Avoiding Java Pitfalls When Integrating Postgres with Spring Boot
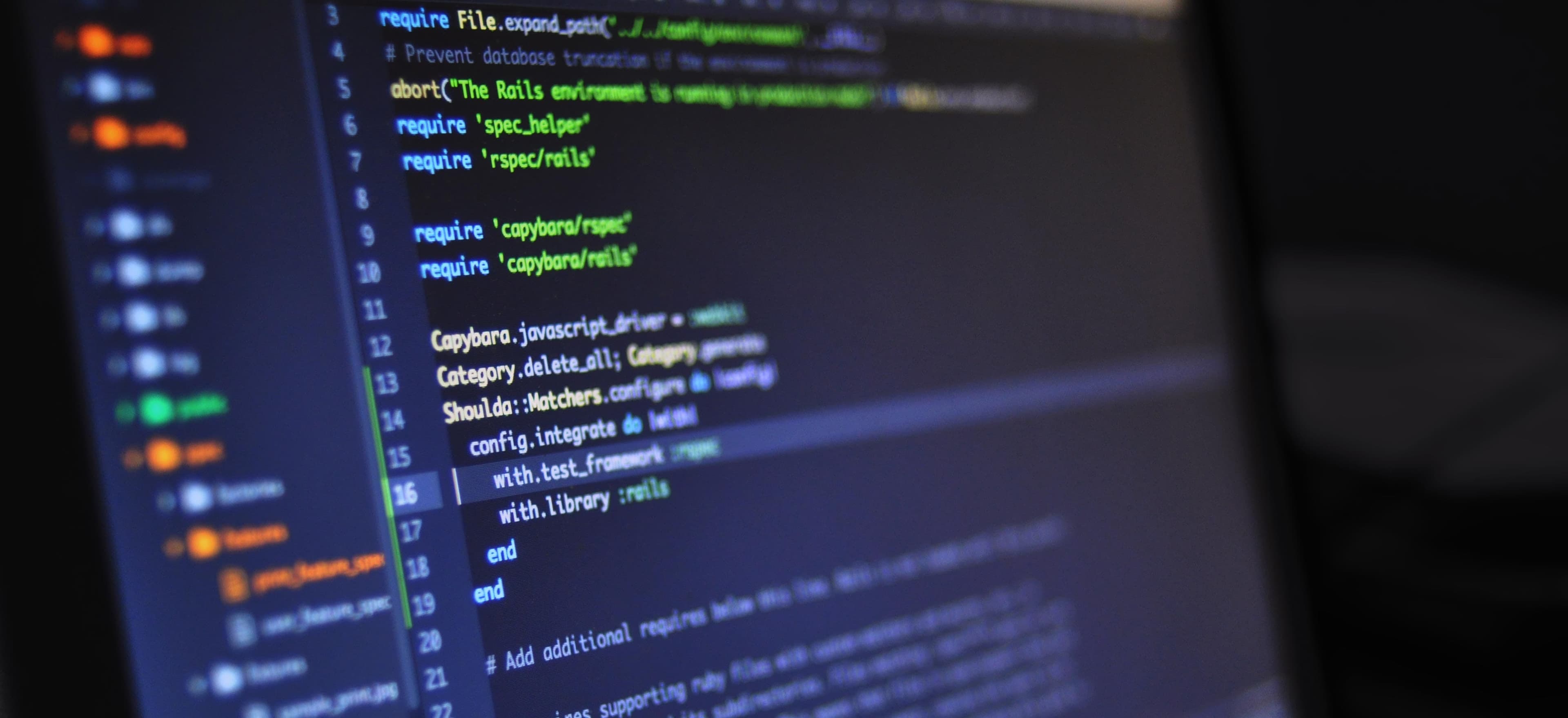
- Published on
Avoiding Java Pitfalls When Integrating Postgres with Spring Boot
Integrating a PostgreSQL database with a Spring Boot application can be both rewarding and challenging. While Spring Boot provides a powerful framework for building Java applications, certain pitfalls can impede the development process. In this blog post, we'll explore these common issues and provide tips on how to avoid them.
Table of Contents
- Understanding PostgreSQL and Spring Boot
- Common Pitfalls
- Misconfigurations
- Lack of Dependency Management
- Inefficient Querying
- Best Practices
- Conclusions and Further Reading
Understanding PostgreSQL and Spring Boot
Spring Boot is an extension of the Spring framework that simplifies the setup and development of new Spring applications. It is particularly well-suited for building web applications and microservices. Meanwhile, PostgreSQL is an advanced, open-source relational database that offers a rich set of features and supports various data types.
