How to Safely Access Private Java Methods?
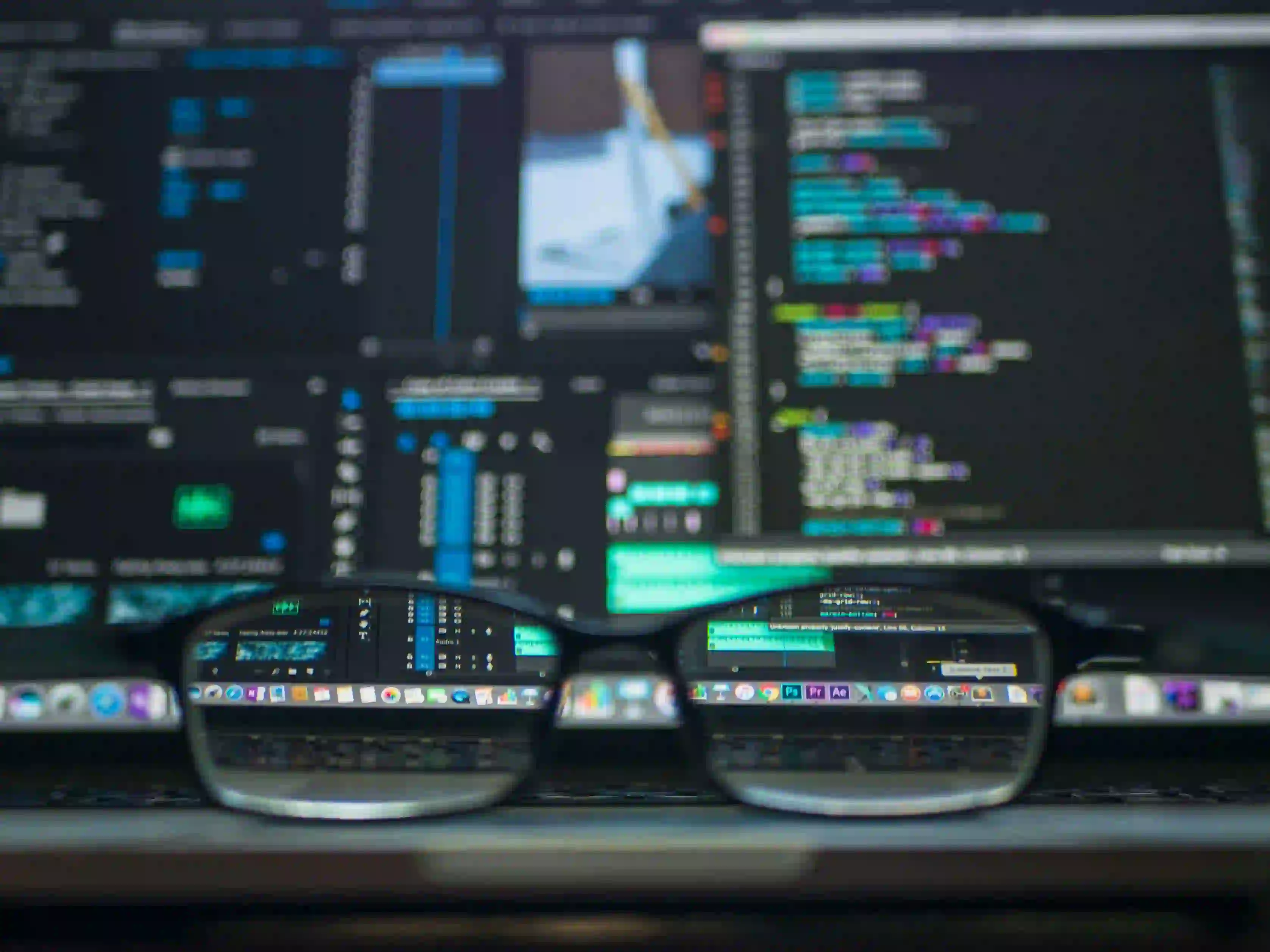
How to Safely Access Private Java Methods
Java, like many other programming languages, enforces encapsulation, a principle of object-oriented programming that promotes the bundling of data with the methods that operate on that data. One key aspect of encapsulation is the visibility of class members (fields and methods). When a method is declared as private
, it means that it is not accessible outside of its own class. While it's often prudent to respect this restriction, there are situations in which accessing a private method becomes necessary, particularly during testing or when working with legacy code. In this post, we'll explore safe ways to access private Java methods, discuss the implications, and provide code examples along the way.
