Integrating Java Backend with React Native Barcode Widget
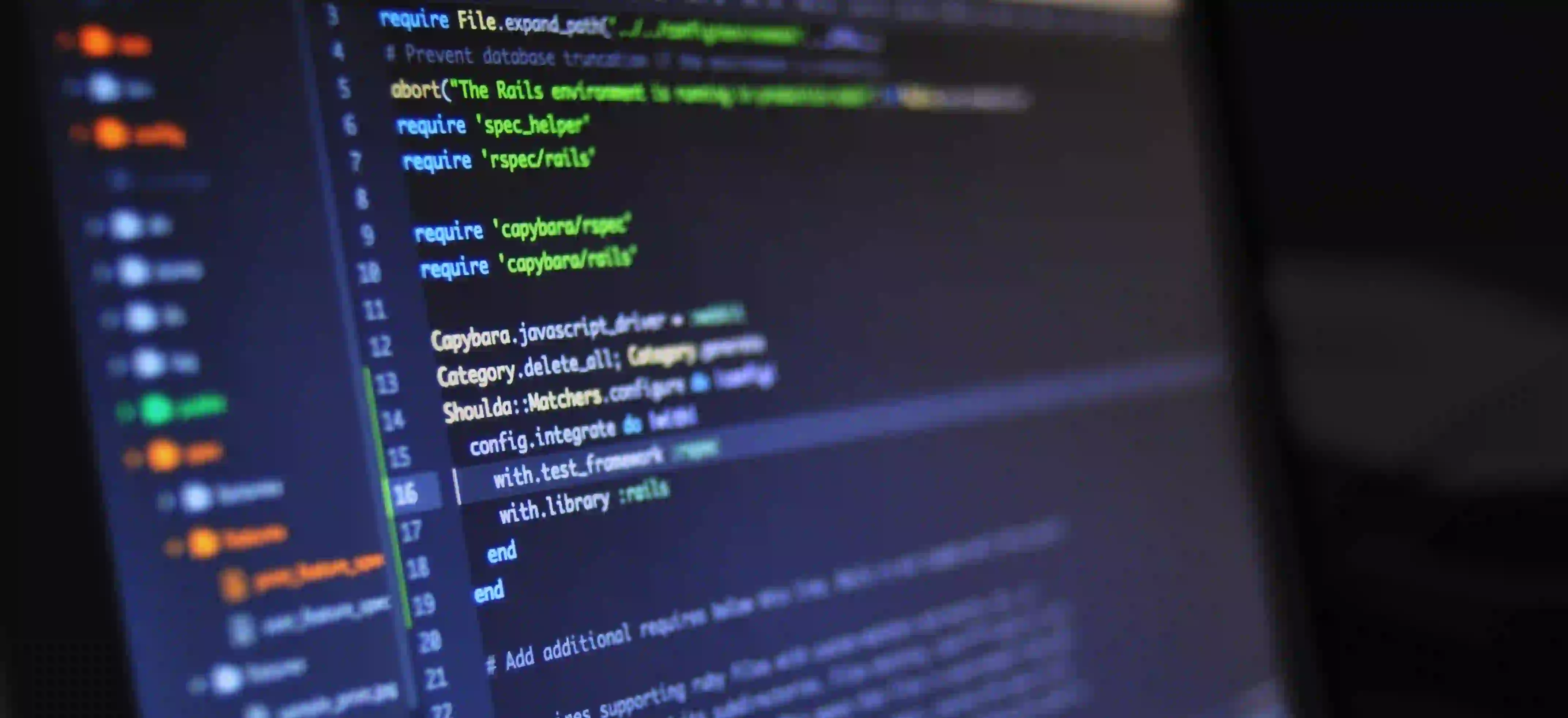
Integrating Java Backend with React Native Barcode Widget
In the ever-evolving landscape of mobile app development, bridging the gap between a robust backend and an intuitive frontend is paramount. For developers leveraging React Native for building mobile applications, integrating various backend technologies becomes crucial. In this post, we will explore how to effectively integrate a Java backend with a React Native barcode widget.
Why Use a Barcode Widget?
A barcode widget is indispensable for applications that require scanning and processing barcodes. This can be useful for a myriad of applications such as inventory management, retail apps, and more. For more insight into building a React Native barcode widget, you can check out Mastering React Native: Building a Barcode Widget.
