Common Java Socket Programming Mistakes You Must Avoid
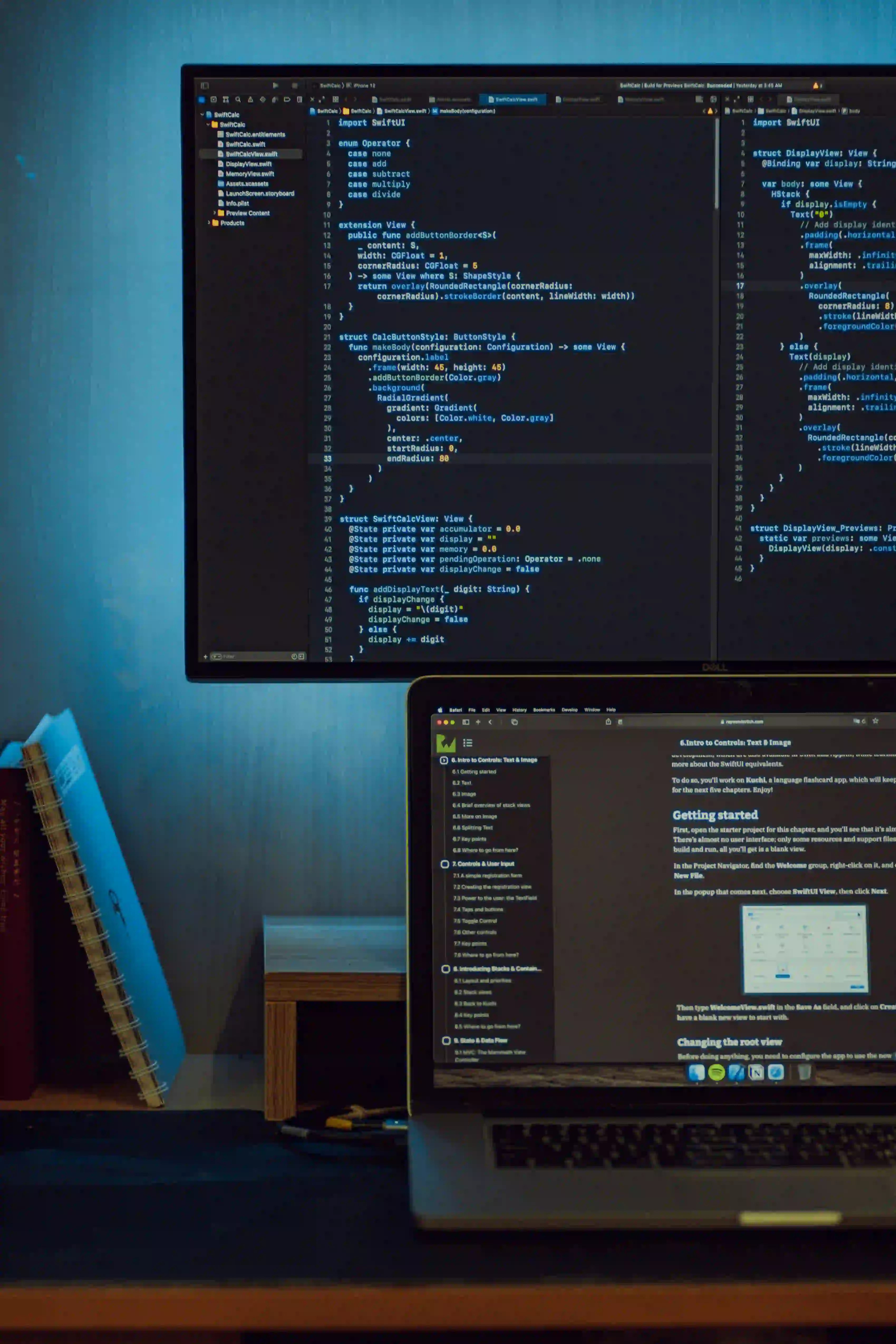
Common Java Socket Programming Mistakes You Must Avoid
Socket programming in Java is an essential skill for building networked applications. Whether you are developing a web server, a chat application, or any client-server communication system, understanding socket programming is crucial. However, there are numerous pitfalls that developers frequently encounter. In this blog post, we'll explore some of the most common Java socket programming mistakes and how to avoid them.
1. Forgetting to Close Resources
One of the most common mistakes is forgetting to close socket connections. Failing to close sockets can lead to resource leaks, which ultimately affect the performance of your application. Always ensure that sockets and streams are closed properly.
Example:
Socket socket = null;
try {
socket = new Socket("localhost", 8080);
// Perform socket operations...
