Boosting Performance: Automating Java Class Specializations
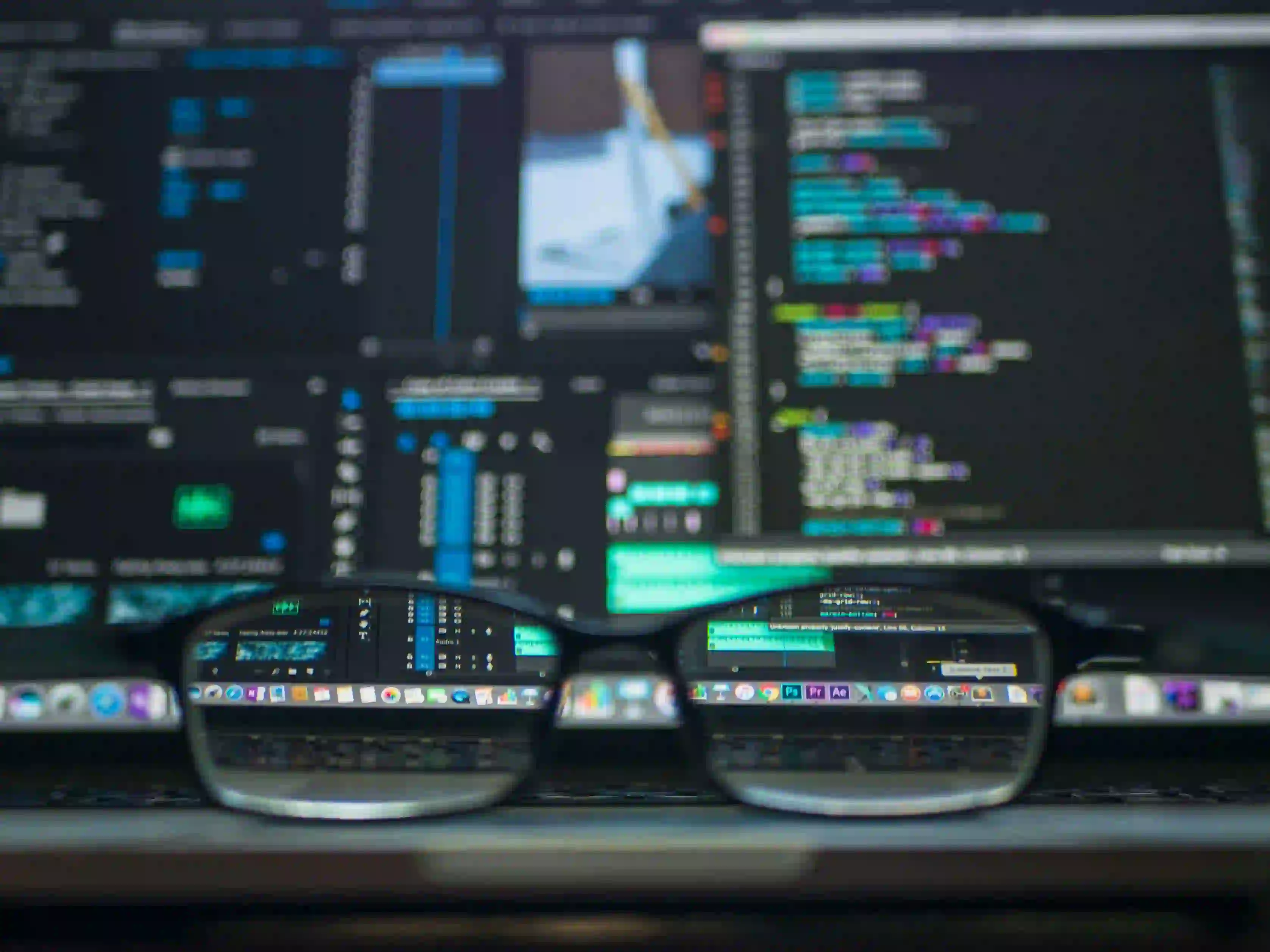
Boosting Performance: Automating Java Class Specializations
In today's fast-paced tech landscape, optimizing performance is crucial, especially when working with a language like Java. As Java continues to evolve, one area that developers can leverage for improved performance is class specialization. This blog post will explore the concept of class specialization in Java and provide insights into how automating this process can result in significant performance improvements.
What is Class Specialization?
Class specialization is the technique of creating specialized versions of a class to optimize the behavior of certain operations. For instance, consider a base class that handles general tasks—this class may be capable of serving diverse scenarios, but it might not be optimized for any specific case. By specializing classes, we can tailor performance to meet distinct needs.
