How to Make Media Queries Work in Internet Explorer".
Java Frameworks to the Rescue
Java frameworks like Spring MVC, Vaadin, and JavaServer Faces (JSF) can help create a structured and elegant approach to handling web-compatible designs. However, they don't inherently resolve front-end issues. Nevertheless, here’s how you can effectively bridge the gap.
Using Spring MVC with Responsive Design
Spring MVC (Model-View-Controller) is an intelligent and popular framework for building web applications in Java.
-
Dynamic Content Serving: You can use Spring MVC to detect the client’s user agent string on the server side and serve different stylesheets based on the browser type.
Here's an example of how you can set responsive links in a Spring MVC controller:
☕snippet.java@Controller public class HomeController { @RequestMapping("/") public String home(HttpServletRequest request, Model model) { String userAgent = request.getHeader("User-Agent").toLowerCase(); if (userAgent.contains("msie") || userAgent.contains("trident")) { model.addAttribute("stylesheet", "ie-styles.css"); } else { model.addAttribute("stylesheet", "styles.css"); } return "home"; } }
In this code snippet, we are checking the User-Agent string to determine if the request comes from Internet Explorer. According to the result, we serve either the IE-specific stylesheet or the regular one.
Develop a Strategy for Stylesheets
To effectively manage responsiveness across browsers, follow best practices for structuring your CSS and JavaScript.
-
Conditional Comments: For older IE versions, you can use conditional comments to load specific stylesheets.
📄snippet.txt<!--[if lt IE 9]> <link rel="stylesheet" type="text/css" href="ie-style.css" /> <![endif]-->
-
Polyfills: To add modern CSS features in older browsers, consider using Respond.js or css3-mediaqueries.js.
Example of a Java Application Using Vaadin
Vaadin is a Java framework that offers a simplified UI framework integrated with responsive CSS. When working with Vaadin, modern web architectures allow for more intuitive design practices versus traditional Java applications.
Here's a simple layout example using Vaadin that adjusts according to the screen size:
@Route("")
public class MainView extends VerticalLayout {
public MainView() {
add(new H1("Welcome to the Responsive App"));
setHorizontalComponentAlignment(Alignment.CENTER);
// Adjust layout based on screen size
ResponsiveLayout resLayout = new ResponsiveLayout();
resLayout.addComponent(createResponsiveCard("Card 1"));
resLayout.addComponent(createResponsiveCard("Card 2"));
add(resLayout);
}
private Component createResponsiveCard(String title) {
Card card = new Card(title);
card.addClassName("responsive-card");
return card;
}
}
Building the CSS for Vaadin
You will largely build your CSS using classes that will provide specific styles for different browsers. Here’s a sample:
/* Normal styling */
.responsive-card {
width: 200px;
margin: 10px;
}
/* Styles for maximum width screens (like mobile) */
@media (max-width: 600px) {
.responsive-card {
width: 100%;
}
}
/* Styles specifically for IE */
@media all and (-ms-high-contrast: none), (-ms-high-contrast: active) {
.responsive-card {
width: 100%;
background: #f0f0f0; /* Example: special IE styling */
}
}
The last media query here targets Internet Explorer specifically, allowing you to provide alternative styling to make your layouts more compatible.
Debugging and Testing
Testing and debugging become critical when it comes to ensuring a smooth user experience across platforms. Tools like:
- BrowserStack: For comprehensive cross-browser testing.
- IE Tab: A Chrome extension to run websites in IE.
Utilizing these tools will make it easier to pinpoint any issues that may occur in IE related to media queries.
A Final Look
In conclusion, mastering Java frameworks can significantly improve your development process, especially when addressing the inconsistencies seen in Internet Explorer's handling of media queries.
Realizing that responsive design is not just a front-end task is vital. Leveraging the power of server-side frameworks such as Spring MVC and Vaadin, you can streamline your development process to accommodate differences across browsers effectively.
For further insights on handling responsive design challenges with CSS in Internet Explorer, don’t forget to check out the detailed guide here: How to Make Media Queries Work in Internet Explorer.
Remember, while you cannot control what browsers your users employ, you can control how your applications react to those environments. Stay ahead by mastering these frameworks to enhance user experience and design fluidity across your Java applications.