Why Access Private Methods?
Understanding the reasons for wanting to access private methods can help clarify the situation. Here are a few common scenarios:
-
Unit Testing: Sometimes, private helper methods contain logic that is crucial for the correct functioning of public methods. Unit tests often focus on testing the behavior of class interfaces, so it may seem necessary to access these private methods directly.
-
Reflection: Java Reflection API provides a way to inspect classes, interfaces, fields, and methods at runtime, regardless of their access modifiers.
-
Legacy Code: Working with code that lacks proper testing or documentation may require accessing private methods to understand how components interact.
Accessing Private Methods Safely
While accessing private methods can be done through reflection, it's important to do so with caution. Below, we'll go through several methods of access, explaining the benefits and potential pitfalls of each.
1. Using Reflection
Reflection is a powerful feature in Java that allows you to inspect classes, obtain metadata, and invoke methods at runtime, even if they are private. Here’s how you can use reflection to invoke a private method.
Code Example
import java.lang.reflect.Method;
public class ReflectionExample {
private String greet(String name) {
return "Hello, " + name + "!";
}
public static void main(String[] args) {
try {
ReflectionExample example = new ReflectionExample();
// Obtain the private method
Method method = ReflectionExample.class.getDeclaredMethod("greet", String.class);
// Set it accessible
method.setAccessible(true);
// Invoke the method
String greeting = (String) method.invoke(example, "World");
System.out.println(greeting); // Output: Hello, World!
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary
In this example, we accomplished the following:
- Get Method: We're using
getDeclaredMethod()
to retrieve the reference to the private method. - Set Accessible: By calling
setAccessible(true)
, we bypass the access checks of the JVM. - Invoke Method: Finally, we invoke the method using
invoke()
, passing the instance and parameters.
However, it is vital to understand that bypassing access control can lead to maintenance issues. If you're using reflection frequently, it might be a sign that the code design needs refactoring.
2. Friend Classes
Another approach to accessing private methods safely is by using friend classes. In this method, we create a companion class whose sole purpose is to perform the testing or interactions with the original class's private methods.
Code Example
public class MainClass {
private String samplePrivateMethod() {
return "Sample Private Method";
}
}
class FriendClass {
public String accessPrivateMethod(MainClass mainClass) {
return mainClass.samplePrivateMethod();
}
}
Commentary
- Encapsulation Maintained: By keeping the access to the method within a specific friend class, we maintain the essence of encapsulation.
- Ease of Testing: This pattern allows for focused unit tests without utilizing reflection, making the codebase cleaner.
3. Subclassing
If the method is not final and you're allowed to subclass it within the same package, another option is to subclass the original class and create a public method in the subclass that calls the private method.
Code Example
public class BaseClass {
private String privateMethod() {
return "Hello from private method!";
}
}
public class SubClass extends BaseClass {
public String accessPrivateMethod() {
return privateMethod();
}
}
Commentary
- Cleaner Design: This approach results in a cleaner design since you use traditional OOP concepts.
- Access Control: Note that the original
privateMethod
remains protected from outside access, preserving encapsulation.
4. Refactoring
Whenever possible, consider refactoring the code to allow for better access. Move the logic of the private method into a protected or package-private method if it is meant to be reused.
Code Example
public class Example {
// Removed private and made it protected
protected String reusableMethod() {
return "Reusable method logic.";
}
public String publicAccess() {
return reusableMethod();
}
}
Commentary
- Testability Enhanced: This allows your private method's logic to be tested without reflection.
- Design Improvements: It encourages better use of OOP principles by promoting method reusability.
Final Considerations
While it can be tempting to access private methods directly for testing or other purposes, it's often not the most effective solution. Reflection, while powerful, can break encapsulation and lead to maintenance challenges. Instead, consider creating friend classes, subclassing, or refactoring your code for safer and more maintainable designs.
Additional Resources
By applying the methods discussed in this post, you can navigate the complexities of Java's access control while maintaining a clean, efficient codebase that adheres to best practices. Happy coding!