Streamline Your Java Code: Move Conditions to Message Files
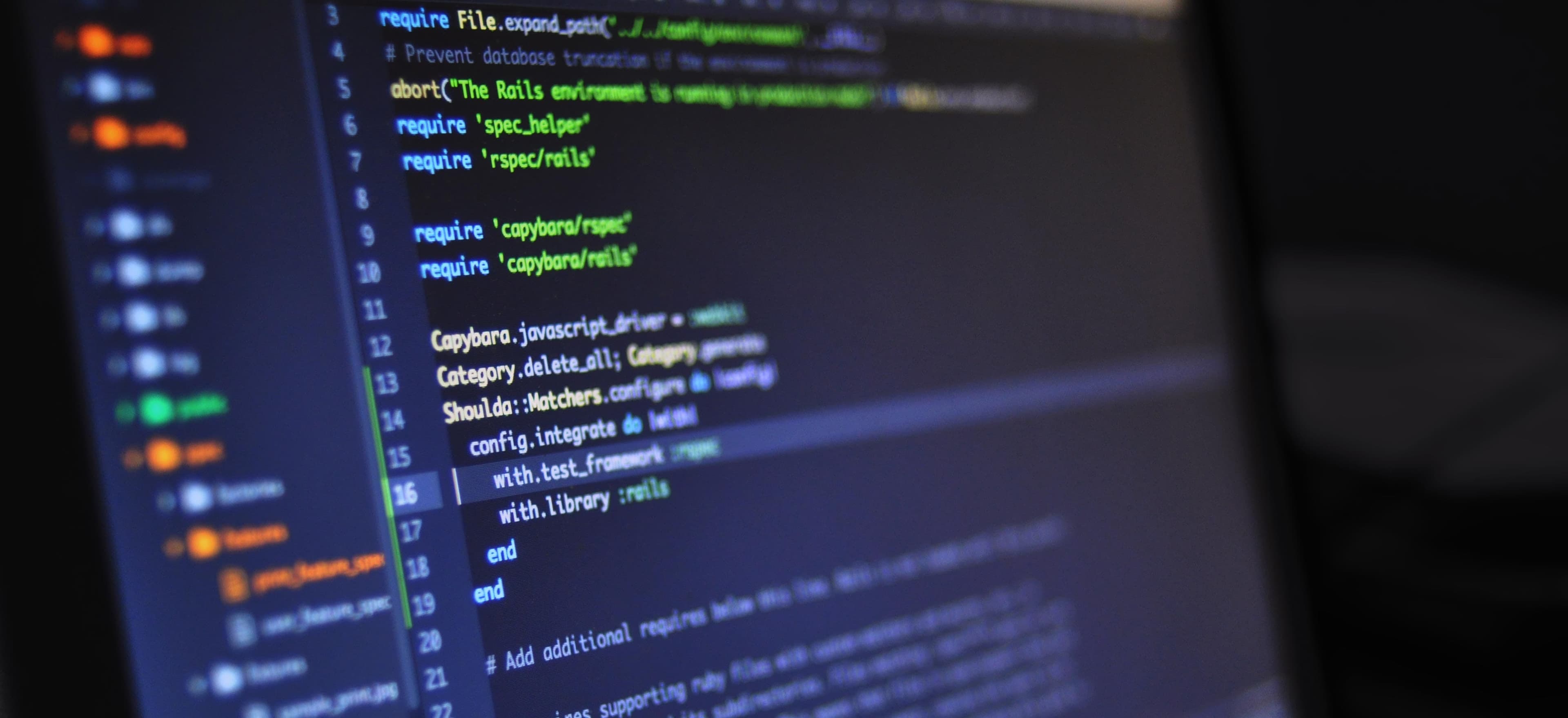
- Published on
Streamline Your Java Code: Move Conditions to Message Files
In software development, maintaining clean, efficient, and scalable code is paramount. One of the key principles in achieving this is to eliminate hardcoded strings and conditions in your code. Instead, consider moving them to external message files. This blog post will explore why you should move conditions to message files in Java and provide practical examples to illustrate the concept effectively.
Why Move Conditions to Message Files?
1. Separation of Concerns
By moving conditions to message files, you can separate the logic of your application from its configurable parameters. This would make your Java code cleaner and easier to maintain. You won’t have to comb through multiple lines of code to make changes related to messages or conditions.
2. Localization
If you plan to expand your application to other regions or languages, message files easily allow for localization. You can simply provide translations for your messages in the corresponding message files without altering the Java code itself.
3. Maintainability
When conditions or messages are encapsulated within message files, a change in functionality or text can be managed outside the core code. This adheres to the DRY (Don't Repeat Yourself) principle and enhances maintainability.
4. Testing
Moving conditions to message files allows for better separation of logic, which facilitates easier testing. Test cases can focus on the behavior and outcome rather than hardcoded values.
Implementation Example
Let's say you have some Java code responsible for user notifications. The following snippet contains hardcoded strings and conditions.
public class UserNotification {
public void notifyUser(int errorCode) {
if (errorCode == 1) {
System.out.println("User not found");
} else if (errorCode == 2) {
System.out.println("Invalid password");
} else {
System.out.println("Unknown error");
}
}
}
Refactoring Steps
-
Create a Properties File Create a file named
messages.properties
that houses the messages for user notifications. Here’s how the content might look:user.not.found = User not found invalid.password = Invalid password unknown.error = Unknown error
-
Load Properties in Java
Next, let's load this properties file in our Java application. We will do this using the
Properties
class to handle reading the file.import java.io.InputStream; import java.util.Properties; public class MessageLoader { private Properties properties; public MessageLoader() { properties = new Properties(); loadMessages(); } private void loadMessages() { try (InputStream input = getClass().getClassLoader().getResourceAsStream("messages.properties")) { if (input == null) { System.out.println("Sorry, unable to find messages.properties"); return; } properties.load(input); } catch (Exception ex) { ex.printStackTrace(); } } public String getMessage(String key) { return properties.getProperty(key, "Message not found"); } }
In this code:
- We load messages from the
messages.properties
file into aProperties
object. - The method
getMessage(String key)
fetches messages based on a key.
- We load messages from the
-
Refactor UserNotification Class
Now, let’s update the
UserNotification
class to use thisMessageLoader
.public class UserNotification { private MessageLoader messageLoader; public UserNotification() { messageLoader = new MessageLoader(); } public void notifyUser(int errorCode) { String messageKey; switch (errorCode) { case 1: messageKey = "user.not.found"; break; case 2: messageKey = "invalid.password"; break; default: messageKey = "unknown.error"; break; } System.out.println(messageLoader.getMessage(messageKey)); } }
Benefits Realized
With this new implementation:
- Your error messages are now housed in a centralized message file.
- Modifications to these messages can be done without touching the code logic.
- Code readability and maintainability have significantly increased.
Closing the Chapter
Moving conditions to message files is an effective way to update your Java codebase, making it cleaner and more manageable. This practice brings a host of benefits, including separation of concerns, enhanced localization capabilities, and better maintainability.
For more on localization and message handling in Java, refer to the Java Internationalization and Localization documentation.
By adopting this methodology, you will not only optimize your code but also prepare it for future growth. Don't shy away from refactoring older code; your future self will thank you!
Happy coding!
Checkout our other articles