Avoiding Java Pitfalls When Connecting Postgres with Spring
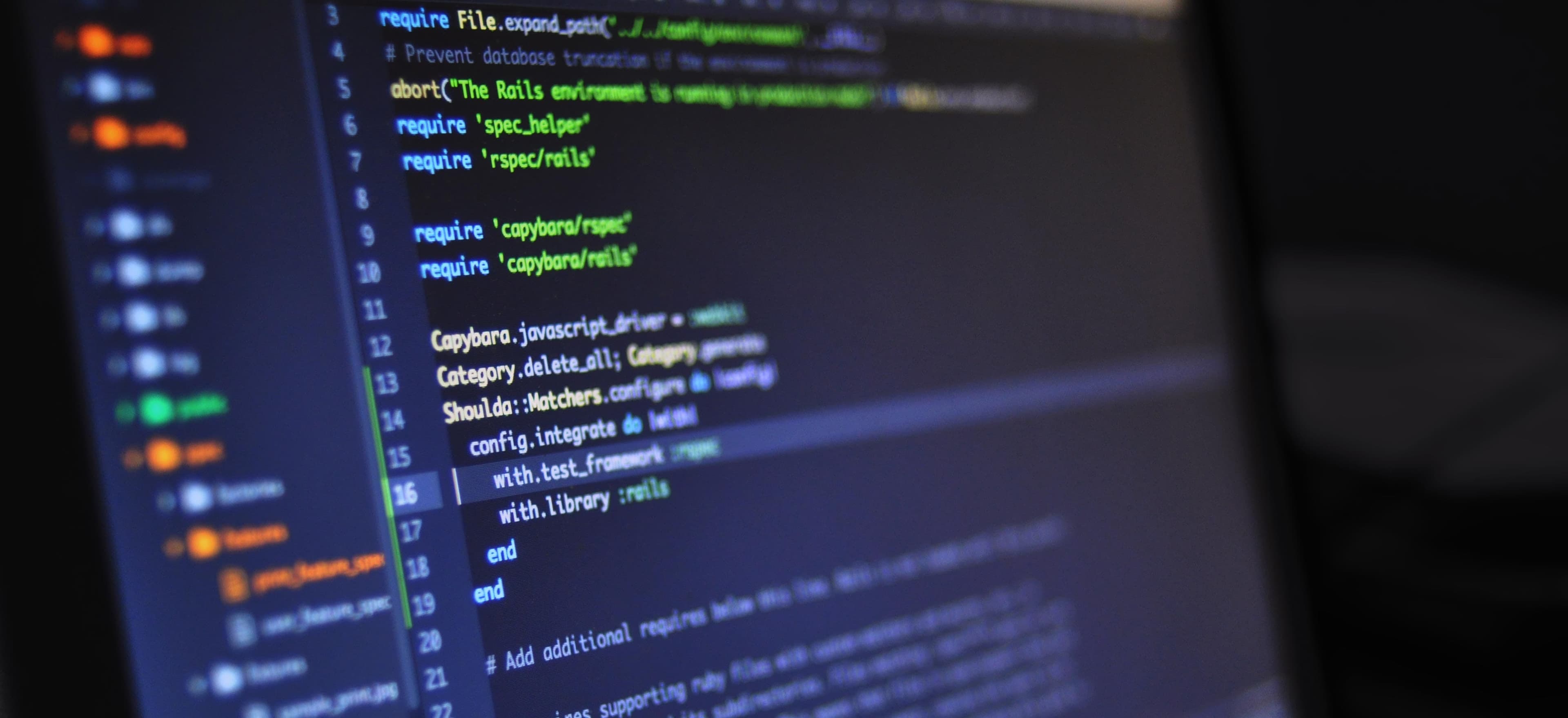
- Published on
Avoiding Java Pitfalls When Connecting Postgres with Spring
Connecting a PostgreSQL database to a Spring application can create powerful and robust web applications. However, developers often encounter various hurdles and pitfalls during this integration. In this post, we will explore common pitfalls and how to avoid them, ensuring a smoother development process. This blog is designed to not only help you avoid these issues but also provide best practices and code snippets for a more resilient application.
Why Use Spring for PostgreSQL?
