Mastering Collision Detection Challenges in LibGDX Games
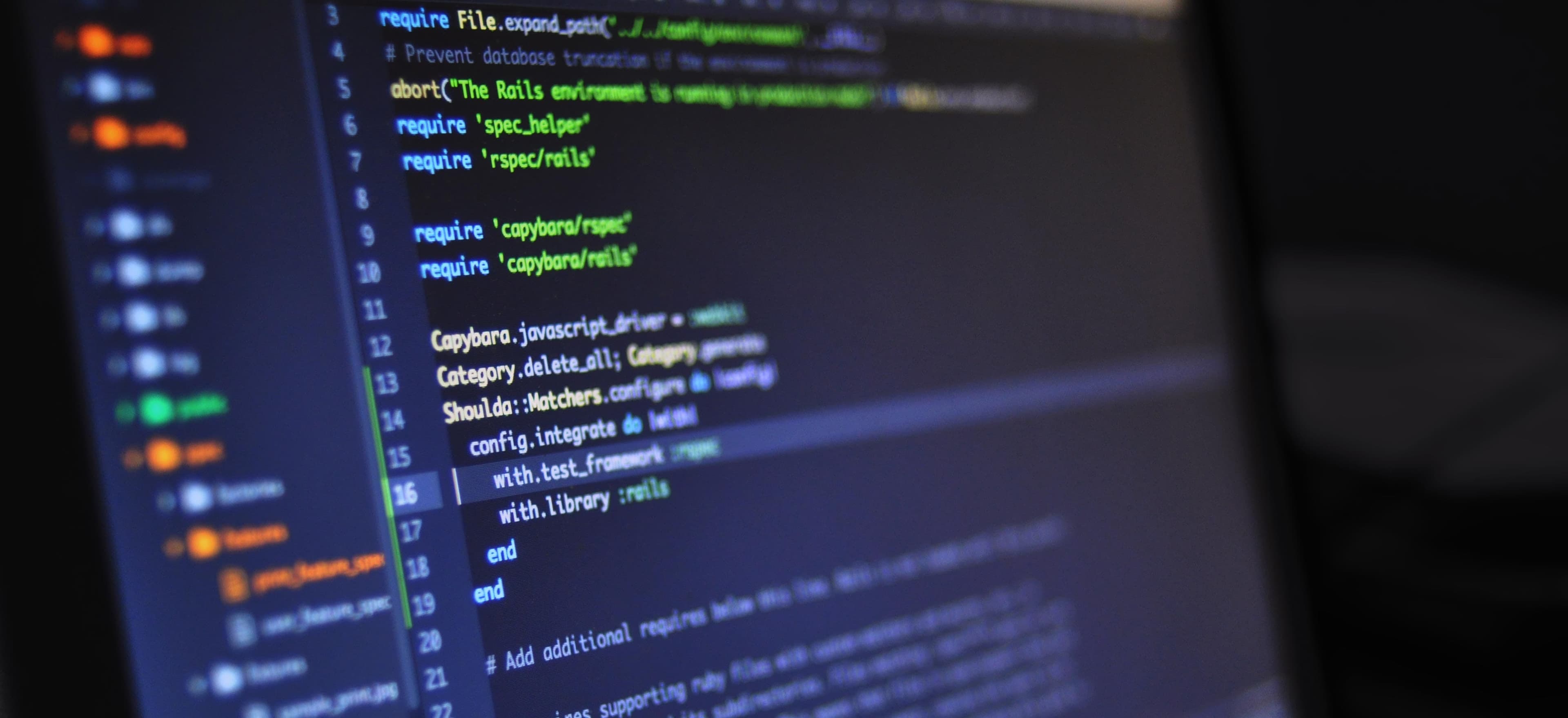
- Published on
Mastering Collision Detection Challenges in LibGDX Games
In game development, collision detection is a critical component that ensures a smooth, interactive experience for players. Whether you're developing a simple 2D platformer or a complex 3D world, understanding how to efficiently implement collision detection can significantly affect the gameplay experience. In this blog post, we’ll dive deep into the methods of collision detection in LibGDX, a popular framework used for building games across multiple platforms.
What is Collision Detection?
Collision detection is a computational problem in computer science and computer graphics that determines when two or more objects collide, overlap, or intersect in a virtual space. In the context of games, this can trigger events such as damage, item pickups, or triggering animations, creating a more immersive experience.
Why Use LibGDX?
LibGDX is an open-source, Java-based game development framework that empowers developers to create games for Windows, macOS, Linux, Android, iOS, and HTML5. Its versatility, flexibility, and robust community support make it an excellent choice for both beginner and experienced game developers.
Basic Concepts of Collision Detection in LibGDX
Before delving into advanced collision detection techniques, let's explore some fundamental concepts:
- Bounding Box: The simplest form of collision detection involves enclosing objects within a box (axis-aligned bounding box or AABB).