Setting Up the Java Backend
Before diving into the integration process, the first step is to set up the Java backend. For our example, we will use Spring Boot, a powerful framework that simplifies the creation of production-ready applications.
Step 1: Create a Spring Boot Application
To kick off, let’s create a new Spring Boot project using Spring Initializr.
-
Navigate to Spring Initializr.
-
Select the following options:
- Project: Maven Project
- Language: Java
- Spring Boot: (latest stable version)
- Group:
com.example
- Artifact:
barcode-backend
- Dependencies: Spring Web, Spring Data JPA, H2 Database
-
Click on "Generate" to download the project and extract it. You can then open it in your favorite IDE, such as IntelliJ IDEA or Eclipse.
Step 2: Implement a Basic Barcode API
Let’s create a simple REST controller that handles barcode data. Below is an example of how to set up your API.
package com.example.barcodebackend.controller;
import org.springframework.web.bind.annotation.*;
import javax.validation.Valid;
@RestController
@RequestMapping("/api/barcodes")
public class BarcodeController {
@PostMapping
public String generateBarcode(@Valid @RequestBody BarcodeRequest request) {
// Logic to generate barcode
return "Generated Barcode: " + request.getData(); // Placeholder logic
}
}
class BarcodeRequest {
private String data;
public String getData() {
return data;
}
public void setData(String data) {
this.data = data;
}
}
Code Explanation
- Annotation @RestController: This annotation marks the class as a REST controller, simplifying the creation of REST APIs.
- @RequestMapping: This specifies the base URL for the REST endpoints.
- @PostMapping: Indicates that this method will handle POST requests aimed at sending data to the API.
- @Valid: Ensures that the incoming request adheres to any validation constraints.
Step 3: Running the Backend
Run your Spring Boot application by executing the main method in BarcodeBackendApplication
. By default, the application will start on localhost:8080.
Setting Up the React Native Application
With our backend ready, it’s time to set up the React Native application that will interface with this Java backend.
Step 1: Create a React Native Project
Create a new React Native project using the following command:
npx react-native init BarcodeApp
Step 2: Install Required Libraries
Navigate into your project and install the libraries required for barcode scanning and making API requests.
npm install @react-native-community/cameraroll axios
Step 3: Implement the Barcode Widget
Now, let’s create a barcode scanning component. This component will utilize the camera to scan barcodes and send the data to our Java backend.
import React, { useState } from 'react';
import { Button, View, Text, Alert } from 'react-native';
import { RNCamera } from 'react-native-camera';
import axios from 'axios';
const BarcodeScanner = () => {
const [barcodeData, setBarcodeData] = useState(null);
const handleBarCodeRead = async (scanData) => {
setBarcodeData(scanData.data);
try {
const response = await axios.post('http://localhost:8080/api/barcodes', {
data: barcodeData,
});
Alert.alert('Success', response.data);
} catch (error) {
console.error(error);
Alert.alert('Error', 'There was an error sending the data to the backend');
}
};
return (
<View style={{ flex: 1 }}>
<RNCamera
style={{ flex: 1 }}
onBarCodeRead={handleBarCodeRead}
captureAudio={false}
/>
{barcodeData && <Text>{`Barcode Data: ${barcodeData}`}</Text>}
</View>
);
};
export default BarcodeScanner;
Code Explanation
- Async Functionality: The
handleBarCodeRead
function is asynchronous, allowing us to wait for the Axios request to be completed. - RNCamera: This component facilitates barcode scanning.
- Axios: Used for making HTTP requests to our Java backend; we send the scanned barcode data in the request body.
- Alert: Used for providing user feedback based on the success or failure of the API call.
Step 4: Running the React Native Application
Make sure you have a running Android or iOS emulator. Use the following command to run your application:
npx react-native run-android
or
npx react-native run-ios
A Final Look
Integrating a Java backend with a React Native barcode widget can significantly enhance your mobile application’s functionality. The combination of React Native’s efficient UI capabilities and Spring Boot's powerful backend functionalities provides a compelling solution for barcode processing applications.
By following the steps outlined in this post, you can successfully scan barcodes and send data to your Java backend service, paving the way for more advanced features.
If you're eager to learn more about barcode widgets in React Native, don't forget to read Mastering React Native: Building a Barcode Widget. Happy coding!