} catch (IOException e) {
e.printStackTrace();
} finally {
if (socket != null) {
try {
socket.close(); // Ensures the socket is closed
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why: The finally
block guarantees that the socket is closed, even if an exception occurs. This helps prevent resources from being left open, which can cause memory leaks.
2. Ignoring Exception Handling
Network operations are fraught with potential exceptions. Ignoring exceptions can lead to undetected issues during runtime, which are much harder to debug. Always handle exceptions explicitly and log them for troubleshooting.
Example:
try {
// Code that might throw an exception
} catch (IOException ex) {
System.err.println("Network error: " + ex.getMessage());
}
Why: By handling exceptions, you not only prevent crashes but also gain insight into what went wrong. This is crucial for debugging.
3. Blocking Operations Without Timeout
Socket operations such as reading from a stream can block indefinitely. If the server does not send any data, your application may hang. Implementing timeouts helps you avoid such situations.
Example:
Socket socket = new Socket();
socket.connect(new InetSocketAddress("localhost", 8080), 2000); // 2 seconds timeout
Why: Setting a connection timeout ensures that your application does not get stuck waiting for a socket operation.
4. Not Using Buffered Streams
When working with socket I/O, it's common to read and write data using raw streams, which can lead to inefficient data handling. Instead, utilize buffered streams for better performance.
Example:
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(new BufferedWriter(new OutputStreamWriter(socket.getOutputStream())));
Why: Buffered streams read and write data in larger chunks, which is much faster than reading or writing byte by byte. This is essential in high-performance applications.
5. Assuming Proper Data Format
Another common mistake is assuming that data will arrive in the format that you expect. Network communication is not always reliable, hence you should implement checks to validate incoming and outgoing data.
Example:
String message = in.readLine();
if (message == null || !message.startsWith("EXPECTED_PREFIX")) {
throw new IOException("Invalid data received");
}
Why: Verifying the format of the data ensures that your application does not malfunction when it receives unexpected input.
6. Neglecting to Handle Multiple Connections
When building a server, it's crucial to manage multiple connections efficiently. A mistake is to handle each connection in a single thread without proper synchronization, which can lead to performance bottlenecks and resource issues.
Example:
ExecutorService executor = Executors.newFixedThreadPool(10);
while (true) {
Socket clientSocket = serverSocket.accept();
executor.execute(new ClientHandler(clientSocket)); // Handle each client in a separate thread
}
Why: Using a thread pool allows you to handle multiple clients simultaneously without exhausting system resources. It's a cleaner and more efficient approach to manage connections.
7. Not Implementing Data Consistency
When you send messages or commands over sockets, it’s essential to achieve consistency, particularly in client-server architectures. Failure to implement a consistent protocol can lead to command desynchronization.
Example:
out.println("COMMAND_START");
out.println("Data content");
out.println("COMMAND_END");
Why: Using a simple protocol to mark the start and end of the data package helps ensure that the client understands what data to expect and prevents corruption or misinterpretation.
8. Running the Server and Client on the Same Port
It’s easy to confuse server and client configurations, particularly regarding port numbers. If both the client and server attempt to use the same port on the same machine, it will not work as they cannot share the same listening point.
Example:
// Server:
ServerSocket serverSocket = new ServerSocket(8080); // Server listens on port 8080
// Client:
Socket socket = new Socket("localhost", 8080); // Client connects to server on port 8080
Why: Ensure that the server is listening on one port and that the client is connecting to that same port, avoiding any conflicts with other running applications.
9. Ignoring Security Considerations
Socket programming often involves sensitive data. Not using secure communication channels can expose your application to various attacks, including man-in-the-middle attacks.
Use SSL/Secure Sockets:
SSLSocketFactory sslSocketFactory = (SSLSocketFactory) SSLSocketFactory.getDefault();
SSLSocket sslSocket = (SSLSocket) sslSocketFactory.createSocket("localhost", 8080);
Why: Implementing SSL/TLS encrypts the data being transferred, ensuring the confidentiality and integrity of your data. Make sure to always use secure connections in production environments.
10. Not Testing Under Load
Finally, many developers neglect to test their socket application under stress conditions. Failing to do so may lead to performance issues at scale.
Recommendations
Consider using tools such as Apache JMeter or Gatling to simulate load and monitor application performance.
Why: Load testing ensures that your application can handle peak traffic efficiently. It exposes deficiencies, allowing you to optimize resources proactively.
Final Considerations
Socket programming in Java can be daunting, especially with the myriad of potential mistakes that can occur. By avoiding these common pitfalls—such as neglecting proper resource management, inadequate error handling, and ignoring data integrity—you can build robust, efficient socket-based applications.
Always be proactive about testing and refining your socket programing skills. As you navigate through the learning process, consider utilizing resources like the Java Networking official documentation and engaging in community discussions to deepen your knowledge.
Happy coding!