Common MapReduce Pitfalls and How to Avoid Them
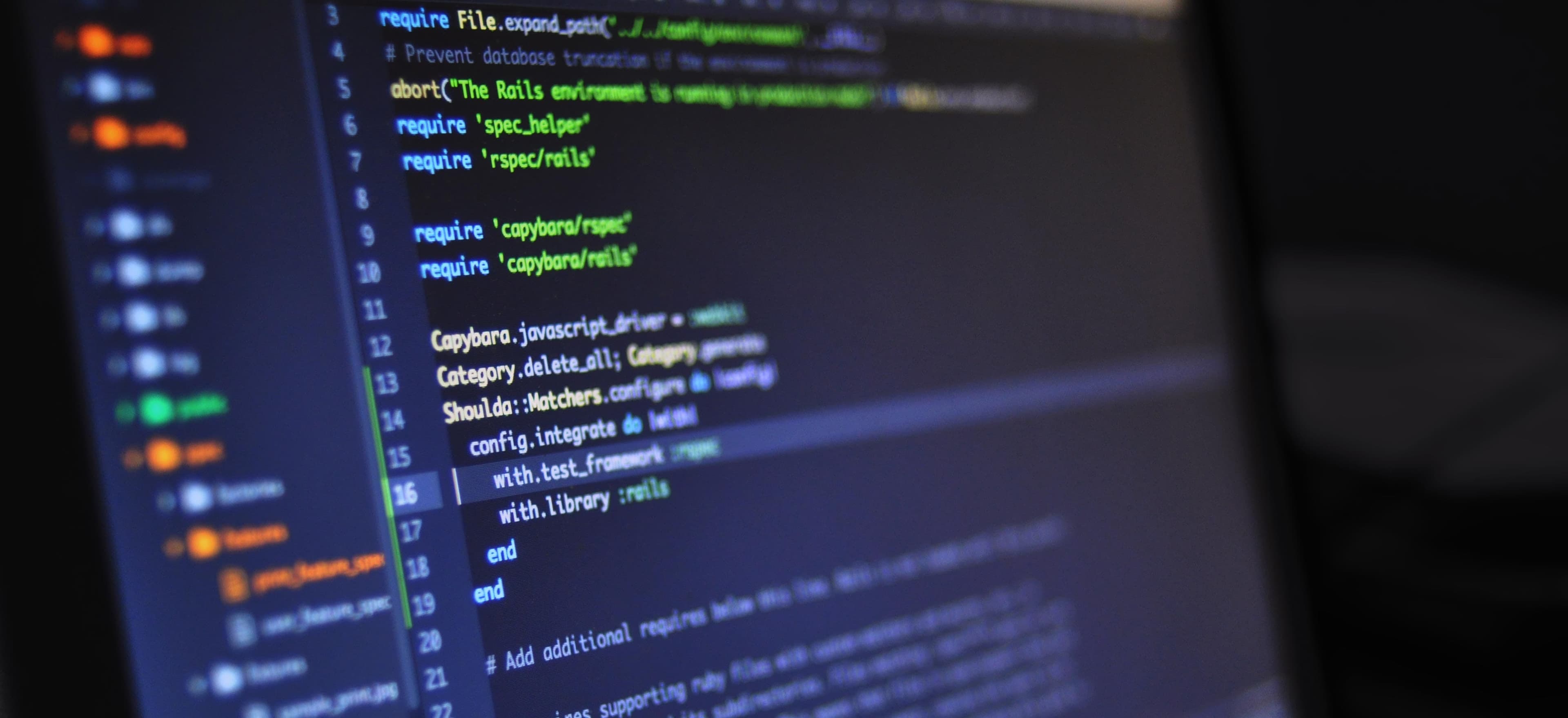
- Published on
Common MapReduce Pitfalls and How to Avoid Them
MapReduce is a powerful programming model that allows for distributed computing across large data sets. Although it offers numerous advantages, developers can encounter pitfalls that may hinder performance or lead to unexpected behavior. In this blog post, we will explore some common pitfalls while using MapReduce and provide best practices for avoiding them.
Understanding the MapReduce Program Structure
Before diving into the pitfalls, let's refresh our understanding of the MapReduce structure.
MapReduce consists of two main functions:
- Map: This function processes input data and produces intermediate key-value pairs.
- Reduce: This function merges and processes the intermediate data based on the keys produced by the map function.
