Integrating Java Backend with React Native Barcode Scanners
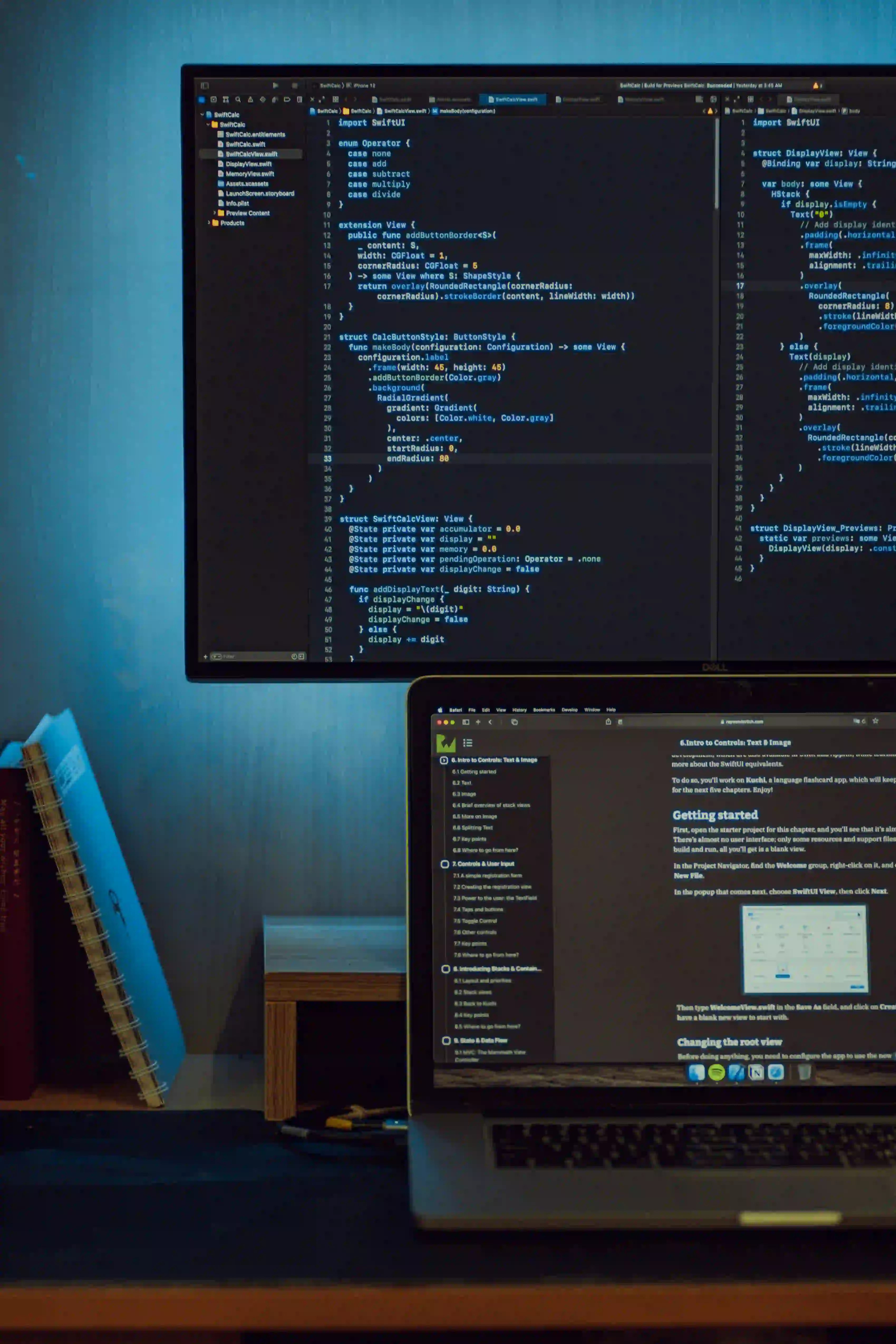
Integrating Java Backend with React Native Barcode Scanners
In the evolving landscape of mobile and web development, integrating a robust backend with a robust frontend is crucial for delivering seamless applications. Barcode scanning has become a staple feature in various applications, ranging from inventory management systems to retail checkout processes. In this post, we'll explore how to integrate a Java backend with React Native to effectively manage barcode scanning operations.
Understanding the Components
Before diving into the integration process, let's clarify the components involved:
- Java Backend: This is where your business logic resides. It handles requests, processes data, and manages connections to databases or other services.
