Mastering Ant vs. Gradle: The Target Task Dilemma
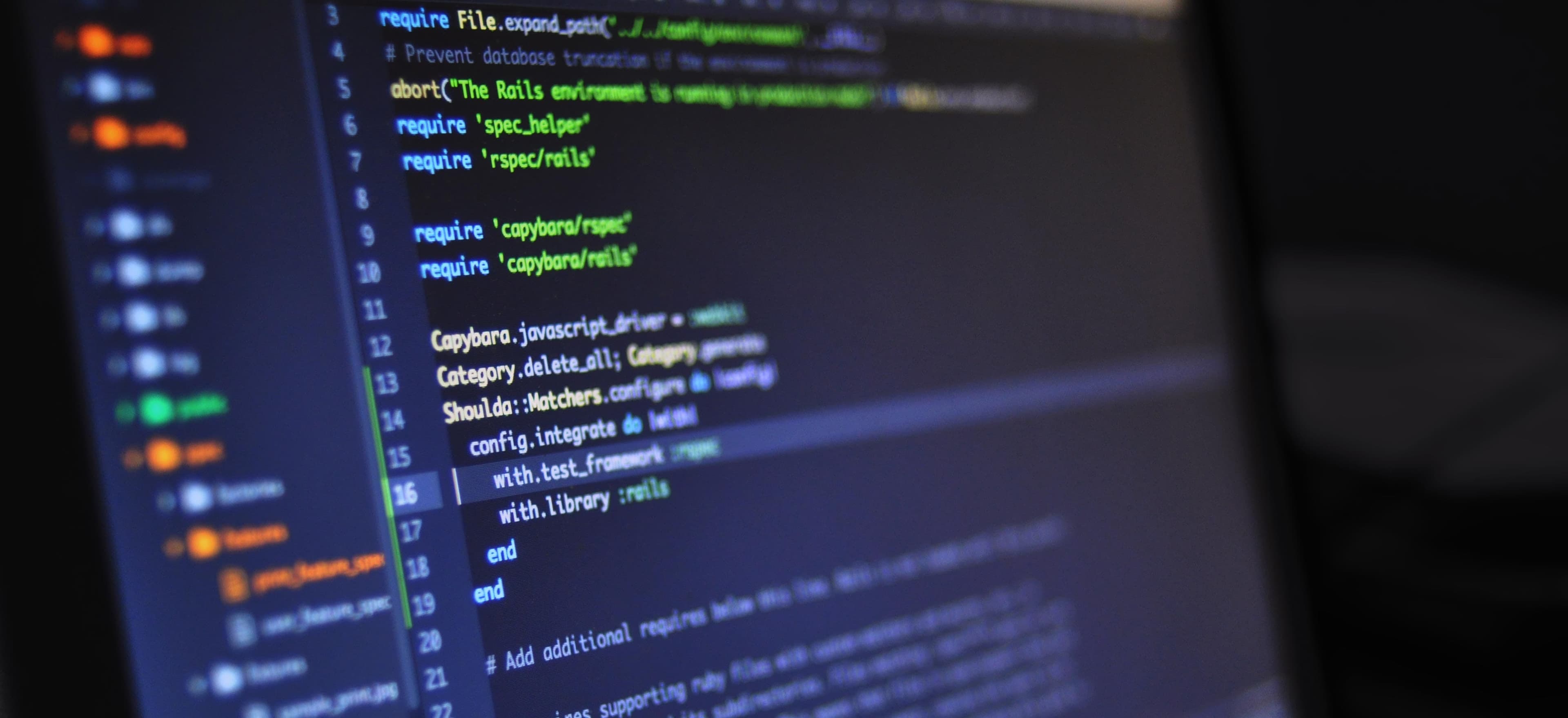
- Published on
Mastering Ant vs. Gradle: The Target Task Dilemma
When it comes to build automation in Java projects, two prominent tools stand out: Apache Ant and Gradle. Both offer unique capabilities and features designed to streamline the build process, but they have fundamental differences in approach, design philosophy, and usability. This post dives deep into these differences, focusing on the target task dilemma within each tool, while providing insights on how to choose the right one for your project needs.
Understanding Build Automation
Build automation is the process of automating the steps required to build, package, and deploy software. It encompasses compiling source code, packaging binaries, running tests, generating documentation, and even deploying applications. Effective build systems enhance productivity, reduce human error, and ensure consistent builds.