Here’s a simplified code snippet demonstrating the map and reduce functions:
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
import java.io.IOException;
public class WordCount {
public static class TokenizerMapper extends Mapper<Object, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
@Override
public void map(Object key, Text value, Context context) throws IOException, InterruptedException {
String[] words = value.toString().split("\\s+");
for (String w : words) {
word.set(w);
context.write(word, one);
}
}
}
public static class SumReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
private IntWritable result = new IntWritable();
@Override
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
result.set(sum);
context.write(key, result);
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "word count");
job.setJarByClass(WordCount.class);
job.setMapperClass(TokenizerMapper.class);
job.setCombinerClass(SumReducer.class);
job.setReducerClass(SumReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
In this example, we count the occurrences of words in a text file. The map
method splits each line into words and emits (word, 1). The reduce
function sums the counts for each word. The simplicity of this structure demonstrates its usability, but many pitfalls can arise.
Common Pitfalls
1. Inefficient Data Partitioning
Problem: An improper choice of input format or insufficient partitioning can lead to data skewness. This means some reducers are overloaded with more data than others, resulting in performance degradation.
Solution: Use custom partitioners. By defining how your keys are distributed across your reducers, you can ensure even load balancing.
Here’s a basic custom partitioner implementation:
import org.apache.hadoop.mapreduce.Partitioner;
public class CustomPartitioner extends Partitioner<Text, IntWritable> {
@Override
public int getPartition(Text key, IntWritable value, int numPartitions) {
return (key.toString().hashCode() & Integer.MAX_VALUE) % numPartitions;
}
}
Make sure to set your custom partitioner in your job configuration:
job.setPartitionerClass(CustomPartitioner.class);
This ensures that your data is evenly split among workers.
2. Not Using Combiner Functions
Problem: In scenarios where the Map function produces a large amount of data, failing to implement a combiner can lead to increased network traffic and slower performance.
Solution: Use a combiner function that executes a local reduce operation on the map output before sending it to the reducers.
In the provided code, job.setCombinerClass(SumReducer.class);
demonstrates the use of the combiner. In many cases, a combiner is identical to the reducer, processing the output of the map function locally to reduce the amount of data transmitted over the network.
3. Ignoring Memory Management
Problem: Depending on your data set's size, ignoring memory allocation can severely impact your job's execution time or even cause it to fail.
Solution: Monitor and fine-tune your memory settings. Using the Hadoop configuration properties such as mapreduce.map.memory.mb
and mapreduce.reduce.memory.mb
can aid in optimizing memory usage.
<property>
<name>mapreduce.map.memory.mb</name>
<value>2048</value>
</property>
<property>
<name>mapreduce.reduce.memory.mb</name>
<value>2048</value>
</property>
This will provide enough memory for both mapper and reducer tasks.
4. Poorly Designed Input and Output Formats
Problem: Using inefficient data formats can significantly slow down I/O operations during map and reduce tasks.
Solution: Prefer widely-used formats like Avro, Parquet, or Sequence File over plain text for input and output data storage. These formats allow for efficient data compression and faster processing times.
For instance, if using Avro:
job.setInputFormatClass(AvroKeyInputFormat.class);
job.setOutputFormatClass(AvroKeyOutputFormat.class);
Refer to Apache Avro for more insights into how to implement these formats.
5. Lack of Error Handling
Problem: Silently failing jobs can be hard to debug, leading to frustration for developers.
Solution: Implement logging and exception handling to identify exactly where the job fails. Hadoop provides a logging framework that can be configured to enhance the transparency of the MapReduce job lifecycle.
Here’s a simple way to log within your Mapper or Reducer:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class TokenizerMapper extends Mapper<Object, Text, Text, IntWritable> {
private static final Logger logger = LoggerFactory.getLogger(TokenizerMapper.class);
@Override
public void map(Object key, Text value, Context context) {
try {
// parsing and processing
} catch (Exception e) {
logger.error("Error processing input: " + value.toString(), e);
}
}
}
6. Not Testing with Small Data Sets
Problem: Deploying MapReduce jobs directly on large data sets can result in wasted resources and time if there are errors.
Solution: Always test with smaller, representative sample data sets before running jobs on the entire data set. This can help mitigate risks and allow for easier debugging.
The Last Word
By remaining aware of these common pitfalls and their corresponding solutions, developers can ensure their MapReduce jobs run smoothly and efficiently. Proper partitioning, smart memory management, and thoughtful input/output formats will save significant time and resources.
Ready to dive into MapReduce? Utilize the tips covered here, and you’ll be equipped to tackle MapReduce challenges head-on. For further reading, check out the Apache Hadoop documentation, which provides deeper insights and advanced configurations.
Happy coding!
Checkout our other articles