- React Native: This is a popular framework for building mobile applications using React. It allows developers to use JavaScript and React to create native applications for Android and iOS.
Combining these technologies can streamline functionalities such as user authentication, data storage, and barcode recognition.
Setting Up Your Java Backend
We'll begin by setting up a simple Java backend using Spring Boot, a framework that simplifies the bootstrapping and development of new Spring applications.
Step 1: Create a Spring Boot Application
Create a new Spring Boot application using your preferred method, such as Spring Initializr. Ensure you include dependencies for Spring Web and Spring Data JPA.
// Application.java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Step 2: Define the REST API
In your Java backend, create a REST controller to handle barcode-related requests. This controller will receive barcode data and respond accordingly.
// BarcodeController.java
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api/barcode")
public class BarcodeController {
@PostMapping("/scan")
public ResponseEntity<String> scanBarcode(@RequestBody BarcodeData barcodeData) {
// Process the scanned barcode data
return ResponseEntity.ok("Scanned barcode: " + barcodeData.getBarcode());
}
}
Explanation
- The
@RestController
annotation indicates that this class contains RESTful services. - In the
scanBarcode
method, we accept a POST request containing the scanned barcode data.
Step 3: Setting Up the Barcode Data Model
Define a model class for the barcode data. This class will encapsulate the incoming barcode information.
// BarcodeData.java
public class BarcodeData {
private String barcode;
// Getter and Setter
public String getBarcode() {
return barcode;
}
public void setBarcode(String barcode) {
this.barcode = barcode;
}
}
Creating the React Native Application
Now that we have our backend set up, let’s create the React Native application that will interact with the Java backend.
Step 1: Initialize React Native Project
Use the React Native CLI to create a new project. Open your terminal and run:
npx react-native init BarcodeScannerApp
cd BarcodeScannerApp
Step 2: Install Libraries
You can utilize a library such as react-native-camera
for scanning barcodes. Install the necessary packages:
npm install react-native-camera axios
Step 3: Creating the Barcode Scanner Component
In your React Native application, create a new component that integrates with the camera and handles barcode scanning.
// BarcodeScanner.js
import React, { useState } from 'react';
import { View, Text, Button } from 'react-native';
import { RNCamera } from 'react-native-camera';
import axios from 'axios';
const BarcodeScanner = () => {
const [barcode, setBarcode] = useState('');
const sendBarcodeData = async (data) => {
try {
const response = await axios.post('http://your-java-backend-url/api/barcode/scan', {
barcode: data,
});
console.log(response.data);
} catch (error) {
console.error(error);
}
};
return (
<View style={{ flex: 1 }}>
<RNCamera
style={{ flex: 1 }}
onBarCodeRead={(e) => {
setBarcode(e.data);
sendBarcodeData(e.data);
}}
/>
{barcode && <Text>Scanned Barcode: {barcode}</Text>}
</View>
);
};
export default BarcodeScanner;
Explanation
- RNCamera captures the camera feed.
- The
onBarCodeRead
event triggers when a barcode is detected. - The detected barcode is sent to the Java backend using Axios.
Step 4: Connecting the Component to Your Application
In your main app file, include the BarcodeScanner
component:
// App.js
import React from 'react';
import { SafeAreaView } from 'react-native';
import BarcodeScanner from './BarcodeScanner';
const App = () => {
return (
<SafeAreaView style={{ flex: 1 }}>
<BarcodeScanner />
</SafeAreaView>
);
};
export default App;
Testing Your Integration
- Run Your Java Backend: Make sure your Spring Boot application is up and running.
- Run Your React Native Application: Use the command below to start your React Native application.
npx react-native run-android
- Scan a Barcode: Point the camera at a barcode, and the application should read the barcode and display it on the screen. You can also check the backend logs to ensure that the data is received correctly.
Additional Considerations
When dealing with barcode scanning in production, consider the following:
- Error Handling: Implement robust error handling to manage failures gracefully.
- Performance: Optimize the camera feed to ensure smooth scanning functionality.
- Security: Secure your backend endpoints, especially if you process sensitive data.
My Closing Thoughts on the Matter
Integrating a Java backend with a React Native application to handle barcode scanning involves setting up RESTful APIs and creating mobile components that interact seamlessly with these services. This integration not only enhances user experience but also streamlines business operations. If you want to dive deeper into mobile development, check out the article Mastering React Native: Building a Barcode Widget.
With this foundational knowledge, you're ready to develop applications that harness the power of barcode scanning effectively. Happy coding!