Spring is a versatile framework that allows the development of Java applications with ease. It provides a comprehensive suite of features for building enterprise-grade applications, including seamless database connectivity. By using Spring, you can:
- Implement many architectural patterns, such as MVC.
- Utilize Dependency Injection for better code management.
- Take advantage of Spring Data JPA, which simplifies data access.
Understanding the Connection Process
Before diving deeper, let's outline the basic steps to connect a PostgreSQL database using Spring. Here’s a simplified overview:
- Add Dependencies: Include required dependencies in your
pom.xml
. - Configure Data Source: Set up the database configurations in
application.properties
. - Create Entity Classes: Design your entity classes corresponding to database tables.
- Create a Repository: Use Spring Data JPA to interact with the database.
- Inject the Repository: Integrate it into your service layer.
Common Pitfalls and How to Avoid Them
1. Dependency Mismanagement
One of the most common pitfalls developers face is incorrect or missing dependencies. A common dependency setup for PostgreSQL and Spring Data JPA in your pom.xml
is as follows:
<dependencies>
<!-- Spring Boot Starter Data JPA -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- PostgreSQL Driver -->
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.2.19</version>
</dependency>
</dependencies>
Make sure to keep your dependencies updated and compatible with the latest Spring version. Maven Repository is a good resource to check for updates.
2. Configuration Errors
You might think that configuring the database is straightforward. However, simple mistakes can lead to connection failures. Your configuration in application.properties
should look something like this:
spring.datasource.url=jdbc:postgresql://localhost:5432/mydb
spring.datasource.username=myuser
spring.datasource.password=mypassword
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
What to watch for:
- Ensure the URL points to the correct database.
- Double-check username and password.
- Specify the correct Hibernate DDL setting to control the schema generation. Using
update
can be convenient, but may not be suitable for production environments.
3. Not Using Connection Pooling
A common oversight is to not implement connection pooling, which is crucial for performance. By default, Spring uses HikariCP for connection pooling. This pool helps manage database connections effectively, reducing the overhead of creating connections on every request. You can customize it in application.properties
:
spring.datasource.hikari.minimum-idle=5
spring.datasource.hikari.maximum-pool-size=10
spring.datasource.hikari.idle-timeout=30000
By configuring these properties, you ensure your application utilizes resources efficiently.
4. Ignoring Exception Management
Errors are inevitable, and not handling them gracefully can crash your application. Rather than allowing unhandled exceptions to propagate, make use of a global exception handler:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(DataIntegrityViolationException.class)
public ResponseEntity<String> handleDatabaseError(DataIntegrityViolationException e) {
return new ResponseEntity<>("Database Error: " + e.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleGlobalError(Exception e) {
return new ResponseEntity<>("An unexpected error occurred: " + e.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
This class captures exceptions thrown during database operations and returns relevant information to the client while preventing application crashes.
5. Annotations Misuse
Correctly using JPA annotations is critical. Misconfigurations can lead to inefficiencies. A proper entity class example is:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@Column(nullable = false, unique = true)
private String email;
// Getters and Setters
}
Why this matters: Properly defining entity properties ensures that JPA generates the correct schema. Make sure to leverage annotations like @Column
to enforce constraints.
6. Not Leveraging Spring Data JPA Features
Spring Data JPA offers various features to simplify your data access logic. For example, creating a repository is straightforward:
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findByEmail(String email);
}
By extending JpaRepository
, you gain access to built-in methods like save()
, delete()
, and findAll()
, allowing for quick data access operations.
7. Ignoring Transaction Management
Transactional issues can lead to data inconsistency. Always annotate service methods that change data with @Transactional
:
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional
public User registerUser(User user) {
return userRepository.save(user);
}
}
By managing transactions, you ensure atomic operations, meaning either all changes are applied, or none are.
Wrapping Up
Connecting PostgreSQL with Spring presents some obstacles but can be mastered with the right knowledge. Ensure your dependencies and configurations are accurate, implement error and transaction management, and leverage Spring Data JPA to handle your database interactions efficiently.
For further insights into database connection issues, particularly when using Express, check out this article on Common Pitfalls When Connecting Postgres to Express.
Resources for Further Learning
By following these best practices and avoiding the pitfalls outlined above, you can ensure a stable and efficient connection between your Spring application and PostgreSQL database. Happy coding!
Checkout our other articles