Why Specialize?
There are several compelling reasons to use class specialization:
- Performance: Specialized classes can lead to quicker execution as they can focus on the exact requirements without the overhead of generalized handling.
- Reduced Memory Footprint: Specialized classes often require smaller memory allocations compared to their general counterparts.
- Maintenance and Clarity: By separating concerns and creating focused classes, the codebase can become easier to understand and maintain.
Now that we've wrapped our heads around the basics, let us dive into the methods for automating class specialization in Java.
Automating Class Specialization
Key Concepts
To effectively automate class specialization, we need to consider two main ideas: Static vs. Dynamic Specialization and Compiler Optimizations.
-
Static Specialization: This involves making design decisions at compile time, hence resulting in optimized bytecode being produced.
-
Dynamic Specialization: This includes techniques such as Just-In-Time (JIT) compilation or runtime code generation.
We’ll delve into techniques with examples focusing on static specialization with the help of generics and annotations.
Using Generics for Specialization
Generics in Java can help create a base class or method that acts differently based on the type parameters provided. Here is an example where we specialize behavior for different data types using generics.
public class DataProcessor<T> {
private final T[] data;
public DataProcessor(T[] data) {
this.data = data;
}
public void process() {
if (data instanceof Integer[]) {
processIntegers((Integer[]) data);
} else if (data instanceof String[]) {
processStrings((String[]) data);
} else {
throw new UnsupportedOperationException("Unsupported data type");
}
}
private void processIntegers(Integer[] integers) {
// Specialized processing for integers
System.out.println("Processing integers");
// Sample operation: sum
int sum = 0;
for (Integer integer : integers) {
sum += integer;
}
System.out.println("Sum: " + sum);
}
private void processStrings(String[] strings) {
// Specialized processing for Strings
System.out.println("Processing strings");
// Sample operation: join
String result = String.join(", ", strings);
System.out.println("Joined: " + result);
}
}
// Usage
public class Main {
public static void main(String[] args) {
DataProcessor<Integer> integerProcessor =
new DataProcessor<>(new Integer[]{1, 2, 3, 4});
integerProcessor.process();
DataProcessor<String> stringProcessor =
new DataProcessor<>(new String[]{"Hello", "World"});
stringProcessor.process();
}
}
Review of the Code
In this code, the DataProcessor
class is defined generically to handle both integer and string arrays. The process
method checks the type and delegates to the appropriate specialized method. This approach leverages compile-time checks while eliminating the need for casting and runtime checks, enhancing performance.
Leveraging Annotations for Specialization
Annotations can act like metadata in Java helping processes determine what actions or optimizations need to be applied to certain classes. By creating custom annotations, we can mark classes for specific treatments.
Let's consider this example:
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
@interface SpecializeFor {
String type();
}
@SpecializeFor(type = "Math")
public class MathProcessor {
public void operate(Integer value) {
// Math specialized operation
System.out.println("Squaring value: " + (value * value));
}
}
@SpecializeFor(type = "String")
public class StringProcessor {
public void operate(String value) {
// String specialized operation
System.out.println("Upper casing value: " + value.toUpperCase());
}
}
public class Main {
public static void main(String[] args) throws Exception {
processWithSpecialization(new MathProcessor(), 4);
processWithSpecialization(new StringProcessor(), "example");
}
private static void processWithSpecialization(Object processor, Object input) throws Exception {
SpecializeFor specializeFor = processor.getClass().getAnnotation(SpecializeFor.class);
if (specializeFor != null) {
System.out.println("Processing with specialization for: " + specializeFor.type());
processor.getClass().getMethod("operate", input.getClass()).invoke(processor, input);
} else {
System.out.println("No specialization found for " + processor.getClass().getSimpleName());
}
}
}
Review of the Code
In the above example, we create a custom annotation called @SpecializeFor
. Each processor class can be annotated with the type it specializes in. In Main
, we dynamically check for the annotation and invoke the appropriate processing method of the respective specialized class.
By leveraging reflection and annotations, we can dynamically decide how to process different data types while maintaining separation of concerns.
Final Considerations
Automating Java class specialization is a powerful method for enhancing performance. By combining generics, annotations, and meticulous design patterns, developers can create highly optimized applications that are easier to maintain and extend.
While the examples shared in this article are just a taste of what is possible, they illustrate the concept of specialization effectively. To delve deeper into Java optimization techniques, consider visiting resources such as Java Performance Tuning and Effective Java by Joshua Bloch.
By mastering these techniques, you can significantly improve the performance of your Java applications and thrive in the competitive landscape of software development. Start experimenting with class specialization today, and watch your applications soar!