- Shapes: LibGDX provides several shapes for collision detection, including circles, polygons, and rectangles.
- Raycasting: This technique allows us to project rays in a specific direction and check for intersections with objects in the environment.
It's important to understand how each of these concepts works together to create an efficient collision detection system.
Creating Basic Shapes
Let's start by creating a simple rectangle and circle in LibGDX:
import com.badlogic.gdx.graphics.glutils.ShapeRenderer;
import com.badlogic.gdx.math.Rectangle;
import com.badlogic.gdx.math.Circle;
public class CollisionDetectionExample {
ShapeRenderer shapeRenderer;
Rectangle rectangle;
Circle circle;
public CollisionDetectionExample() {
shapeRenderer = new ShapeRenderer();
rectangle = new Rectangle(50, 50, 100, 100);
circle = new Circle(200, 200, 50);
}
public void render() {
shapeRenderer.begin(ShapeRenderer.ShapeType.Filled);
shapeRenderer.setColor(Color.RED);
shapeRenderer.rect(rectangle.x, rectangle.y, rectangle.width, rectangle.height);
shapeRenderer.setColor(Color.BLUE);
shapeRenderer.circle(circle.x, circle.y, circle.radius);
shapeRenderer.end();
}
}
Why Use Shapes?
Using shapes like Rectangle and Circle simplifies the collision detection process. They are faster to compute than pixel-perfect collision detection, which can be computationally expensive.
Implementing Collision Detection
Now, let's implement basic collision detection between the rectangle and the circle. LibGDX provides several built-in methods to facilitate this.
AABB Collision Detection
A common method for detecting collisions between bounding boxes is through axis-aligned bounding box (AABB) collision detection. Here's how it works:
public boolean checkRectangleCircleCollision(Rectangle rectangle, Circle circle) {
float closestX = Math.max(rectangle.x, Math.min(circle.x, rectangle.x + rectangle.width));
float closestY = Math.max(rectangle.y, Math.min(circle.y, rectangle.y + rectangle.height));
float distanceX = circle.x - closestX;
float distanceY = circle.y - closestY;
return (distanceX * distanceX + distanceY * distanceY) < (circle.radius * circle.radius);
}
Commenting the Code
- closestX/closestY: These variables find the nearest point on the rectangle to the center of the circle.
- distanceX/distanceY: These variables track the distance between the circle's center and the closest point on the rectangle.
- Collision Check: By checking if the squared distance is less than the squared radius of the circle, we determine if the circle overlaps the rectangle.
Testing for Collisions
To check for collisions during an update cycle, invoke the collision detection method like this:
public void update() {
if (checkRectangleCircleCollision(rectangle, circle)) {
System.out.println("Collision detected!");
}
}
Improving Performance with Spatial Partitioning
For games with many objects, naive collision detection can severely impact performance. This leads us into the realm of spatial partitioning.
Spatial partitioning divides the game world into sections to minimize the number of collision checks. Techniques like Quadtrees (for 2D games) and Octrees (for 3D games) leverage this idea.
!Spatial Partitioning
Consider implementing a quadtree if your game consists of numerous objects. Here’s how to start:
public class Quadtree {
// Add your quadtree implementation here...
}
This implementation can significantly improve collision detection efficiency by reducing the number of checks needed.
Advanced Collision Techniques
Pixel-Perfect Collision Detection
Though AABB is efficient, it may not be enough for all scenarios. In some cases, pixel-perfect collision detection is required. This technique checks whether pixels from two textures overlap.
In LibGDX, you can leverage the Pixmap
class to achieve this:
private boolean pixelPerfectCollision(Texture textureA, Vector2 positionA, Texture textureB, Vector2 positionB) {
// Create pixmap and perform pixel collision detection here
}
While pixel-perfect detection is helpful, remember that it is computationally expensive and should be reserved for critical collision checks.
Physics Libraries Integration
For more complex collision detections, consider integrating a physics library like Box2D. It provides robust features such as body dynamics, friction, and restitution, allowing for realistic game interactions.
Learn more about LibGDX Box2D integration.
Wrapping Up
Collision detection is foundational to any game. By utilizing LibGDX’s built-in mechanisms and advanced techniques like spatial partitioning, developers can achieve efficient and effective collision detection. Whether using AABB, pixel-perfect detection, or incorporating physics libraries such as Box2D, mastering these concepts propels your game development skills to the next level.
As you continue to explore collision detection in LibGDX, remember the importance of performance optimization, especially for larger games. Stay tuned for more insights into various game development topics, and don't hesitate to reach out to the community for assistance and ideas. Happy coding!
Additional Resources
- LibGDX Wiki
- Official LibGDX Documentation
- Pixel Perfect Collision Detection
Feel free to comment below if you have any questions or share your own techniques for collision detection in LibGDX.