Ant: The Traditional Powerhouse
Apache Ant, developed in the late 1990s, is often regarded as a mainstream option for building Java applications. It follows an XML-based configuration system and provides a rich set of built-in tasks. A fundamental feature of Ant is its reliance on targets, encompassing a sequence of tasks to be executed.
Key Features of Ant
- XML Configuration: Ant build files are defined in XML format, allowing for clear task hierarchies and dependencies.
- Extensible: Users can create custom tasks to handle proprietary or complex operations.
- Procedural Approach: Ant executes tasks in a sequential manner, which is intuitive for many developers.
Example Ant Build File
<project name="ExampleProject" default="compile" basedir=".">
<property name="src" value="src"/>
<property name="build" value="build"/>
<target name="clean">
<delete dir="${build}"/>
</target>
<target name="compile">
<mkdir dir="${build}"/>
<javac srcdir="${src}" destdir="${build}">
<include name="**/*.java"/>
</javac>
</target>
</project>
Why This Code Matters: The clean
target ensures an empty build directory, while compile
prepares the build environment and compiles source files. This separation of concerns enhances organization within the build process.
Gradle: The Modern Contender
Gradle emerged as a modern alternative to Ant, boasting a more intuitive DSL (Domain Specific Language) built on Groovy and Kotlin. This design allows it to use code to describe builds rather than mere XML.
Key Features of Gradle
- Declarative Build Scripts: Natural syntax for defining builds makes them easier to read and write.
- Incremental Builds: Gradle supports full and incremental builds based on task dependencies, enhancing speed.
- Built-in Dependency Management: Dependency resolution is simple and flexible, integrating seamlessly with Maven repositories.
Example Gradle Build File
plugins {
id 'java'
}
group 'com.example'
version '1.0-SNAPSHOT'
repositories {
mavenCentral()
}
dependencies {
testImplementation 'junit:junit:4.12'
}
tasks.register('clean', Delete) {
delete rootProject.buildDir
}
tasks.register('compileJava') {
doLast {
javaCompile {
source 'src'
destinationDir file("$buildDir/classes")
}
}
}
Why This Code Matters: Here, Gradle optimizes the clean and compile tasks without verbosity. Each task is regarded as a first-class citizen, enabling composability and easier maintenance.
The Target Task Dilemma
The target task dilemma refers to the challenges developers face in understanding which tasks to execute, how to structure them, and what dependencies exist between them in build automation tools.
Ant and the Target Approach
In Ant, targets are explicit—defined and invoked without much context about dependencies. To compile a Java application, one must remember to invoke several targets manually.
For example, to compile, the developer must run:
ant clean
ant compile
This could lead to errors if developers forget to clean prior to building.
Dependency Management
Ant allows the specification of dependencies between targets, but it’s less intuitive. Each target must explicitly declare which tasks it depends on, leading to possible oversights.
Gradle and the Dependency Mechanism
Gradle’s approach to tasks is more dynamic. It defines the resolution order based on task dependencies. Thus, calling:
gradle clean compileJava
automatically handles the tasks in the correct order, without worrying if one was omitted.
Why Choose One Over the Other?
When considering which build tool to use, focus on the following criteria:
-
Project Size and Complexity:
- Use Ant for small, legacy projects or where XML-based configurations are preferred.
- Choose Gradle for larger, complex projects requiring sophisticated dependency management and ease of plugins.
-
Learning Curves:
- Ant has a straightforward structure but can become cumbersome with larger projects.
- Gradle has a steeper learning curve but allows for more flexibility and maintains clearer syntax.
-
Ecosystem Compatibility:
- Gradle integrates seamlessly with modern tools like Spring Boot, Android development, and CI/CD pipelines, making it a preferred choice in newer technology stacks.
In Conclusion, Here is What Matters
As these tools evolve, the Ant versus Gradle debate remains crucial in the Java ecosystem. Understanding the target task dilemma offers insights into how to structure build processes effectively, preventing issues related to task management.
For more comprehensive knowledge about build tools and their use cases, check out Gradle User Manual and the Apache Ant Documentation.
Making an informed choice between Ant and Gradle involves weighing the pros and cons based on project requirements. Regardless of the decision, both tools can significantly enhance your Java build process by automating routine tasks, thereby championing efficiency and promoting good practices in software development.
Next Steps
Take the time to experiment with both Ant and Gradle in your development environment. As you gain familiarity, you will discover which tool resonates better with your workflow and enhances your productivity. After all, mastering build tools can be a game changer in how we develop modern software.
Checkout our other articles