By leveraging Spring Boot with PostgreSQL, developers can create robust applications that handle data efficiently. However, as you'll discover in this article, careful handling and understanding of both technologies are essential to avoid common pitfalls.
Common Pitfalls
1. Misconfigurations
One of the first hurdles in connecting PostgreSQL with Spring Boot is misconfiguration of the application properties. If the database URL or credentials are incorrect, your application will be unable to connect to the database.
Example:
Imagine you have the following Spring Boot application properties:
spring.datasource.url=jdbc:postgresql://localhost:5432/mydb
spring.datasource.username=myuser
spring.datasource.password=mypassword
Why it's important: Each part of this configuration needs to be correct. If your username or password is invalid, you'll encounter authentication errors when trying to connect.
To avoid misconfigurations:
- Ensure that the PostgreSQL server is running and accessible.
- Verify that the requested database (mydb) exists.
- Confirm that the username and password have the appropriate permissions.
2. Lack of Dependency Management
Using a Spring Boot application means you can take advantage of its built-in dependency management. However, failing to include the right dependencies for PostgreSQL can result in runtime failures.
Example:
Make sure your pom.xml
(for Maven users) includes the PostgreSQL dependency:
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.2.20</version>
</dependency>
Why it's important: This dependency is crucial for your application to communicate with PostgreSQL. Not including it will lead to ClassNotFoundException
for the PostgreSQL driver when your application starts.
3. Inefficient Querying
Inefficient SQL queries are another common pitfall that can lead to performance degradation. Writing unoptimized queries can result in slow performance, especially when dealing with large datasets.
Example:
Using raw SQL queries can sometimes lead to inefficiency:
@Query("SELECT * FROM users WHERE isActive = true")
List<User> findActiveUsers();
Why it's important: While this query works, if your users table is large, fetching all active users without proper indexing might take a long time.
To improve efficiency:
- Use pagination: Annotations like
@PageableDefault
can help manage large datasets. - Index fields: Make sure that fields referenced in your queries have appropriate indexes.
Best Practices
1. Use Spring Data JPA
Spring Data JPA streamlines the process of interacting with databases, which reduces the boilerplate code significantly. Using Spring Data JPA also allows you to define methods in your repository interface without writing the implementation.
Example:
Here's a sample repository interface:
public interface UserRepository extends JpaRepository<User, Long> {
List<User> findByIsActive(Boolean isActive);
}
Why it's important: This allows you to leverage predefined methods provided by JPA, such as finding users by active status, without needing to write explicit SQL queries.
2. Implement Exception Handling
Exception handling will help identify and manage errors efficiently. Spring provides the @ControllerAdvice
annotation, allowing you to centralize exception handling code.
Example:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(DataAccessException.class)
public ResponseEntity<String> handleDatabaseErrors(DataAccessException ex) {
return new ResponseEntity<>("Database error occurred: " + ex.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Why it's important: By catching specific exceptions related to database access, you can provide clear and user-friendly error messages.
3. Connection Pooling
Using a database connection pooling library such as HikariCP can significantly improve the performance of your application. Connection pools hold a pool of connections that can be reused, enhancing efficiency.
Example in application.properties:
spring.datasource.hikari.maximumPoolSize=10
spring.datasource.hikari.minimumIdle=5
Why it's important: Limiting the number of connections prevents the database from being overwhelmed while allowing for efficient use of resources.
4. Regular Maintenance and Testing
Regular maintenance checks, such as updating dependencies and conducting performance testing, are critical. Additionally, make sure to write unit tests that specifically check the database interactions.
Example:
Here's a simple unit test that checks if a user can be saved in the database.
@Test
public void testSaveUser() {
User user = new User();
user.setName("John Doe");
user.setIsActive(true);
User savedUser = userRepository.save(user);
assertNotNull(savedUser.getId());
assertEquals("John Doe", savedUser.getName());
}
Why it's important: Regular testing ensures that your database interactions behave as expected and helps catch issues early.
In Conclusion, Here is What Matterss and Further Reading
Integrating PostgreSQL with Spring Boot can be a straightforward process if you understand the common pitfalls and implement appropriate solutions. Always remember to double-check your configurations, manage your dependencies carefully, and follow best practices for querying and exception handling.
For those who are looking to learn more about common pitfalls in connecting databases with frameworks like Express, consider reading the article titled Common Pitfalls When Connecting Postgres to Express.
Having a robust application not only improves performance but also enhances the user experience significantly. By implementing these recommendations, you can ensure a smoother integration process and create a more resilient application. Happy coding!